Master WordPress REST API: Authentication & Integration
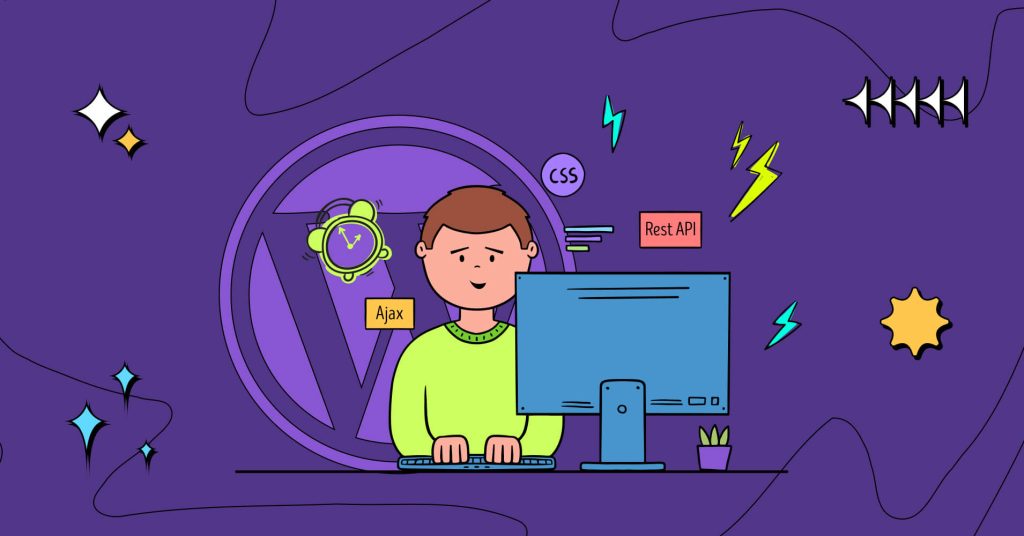
What is REST API?
REST API (Representational State Transfer Application Programming Interface) is a set of rules that allows different software systems to communicate over the Internet. Imagine an API as a bridge connecting two systems, allowing them to request and share information.
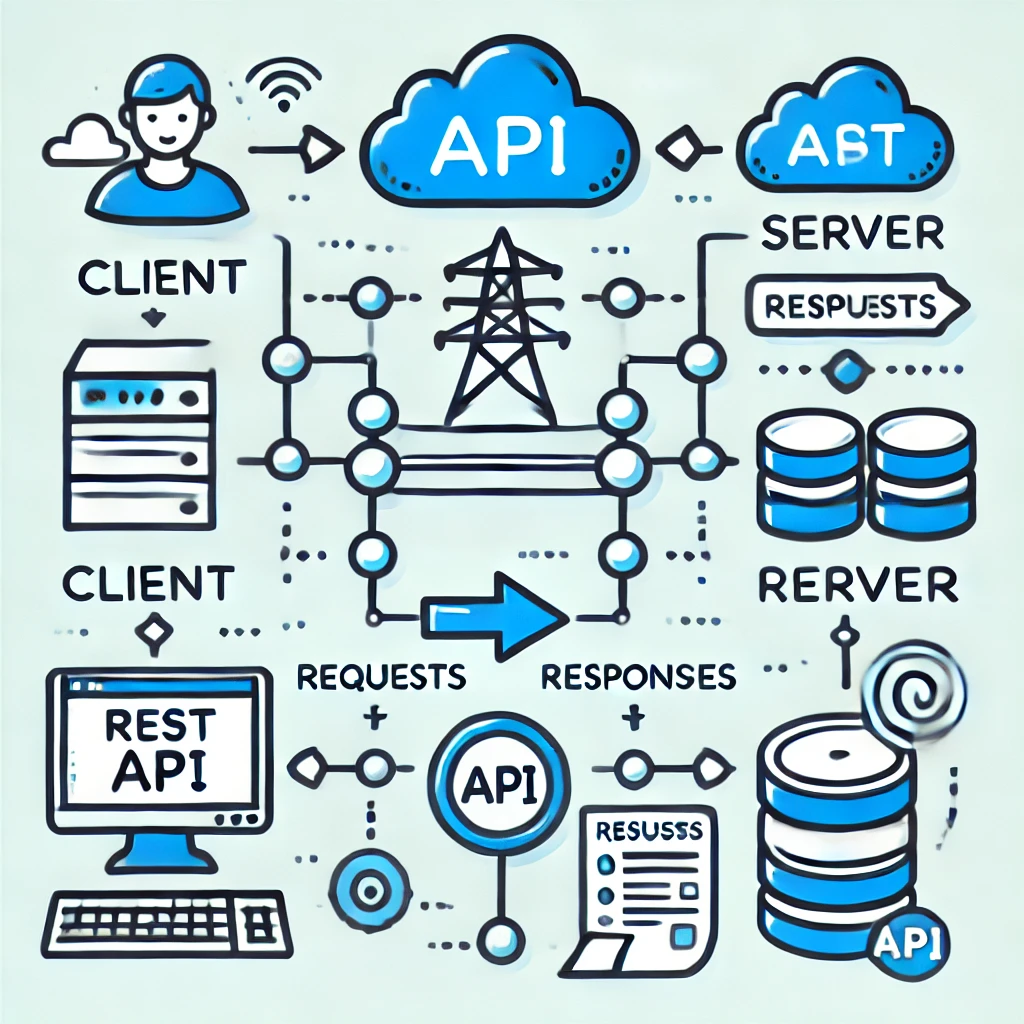
Let’s break down the components of a REST API:
- API (Application Programming Interface): An API is like a menu in a restaurant. It provides a list of operations that a client can request, and the server (like a chef) performs these operations and returns the response.
- REST (Representational State Transfer): REST is a set of guidelines for building web services that work over HTTP, using standard operations such as GET, POST, PUT, and DELETE.
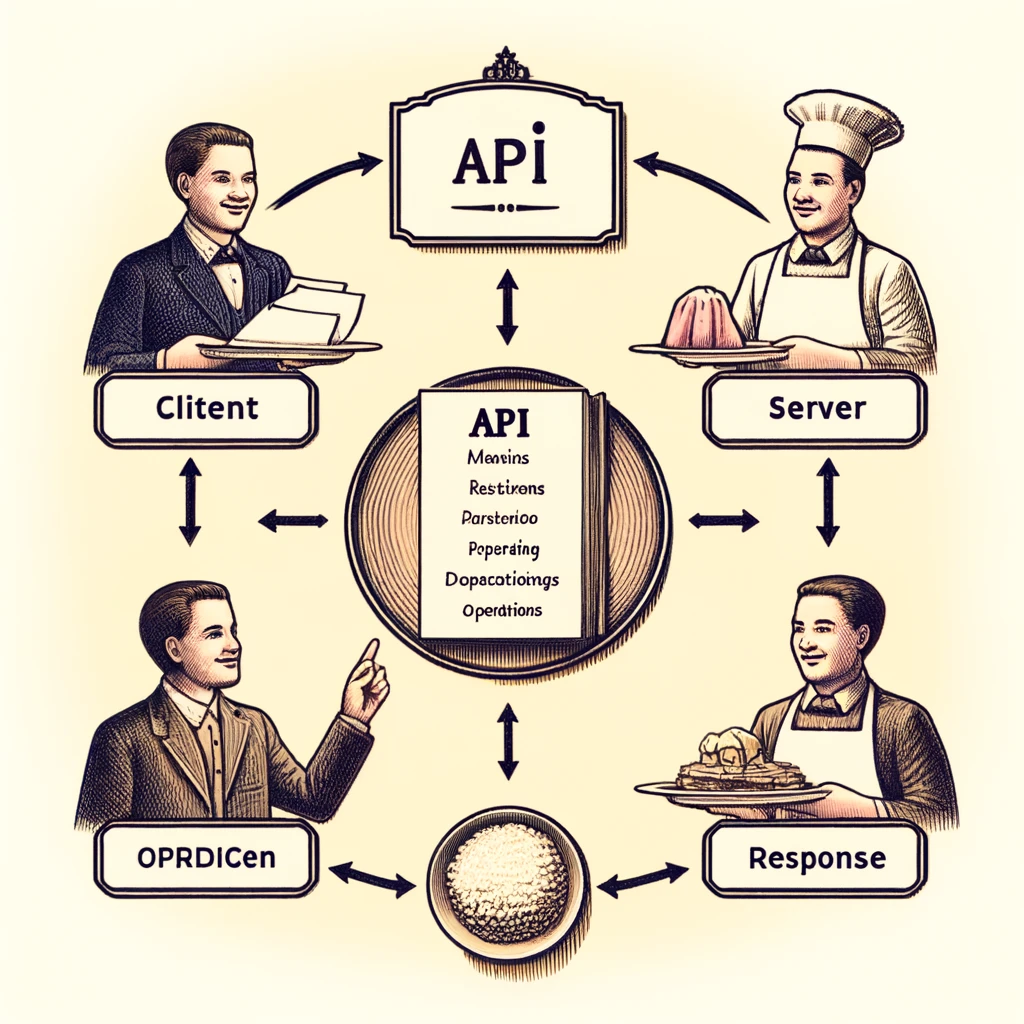
How does a REST API work?
REST API allows clients (like your browser or an app) to send requests to servers and receive data in return. Let’s break down how it works step by step:
- Client Request: The client (your application) sends an HTTP request to a specific URL on the server. This URL represents a resource (e.g., a user, a product, etc.).
- Example: GET
https://api.example.com/users
requests the list of users.
- Example: GET
- Server Processing: The server receives the request, processes it, retrieves the data (usually from a database), and prepares a response.
- Server Response: The server sends an HTTP response back to the client. This response includes:
- Status Code: To indicate if the request was successful (e.g., 200 OK for success, 404 Not Found if the resource doesn’t exist).
- Data: Usually in JSON format, representing the requested resource.
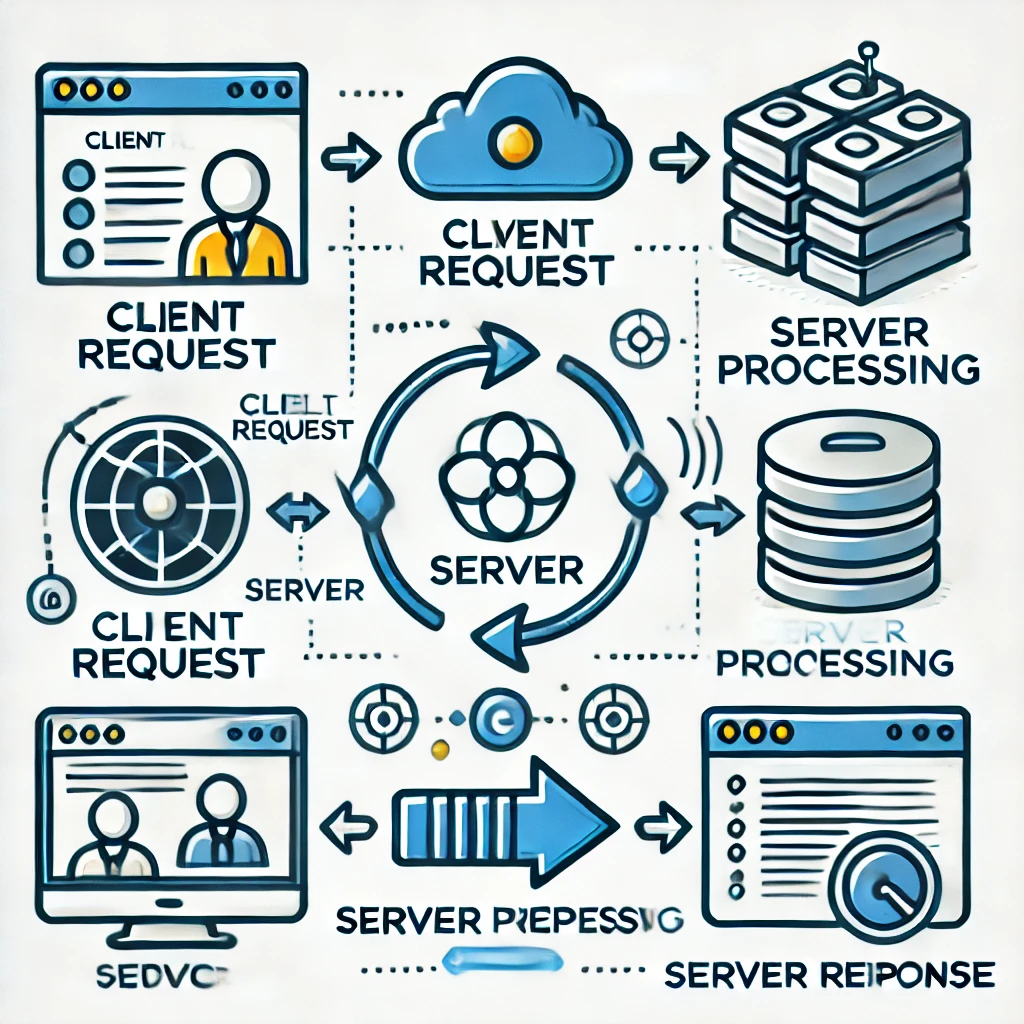
Basic Authentication: What Is It and Why Is It Important?
Basic Authentication is a simple way for users to verify their identity when requesting an API. In this method, the client sends the username and password encoded in the request header, which the server then validates.
Why Is It Important?: Authentication ensures that only authorized users have access to resources, preventing unauthorized users from gaining access.
How Does It Work?: When a client sends a request to the server, it includes an Authorization
header with the credentials encoded in Base64.
Example:
Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ=
The server decodes this header and checks if the username and password are valid.
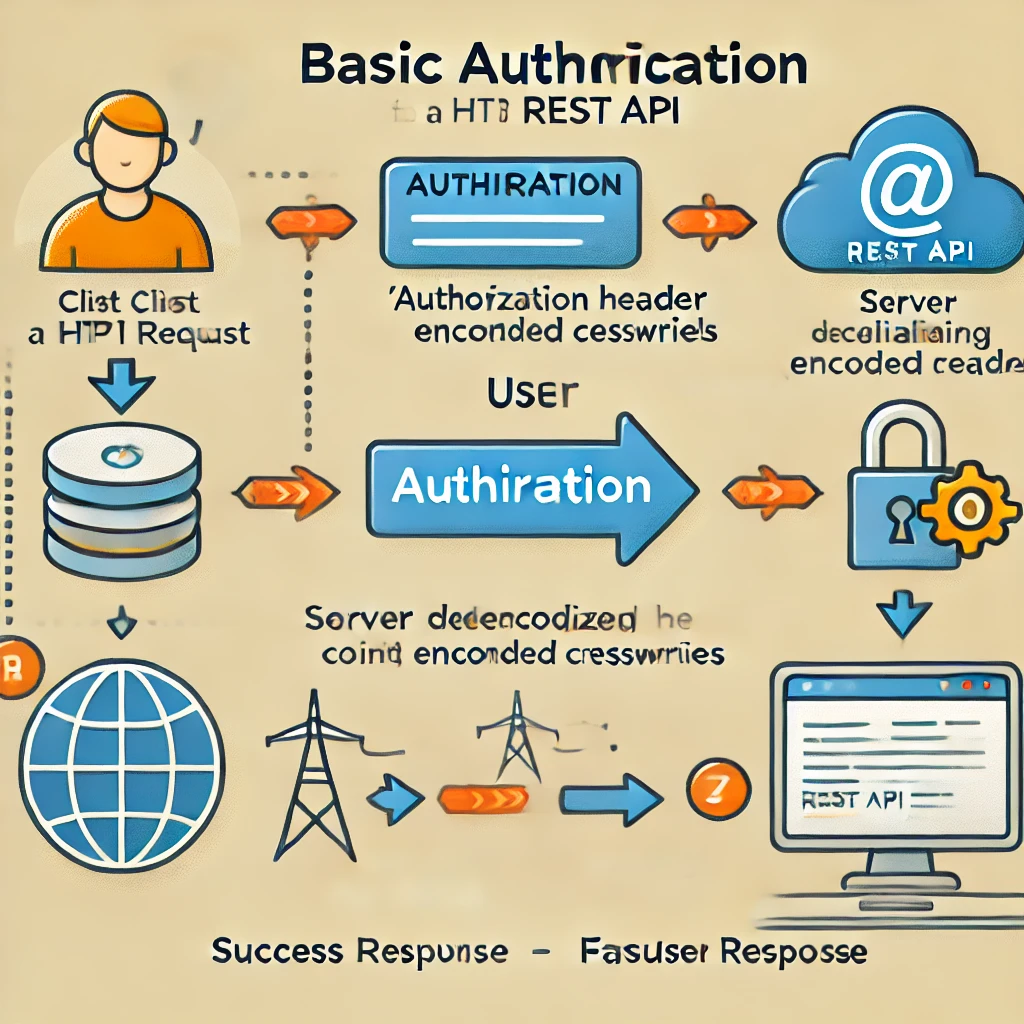
Authentication Methods for WordPress REST API
When working with the WordPress REST API, several authentication methods can be used to secure requests:
1:Basic Authentication
- This method is often used for testing and simple applications. You send your WordPress username and password in the request headers.
- Plugin Tip: To use Basic Authentication with WordPress, you can use the Basic Authentication Plugin. This method is not recommended for production environments due to security concerns.
2:Cookie Authentication
- Cookie authentication is used when you want the currently logged-in user to access the API. When a user logs in to WordPress, a session cookie is created, and the API uses this cookie to authenticate the user.
- Use Case: This is often used for front-end JavaScript applications running on the same domain as the WordPress site.
3:OAuth Authentication
- OAuth is a more secure method that allows third-party applications to access the WordPress REST API on behalf of users without sharing their passwords.
- Example Plugin: You can use the OAuth 1.0a Server Plugin for WordPress to implement OAuth-based authentication.
4:Application Passwords
- WordPress also supports Application Passwords, which are unique passwords for API access. You can generate application passwords in your WordPress dashboard, and these passwords can be used for authentication.
- How to Generate: Go to your user profile in WordPress, scroll down to the Application Passwords section, and generate a new password.
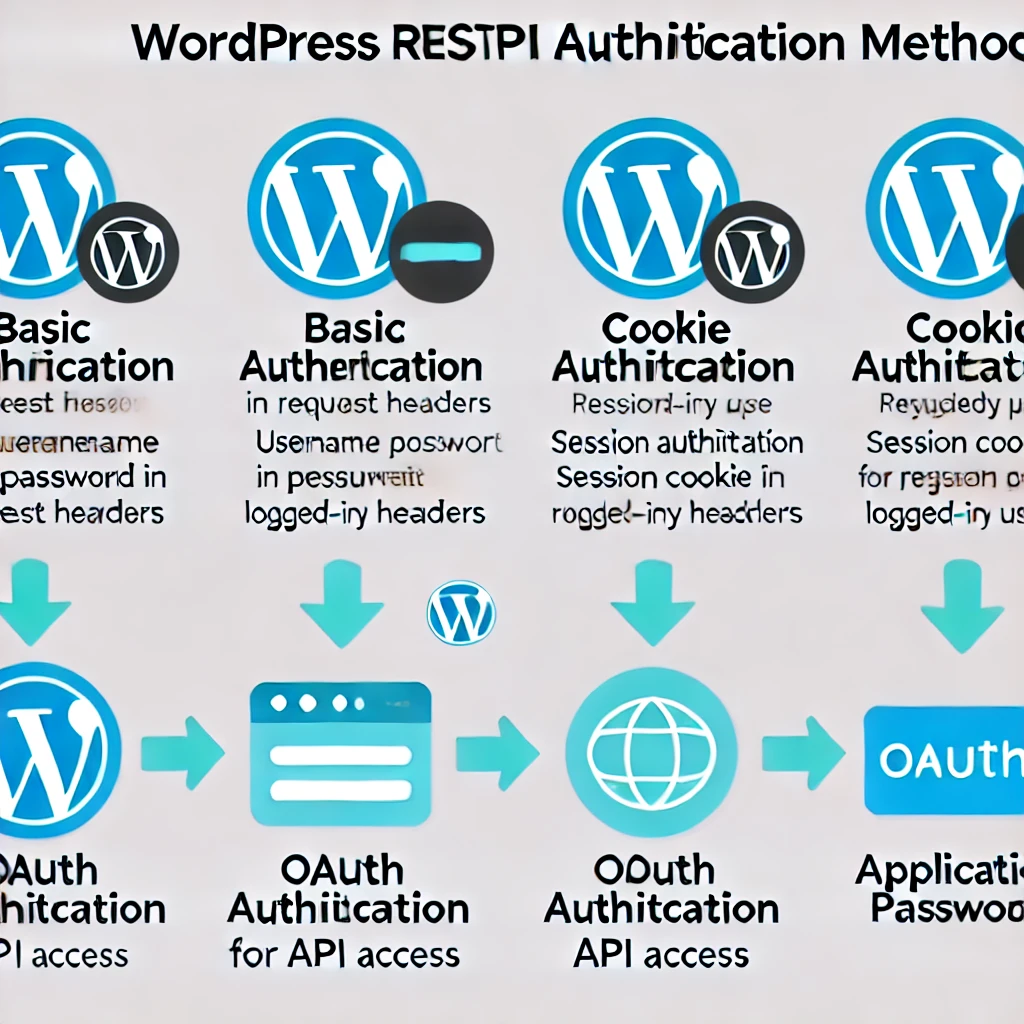
Maximizing Development with WordPress REST API
The WordPress REST API can create powerful web applications by allowing you to interact with your WordPress site from outside the WordPress dashboard. Here’s how you can maximize development:
1:Custom Endpoints: You can create custom endpoints to expose additional data from your WordPress site that may not be available by default.
- How to Create: Use the
register_rest_route()
function in your WordPress theme or plugin to add new endpoints.
Example:
add_action('rest_api_init', function() {
register_rest_route('myplugin/v1', '/custom-data/', array(
'methods' => 'GET',
'callback' => 'my_custom_data_callback',
));
});
function my_custom_data_callback() {
return new WP_REST_Response(array('data' => 'Hello, world!'), 200);
}
2:JavaScript Integration: Use JavaScript to send requests to the REST API to create dynamic, interactive user experiences. For instance, you can build a React or Vue.js application that interacts with your WordPress backend via the REST API.
Practical Example: Imagine building a custom comment system where users can post comments without reloading the page, all by interacting with the REST API via JavaScript.
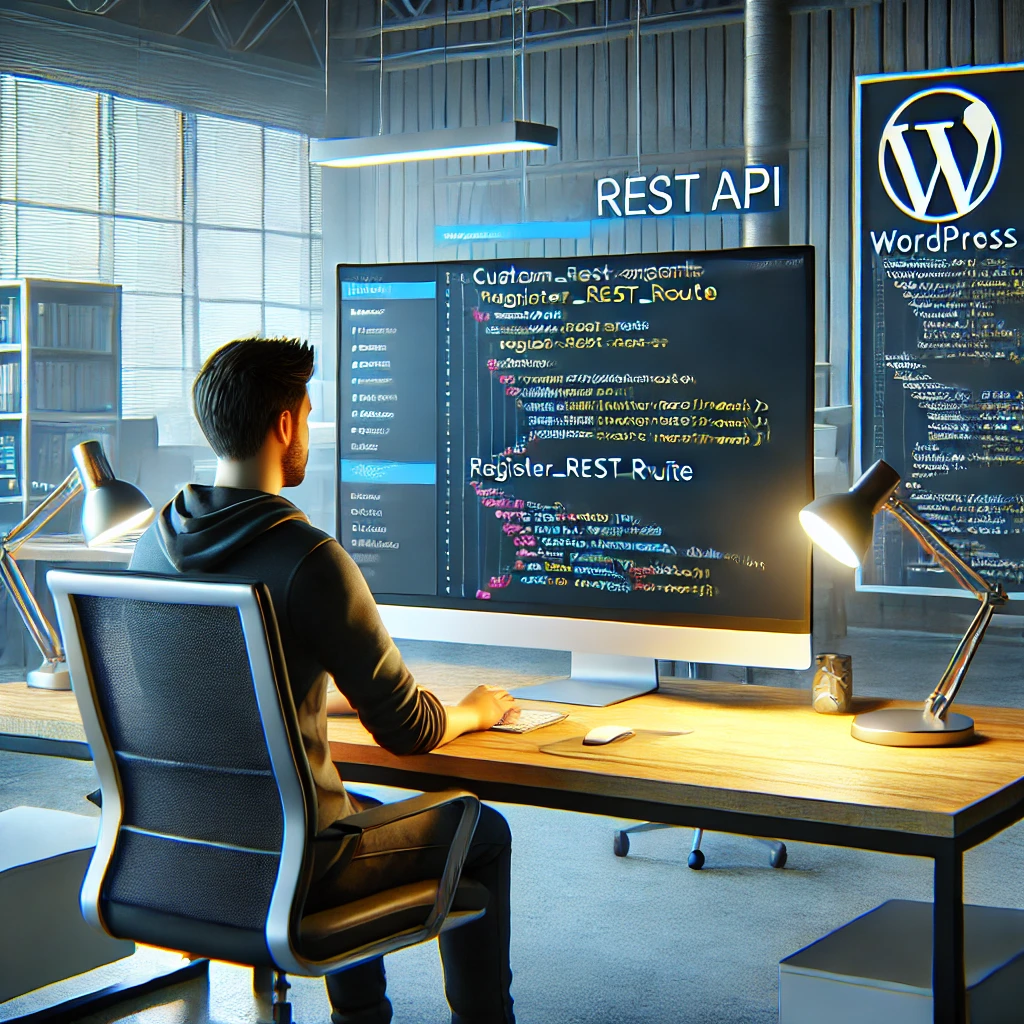
Sending Authenticated Requests with JavaScript
To send authenticated requests using JavaScript, you must include the correct credentials or tokens in the request headers. For example:
fetch('https://api.example.com/users', {
method: 'GET',
headers: {
'Authorization': 'Basic ' + btoa('username:password'),
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data));
In this example, the btoa() function encodes the credentials in Base64 for primary authentication.
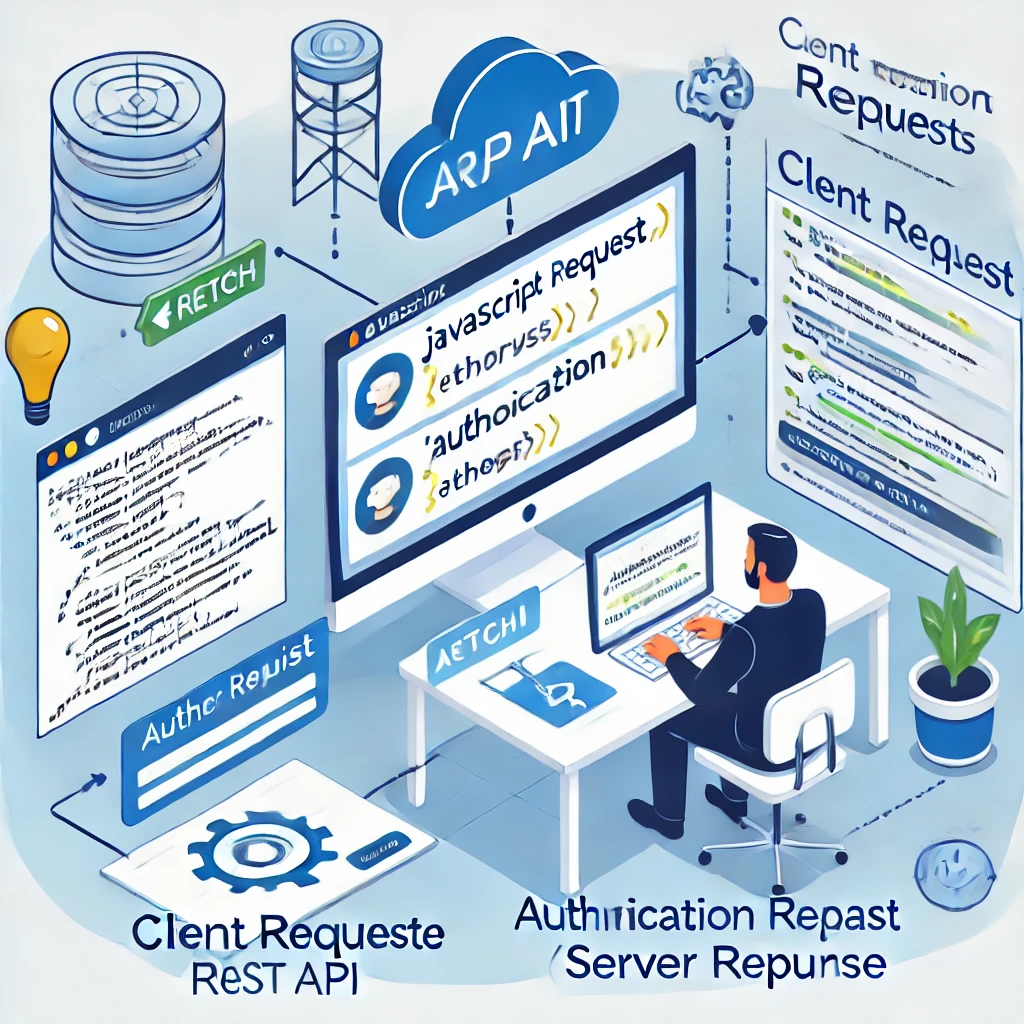
Cookie Authentication
When working with cookie authentication, you can use the WordPress logged-in user’s session cookies to access the REST API. Here’s a step-by-step example of how it works:
- User Logs In The user logs in to your WordPress site, and WordPress sets an authentication cookie in the user’s browser.
- Send Requests: When your JavaScript code requests the REST API, the browser includes the cookie in the request headers, and WordPress knows which user is making the request.
Sending Requests with the WordPress HTTP API
You can also use the WordPress HTTP API to send requests from within your WordPress site. The HTTP API is a set of functions WordPress provides to interact with REST APIs.
Example of sending a GET request:
$response = wp_remote_get('https://api.example.com/users');
if (is_wp_error($response)) {
$error_message = $response->get_error_message();
echo "Something went wrong: $error_message";
} else {
$body = wp_remote_retrieve_body($response);
echo $body;
}
This example uses the wp_remote_get() function to send a GET request to an external API and retrieve the response.
Summary
In this guide, we covered:
- What is REST API, and how does it allow different systems to communicate?
- How REST API works, including how requests are made and processed.
- Authentication Methods, such as basic authentication, cookie authentication, OAuth, and application passwords, and why they are essential.
- WordPress REST API Authentication, including how to use JavaScript and PHP to send authenticated requests.
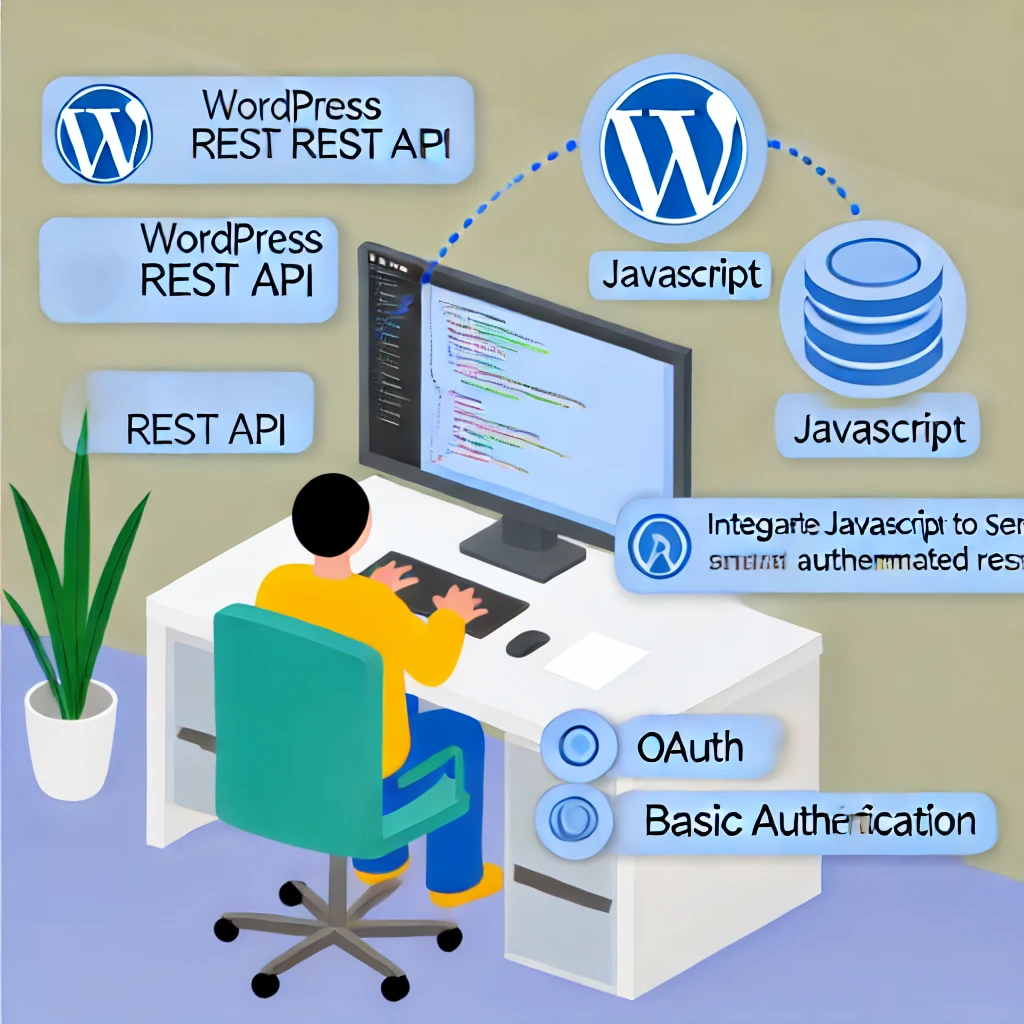
FAQ: Common Questions About REST API and Authentication
- Can I use REST API without Authentication?
- Yes, but only for public data. For any sensitive data, you need proper Authentication.
- Is Basic Authentication Secure?
- Basic Authentication is not secure for production because credentials are encoded, not encrypted. It’s better suited for testing or over HTTPS.
- What are Application Passwords used for?
- Application Passwords authenticate API requests without sharing your primary WordPress password. They help integrate third-party applications.
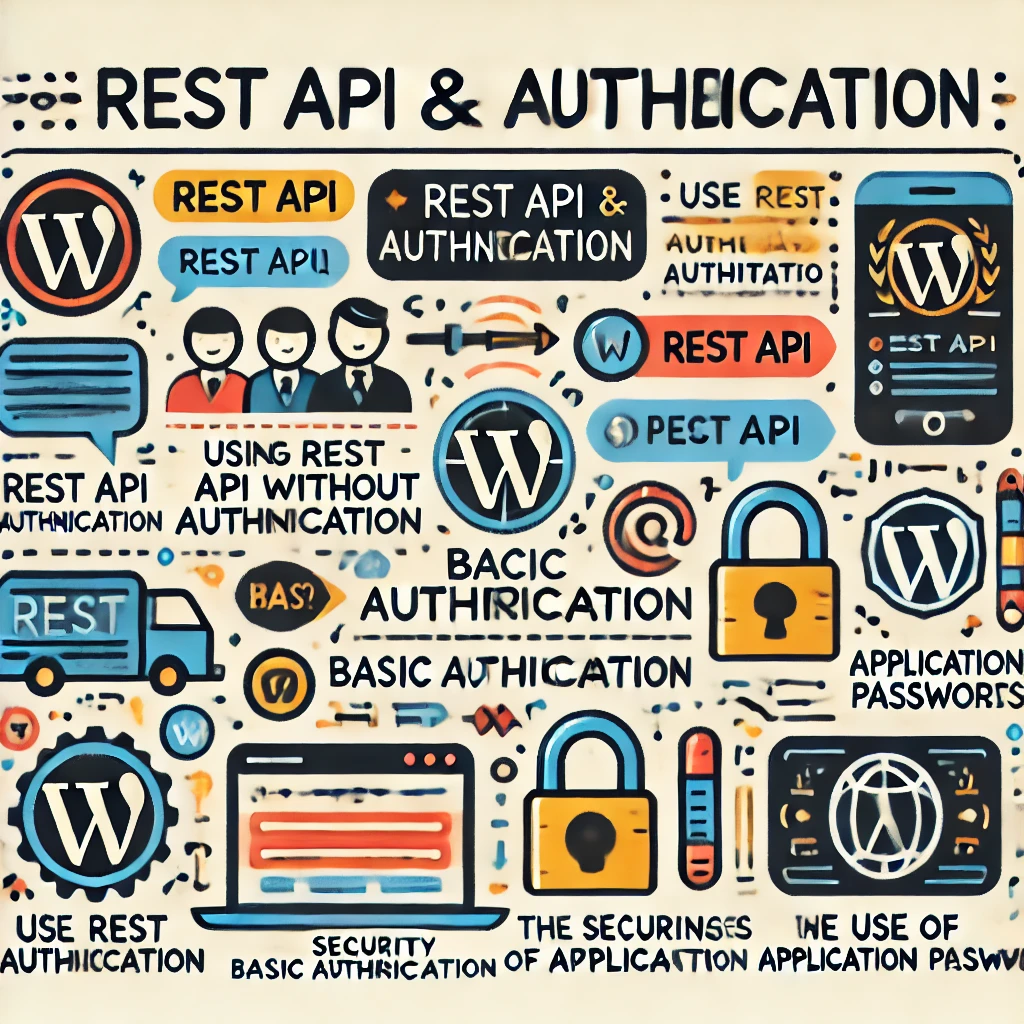
Next Steps
- Practice Authentication: Try implementing different authentication methods in a local WordPress site.
- Create Custom Endpoints: Experiment with creating custom endpoints in WordPress to extend the functionality of your REST API.
- JavaScript and PHP Integration: Practice sending requests using JavaScript (like fetch) and PHP (using the WordPress HTTP API).
- Use Postman for Testing: Use tools like Postman to test your REST API endpoints and practice sending authenticated requests.
Happy coding, and keep exploring! REST API is a powerful tool, and understanding how to use it will unlock endless possibilities in web development.
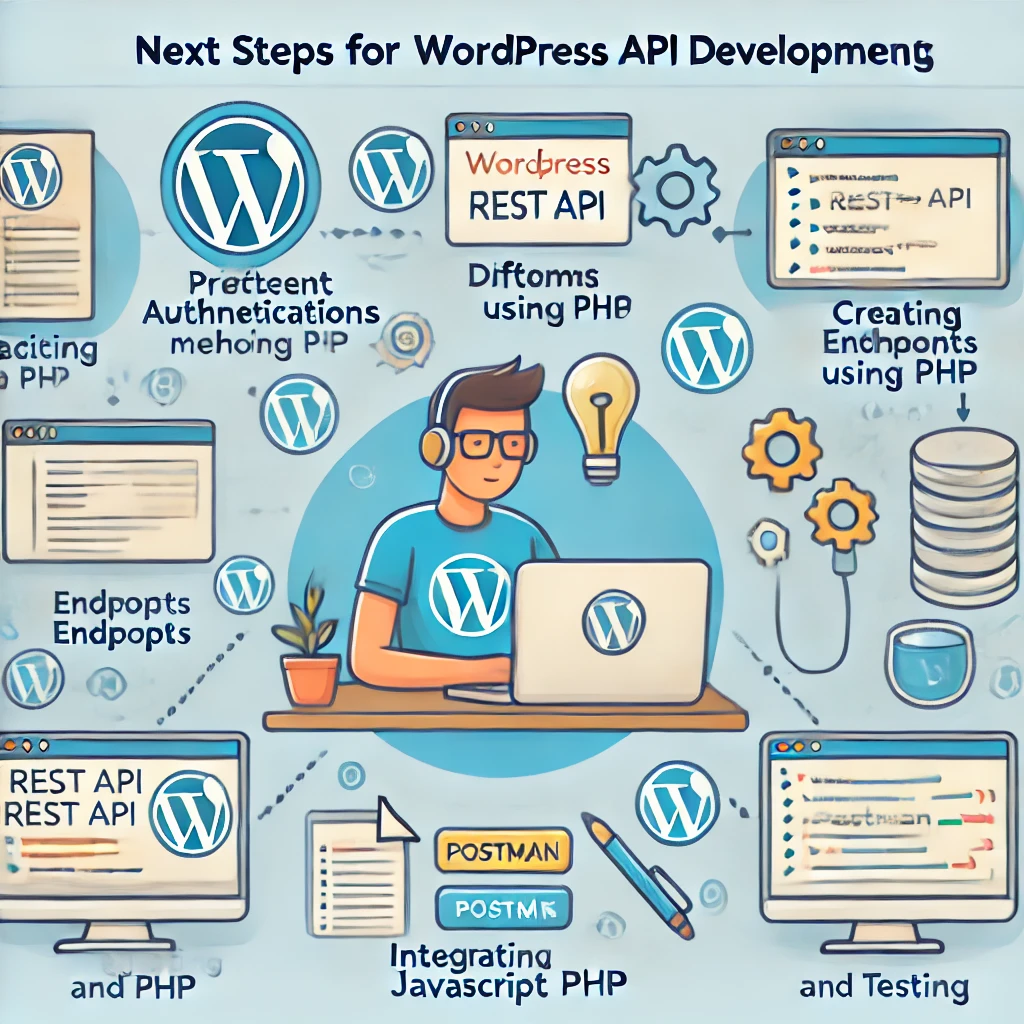
Responses