Mastering WordPress Plugin Development: From Basics to Advanced Techniques
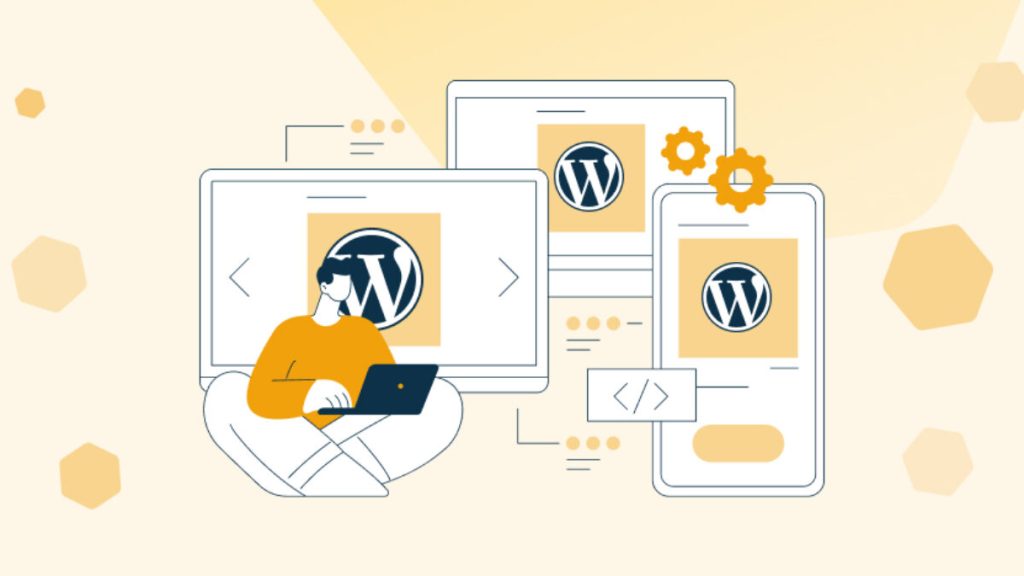
Thanks to its flexible and extendable plugin system, WordPress powers millions of websites worldwide. Plugins allow developers to add new functionality to WordPress without modifying its core files, making it one of the most popular platforms for building websites. However, creating high-quality plugins requires more than just basic coding knowledge. This guide’ll explore WordPress plugin development, covering everything from essential hooks and database handling to performance optimization, security, and even commercialization strategies.
1. Introduction to WordPress Plugins(option)
A WordPress plugin is a collection of code that adds specific functionality to a WordPress site. Whether you need to create a custom contact form, integrate with a third-party API, or enhance your site’s SEO, plugins are the go-to solution for extending WordPress.
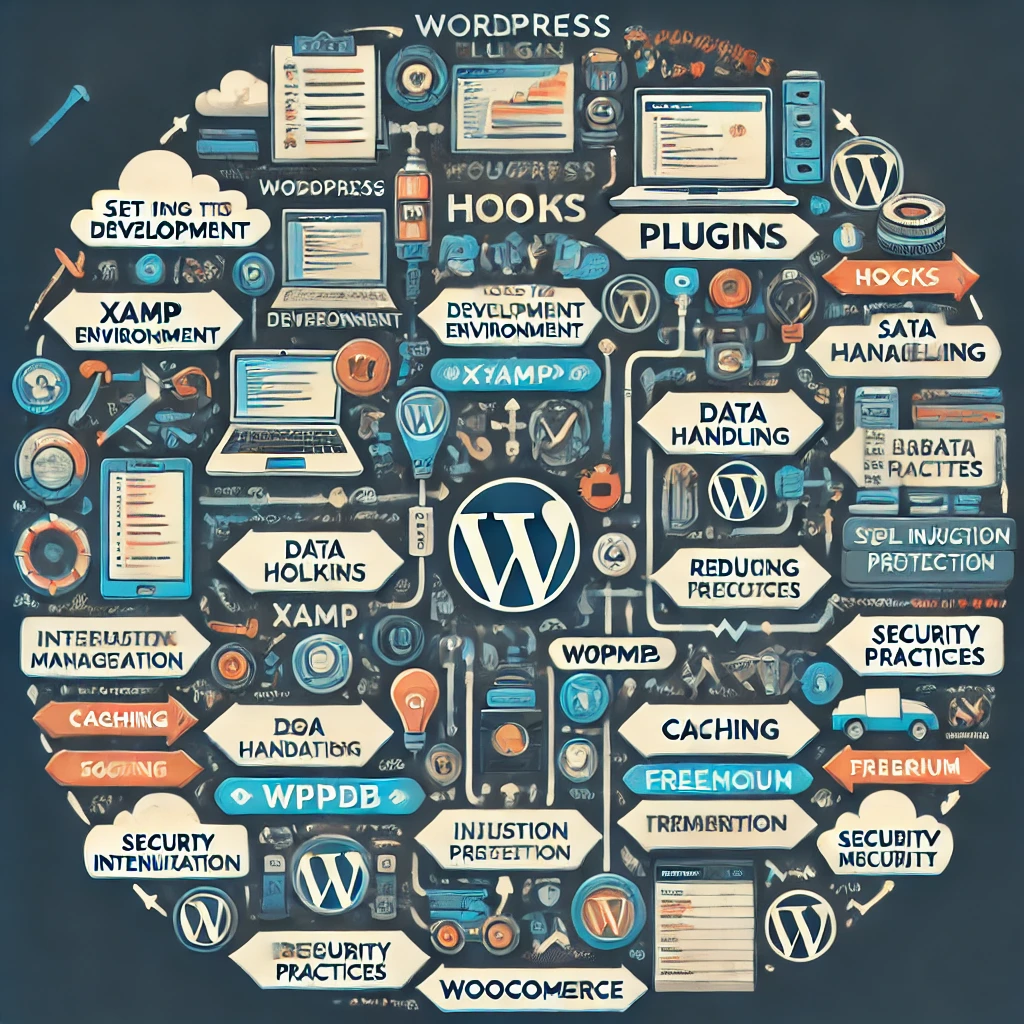
What Makes Plugins So Important?
Plugins allow you to:
- Add features to your website without modifying the core WordPress files.
- Customize existing functionalities.
- Build unique website experiences tailored to your business needs.
The natural beauty of WordPress plugins is their modularity: you can activate, deactivate, and update them independently of the WordPress core.
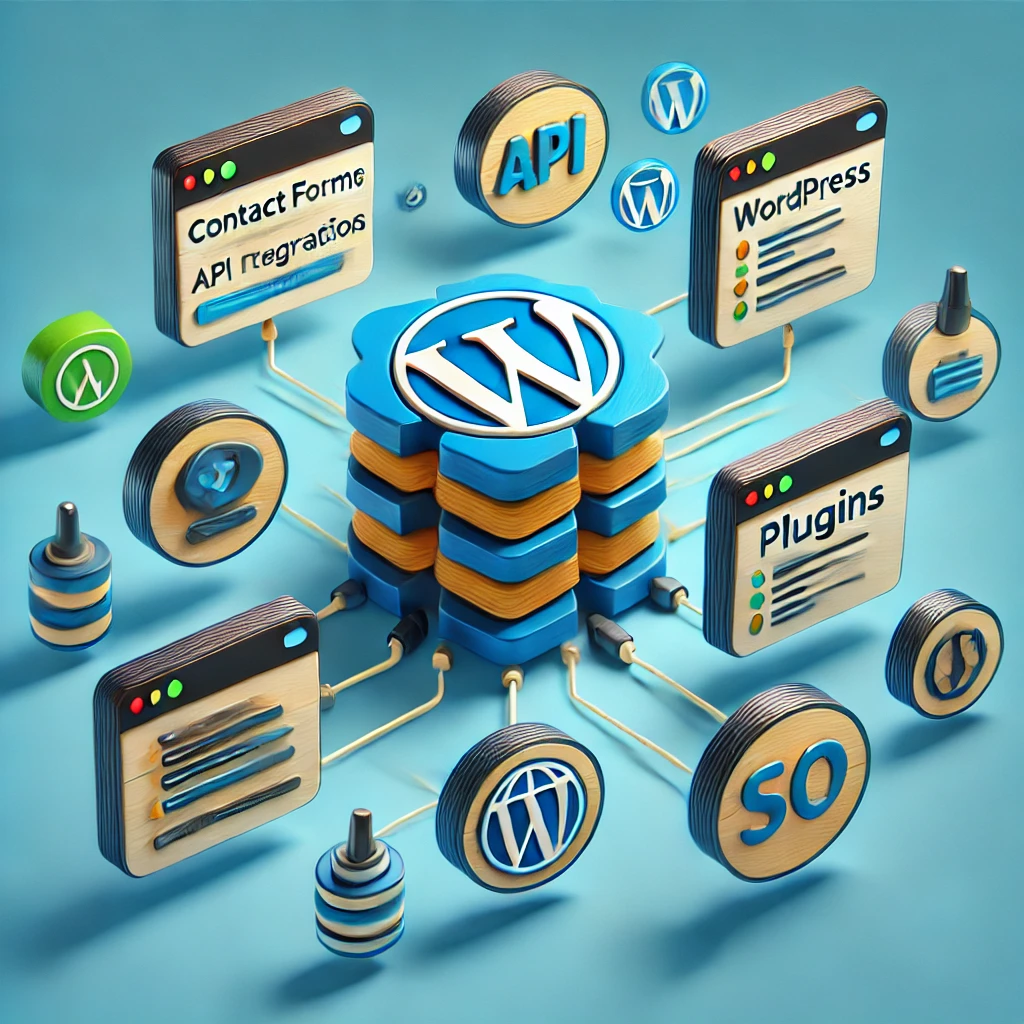
2. Setting Up a Development Environment
Before jumping into coding, setting up a reliable development environment is essential. While WordPress offers flexibility, a solid local environment ensures smooth development and debugging.
Recommended Tools
- Local Server: To set up a local development environment, use XAMPP (Windows) or MAMP (macOS).
- Code Editor: Visual Studio Code or PhpStorm for an efficient coding experience with built-in WordPress support.
- Debugging Tools: Install Query Monitor for real-time insights into your plugin’s performance, database queries, and potential issues.
Once everything is set up, you can create a plugin folder in wp-content/plugins and start coding.
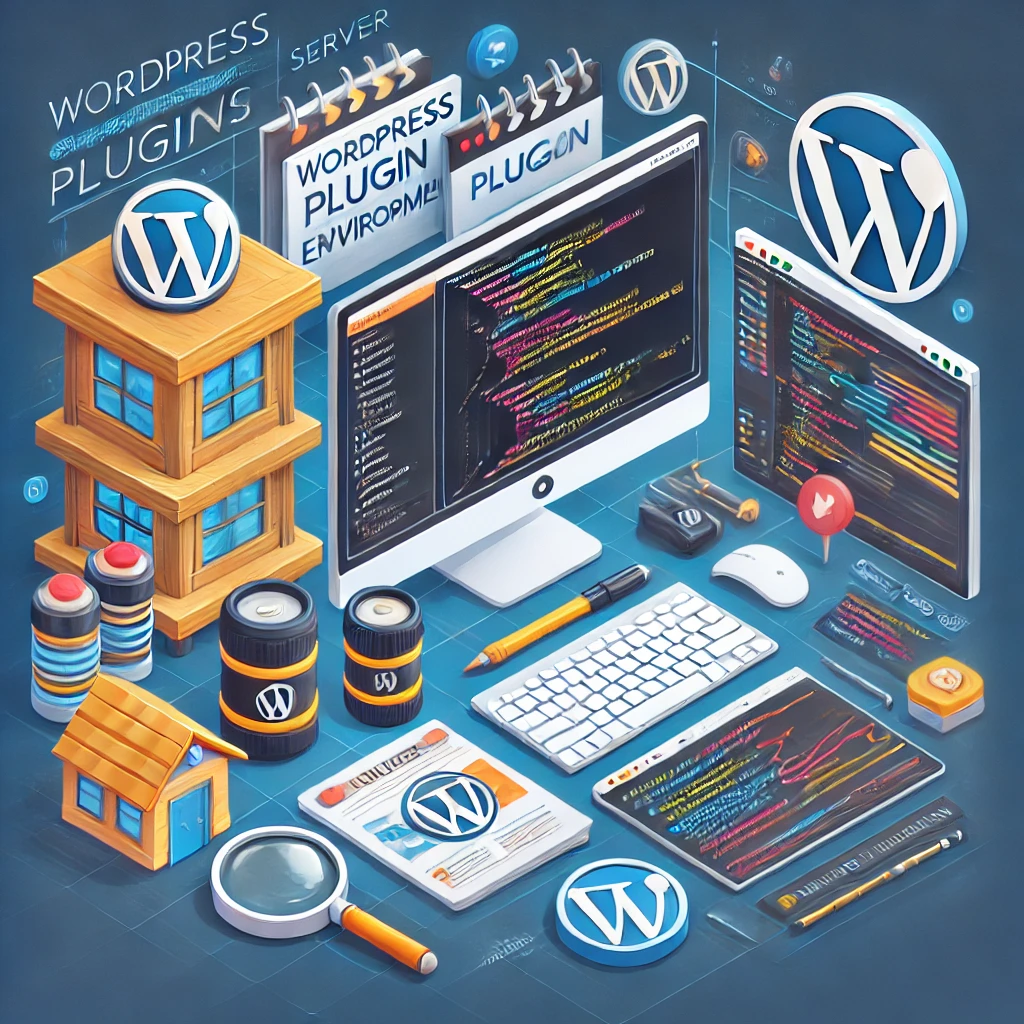
Example: Creating Your First Plugin
/*
Plugin Name: Sample Plugin
Description: A simple WordPress plugin.
Version: 1.0
Author: Your Name
*/
// Hook into the 'wp_footer' action to add content to the footer
add_action('wp_footer', 'sample_plugin_footer');
function sample_plugin_footer() {
echo '<p>This is a custom footer message added by Sample Plugin.</p>';
}
This simple plugin hooks into WordPress’s footer and adds custom text.
3. Hooks: The Backbone of Plugin Development
One of the core concepts in WordPress plugin development is hooks. Hooks allow developers to add or change functionality without altering WordPress core files. There are two types of hooks:
- Actions: Used to trigger functions at specific points, such as when a post is published.
- Filters: Used to modify data before it is displayed, such as changing content before it appears on the page
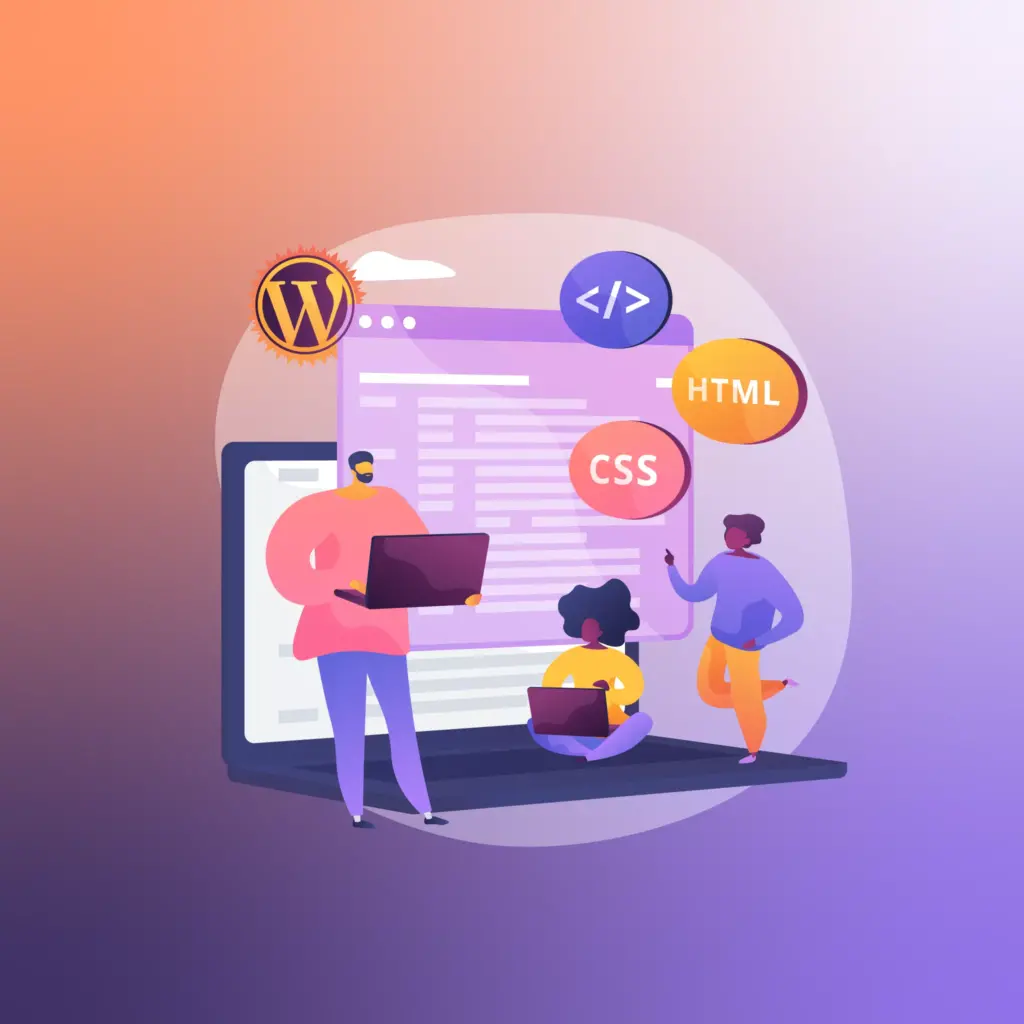
Action Example: Sending Notifications on Post Publish
add_action('publish_post', 'notify_admin_on_publish');
function notify_admin_on_publish($post_ID) {
wp_mail('admin@example.com', 'New Post Published', 'A new post has been published.');
}
This code sends an email to the admin every time a new post is published.
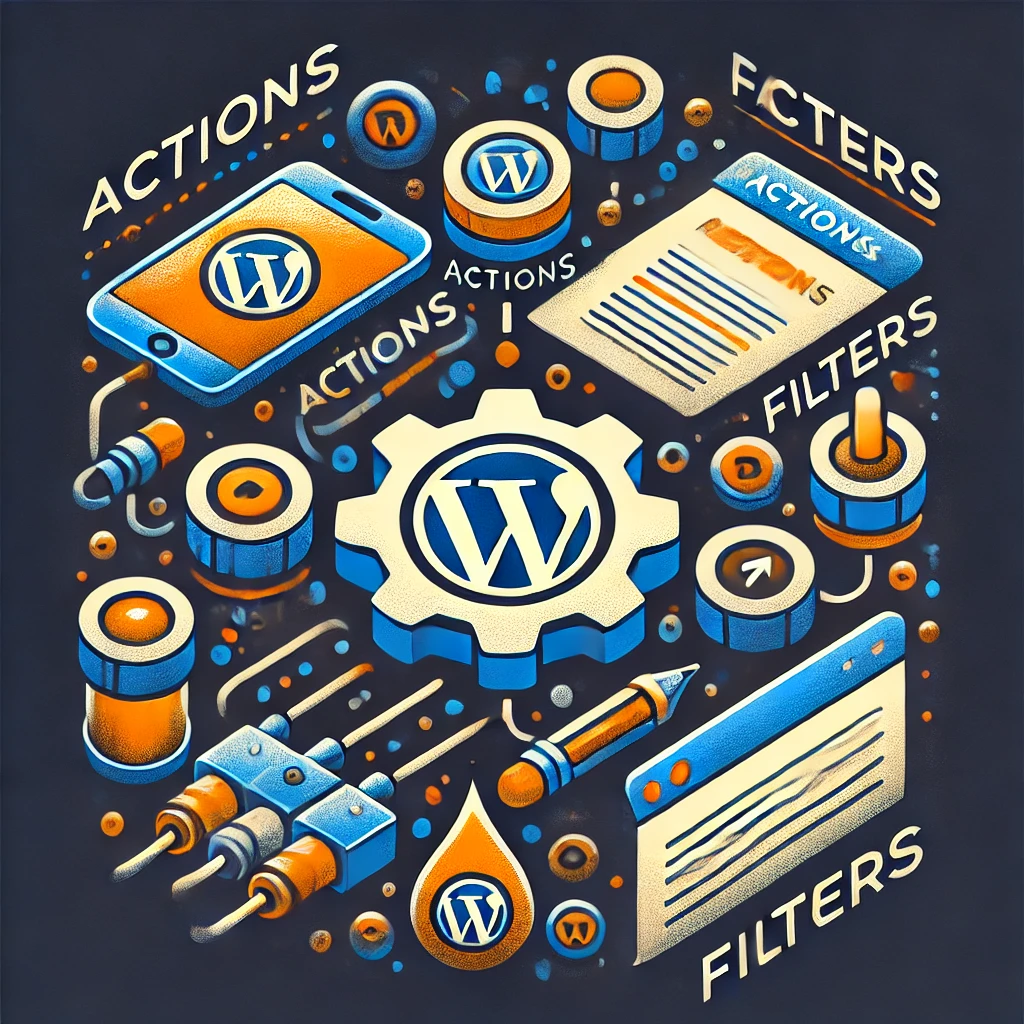
Filter Example: Modifying Post Content
php
Copy code
add_filter('the_content', 'add_message_to_content');
function add_message_to_content($content) {
return $content . '<p>Thank you for reading!</p>';
}
This adds a message to the end of every post.
Hooks are what make WordPress so extendable, and mastering them is essential for plugin development.
4. Data Handling: Leveraging WPDB for Efficient Storage
WordPress offers a powerful database abstraction layer called WPDB. It allows you to interact with the WordPress database securely, using prepared statements to avoid SQL injection attacks.
Example: Creating a Custom Database Table
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id mediumint(9) NOT NULL AUTO_INCREMENT,
name tinytext NOT NULL,
email text NOT NULL,
PRIMARY KEY (id)
) $charset_collate;";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($sql);
This creates a custom table in the WordPress database when the plugin is activated. By using WPDB, you can handle data securely and efficiently.
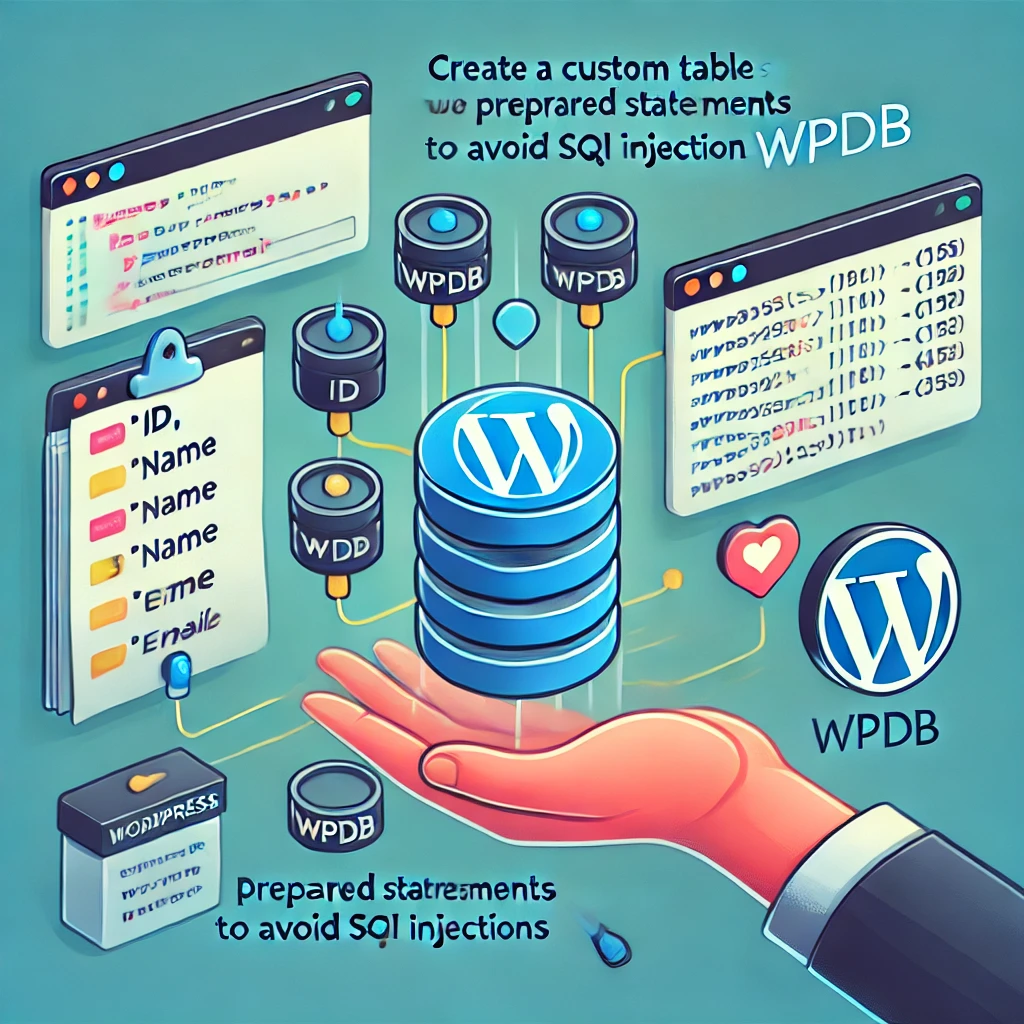
Optimizing Database Queries with Caching
Instead of querying the database every time, caching results can significantly improve your plugin’s performance.
Example: Caching Query Results
$results = wp_cache_get('custom_query_results');
if (!$results) {
$results = $wpdb->get_results("SELECT * FROM $table_name");
wp_cache_set('custom_query_results', $results);
}
Caching reduces the load on the database and speeds up your website.
5. Performance Optimization: Speeding Up Your Plugin
In large-scale WordPress sites, performance is crucial. Optimizing your plugin ensures it won’t slow down the website, especially under heavy traffic.
Database Query Optimization
The fewer queries your plugin makes, the faster it will run. Avoid querying the database on every page load if you can store results temporarily.
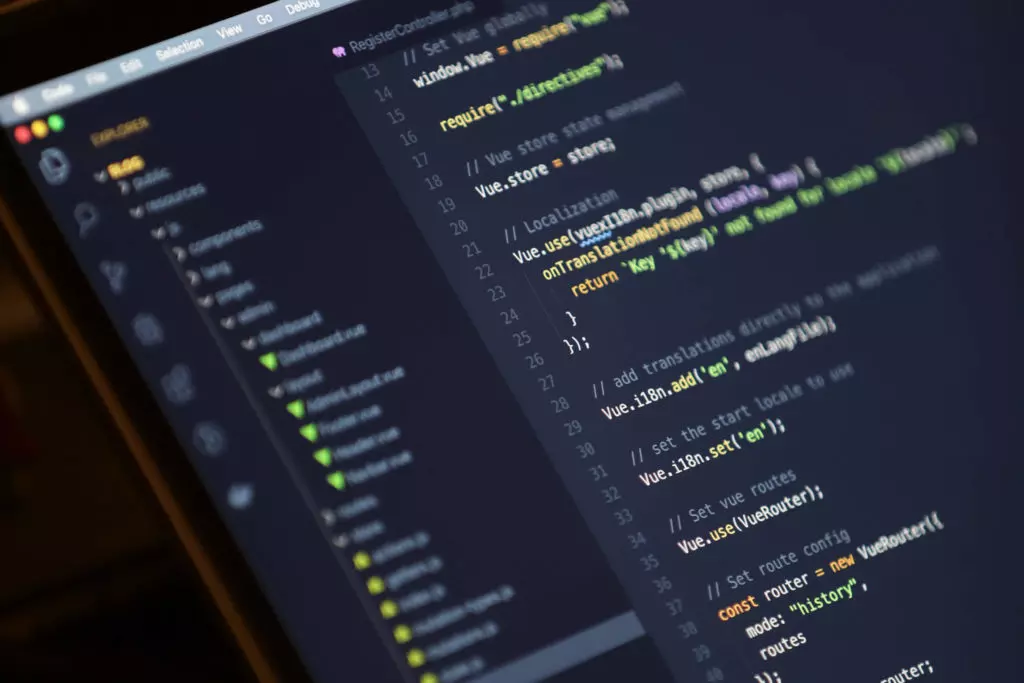
Reducing HTTP Requests
Minifying and combining CSS and JavaScript files reduces the number of HTTP requests and improves load time.
Example: Enqueuing Minified JavaScript
function enqueue_custom_scripts() {
wp_enqueue_script('custom-js', plugin_dir_url(__FILE__) . 'js/custom.min.js', array(), '1.0', true);
}
add_action('wp_enqueue_scripts', 'enqueue_custom_scripts');
Preloading Critical Assets
Where possible, preload critical assets to ensure they are available when needed, thus improving perceived performance for users.
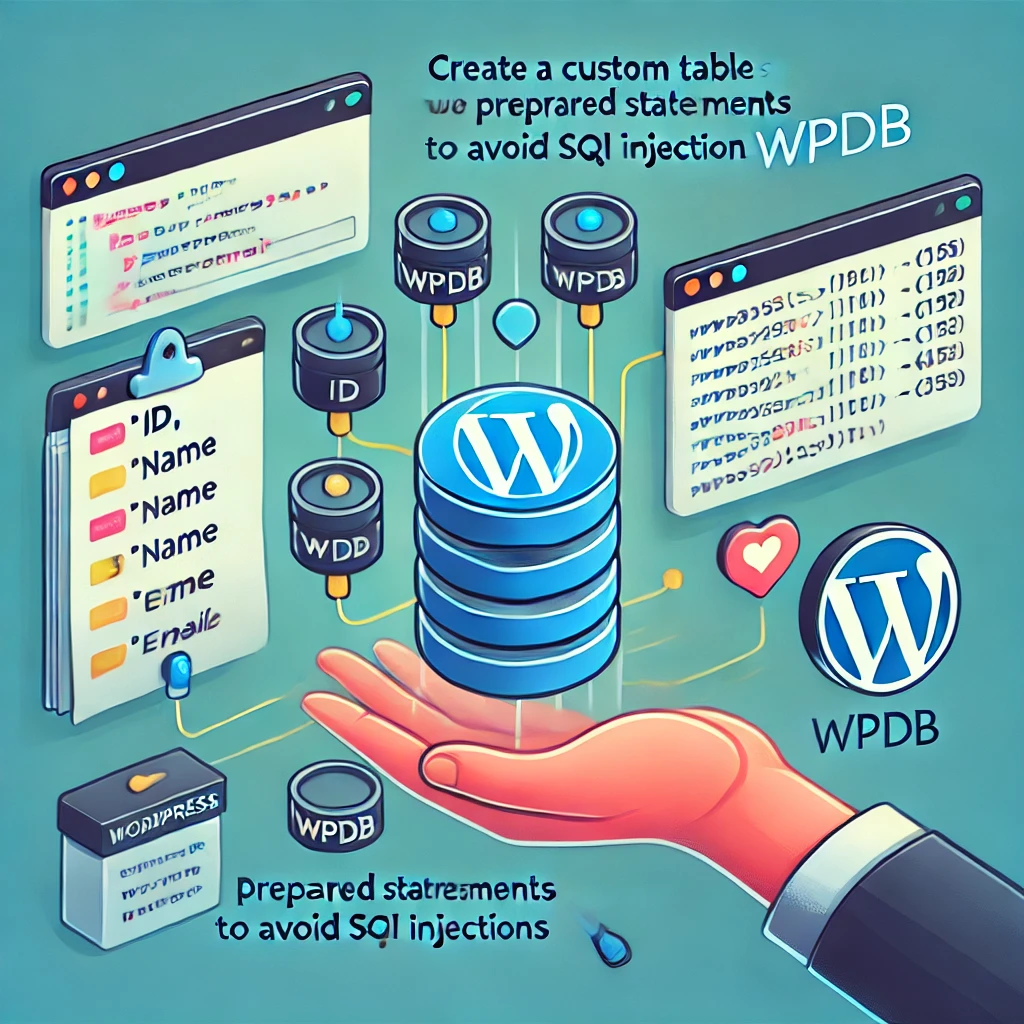
6. Security: Protecting Your Plugin from Vulnerabilities
Security is a top priority when developing plugins, as poorly coded plugins can expose websites to attacks such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
Preventing SQL Injection
When interacting with the database, always use prepared statements.
$wpdb->prepare("INSERT INTO $table_name (name, email) VALUES (%s, %s)", $name, $email);
Escaping Output
Use WordPress’s built-in escaping functions like esc_html()
to prevent XSS attacks by sanitizing user input and output.
echo esc_html($user_input);
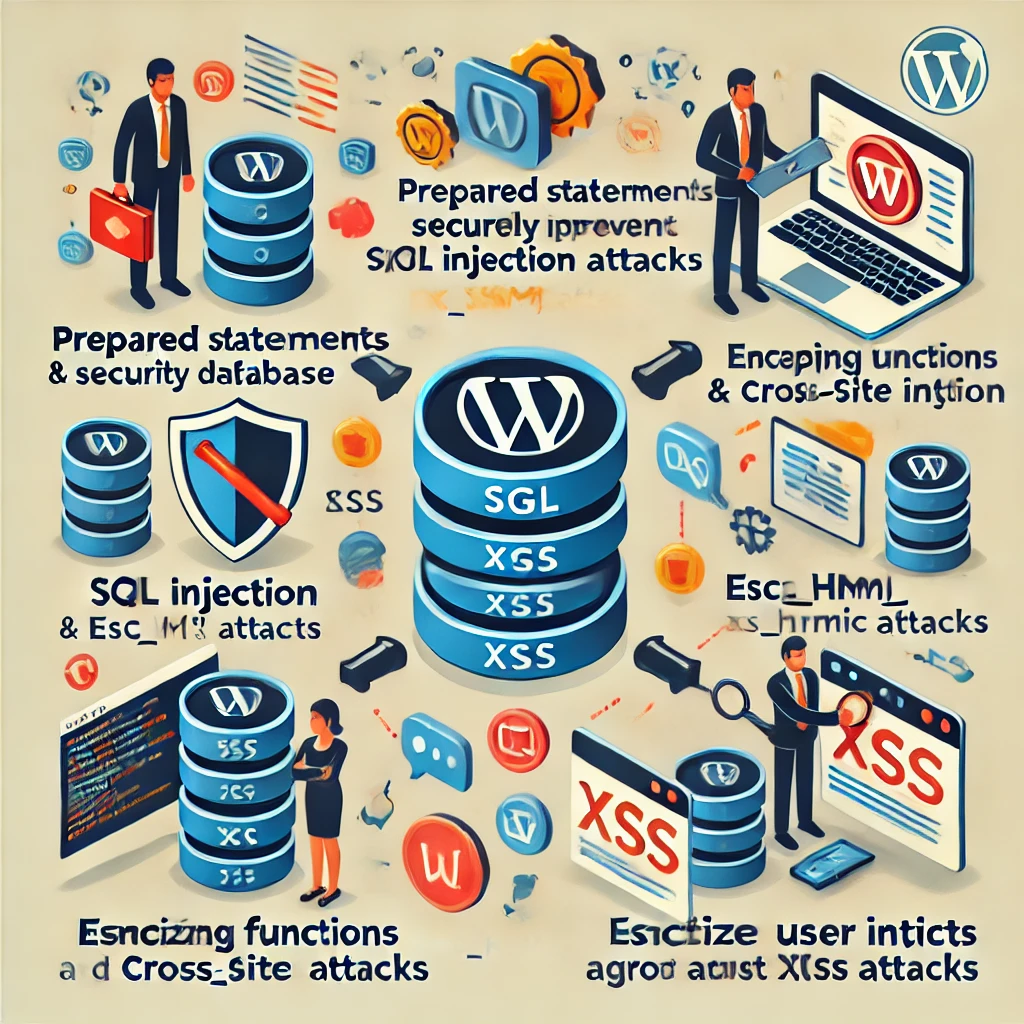
Nonces for CSRF Protection
When creating forms, use nonces to prevent CSRF attacks.
wp_nonce_field('my_form_action', 'my_nonce');
This generates a unique nonce field to verify form submissions.
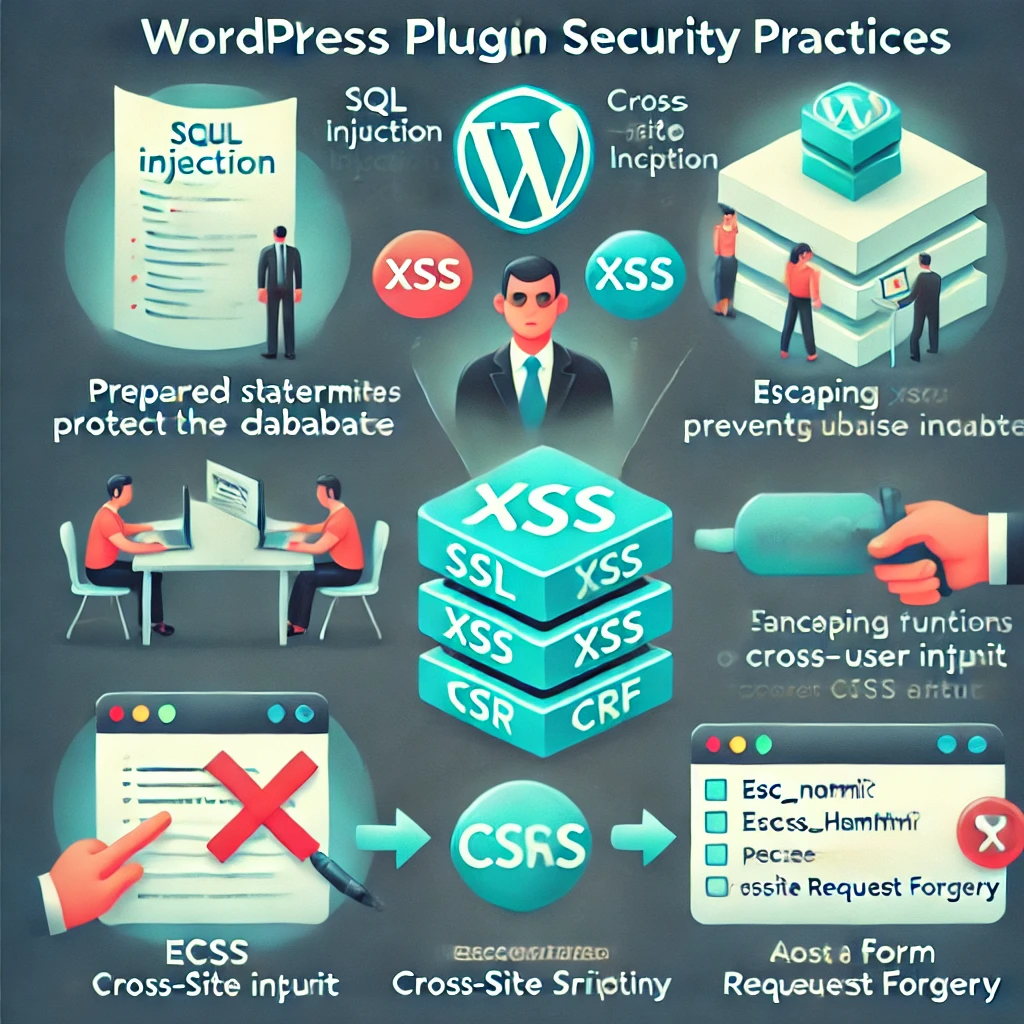
7. Internationalization: Making Your Plugin Multilingual
If you want to distribute your plugin globally, internationalization is essential. WordPress makes it easy to translate your plugin into multiple languages.
Example: Preparing Text for Translation
_e('Submit', 'your-plugin-textdomain');
By using functions like __()
and _e()
, you make your plugin ready for translation files, which will display the correct language based on the user’s settings.
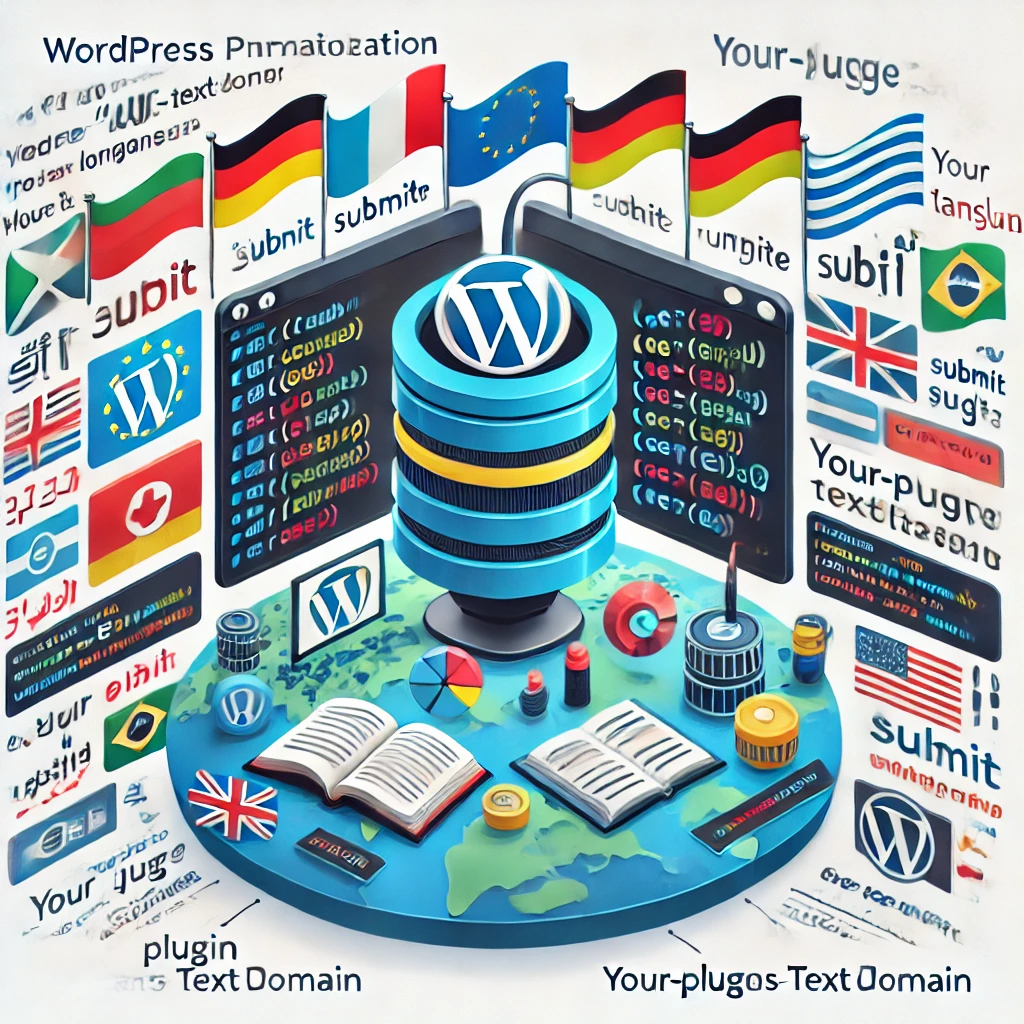
8. Commercializing Your Plugin
Turning your plugin into a product that generates revenue is a viable strategy. Many developers offer free versions of plugins with paid upgrades or additional features.
Freemium Model
The Freemium model allows you to offer a free, basic version of your plugin while charging for premium features.
- WooCommerce Integration: You can sell the premium version of your plugin via WooCommerce directly on your website.
- WordPress Plugin Repository: Upload the free version of your plugin to the WordPress Plugin Directory to increase visibility, then link users to the paid version.
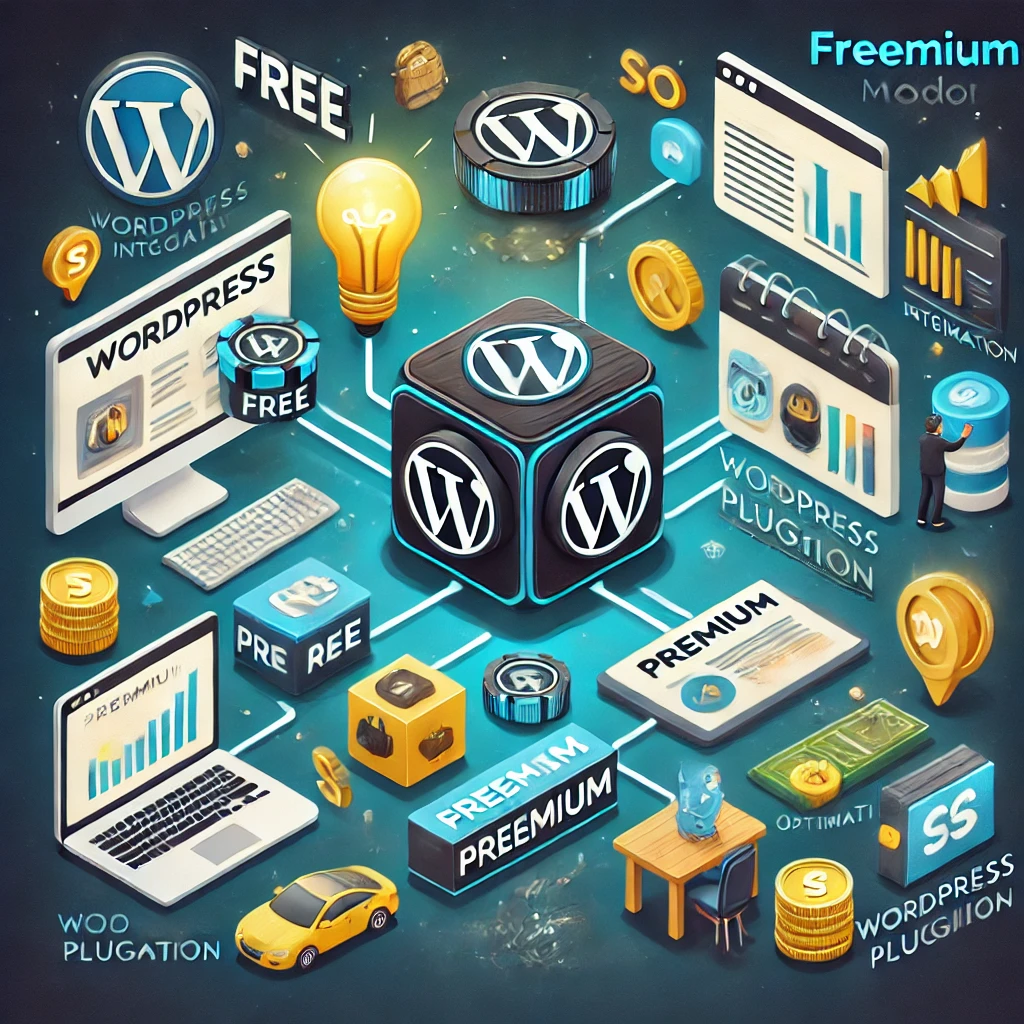
Marketing and SEO
Promote your plugin by creating high-quality content around it, ensuring it ranks in search engines. Blog posts, tutorials, and case studies can help build an audience around your plugin.
9. Best Practices and Real-World Examples
Many popular plugins like WooCommerce and Yoast SEO offer excellent examples of well-structured code and optimal plugin design. Analyzing successful plugins can offer valuable insights for your own development.
Case Study: WooCommerce
- Modular Design: WooCommerce uses a modular approach, allowing developers to extend its functionality with additional extensions and plugins.
- Database Management: WooCommerce optimizes database performance by efficiently handling large volumes of data.
Learning from these examples can improve the structure and performance of your plugin
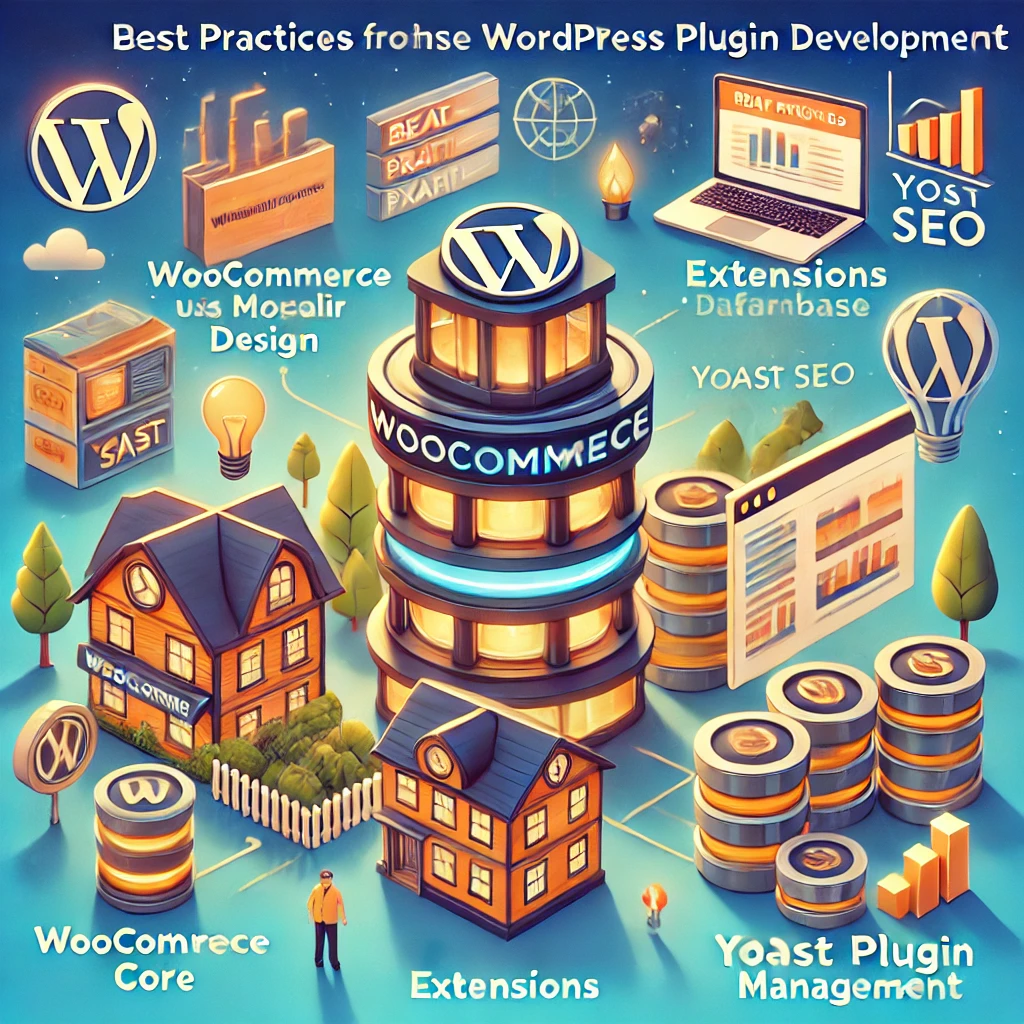
Conclusion: Expanding Your Plugin Development Skills
WordPress plugin development is an incredibly versatile and powerful skill. By mastering hooks, database management, performance optimization, and security, you can create high-quality, scalable plugins that enhance WordPress’s capabilities. Furthermore, understanding how to commercialize your plugin can turn your development efforts into a profitable business.
As you continue to develop plugins, keep up with the latest WordPress trends—such as the shift towards the Gutenberg block editor—and integrate new technologies to stay ahead of the curve. Happy coding!
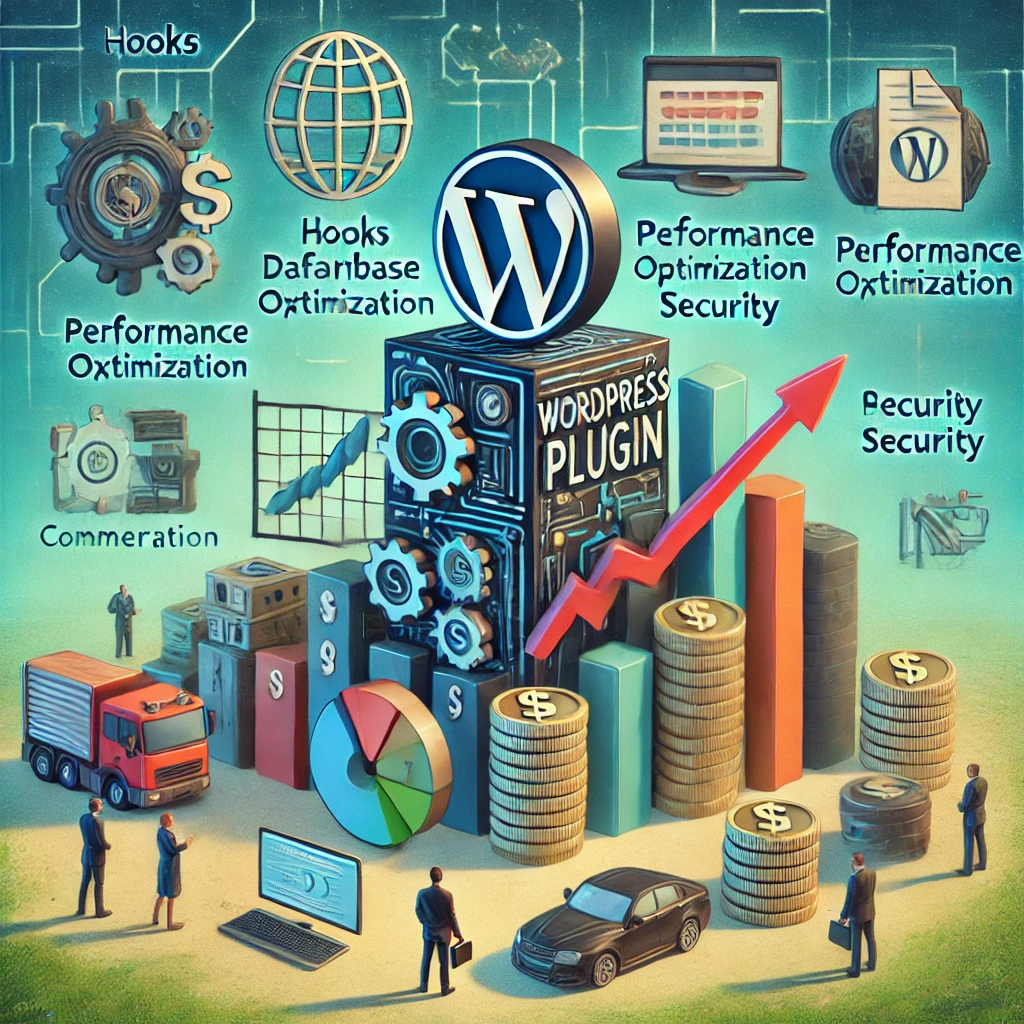
Responses