Dominio de JavaScript en WordPress: Guía de buenas prácticas
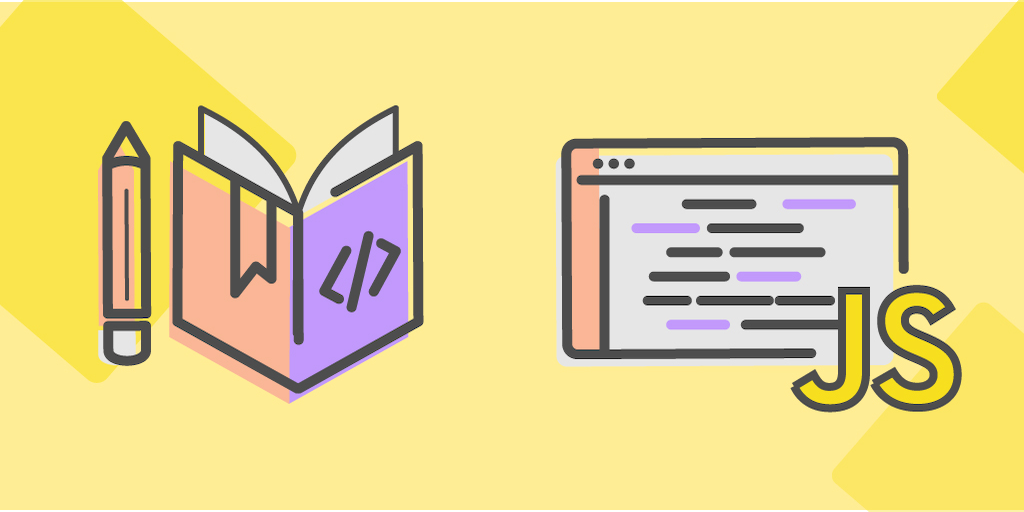
Dominar las normas de codificación JavaScript de WordPress: Una guía completa para desarrolladores
Los estándares de codificación de JavaScript en WordPress son cruciales para mantener la coherencia, la legibilidad y un código de alta calidad, tanto si está desarrollando temas, plugins o contribuyendo al núcleo. Estas normas garantizan que su JavaScript se integre a la perfección con PHP, HTML y CSS y facilitan la colaboración entre equipos diversos. Vamos a sumergirnos en estas normas, desglosándolas con ejemplos prácticos para hacerlas accesibles a todos los desarrolladores.
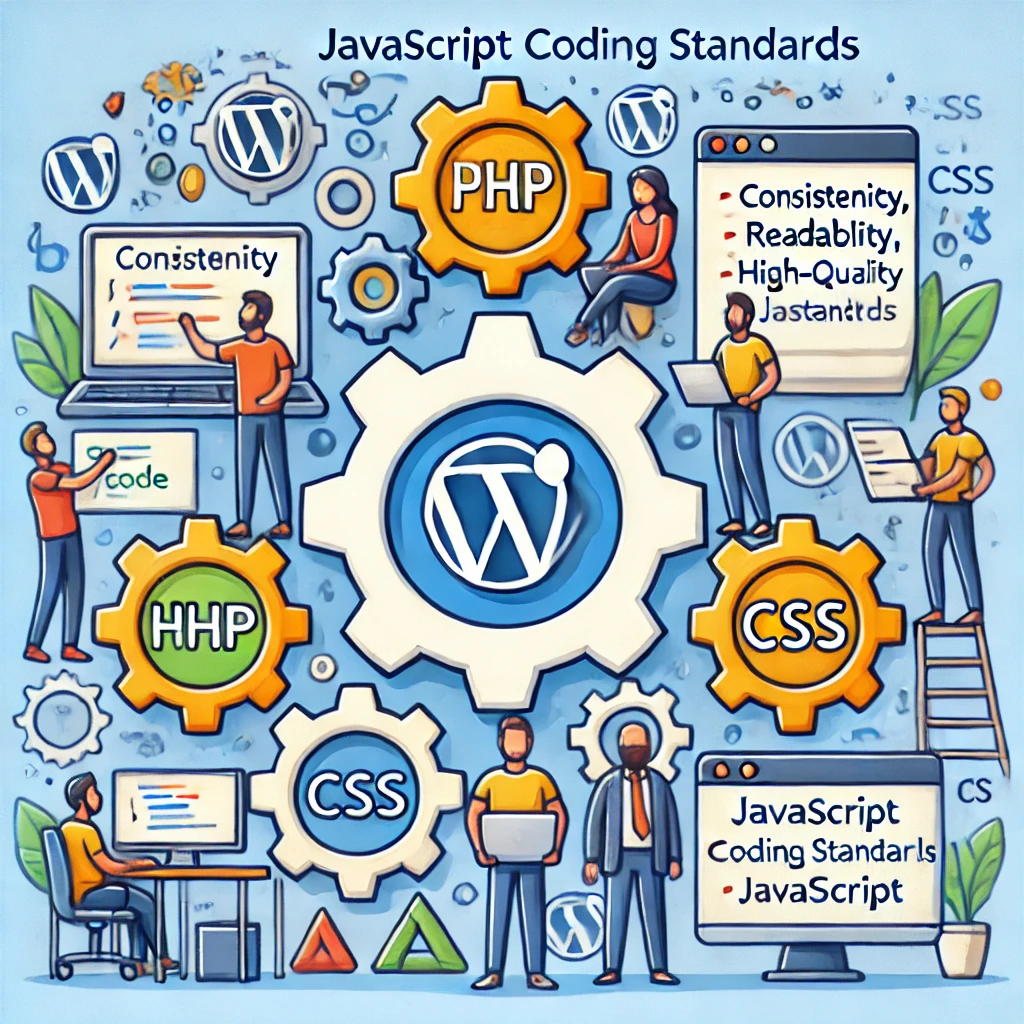
Visión general: Construyendo sobre la base de jQuery
Las normas de codificación JavaScript de WordPress se derivan del Guía de estilo jQuery JavaScript, introducido originalmente en 2012. Aunque inicialmente estaba dirigido a proyectos jQuery, su éxito llevó a una adopción generalizada más allá del framework. Sin embargo, WordPress tiene su propia versión de estos estándares, que difiere ligeramente de la guía original de jQuery.
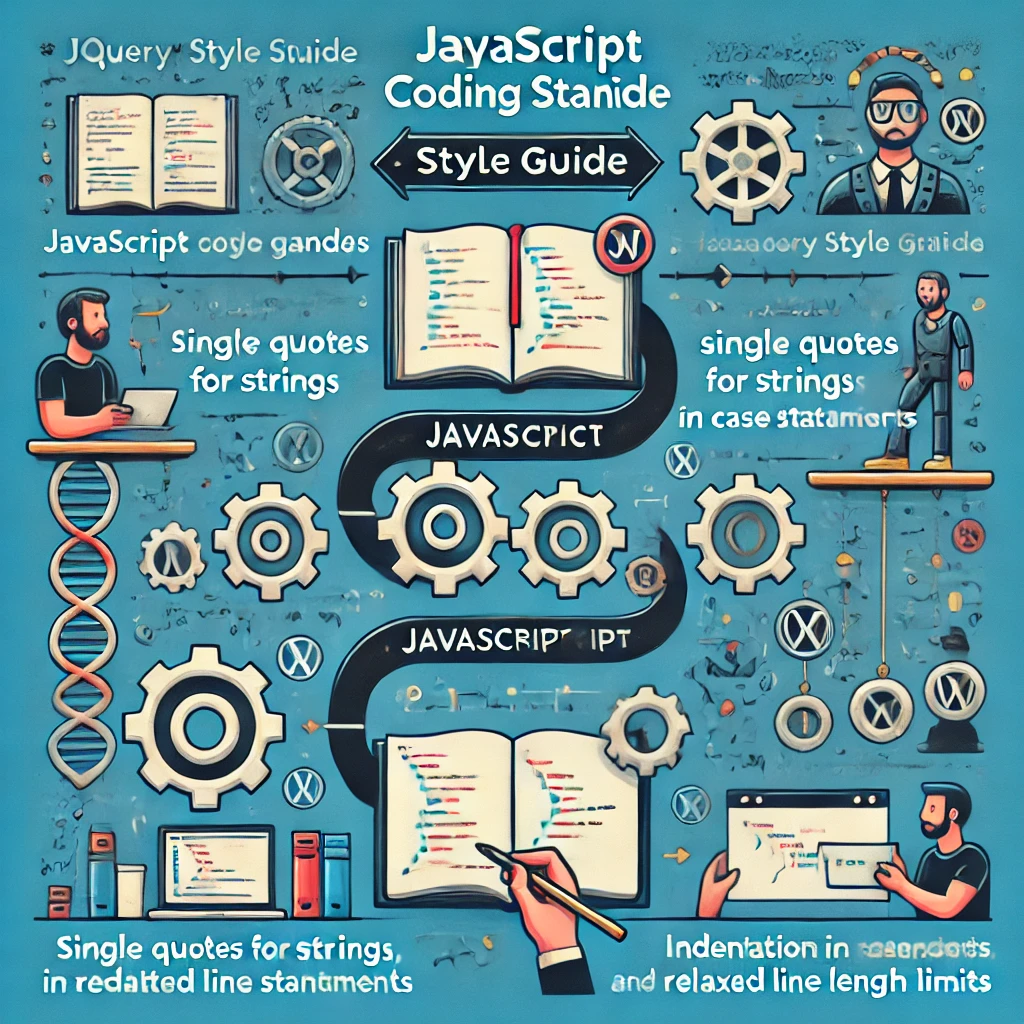
Las diferencias clave incluyen:
- Citas simples para cuerdas: WordPress prefiere las comillas simples para las declaraciones de cadenas.
- Sangría de la declaración de caso: En WordPress, las sentencias case están sangradas dentro de un bloque switch.
- Sangría de función coherente: Todo el contenido dentro de una función está sangrado, incluyendo las envolturas de cierre de todo el archivo.
- Límite de longitud de línea relajado: Mientras que jQuery impone un límite de 100 caracteres por línea, WordPress lo fomenta pero no lo impone estrictamente.
Áreas principales cubiertas por los estándares JavaScript de WordPress
1.Formato del código y sangría
Un formato y una sangría adecuados son esenciales para un código legible y fácil de mantener. El estándar de WordPress hace hincapié en:
- Indentación: Utilice pestañas en lugar de espacios para la sangría. Cada bloque lógico debe ir sangrado para facilitar su legibilidad. Las tabulaciones ayudan a mantener la coherencia, especialmente cuando diferentes desarrolladores trabajan en el mismo código base.
( función ( $ ) {
// Las expresiones de bloque están sangradas
function hacerAlgo() {
// El código de la función también está sangrado
console.log( 'HacerAlgo' );
}
} )( jQuery );
En el ejemplo anterior, la función doSomething() y su contenido están sangrados para mostrar que forman parte del IIFE.
En el ejemplo anterior, la función doSomething() y su contenido están sangrados para mostrar que forman parte del IIFE.
var html = '<p>La suma de ' + a + ' y ' + b + ' es ' + ( a + b ) + '</p>';
elementos
.addClass( 'foo' )
.children()
.html( 'hola' )
.end()
.appendTo( 'body' );
Aquí, cada método de la cadena comienza en una nueva línea, lo que hace que la secuencia de operaciones sea más legible.
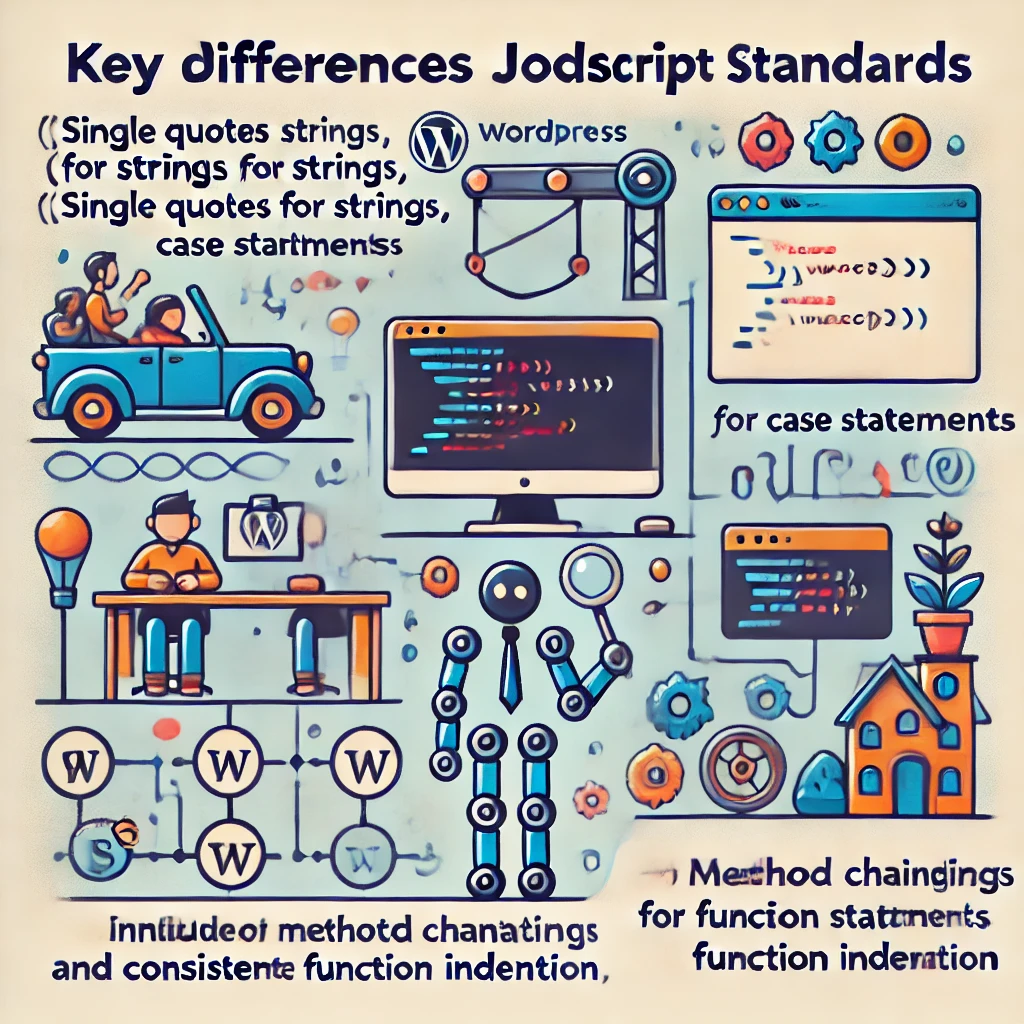
2. Espaciado entre objetos y matrices
Objetos y matrices: Un espaciado coherente es crucial para la claridad visual, especialmente cuando se trabaja con estructuras de datos complejas. Siga estas directrices para el espaciado:
// Forma correcta de declarar objetos
var obj = {
nombre: 'Juan',
edad: 27
altura: 179
};
// Forma incorrecta de declarar objetos (no condensar propiedades)
var obj = { nombre: 'Juan', edad: 27, altura: 179 };
// Los arrays también deben seguir un espaciado coherente
var array = [ 1, 2, 3 ];
El espaciado correcto en objetos y matrices garantiza que su estructura de datos sea visualmente distinta, lo que ayuda a la hora de depurar o revisar el código.
3. Punto y coma
Utilice siempre punto y coma para terminar las sentencias. Omitir el punto y coma puede provocar problemas inesperados durante la inserción automática de punto y coma (ASI) de JavaScript.
var array = [ 1, 2 ];
console.log( '¡Hola, mundo!' );
Aunque JavaScript puede inferir a veces puntos y comas, es una buena práctica incluirlos explícitamente para evitar ambigüedades, especialmente cuando se combinan varios fragmentos de código o se contribuye a proyectos en equipo.
4. Declaraciones de variables: const
, deje
y var
- Utilice const para variables cuyo valor no cambiará. Esto ayuda a prevenir la reasignación accidental y deja claras sus intenciones a otros desarrolladores.
- Utilice deje para las variables cuyo valor pueda cambiar dentro de un ámbito determinado. Esto asegura que la variable tiene un ámbito de bloque, evitando problemas relacionados con el hoisting.
- **Evitar**var a menos que sea necesario, ya que tiene alcance de función, lo que puede dar lugar a comportamientos no deseados debido a la elevación.
const userName = 'Alice'; // Utilice const para valores fijos
let userAge = 30; // Utilice let para valores que puedan cambiar
Utilizando const
y deje
ayuda adecuadamente a mejorar la seguridad del código y facilita el razonamiento sobre los tiempos de vida de las variables.
5. Convenciones de denominación
Unas convenciones de nomenclatura coherentes hacen que el código sea más legible y fácil de mantener:
- CamelCase: Utilice camelCase para los nombres de variables y funciones. Por ejemplo,
userId
ofetchUserData
. - Clases: Utilice UpperCamelCase (PascalCase) para los nombres de clase.
- Constantes: Utilice SCREAMING_SNAKE_CASE para las constantes.
const MAX_CONNECTIONS = 5;
clase UserProfile {
constructor( nombre ) {
this.nombre = nombre;
}
}
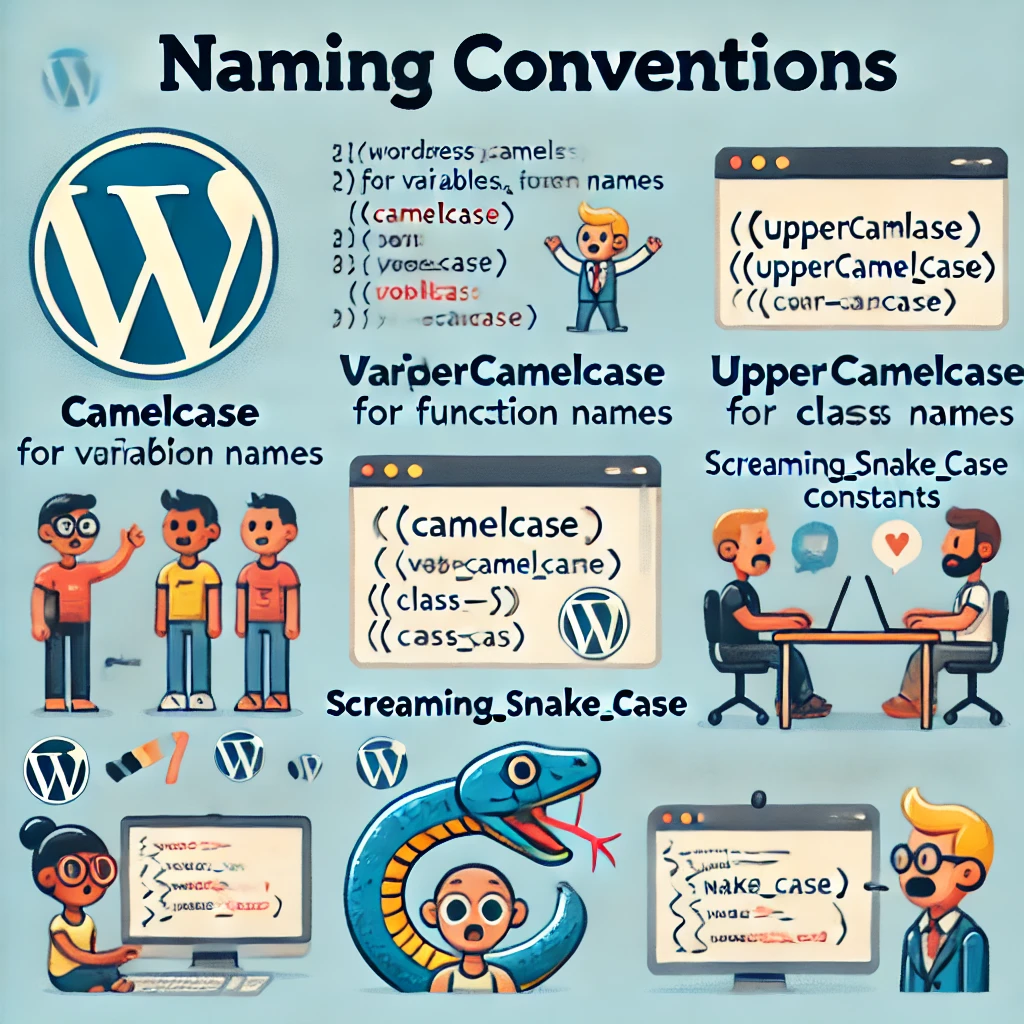
CamelCase para variables y funciones ayuda a diferenciarlas de las clases y constantes, contribuyendo a una mayor claridad del código.
6. Comprobaciones de igualdad
Utilice siempre igualdad/desigualdad estricta (===******** y !==) en lugar de las comprobaciones abstractas (== y !=). Esto ayuda a evitar tipos de coerción inesperados que pueden dar lugar a errores.
if ( nombre === 'Juan' ) {
// Comprobación estricta de igualdad
console.log( '¡Hola, Juan!' );
}
if ( resultado !== false ) {
// Comprobación estricta de desigualdad
console.log( 'El resultado no es falso' );
}
La igualdad estricta garantiza que tanto el tipo como el valor se comparan, lo que hace que su código sea más predecible.
7. Manipulación de cadenas
Utilice comillas simples para cadenas a menos que la cadena contenga una comilla simple, en cuyo caso utilice comillas dobles para evitar el escape.
var saludo = "¡Hola mundo!
var statement = "Que tenga un buen día";
Esta regla garantiza la coherencia en todo el código base, lo que facilita que los desarrolladores sigan las mismas prácticas.
8. Declaraciones de cambio
Las sentencias switch deben tener un romper para cada caso (excepto para el predeterminado) para evitar errores de caída. Además, sangrar declaraciones de caso deben estar en una pestaña dentro del interruptor.
switch ( event.keyCode ) {
case $.ui.keyCode.ENTER:
case $.ui.keyCode.SPACE:
executeFunction();
break;
case $.ui.keyCode.ESCAPE:
cancelFunction();
break;
por defecto:
defaultFunction();
}
Una sangría adecuada y el uso de pausas evitan comportamientos no deseados cuando se dan varios casos.
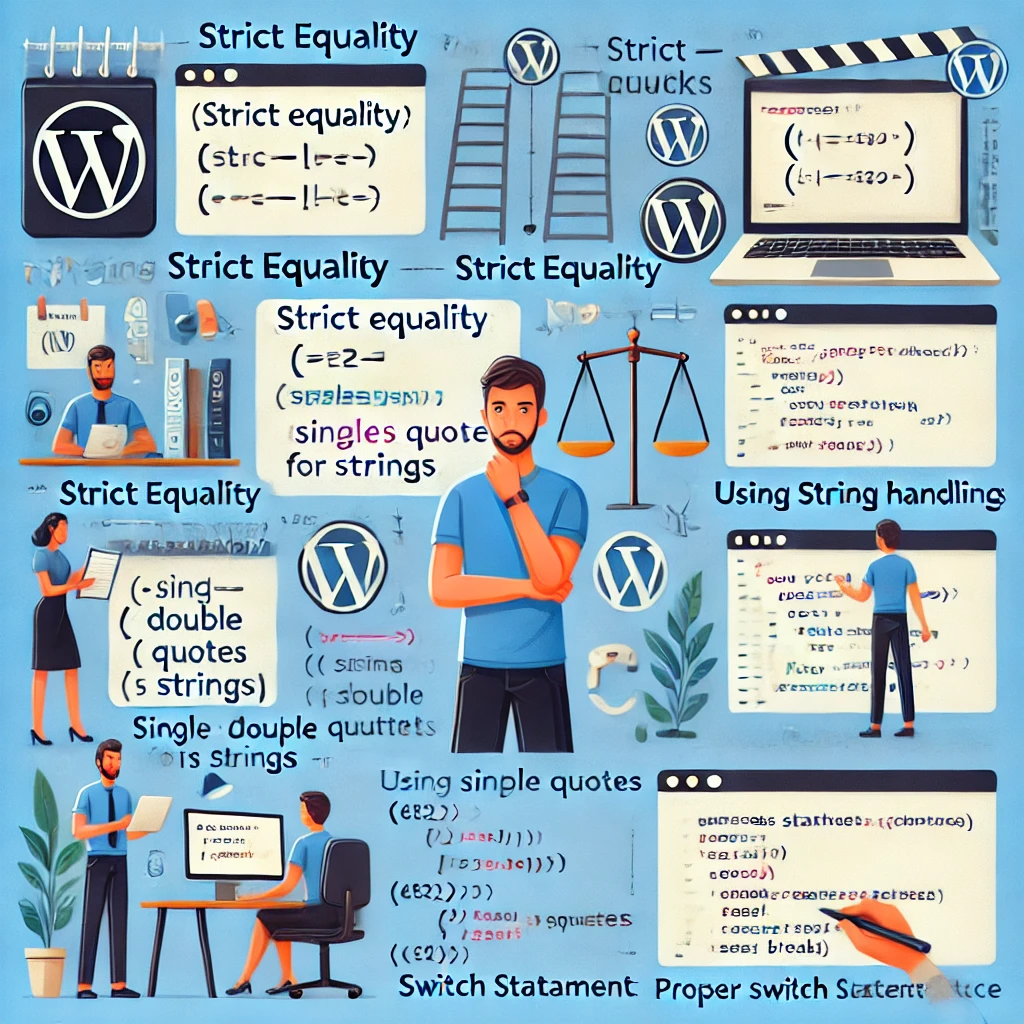
Mejores prácticas en el desarrollo JavaScript para WordPress
1. Evite las variables globales
Las variables globales pueden dar lugar a contaminación del espacio de nombres y conflictos con otros scripts. En su lugar, encapsule su código dentro de un Expresión de función inmediatamente invocada (IIFE) para crear un ámbito local.
( function() {
const localVar = 'Ámbito de esta función';
// El código aquí está protegido del ámbito global
} )();
Encapsular el código reduce el riesgo de conflictos, especialmente cuando se trabaja en entornos con múltiples bibliotecas de terceros.
2. Documentación y comentarios
WordPress sigue el Norma JSDoc 3 para documentar el código JavaScript. La documentación es crucial para comprender la funcionalidad de métodos, clases y funciones complejas.
- Comentarios de una línea: Utilice
//
para explicaciones breves, sobre todo al describir una línea de código específica. - Comentarios multilínea: Utilice
/* */
para explicaciones más detalladas o para describir un bloque de código.
/**
* Suma dos números.
*
* @param {number} a - El primer número.
* @param {number} b - El segundo número.
* @devuelve {number} Suma de a y b.
*/
function add( a, b ) {
devuelve a + b;
}
Documentar el código con JSDoc permite a las herramientas generar documentación automáticamente y ayuda a los desarrolladores a comprender su código sin tener que sumergirse en profundidad en la implementación.
3. Tratamiento de errores
Utilice intentar...atrapar para manejar los posibles errores con elegancia. La gestión de errores garantiza que los problemas inesperados no provoquen el fallo de toda su aplicación.
try {
const datos = JSON.parse( jsonString );
} catch ( error ) {
console.error( 'JSON no válido:', error );
}
Una gestión adecuada de los errores mejora la resistencia de su código, facilitando su depuración y mantenimiento.
Normas de codificación de JavaScript en la práctica
Para asegurarse de que su código JavaScript se adhiere a los estándares de WordPress
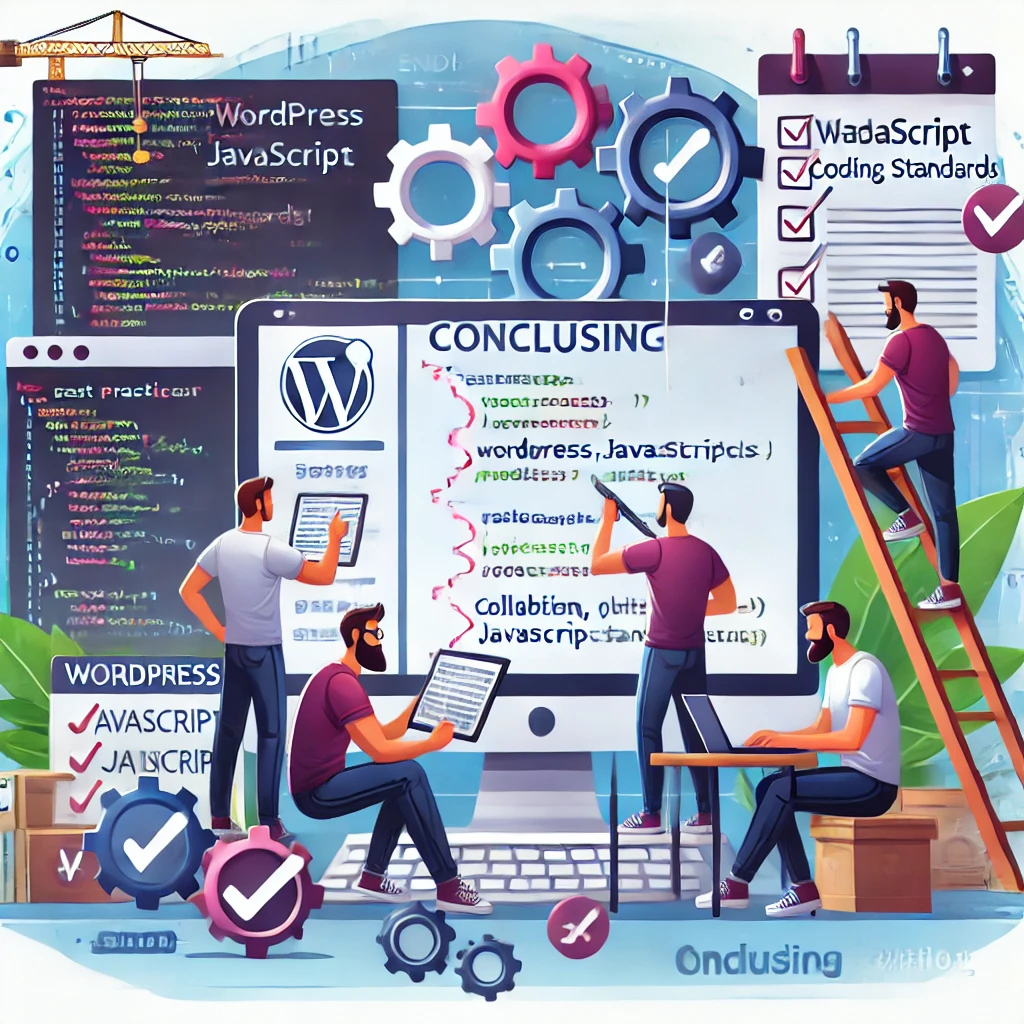
Respuestas