Enhancing WordPress with JavaScript: A Comprehensive Guide to Best Practices and Advanced Integration
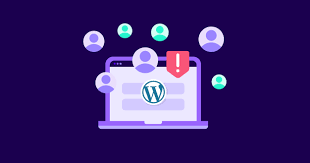
WordPress is a highly adaptable content management system, and JavaScript has become essential in transforming static pages into dynamic, interactive experiences. Do you know that websites using interactive JavaScript features can see up to a 40% increase in user engagement? Over the years, JavaScript’s role in WordPress has evolved significantly from simple client-side scripts to complex frameworks like React, Vue.js, and Angular that enable rich, interactive features. Integrating JavaScript into WordPress has empowered developers to create faster, more user-friendly experiences and has been instrumental in modernizing website design. For example, introducing the Gutenberg editor, which leverages React, has wholly transformed content creation within WordPress.
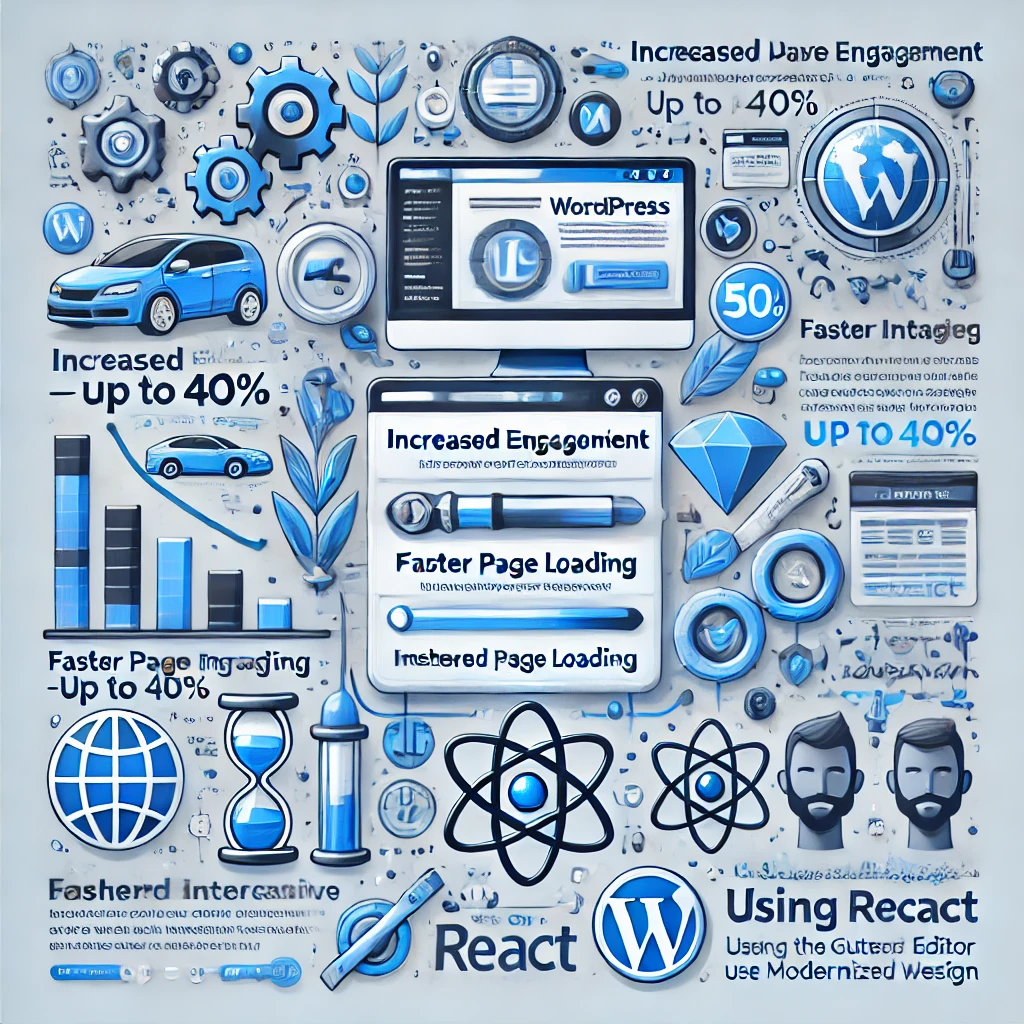
Studies have shown that interactive websites improve user experience and boost metrics like dwell time, conversion rates, and page views. In fact, according to recent statistics, websites with advanced JavaScript features can see a 20-40% increase in user engagement compared to static sites. But to harness its full potential while maintaining performance, security, and scalability, it’s crucial to follow advanced best practices when integrating JavaScript into your WordPress site. This article will explore in-depth strategies and considerations for effectively managing JavaScript in WordPress, ensuring that your site stays optimized, secure, and future-proof.
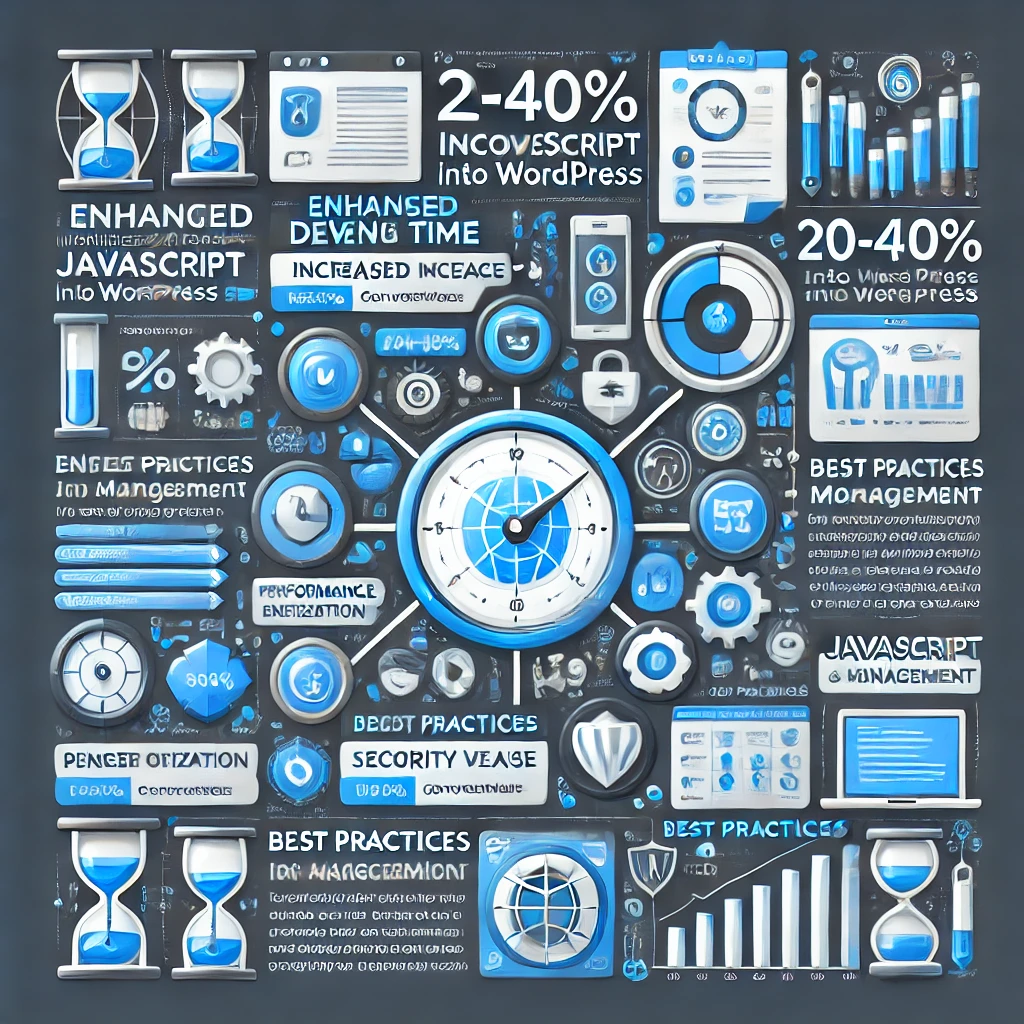
1. Understanding JavaScript’s Role in WordPress
JavaScript is no longer a supplementary language; it’s at the core of many modern websites, including WordPress-based ones. Through frameworks like React (used by WordPress’s Gutenberg editor), JavaScript enables real-time interactivity, such as dynamic content loading, form validation, image sliders, and more. These features not only improve user experience but can also increase conversions, reduce bounce rates, and make content more engaging.
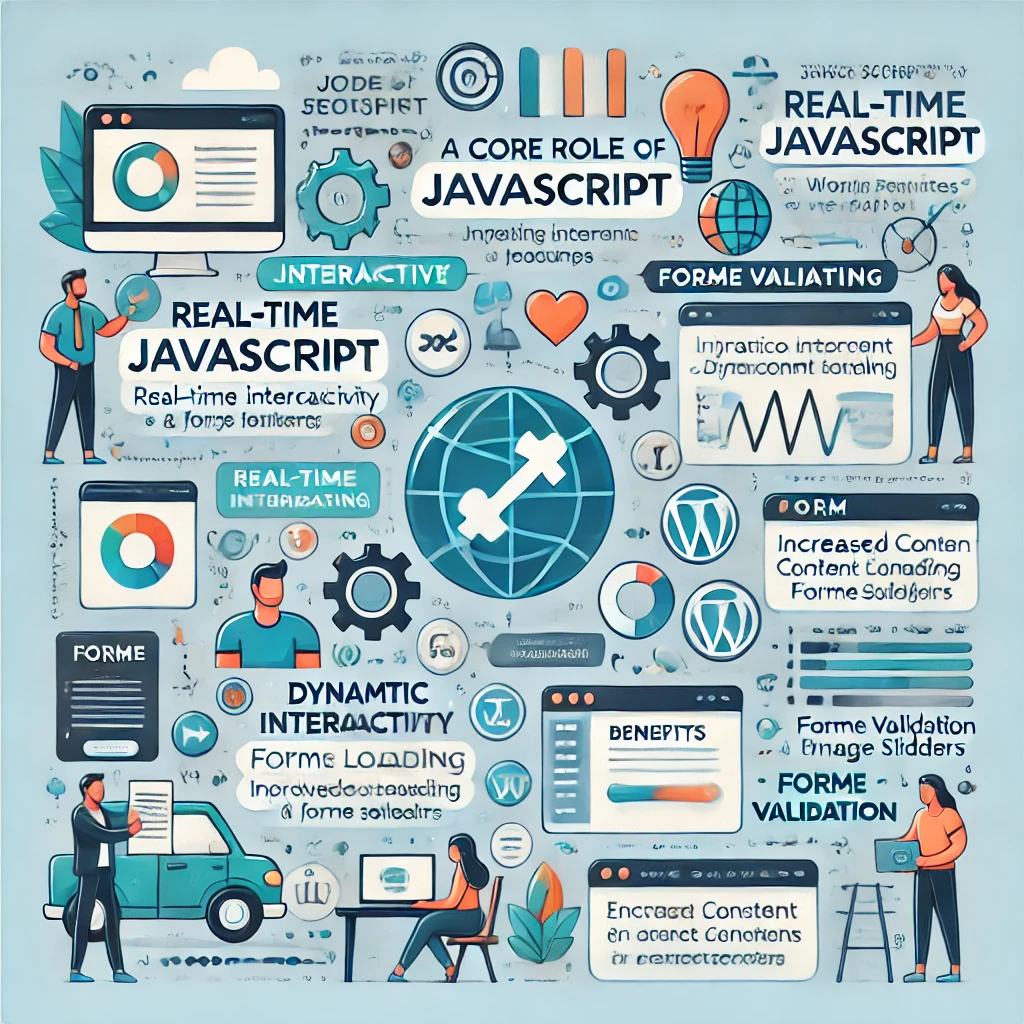
Moreover, modern frameworks like Vue.js and Angular are also being used in WordPress development to create Single Page Applications (SPAs) and deliver highly interactive components. These frameworks integrate seamlessly with WordPress’s REST API, allowing developers to pull content dynamically and create a smooth user experience without reloading pages.
However, with great power comes great responsibility. Poorly managed JavaScript can lead to issues like longer load times, security vulnerabilities, and conflicts with other plugins or themes. This makes understanding and following best practices essential.
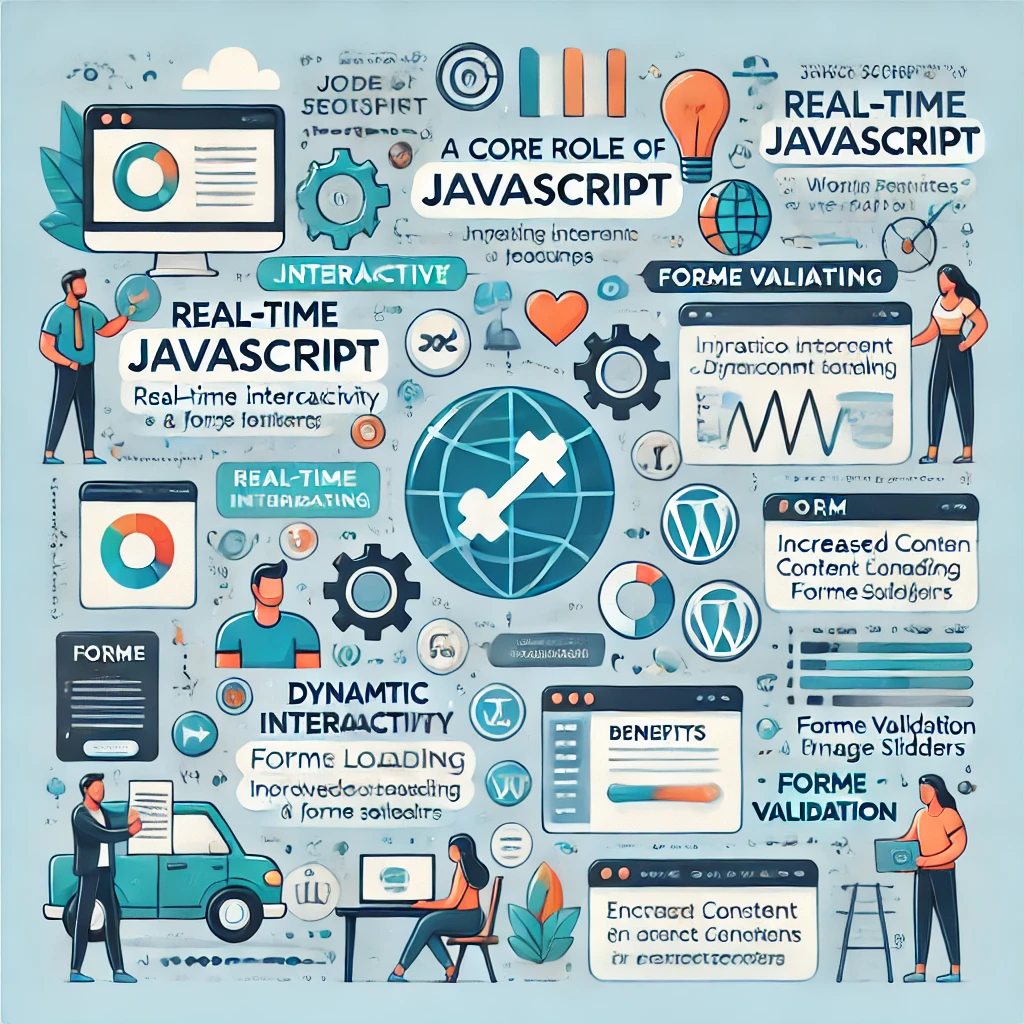
2. Optimal Methods for Adding JavaScript to WordPress
JavaScript is no longer a supplementary language; it’s at the core of many modern websites, including WordPress-based ones. JavaScript enables real-time interactivity through frameworks like React (used by WordPress’s Gutenberg editor), such as dynamic content loading, form validation, image sliders, and more. These features improve user experience and can increase conversions, reduce bounce rates, and make content more engaging.
Moreover, modern frameworks like Vue.js and Angular are also used in WordPress development to create single-page applications (SPAs) and deliver highly interactive components. These frameworks integrate seamlessly with WordPress’s REST API, allowing developers to pull content dynamically and make a smooth user experience without reloading pages.
However, with great power comes great responsibility. Poorly managed JavaScript can lead to longer load times, security vulnerabilities, and conflicts with other plugins or themes. This makes understanding and following best practices essential.
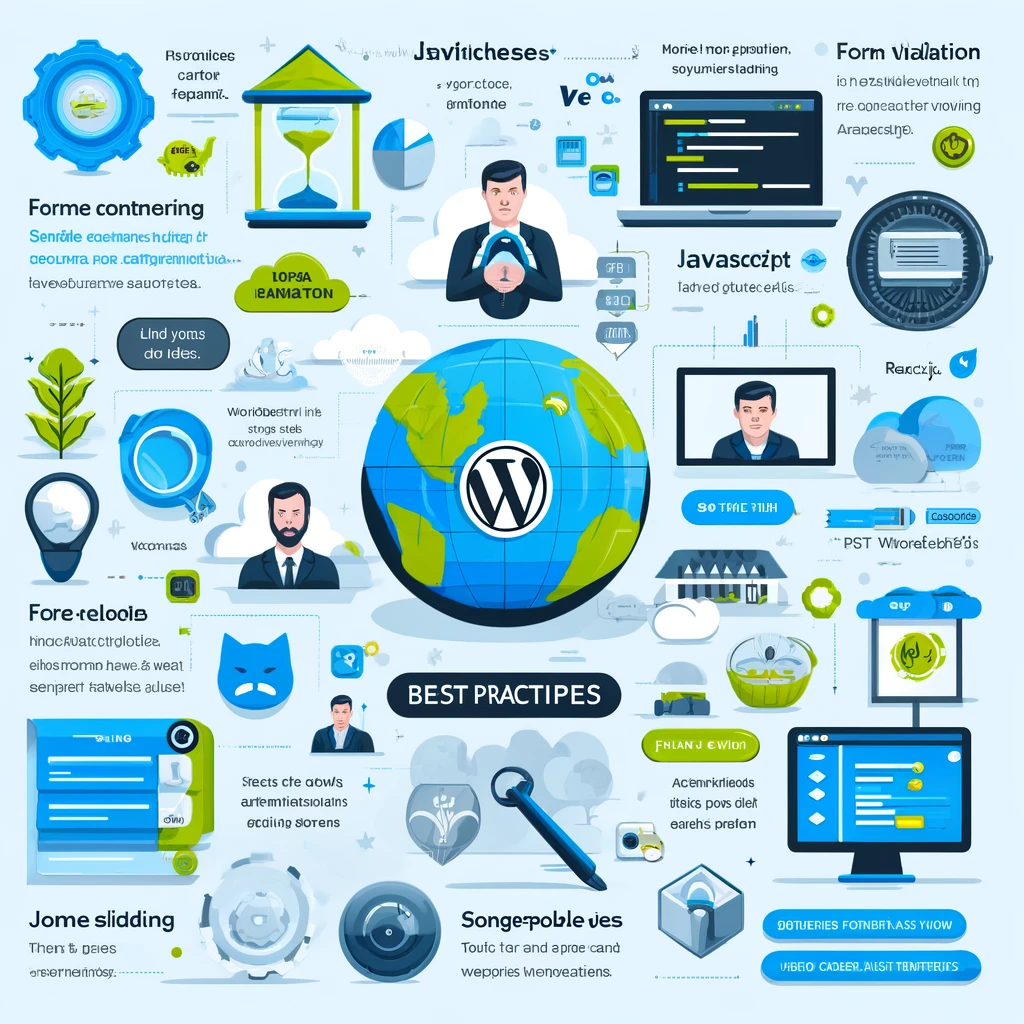
There are multiple methods for adding JavaScript to a WordPress site, but they aren’t all created equal. Here are the most effective ones:
A. Enqueuing JavaScript in functions.php
To ensure your JavaScript loads only when and where it’s needed, enqueuing is the preferred method. The wp_enqueue_script()
function allows you to add JavaScript with precise control over dependencies, file locations, and load order.
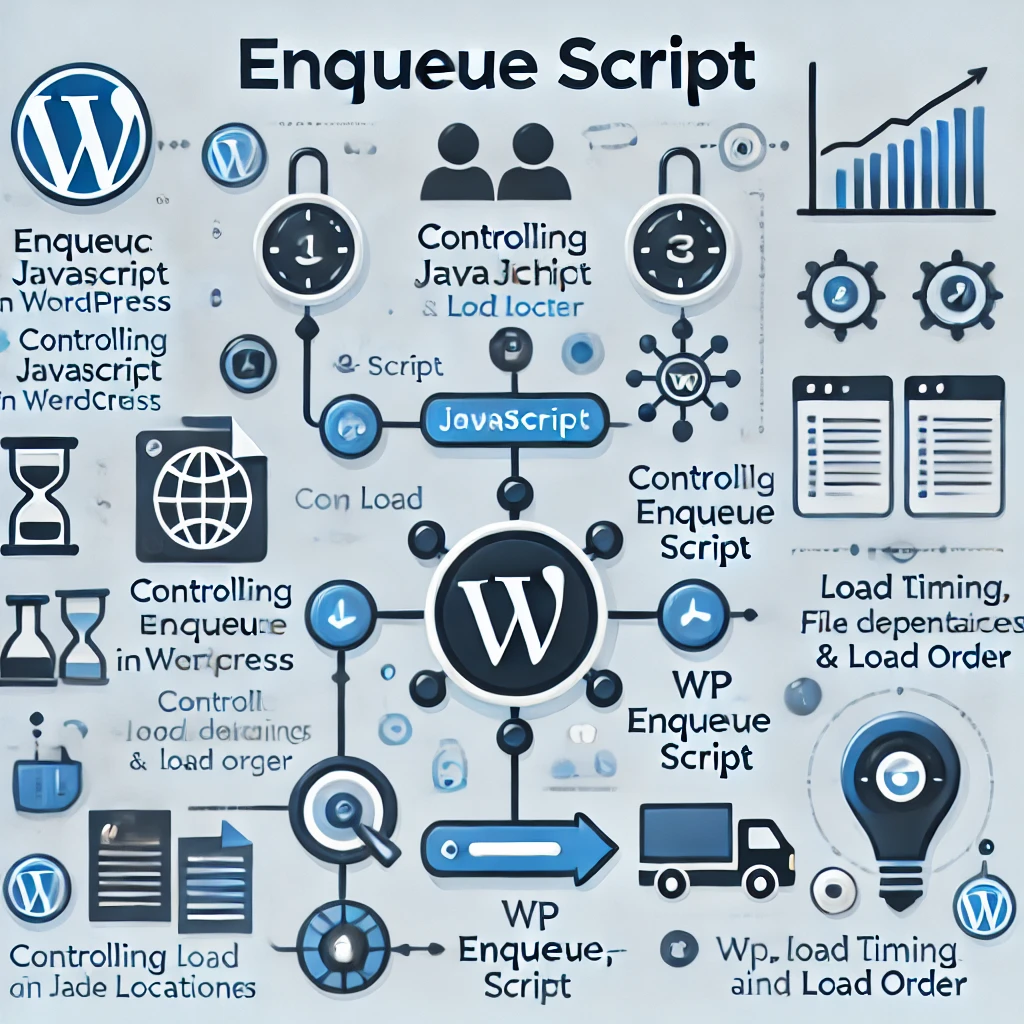
Example:
function my_custom_scripts() {
wp_enqueue_script('custom-js', get_template_directory_uri() . '/js/custom-script.js', array('jquery'), null, true);
}
add_action('wp_enqueue_scripts', 'my_custom_scripts');
Why Enqueue? This method minimizes redundancy, reducing code bloat. It also keeps code organized and centralized, allowing WordPress to handle dependencies and ensure compatibility across themes and plugins.
Use Case: For an e-commerce site, enqueuing JavaScript for product sliders ensures they load only on product pages, enhancing page speed without impacting other sections of the site. For large-scale websites like online magazines or media platforms, enqueuing scripts helps manage performance effectively by ensuring only necessary scripts are loaded on specific pages, thereby optimizing resource usage.
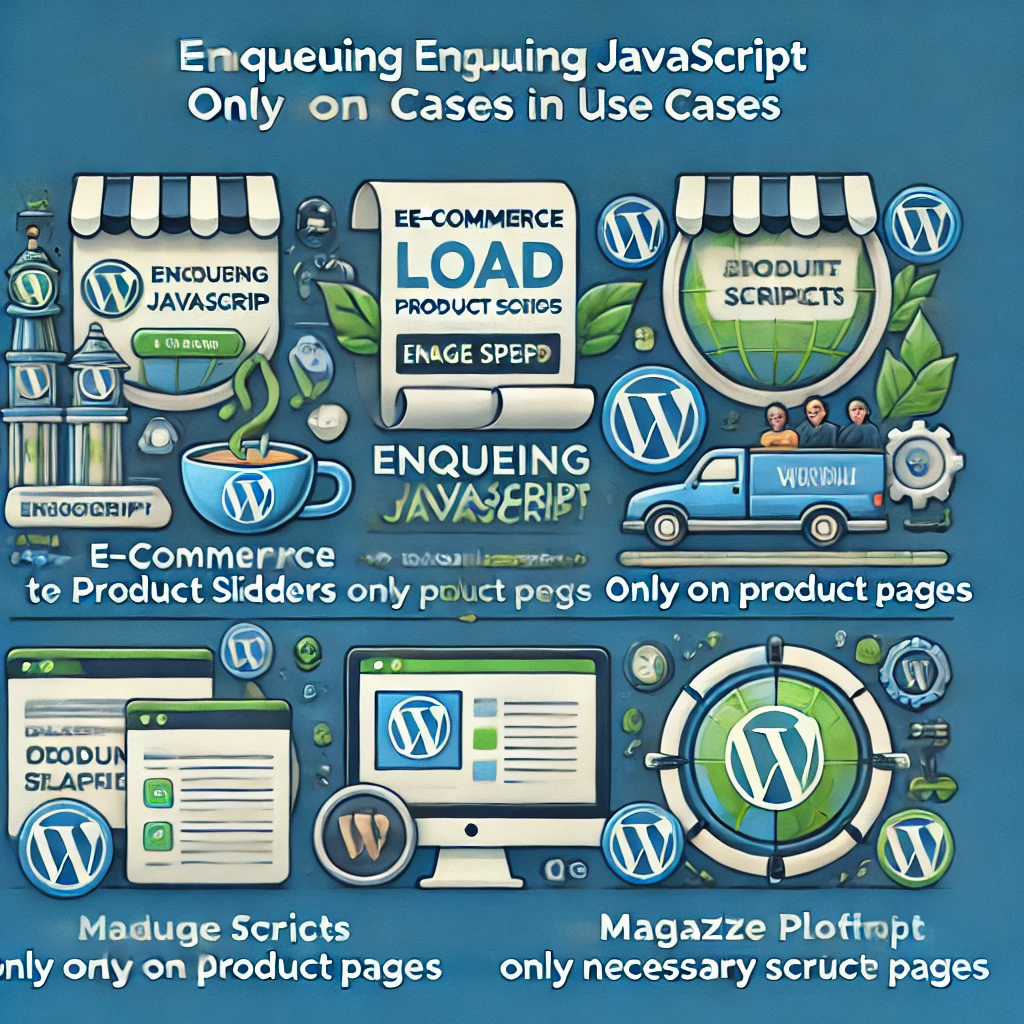
B. Using JavaScript Injection Plugins
For non-technical users, plugins such as Insert Headers and Footers and WPCodeBox provide a user-friendly way to add JavaScript to specific areas of the site, like headers or footers. This can be ideal for tracking scripts or third-party integrations that don’t require heavy customization.
Use Case: A blog might use a JavaScript injection plugin to add Google Analytics tracking to all pages without manually editing theme files. For small businesses that need to integrate third-party widgets like chatbots or advertising scripts, these plugins provide an easy solution without requiring deep technical knowledge.
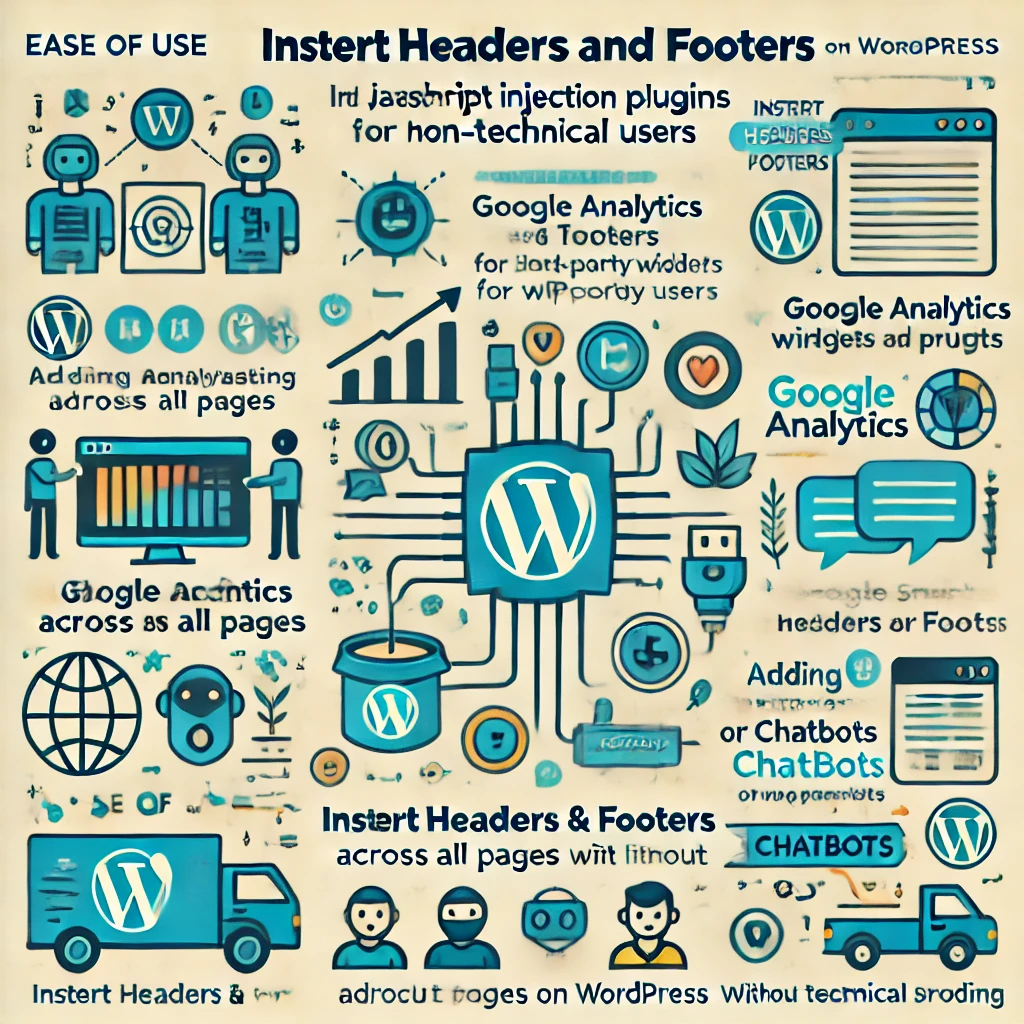
C. Leveraging Gutenberg’s Block Editor
If you’re working with the Gutenberg editor, custom JavaScript can be applied on a block level, enabling unique functionality on individual blocks. This is useful for adding interactions to specific sections without affecting the entire site.
Use Case: A portfolio site could use JavaScript to create hover animations for specific Gutenberg blocks, adding interactivity to showcased projects. Similarly, an educational site could use custom JavaScript to enhance quizzes and interactive learning modules, making content more engaging for users.
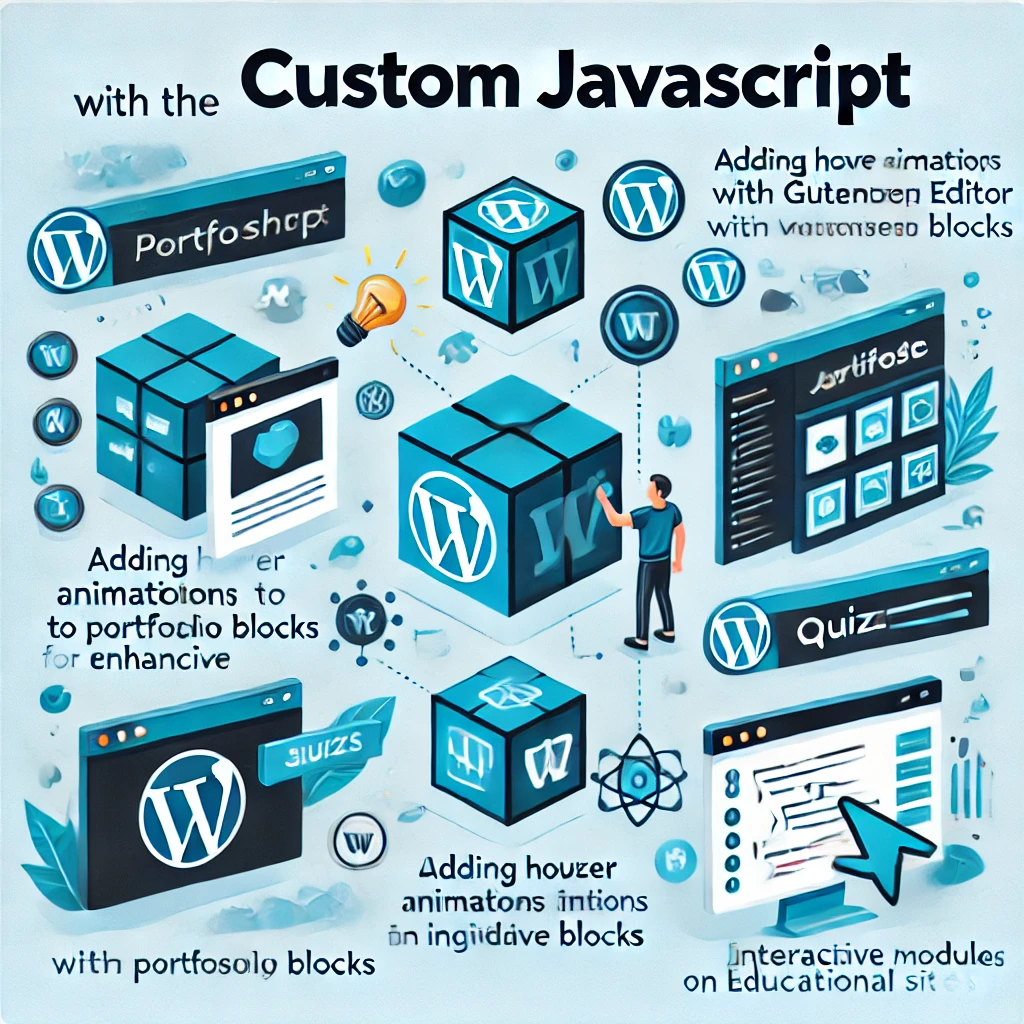
3. Advanced Best Practices for Managing JavaScript in WordPress
To keep your site fast, secure, and scalable, adhere to these advanced best practices when working with JavaScript in WordPress.
A. Minify and Combine JavaScript Files
For optimal performance, reduce the number of JavaScript files by combining them into a single file and minimizing their size:
- Minification: Removing unnecessary whitespace and comments from JavaScript files reduces their size, speeding up page load times.
- Combining Files: By consolidating multiple scripts, you reduce the number of HTTP requests the server has to handle.
- Tool Recommendations: WP Rocket, Autoptimize, and WP Fastest Cache offer robust options for combining and minifying files automatically.
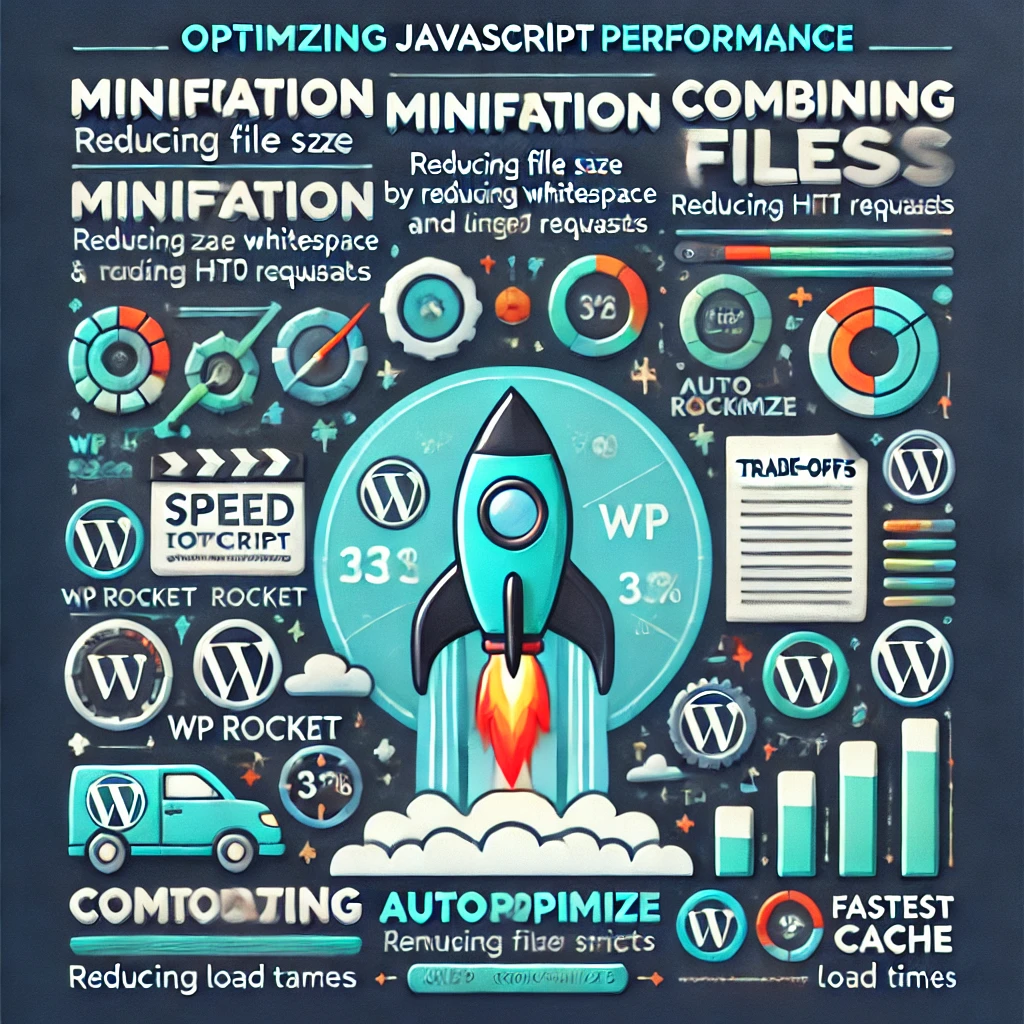
Trade-Offs: Combining JavaScript files can lead to larger single files, which may negatively impact initial load times if not done correctly. Use this approach carefully for sites with numerous scripts. For example, a large media site might need to selectively combine scripts to avoid creating overly large files that slow down page loads.
B. Enable Asynchronous and Deferred Loading
JavaScript can slow down the critical rendering path, so it’s important to load it asynchronously whenever possible:
- Async vs. Defer: Using
async
allows scripts to load simultaneously with other content, whiledefer
waits until the page fully loads. This is crucial for non-essential JavaScript, such as analytics, which doesn’t need to block rendering.
Example:
function add_async_defer($tag, $handle) {
if ('custom-js' !== $handle) {
return $tag;
}
return str_replace(' src', ' async="async" src', $tag);
}
add_filter('script_loader_tag', 'add_async_defer', 10, 2);
Use Case: For a portfolio site, loading non-critical scripts like animations using defer
ensures that primary content loads quickly, improving user experience. Performance metrics show that using defer
for non-essential scripts can decrease load times by up to 30%, resulting in a smoother user experience and reduced bounce rates.
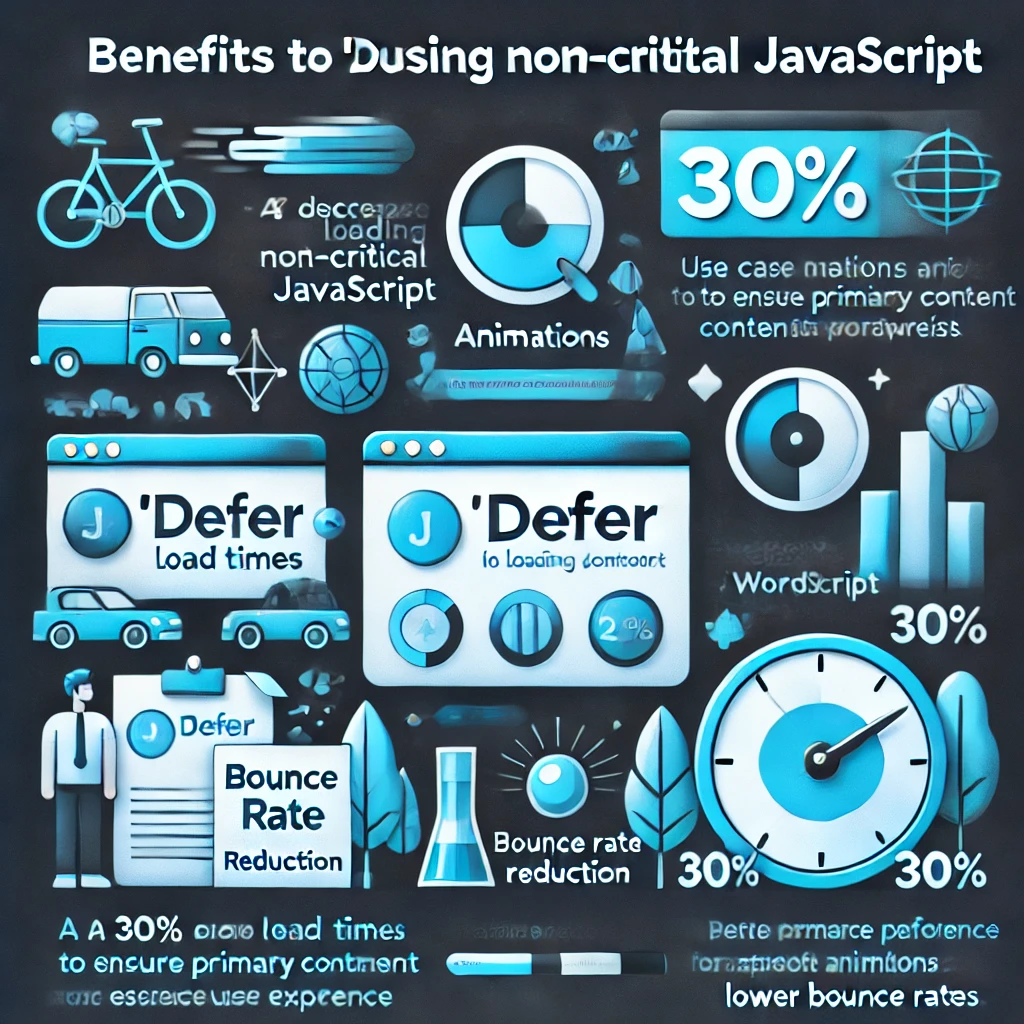
C. Always Use a Child Theme for Customizations
Editing functions.php
directly in the main theme folder is not recommended, as theme updates will overwrite customizations. Instead:
- Child Themes: Create a child theme where you can safely modify
functions.php
without risking compatibility issues or losing your customizations during updates. - How to Set Up: Copy the parent theme’s files into a new directory, include a
style.css
file, and enqueue JavaScript as needed in the child theme’sfunctions.php
.
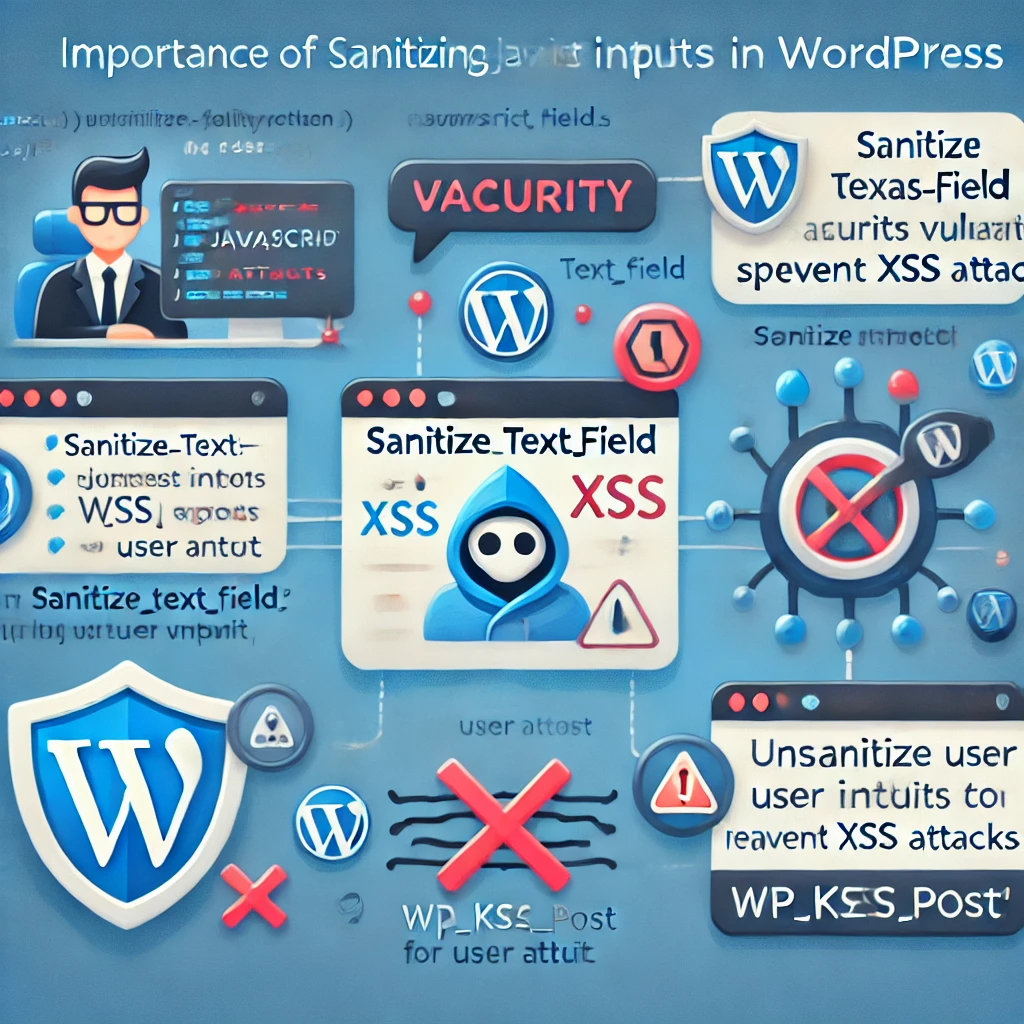
4. Enhancing Security in JavaScript Management
JavaScript is often a vector for security vulnerabilities, especially through cross-site scripting (XSS) attacks. Here’s how to secure your code:
A. Sanitize User Inputs
Always sanitize any input that could be inserted into JavaScript, especially if it originates from user data. WordPress functions like sanitize_text_field()
and wp_kses_post()
help filter inputs.
Common Vulnerability: If user-generated content is used without sanitization, it may lead to XSS attacks, where malicious scripts can be injected into your site. Always sanitize data to prevent this.
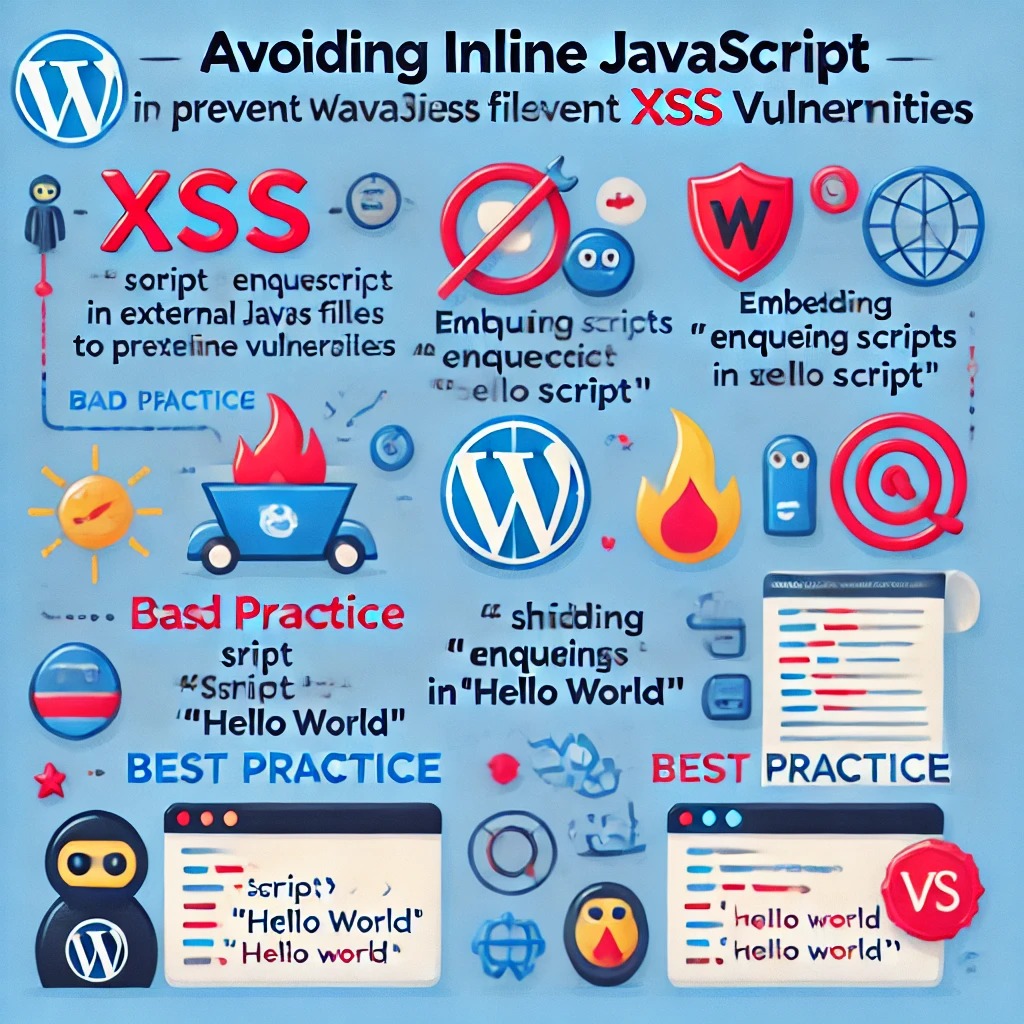
B. Avoid Inline JavaScript
Avoid inline JavaScript wherever possible, as it can introduce XSS vulnerabilities. Instead, enqueue scripts properly and store them in separate files.
Bad Practice Example: Adding <script>alert('Hello World');</script>
directly in a post introduces security risks. Instead, enqueue the script in an external file.
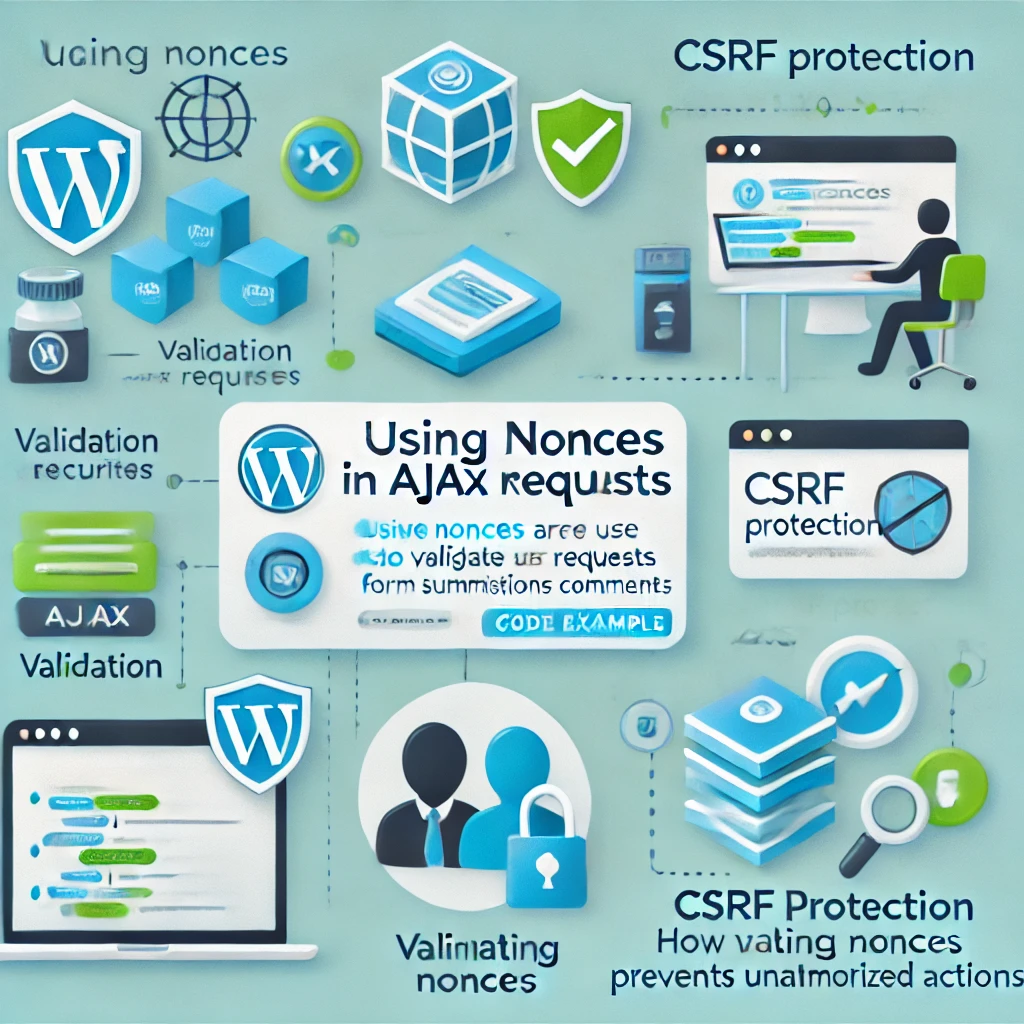
C. Use Nonces for AJAX Requests
When handling AJAX requests in WordPress, use nonces (number used once) to validate requests and prevent unauthorized access:
Example:
function my_ajax_script() {
wp_enqueue_script('ajax-script', get_template_directory_uri() . '/js/ajax.js', array('jquery'), null, true);
wp_localize_script('ajax-script', 'my_ajax_obj', array(
'ajax_url' => admin_url('admin-ajax.php'),
'nonce' => wp_create_nonce('my_nonce')
));
}
add_action('wp_enqueue_scripts', 'my_ajax_script');
Use Case: For a membership site, using nonces ensures that only authorized users can perform actions like submitting forms or posting comments, thereby enhancing security. Additionally, implementing CSRF (Cross-Site Request Forgery) protections by validating nonces for all AJAX requests can further prevent unauthorized actions.
5. Advanced Performance Optimization Techniques
A. Modular JavaScript Techniques
To maintain scalability and performance, consider breaking your JavaScript code into modules. Modular JavaScript helps keep your codebase organized and maintainable.
- Code Splitting: Split large JavaScript files into smaller modules that load only when needed. This can be particularly useful in WordPress when certain features are only required on specific pages.
- Tools: Use Webpack or Gulp to manage JavaScript assets, bundle code, and apply optimizations like tree shaking, which removes unused code.
Use Case: A large-scale e-commerce site could use code splitting to load cart functionality only when a user navigates to the checkout page, thereby improving initial load speed for product listing pages.
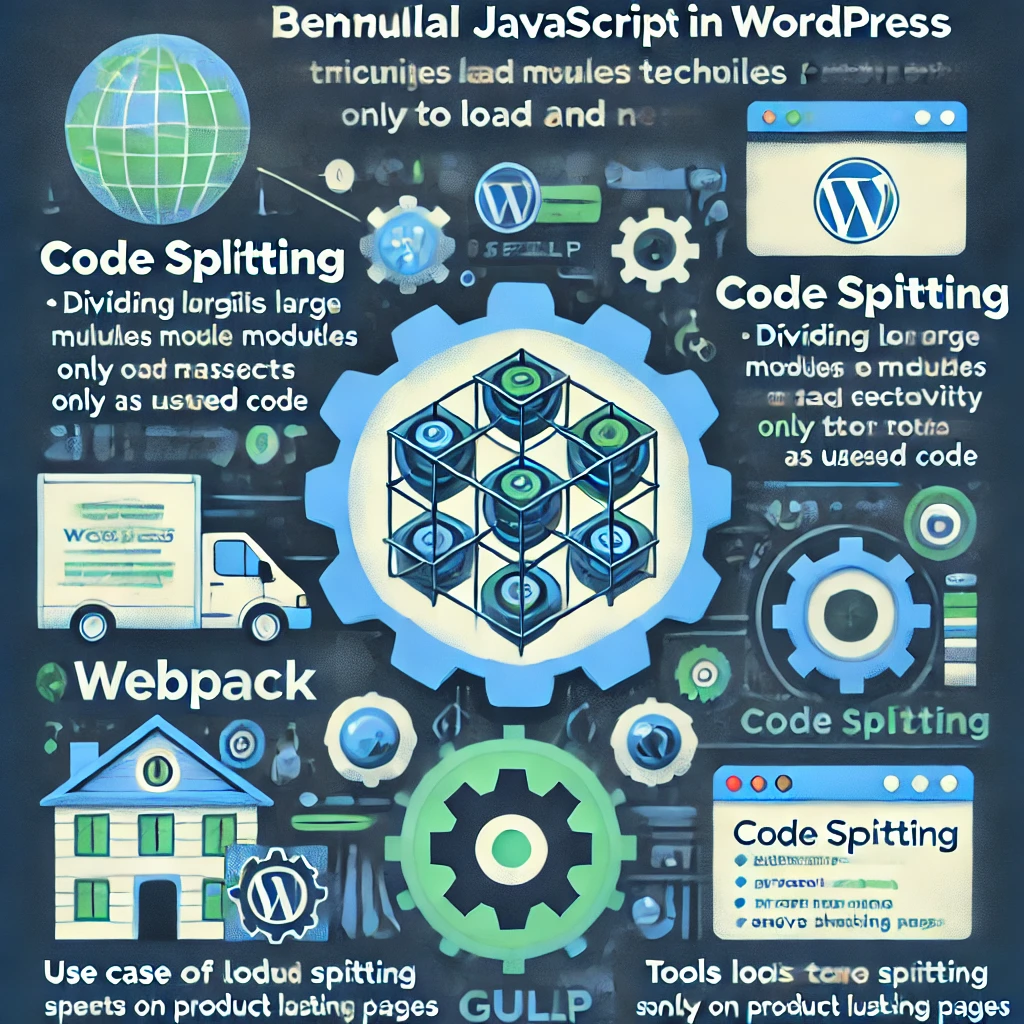
6. JavaScript Testing in Depth
Thorough testing is essential to avoid JavaScript errors that can disrupt the user experience. Here’s a guide to testing effectively:
A. Unit Testing with Jest
Use Jest to write unit tests for your JavaScript code, ensuring that individual functions perform as expected.
Example: Write tests for utility functions used across your WordPress theme to validate their output under different conditions.
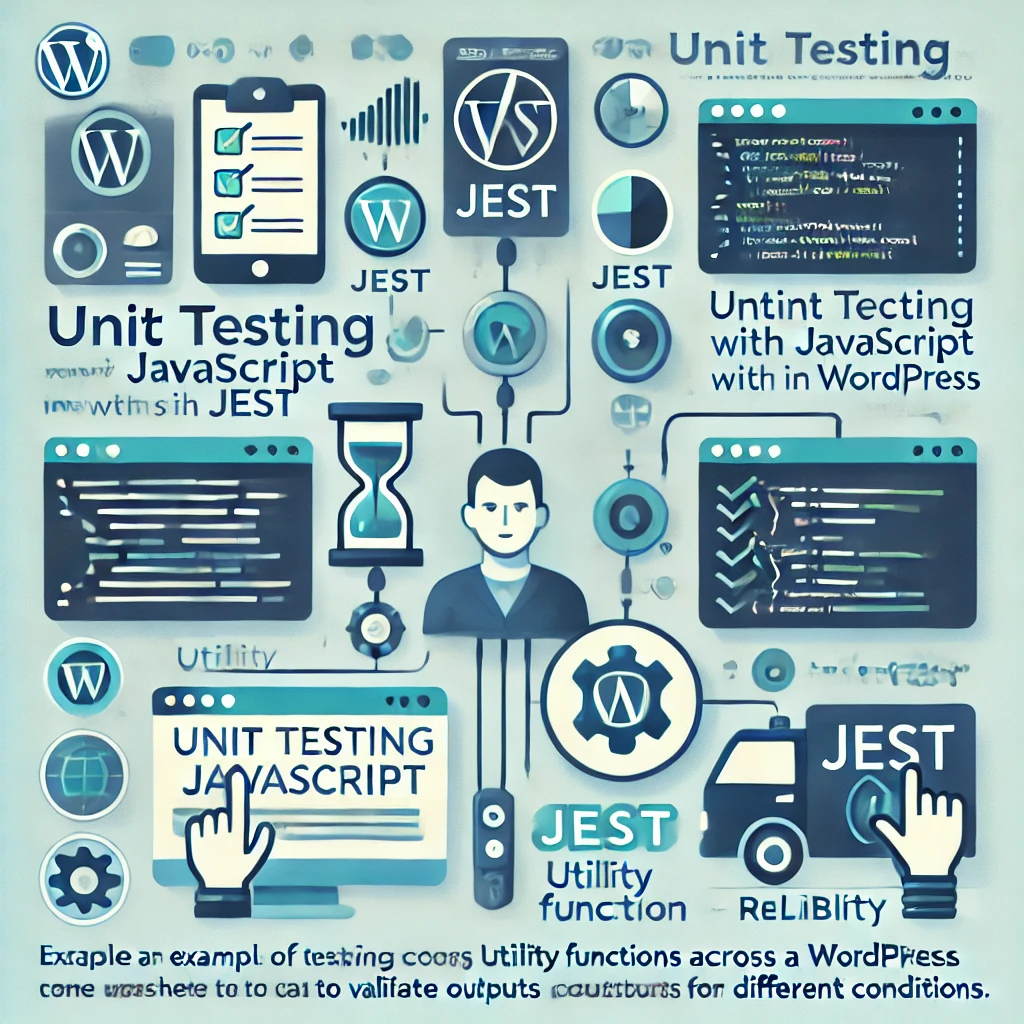
B. Integration Testing in a WordPress Environment
Integration testing ensures that your JavaScript works well within the WordPress environment, interacting correctly with other scripts and plugins.
- Tools: Cypress is an excellent tool for end-to-end testing, particularly for user interactions on your WordPress site, such as form submissions, button clicks, and AJAX requests.
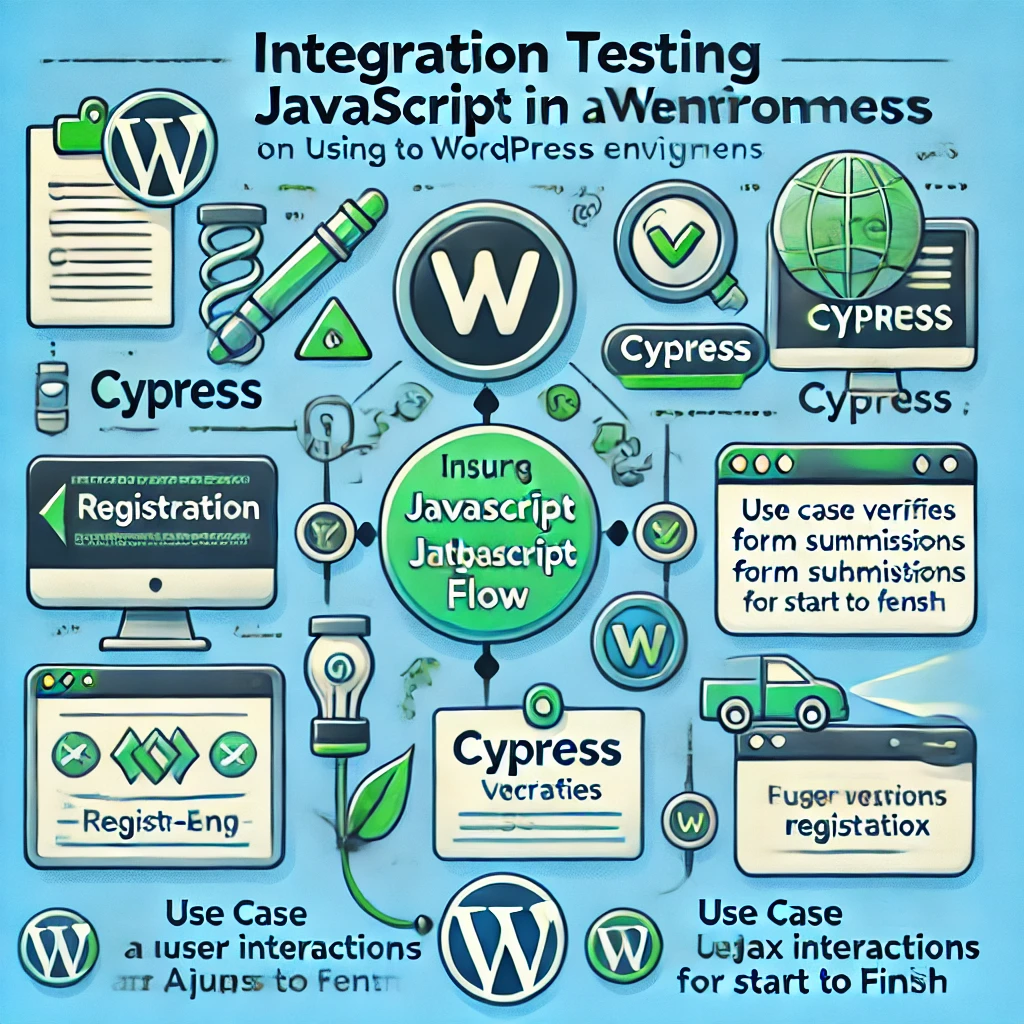
Use Case: For a membership site, use Cypress to test the entire registration flow, from entering user details to receiving a confirmation email, ensuring that JavaScript-driven interactions work seamlessly.
C. Use Browser Developer Tools
The developer console in Chrome, Firefox, or Edge provides insights into errors, performance issues, and network activity, making it easy to debug issues quickly.
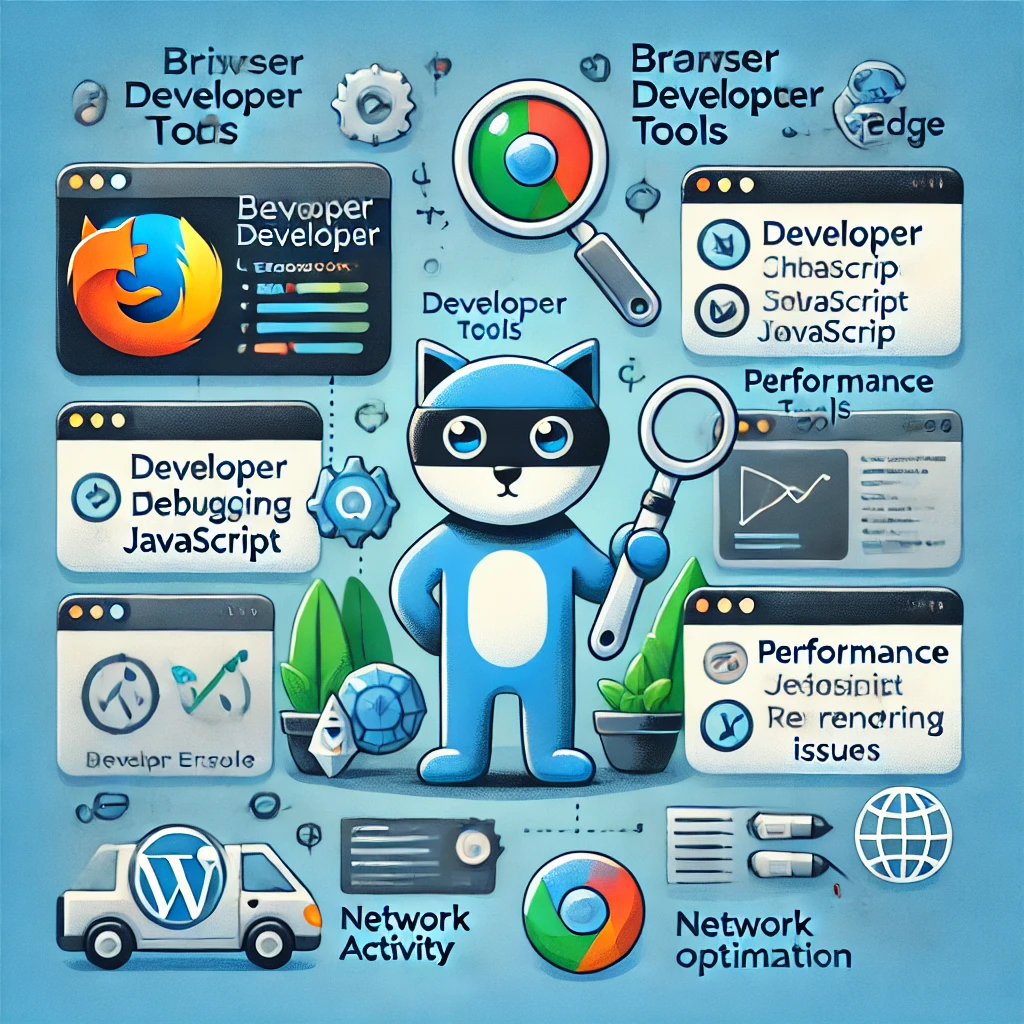
D. Conduct Cross-Browser Testing
Different browsers interpret JavaScript differently. Testing across Chrome, Firefox, Safari, and Edge ensures consistency for all users.
E. Use Tools like Google Lighthouse for Performance
Google Lighthouse and PageSpeed Insights analyze your page performance, identifying areas where JavaScript impacts load times and offering suggestions for improvement.
Additional Testing Tools: Plugins like Query Monitor can help identify JavaScript-related issues specific to WordPress. Jest can also be used for unit testing JavaScript functionality.
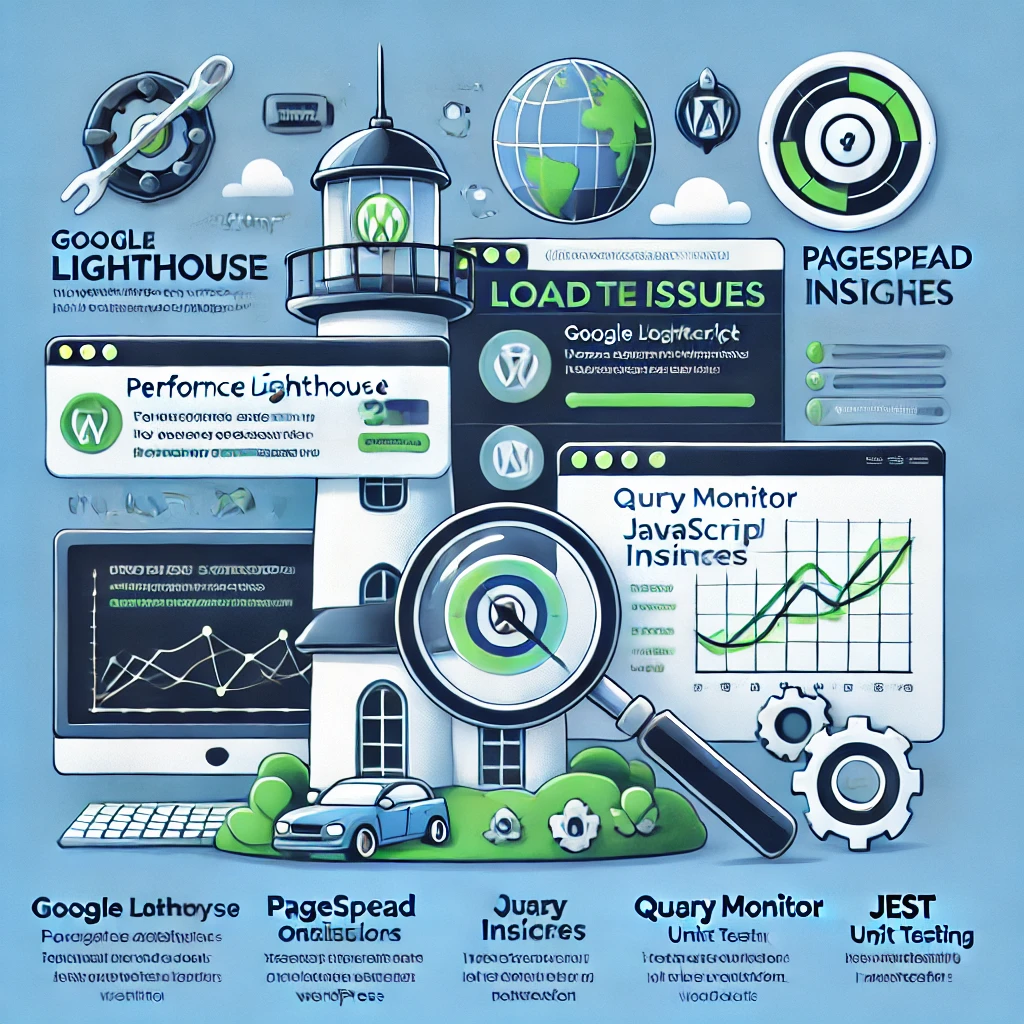
7. JavaScript Optimization Plugins
WordPress offers plugins specifically for optimizing JavaScript, making it easier to manage advanced configurations without in-depth coding knowledge:
- Autoptimize: Combines, minifies, and defers JavaScript files, along with additional caching options for improved performance.
- WP Rocket: A premium caching plugin with extensive optimization features for CSS and JavaScript, including lazy loading and deferred script loading.
- Asset CleanUp: Allows you to unload JavaScript on specific pages, reducing unnecessary code on pages where certain scripts aren’t needed.
Tool Comparisons: WP Rocket is ideal for users who need an all-in-one caching and optimization solution, while Autoptimize is great for those looking for a free option with robust features. Asset CleanUp is particularly useful for targeting specific pages to reduce load. For instance, a personal blog may only need Autoptimize for simple optimizations, whereas an online store with complex functionality might benefit more from WP Rocket’s premium features.
8. JavaScript and SEO Considerations
JavaScript can impact SEO, especially in how search engines crawl and index content. Here are some tips to ensure JavaScript does not harm your SEO:
- Server-Side Rendering (SSR): If you’re using heavy JavaScript for content, consider using SSR to ensure search engines can easily crawl your content.
- Lazy Loading: Properly implemented lazy loading can improve SEO by ensuring above-the-fold content loads quickly.
- Google Search Console: Use Google Search Console to ensure your JavaScript-based content is being indexed correctly.
SEO Challenges: Search engines may struggle with indexing JavaScript-heavy content. For example, delayed rendering caused by client-side JavaScript can lead to missing content in SERPs. To mitigate this, ensure that critical content is server-rendered or use prerendering tools to create snapshots of your pages for search engines.
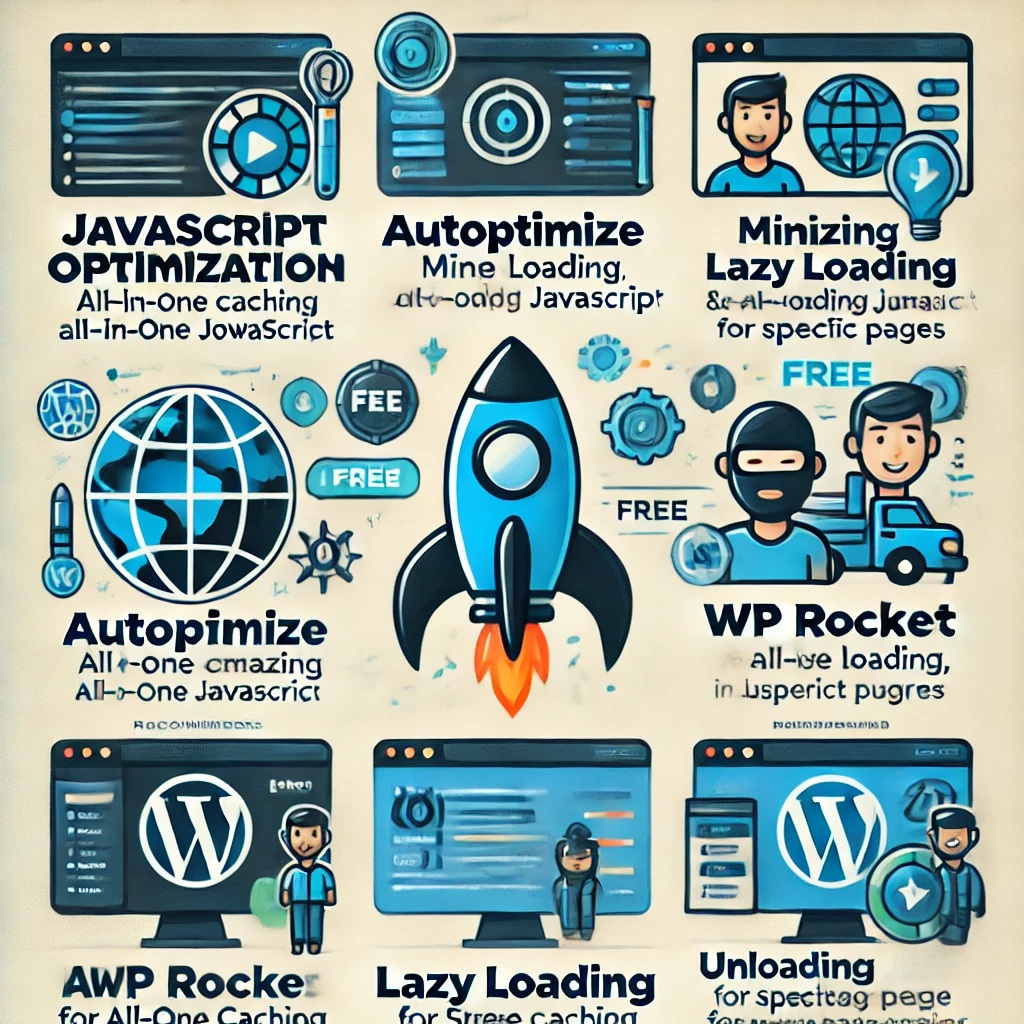
9. Visual Aids
Adding diagrams or flowcharts to explain JavaScript loading methods (async
, defer
, enqueuing) could make the article more visually engaging and help readers understand these concepts more clearly. Consider using simple flowcharts to explain loading strategies. For example, a flowchart illustrating the different stages of JavaScript loading—synchronous, asynchronous, and deferred—can help readers visualize the impact on page performance.
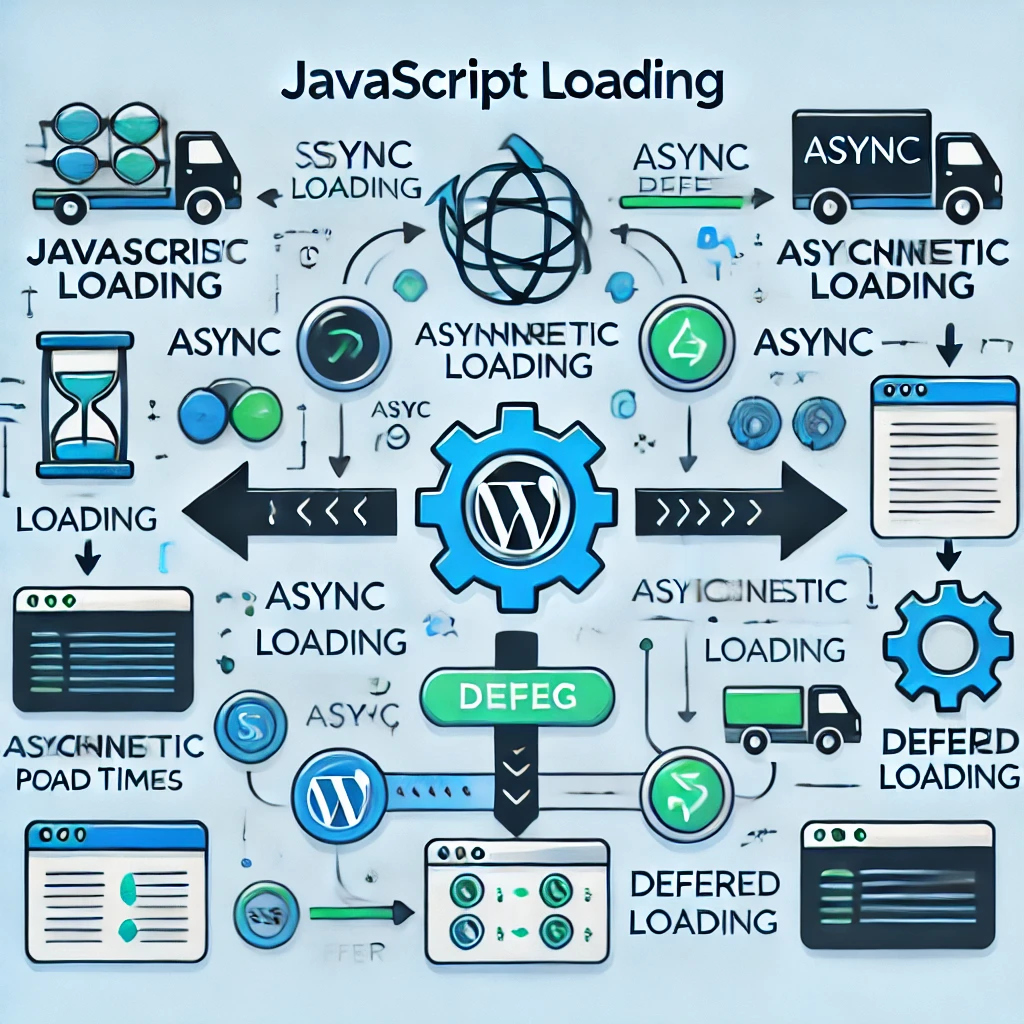
Conclusion
Adding JavaScript to WordPress opens up a world of functionality, interactivity, and user engagement. However, it’s essential to follow best practices and advanced strategies to ensure that your site remains optimized, secure, and maintainable. By enqueuing scripts, minimizing and combining files, enabling asynchronous loading, and taking security precautions, you can harness JavaScript’s power without sacrificing performance.
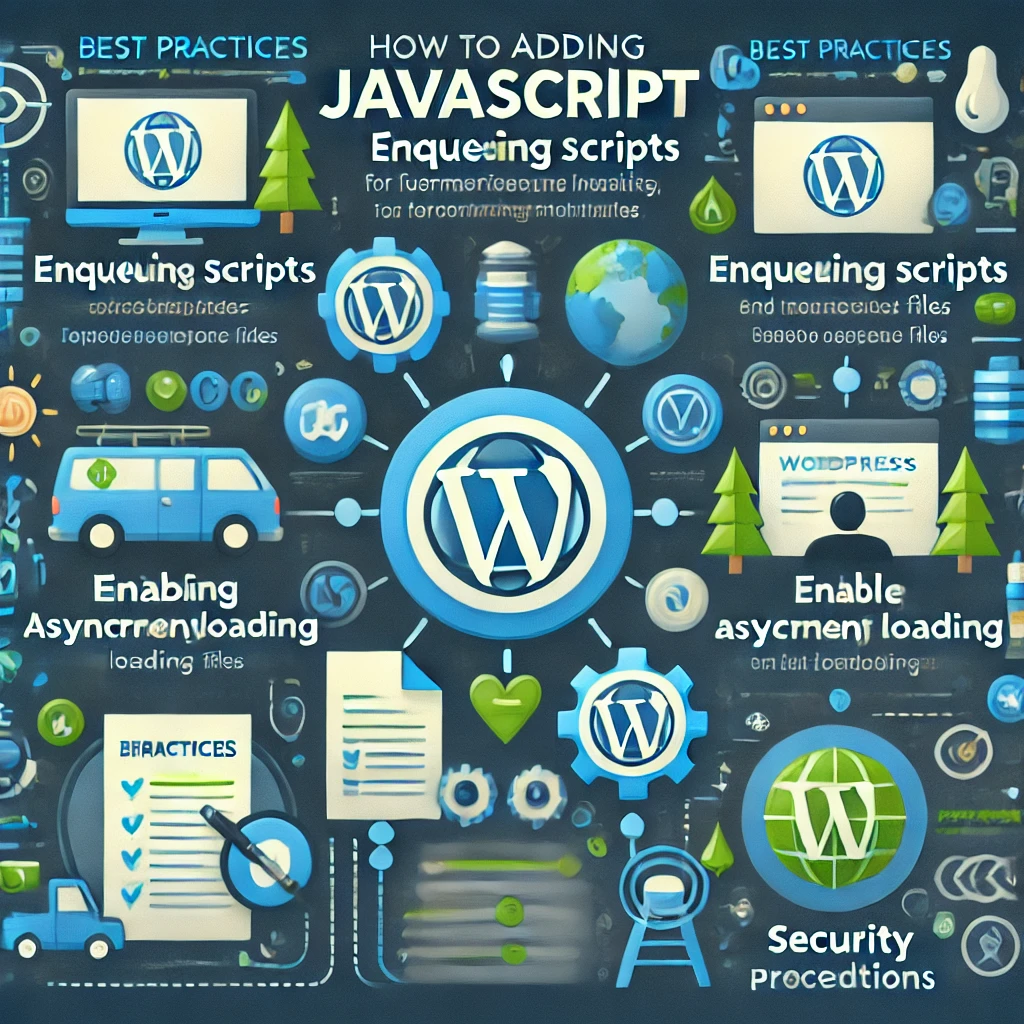
Actionable Next Steps:
- Create a Child Theme: Start by creating a child theme to make any JavaScript customizations safely.
- Enqueue JavaScript Properly: Use the
wp_enqueue_script()
method to ensure efficient and conflict-free loading. - Run Performance Tests: Utilize tools like Google Lighthouse before and after implementing JavaScript changes to assess their impact.
- Test Your Changes: Utilize browser developer tools, cross-browser testing, and tools like Cypress to ensure compatibility.
- Optimize for SEO: Use Google Search Console and ensure proper rendering for JavaScript-heavy content.
- Security Audit: Perform a security audit using a WordPress security plugin and ensure nonces are used for AJAX requests.
JavaScript is here to stay, and by mastering its integration into WordPress, you’re well-equipped to create a responsive, high-performance site that delights users and stands the test of time.
Responses