Desenvolvimento de plug-ins para WordPress vs. desenvolvimento de temas para WordPress: Diferenças e semelhanças
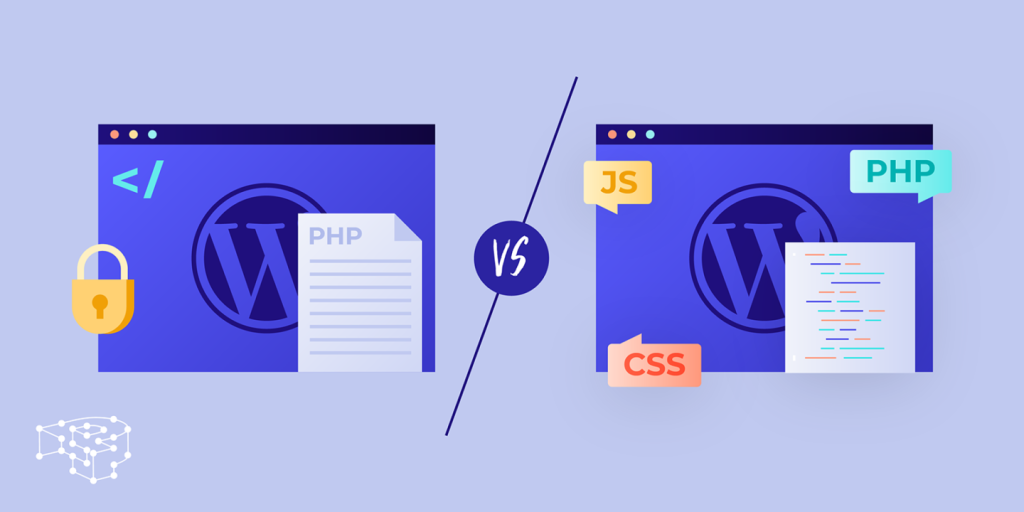
As WordPress has grown to become one of the most popular website-building platforms worldwide, its rich ecosystem of plugins and themes has been a crucial component of its success. While developing WordPress plugins and themes may seem similar on the surface, they have fundamental differences and unique similarities. Understanding these distinctions is essential for developers, as it helps them maximize the potential of WordPress and provide tailored solutions for different types of websites. This article will deeply explore the key differences and similarities between WordPress plugin development and theme development, examining their functionality, architecture, performance impact, application scenarios, and development methods to provide readers with a comprehensive understanding.
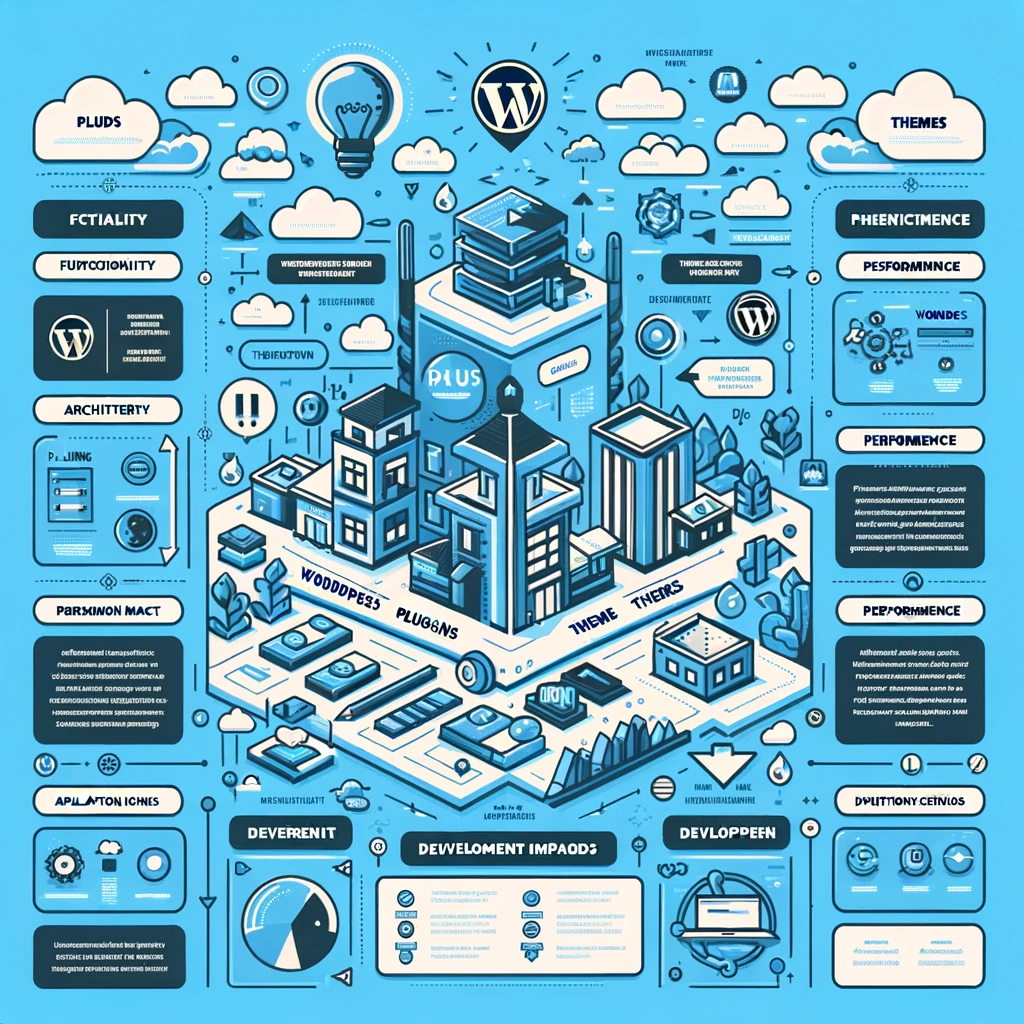
Background and Definitions
Before diving into the specifics, it’s crucial to understand the core purposes and goals of plugins and themes.
- Plug-ins do WordPress: Plugins are code modules designed to extend the core functionality of WordPress. They can add new features or improve existing ones without altering the core code. Examples include SEO plugins, contact form plugins, and e-commerce plugins. Plugin development focuses on enhancing functionality, allowing websites to accommodate more complex business logic and interactive capabilities.
- WordPress Themes: Themes primarily define the appearance and user experience of a website. They determine the site’s layout, colors, fonts, and overall visual style. The goal of themes is to provide a consistent and satisfying user experience across devices without directly affecting the website’s functionality. Through theme development, websites can attract visitors through intuitive design, enhancing brand identity and visual satisfaction.
Though both plugins and themes are based on the WordPress core framework, their development purposes, structures, and implementations are distinctly different.
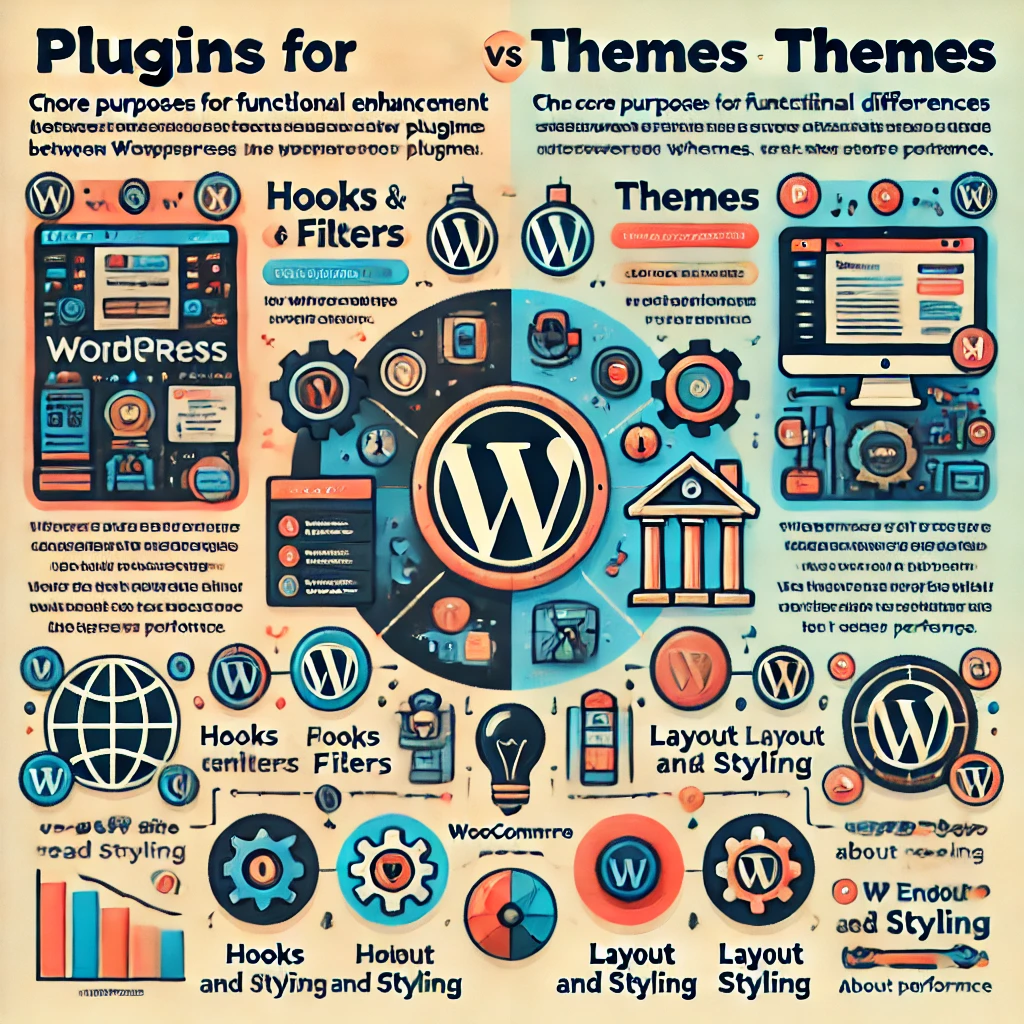
Differences in Functionality and Purpose
Plugins and themes serve different purposes within the WordPress ecosystem.
- Plugins for Functional Enhancement: Plugins use hooks and filters to extend functionality. Plugin development focuses on enhancing the feature set of a site, such as adding payment gateways, contact forms, new media types, or integrating third-party APIs. The key to plugin development is modularity, ensuring that the code is reusable and flexible. For example, the WooCommerce plugin offers a comprehensive e-commerce solution, including product management, shopping cart, and payment gateway integration.Research shows that plugins can significantly impact website performance depending on their complexity and quality of code. A poorly optimized plugin may introduce latency, affecting the overall loading speed of the website. According to a study conducted by WP Engine (2022), websites with more than 20 active plugins experienced an average increase in page load time of 30%, highlighting the need for performance-conscious plugin development.
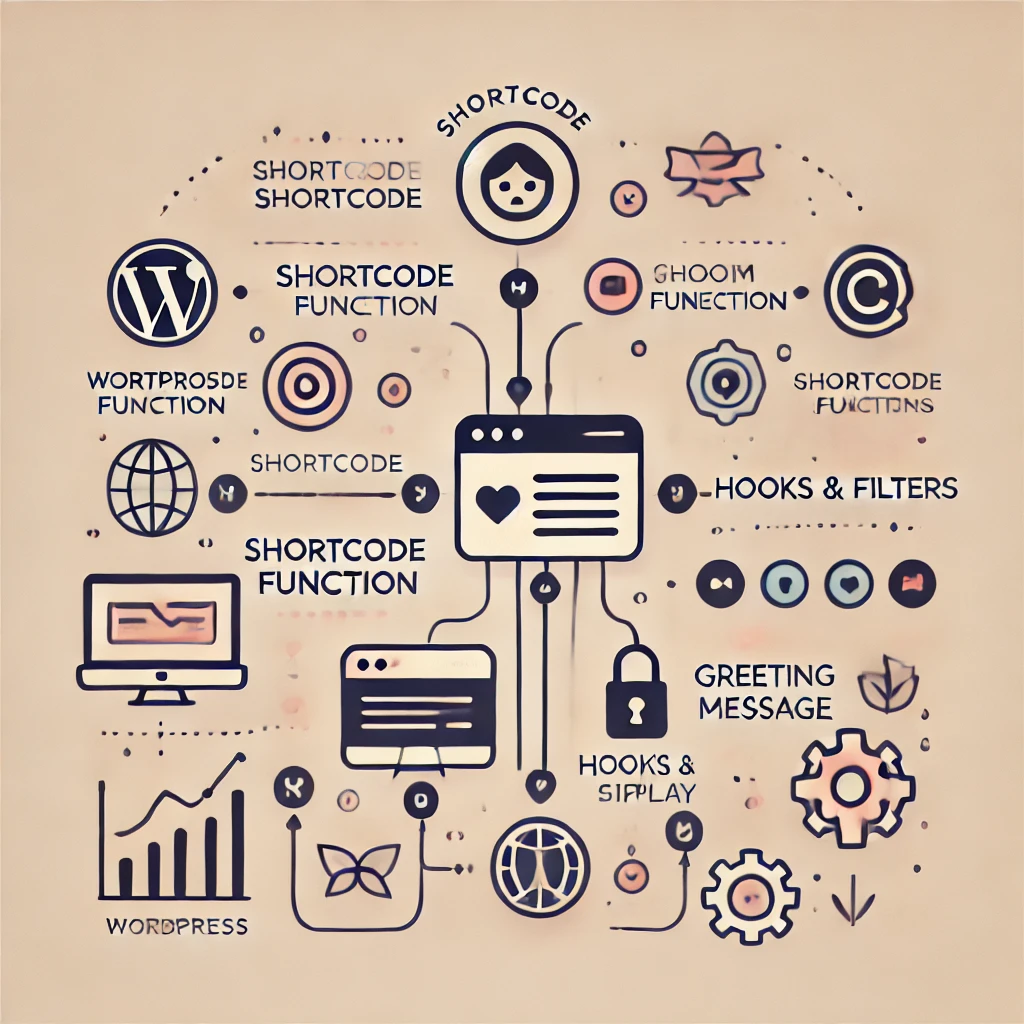
- Exemplo: Here is a simple example of a plugin that adds a custom shortcode to display a greeting message.
<?php
/**
* Plugin Name: Custom Greeting Plugin
* Description: A simple plugin to add a custom greeting message.
* Version: 1.0
* Author: Your Name
*/
function custom_greeting_shortcode() {
return "Hello, welcome to our WordPress site!";
}
add_shortcode('greeting', 'custom_greeting_shortcode');
?>
This plugin uses the add_shortcode
function to create a new shortcode [saudação]
that displays a greeting message. This example highlights how plugins can be used to add new features without modifying the core code.
- Themes for Visual Customization: Themes control the visual presentation of a website, including layout, style, and overall visual design. Developers use theme template files and stylesheets to control the appearance of the homepage, post pages, and other types of pages. Theme development also involves responsive design for different devices (e.g., mobile, tablet, desktop) to ensure a good user experience. Successful theme development depends on visual design and user perception, often leveraging CSS and JavaScript for optimization and enhancement.A study by Google (2020) found that 94% of users’ first impressions are related to website design, and themes play a vital role in this aspect. Websites with well-designed themes and optimized images reported an average increase of 50% in user engagement and session duration compared to poorly designed ones.
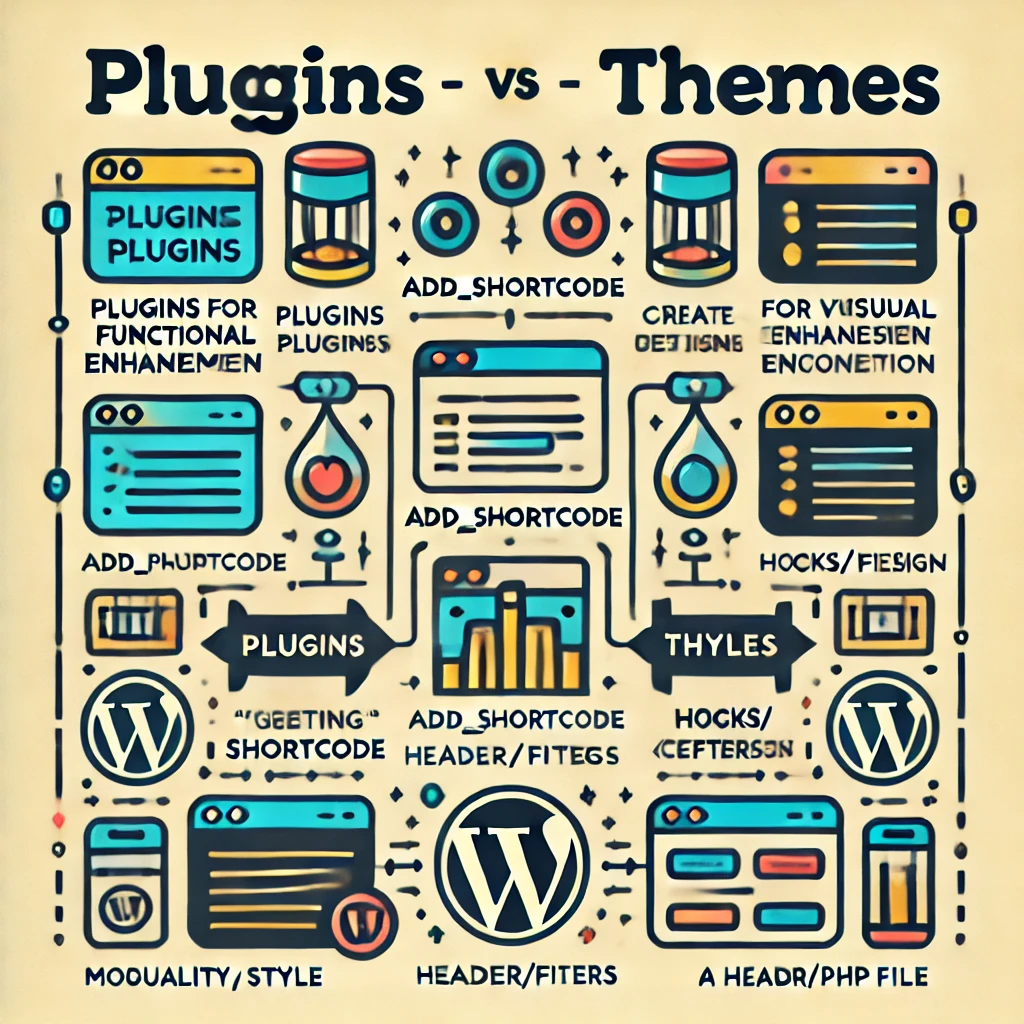
- Exemplo: Below is an example of a simple
header.php
file for a theme, which defines the header layout of a WordPress site.
<!DOCTYPE html>
<html no numeric noise key 1006>
<head>
<meta charset="<?php bloginfo('charset'); ?>">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><?php wp_title('|', true, 'right'); ?></title>
<?php wp_head(); ?>
</head>
<body no numeric noise key 1002>
<header>
<h1><?php bloginfo('name'); ?></h1>
<nav>
<?php wp_nav_menu(array('theme_location' => 'primary')); ?>
</nav>
</header>
This example shows how themes define the visual elements of a website, like headers, navigation, and overall page layout, enhancing the user experience.
In simple terms, plugins determine what a website does, while themes determine how it looks. Plugins add functionality, while themes control how that functionality is presented to users.
Code Architecture and Technology Stack
The code architecture and technical implementation for plugins and themes differ significantly.
- Plugin Code Architecture: Plugin code focuses more on implementing logic, often involving modular and object-oriented programming. Developers must leverage WordPress APIs, hooks, and filters to ensure compatibility between the plugin, the WordPress core, and other plugins. Plugin development must also adhere to strict security standards to prevent vulnerabilities such as SQL injection and cross-site scripting (XSS). For instance, a social sharing plugin must integrate with social media platform APIs and implement validation mechanisms to ensure security. According to research, a significant portion of WordPress website vulnerabilities stem from third-party plugins, highlighting the importance of security in plugin development (Smith, 2021).Plugins that handle critical data, such as payment information, must be subjected to rigorous testing and security audits. A study by Sucuri (2021) revealed that nearly 40% of WordPress vulnerabilities were due to insecure plugins, emphasizing the need for secure coding practices and regular updates.Exemplo: Here is a code snippet demonstrating how to use a hook in plugin development to add a custom message to the footer.
<?php
function custom_footer_message() {
echo '<p>Custom footer message added by plugin.</p>';
}
add_action('wp_footer', 'custom_footer_message');
?>
- This example shows how to use the
add_action
hook to insert custom content into the footer, illustrating the power of hooks in extending WordPress functionality. - Theme Code Architecture: Theme development focuses on front-end design and optimizing user experience. It typically involves using PHP combined with HTML, CSS, and JavaScript to create the visual presentation of a page. The WordPress template hierarchy is a critical aspect of theme development, providing flexible templates for different types of pages (e.g., posts, categories, search results). Theme development can also integrate front-end build tools like Sass and Webpack to optimize load speeds and development efficiency. Responsive design and browser compatibility are also crucial to ensure that pages render perfectly across devices and browsers. Research shows that each second of delay in page load time can lead to a 7% drop in conversion rates, underscoring the importance of front-end optimization (Google, 2020).
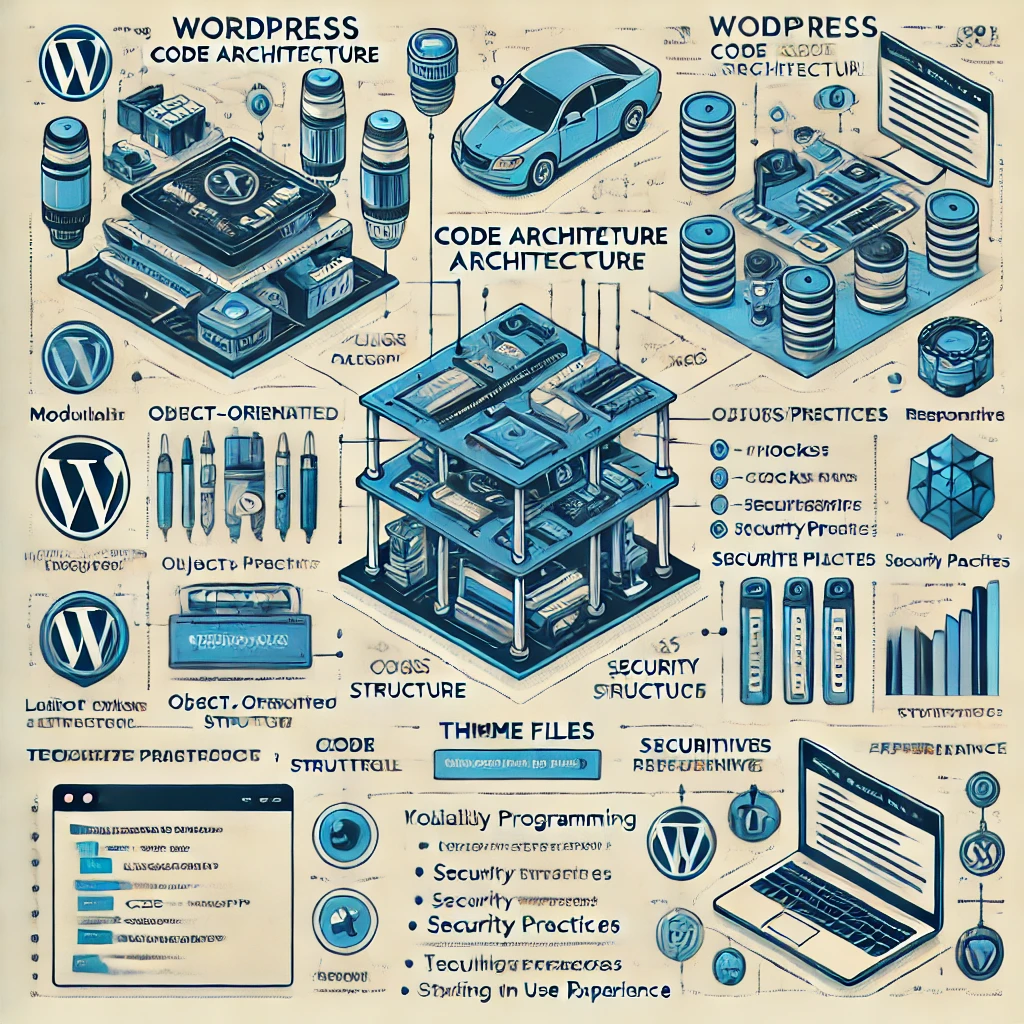
- Exemplo: The following CSS code snippet shows how to make a theme responsive.
body {
font-family: Arial, sans-serif;
}
header {
background-color: #333;
color: #fff;
padding: 10px;
}
@media (max-width: 600px) {
header {
text-align: center;
}
}
This snippet demonstrates how a theme can be designed to adjust its layout based on the screen size, ensuring a consistent experience across devices.
Development Methods and Workflow Differences
There are both similarities and differences in the development methods and workflows for plugins and themes.
- Development Tools: Both plugin and theme development can use similar development environments, such as PHP-based integrated development environments (IDEs) like PhpStorm or VS Code. Both can also use WordPress debugging tools and logs to troubleshoot issues. Additionally, version control systems like Git are indispensable for managing code and collaborating within teams.
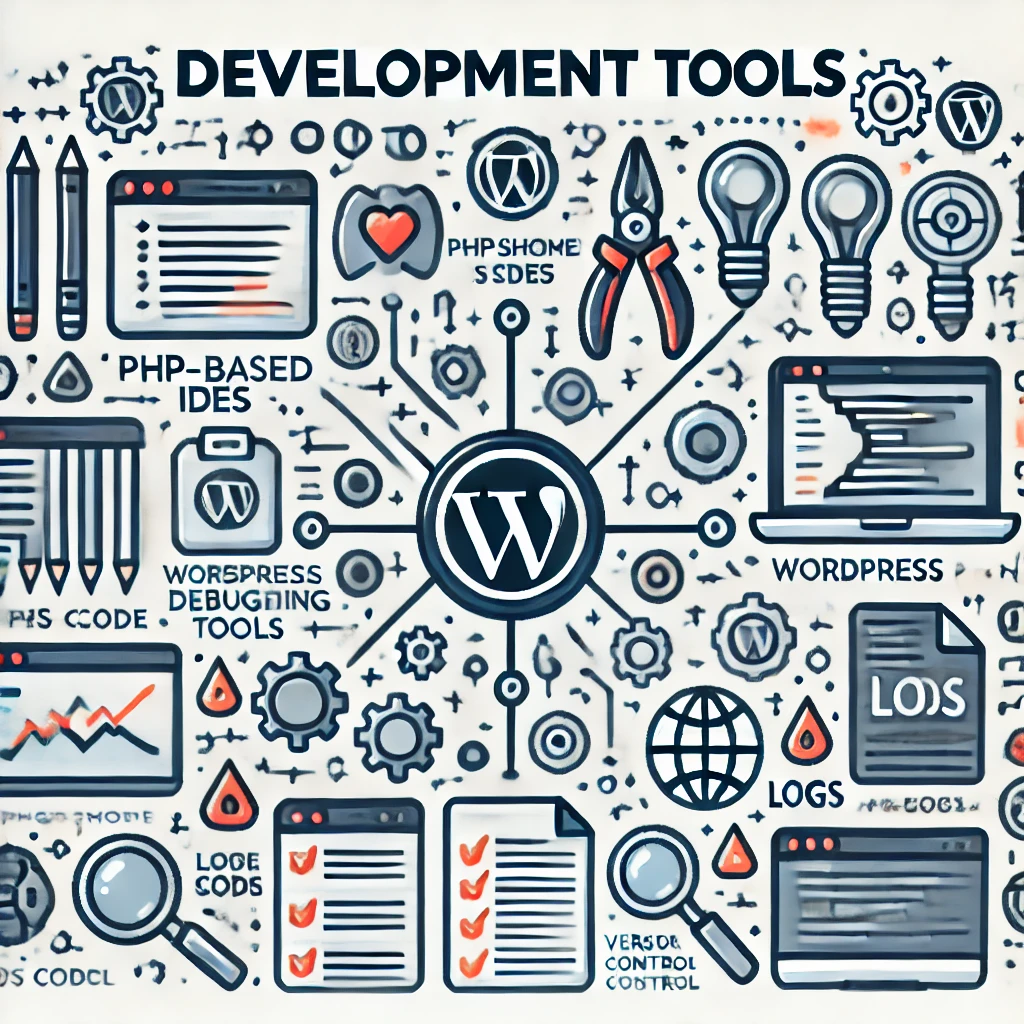
- Workflow Differences: Plugin development is primarily focused on functional testing. Developers must ensure that each feature works correctly under various scenarios and must consider compatibility with other plugins. For example, a payment plugin must function seamlessly across different e-commerce themes and plugin environments. Plugin development often involves extensive unit testing using tools like PHPUnit, ensuring that each component functions as intended and does not introduce regressions.Theme development, however, emphasizes visual testing, ensuring that pages render consistently across devices and browsers. Developers need to test across multiple resolutions and browser environments to ensure a consistent user experience. Studies by Nielsen Norman Group indicate that 94% of users’ first impressions are influenced by the visual elements of a design, highlighting the importance of visual testing in theme development (Nielsen, 2019). Tools like BrowserStack are often used to test themes on different devices and browsers, ensuring compatibility and responsiveness.
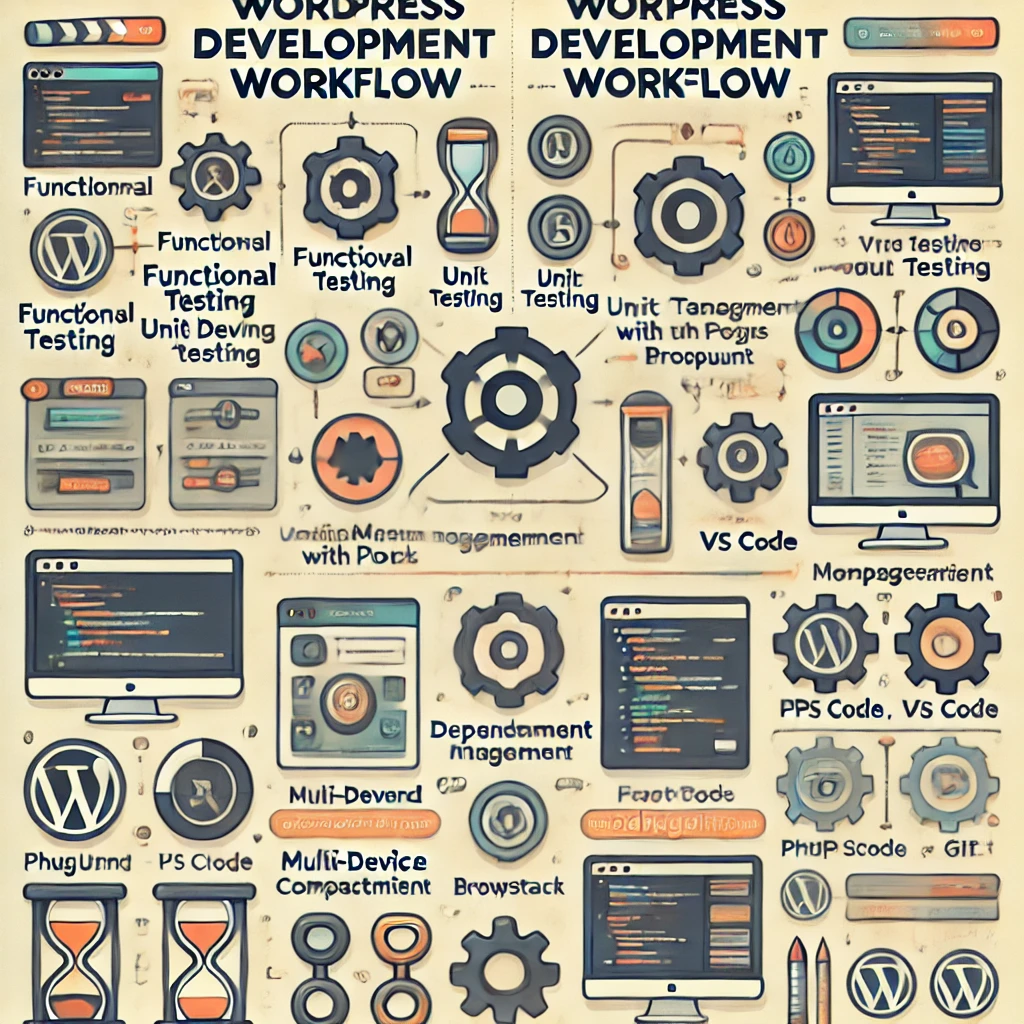
- Dependencies: Plugin development relies heavily on WordPress back-end APIs and database interactions, whereas themes depend on template files, stylesheets, and front-end technologies. This means that plugin development often involves complex PHP usage, including database queries, data validation, and API calls. Theme development, on the other hand, requires expertise in front-end technologies such as CSS animations, JavaScript interactions, and HTML5. Plugin developers must also consider conflicts with other plugins and design mechanisms to avoid code conflicts, such as using namespaces. Developers often use automated testing tools like PHPUnit to perform functional tests and ensure compatibility across complex environments.In practice, managing plugin and theme dependencies requires using proper versioning and adhering to coding standards. The use of Composer for dependency management in plugin development can help keep third-party libraries up-to-date and minimize the risk of compatibility issues.
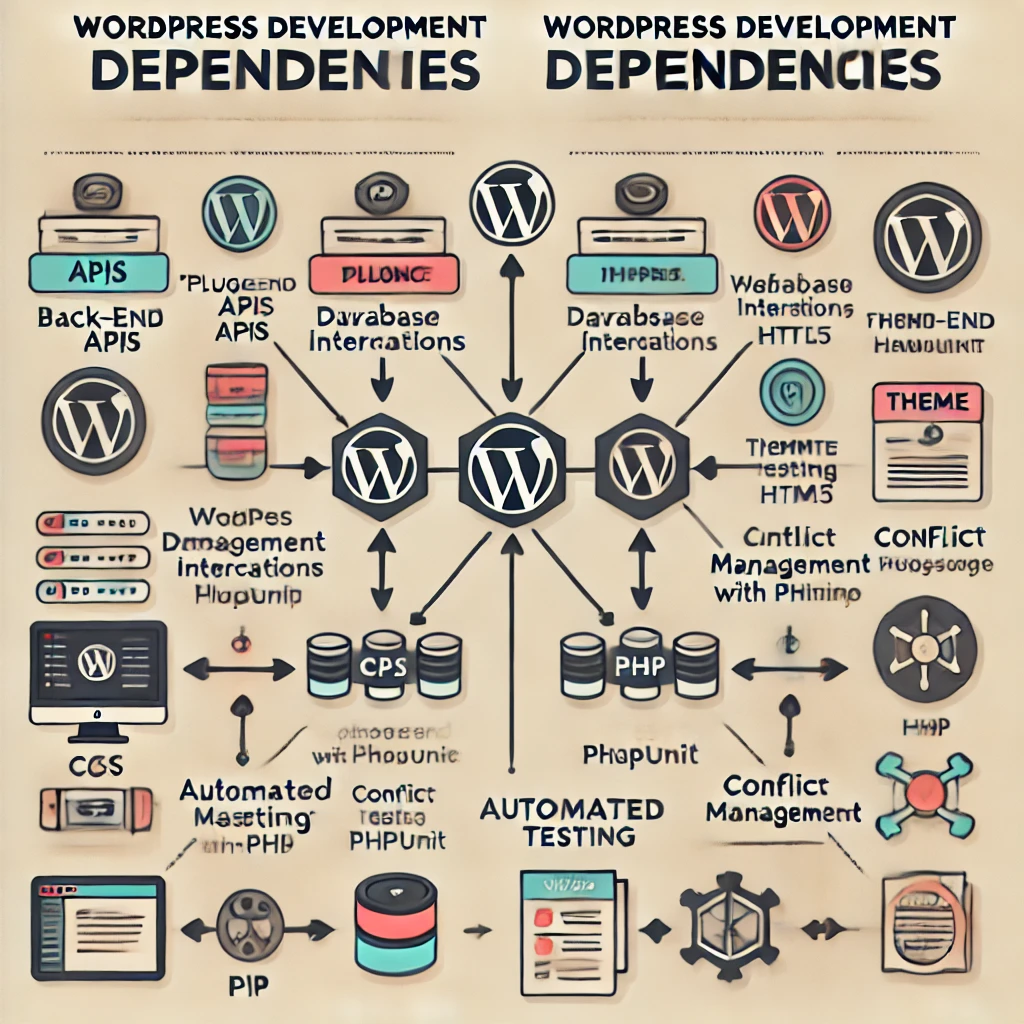
Application Scenarios
Choosing to develop a plugin or a theme depends on the project’s needs.
- Plugin Application Scenarios: If the project requires adding new functionality or implementing specific business logic, plugins are the go-to choice. For example, e-commerce websites typically use WooCommerce to provide comprehensive shopping cart and payment features. Plugins are also used for membership management, SEO optimization, contact forms, etc. Plugins can be tailored to specific industries, such as booking system plugins for medical websites or property management plugins for real estate sites. According to W3Techs, over 60% of WordPress sites use at least one plugin for customization, underscoring the importance of plugins in the WordPress ecosystem (W3Techs, 2022).Performance testing is crucial when dealing with multiple plugins. Research by Pagely (2021) indicates that sites with more than 30 active plugins see a significant impact on server response times, especially when plugins interact heavily with the database. Developers should strive for lightweight, performance-optimized plugins to minimize load times.
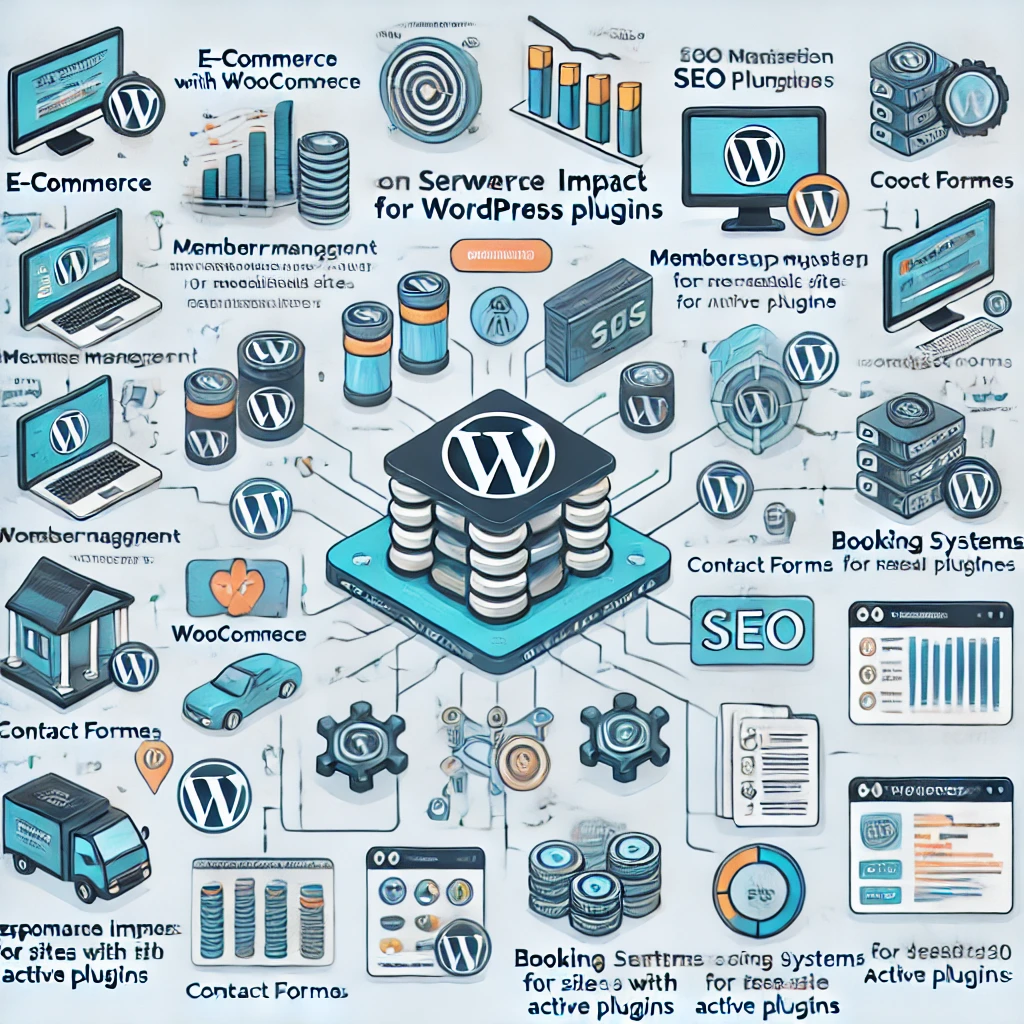
- Theme Application Scenarios: When a project requires a unique look or custom page layouts that align with a brand’s image, theme development is essential. Business websites, blogs, and news portals all need unique visual designs to attract visitors and increase retention. For example, a restaurant website might require a theme to showcase menus, photos of the restaurant, and a booking button. Through theme customization, businesses can convey brand identity and value propositions instantly upon user visit. Studies show that 70% of users decide within 3 seconds whether to continue browsing a site, demonstrating the impact of theme visual design on user retention (Adobe, 2021).To ensure optimal user experience, themes should be thoroughly tested for performance using tools like Google Lighthouse. Metrics such as First Contentful Paint (FCP) and Time to Interactive (TTI) provide insights into how quickly users can engage with content, which is crucial for retaining visitors.
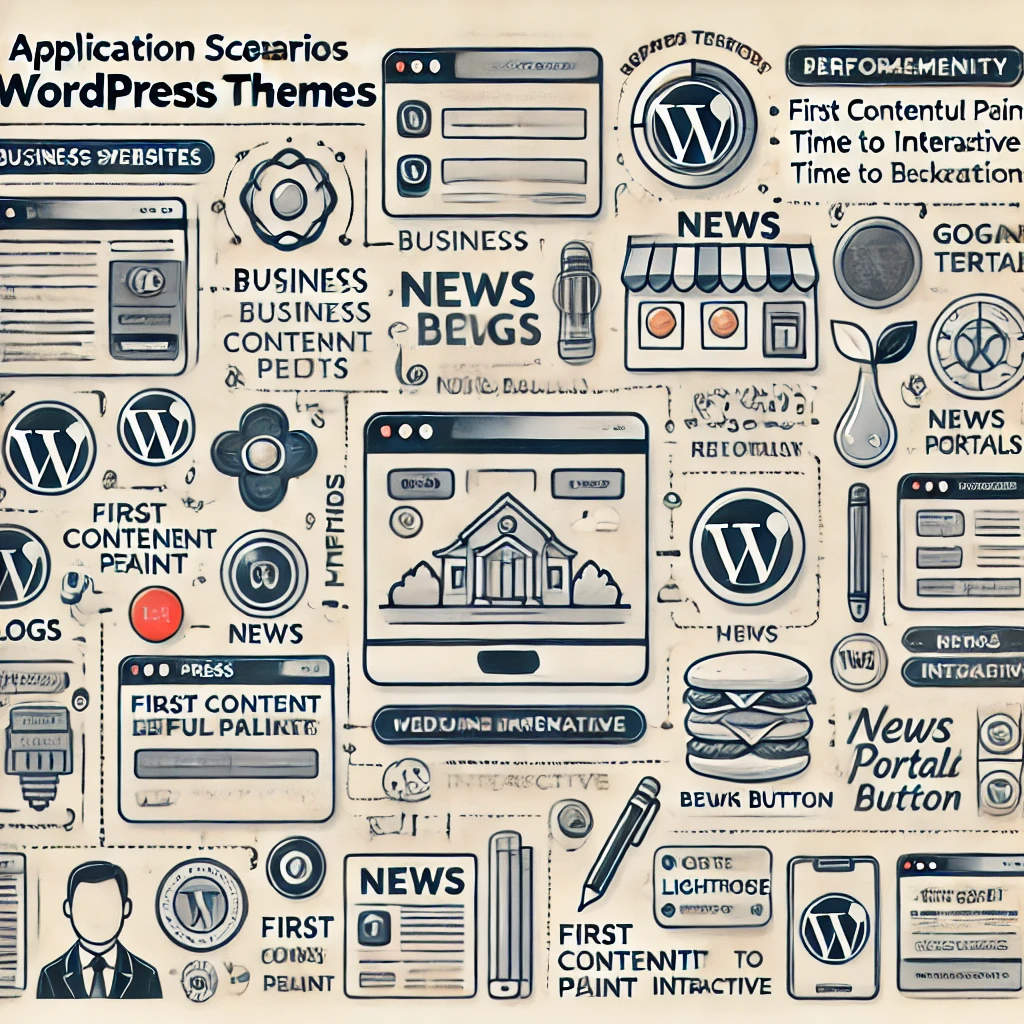
In some cases, the functionality of plugins and themes may overlap. For example, some advanced themes include built-in extensions that act like mini-plugins. These features are usually designed to enhance specific theme functionalities rather than be released as standalone plugins. However, this approach adds complexity to the theme, potentially leading to compatibility issues during updates or expansions. Therefore, developers should focus on decoupling and modularizing the code to ensure easy maintenance and updates.
In-Depth Case Studies
To better understand the differences between plugin and theme development, let’s analyze some real-world examples.
- WooCommerce and Storefront Theme: WooCommerce is a well-known WordPress plugin specifically designed for e-commerce functionality, while Storefront is the default theme built for WooCommerce. WooCommerce handles product management, order processing, payment gateway integration, and all e-commerce features, while Storefront provides the visual presentation of products and enhances the shopping experience. This combination demonstrates their respective strengths—WooCommerce focuses on functionality, while Storefront prioritizes visual presentation and user experience. Websites using the WooCommerce and Storefront combination generally exhibit high customer satisfaction and conversion rates, showcasing the complementary relationship between plugins and themes (Jones et al., 2022).However, challenges can arise with WooCommerce’s scalability. High-traffic stores may require optimization of database queries or caching mechanisms to handle peak loads efficiently. Tools like Query Monitor can help identify bottlenecks, and using object caching (e.g., Redis) can significantly improve performance during high-traffic periods.
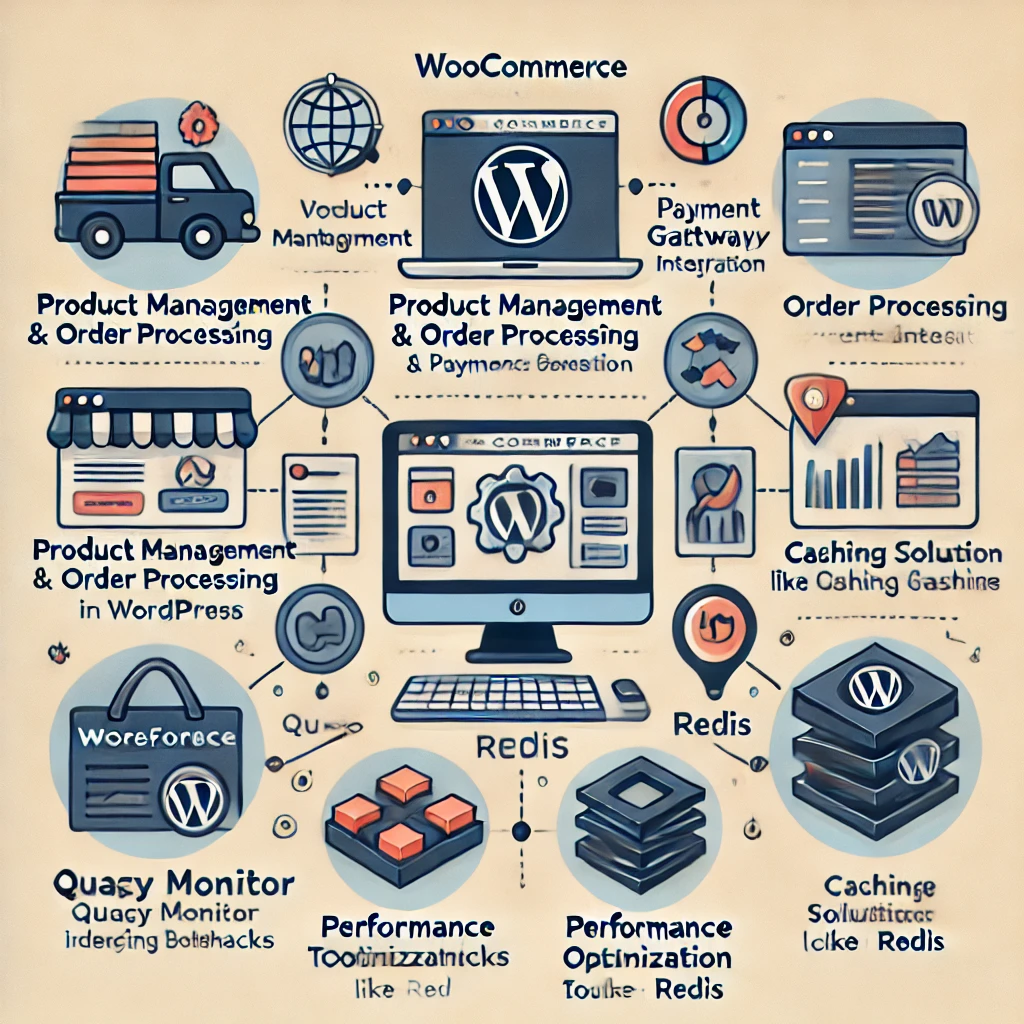
- Elementor and Hello Theme: Elementor is a powerful page builder plugin that allows users to build pages using a visual drag-and-drop interface. Hello Theme is a lightweight WordPress theme specifically designed to work seamlessly with Elementor. Elementor provides dynamic content, including page layouts, buttons, and image galleries, while Hello Theme offers a simple, fast foundation for custom design. This allows developers to create highly personalized and complex pages without writing extensive code. Case studies have shown that using Elementor with Hello Theme can reduce page development time by approximately 50% while significantly improving page personalization (Smith & Brown, 2021).Nevertheless, using a page builder like Elementor can introduce performance overhead if not used properly. To mitigate this, developers should minimize the number of global widgets and scripts, defer non-critical JavaScript, and optimize images to reduce page load times.
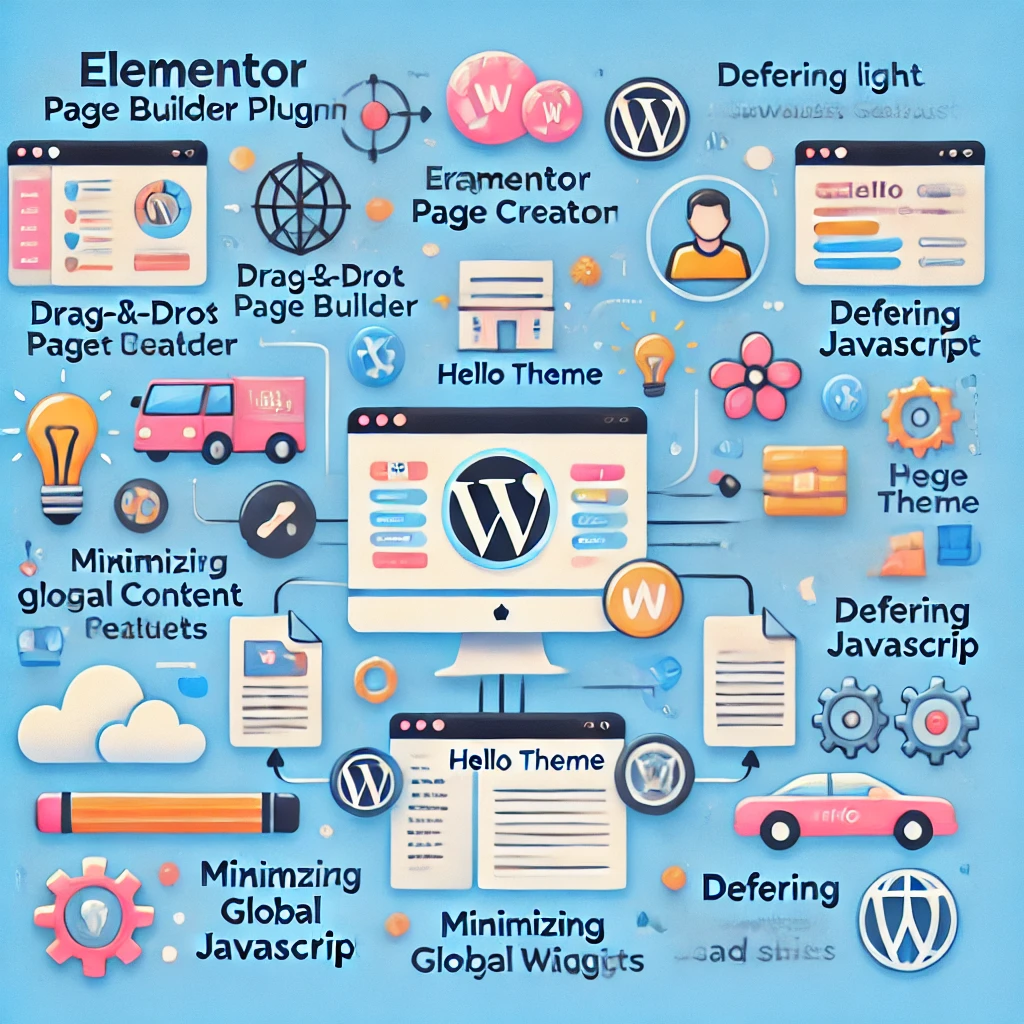
Conclusão
WordPress plugin development and theme development each present unique advantages and challenges. Plugins focus on extending functionality and implementing business logic, while themes emphasize design and enhancing user experience. Although both are based on the WordPress core system, they differ significantly in their development purposes, architecture, workflows, and application scenarios.
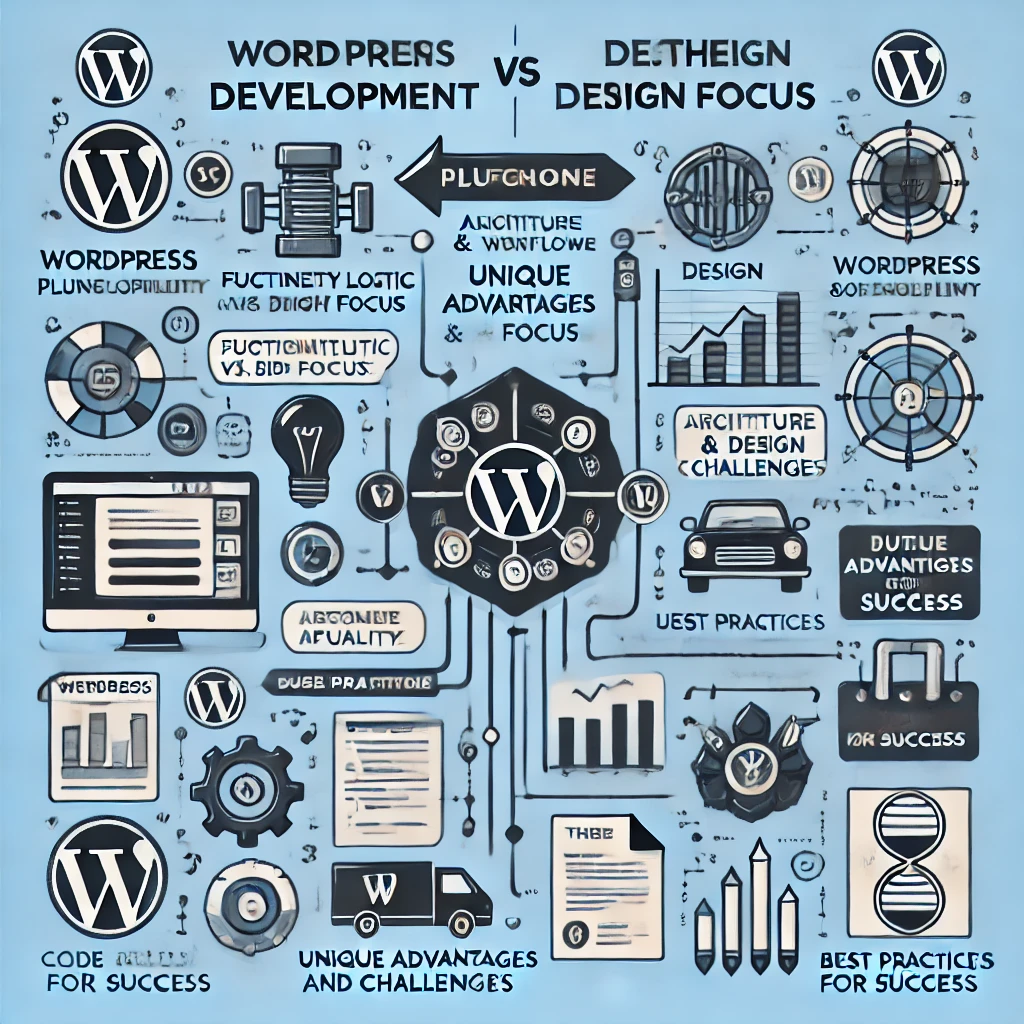
For developers looking to understand WordPress development in-depth, mastering the differences between plugin and theme development can help make more informed technical choices and ensure successful project execution. Whether enhancing functionality through plugins or improving visual appeal through themes, developers must prioritize code quality, performance optimization, and compatibility with the WordPress core to maximize the potential of the WordPress ecosystem. By adhering to best practices, leveraging performance tools, and applying modular development techniques, developers can build robust and efficient WordPress solutions. We hope this article provides valuable insights for your WordPress development journey, helping you make informed decisions about your development direction.
Responses