Enhancing WordPress with Custom Hooks and Filters: A Guide to Flexible Feature Additions
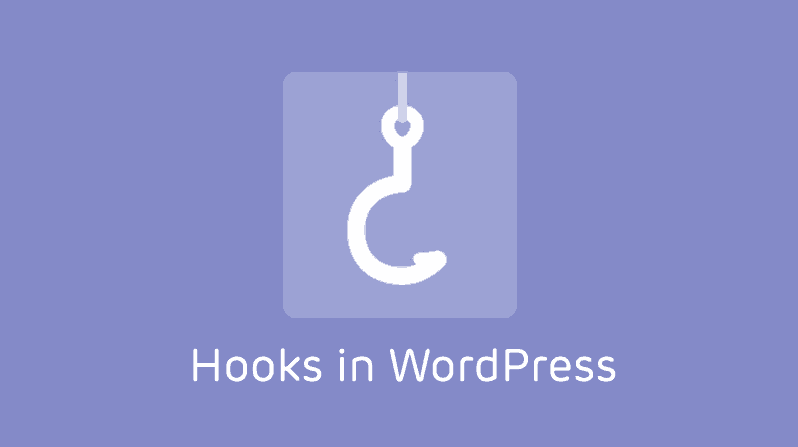
Have you ever wanted to modify a WordPress feature but hesitated because you didn’t want to risk altering core files? This is a common challenge developers face when aiming to customise their websites without compromising the site’s stability or risking future updates. Fortunately, WordPress offers a solution through its hooks system.
This article will explore how to use Actions and Filters to enhance WordPress functionality. We will guide you through practical examples, demonstrating how you can implement custom features seamlessly and avoid the risks associated with directly modifying core files.
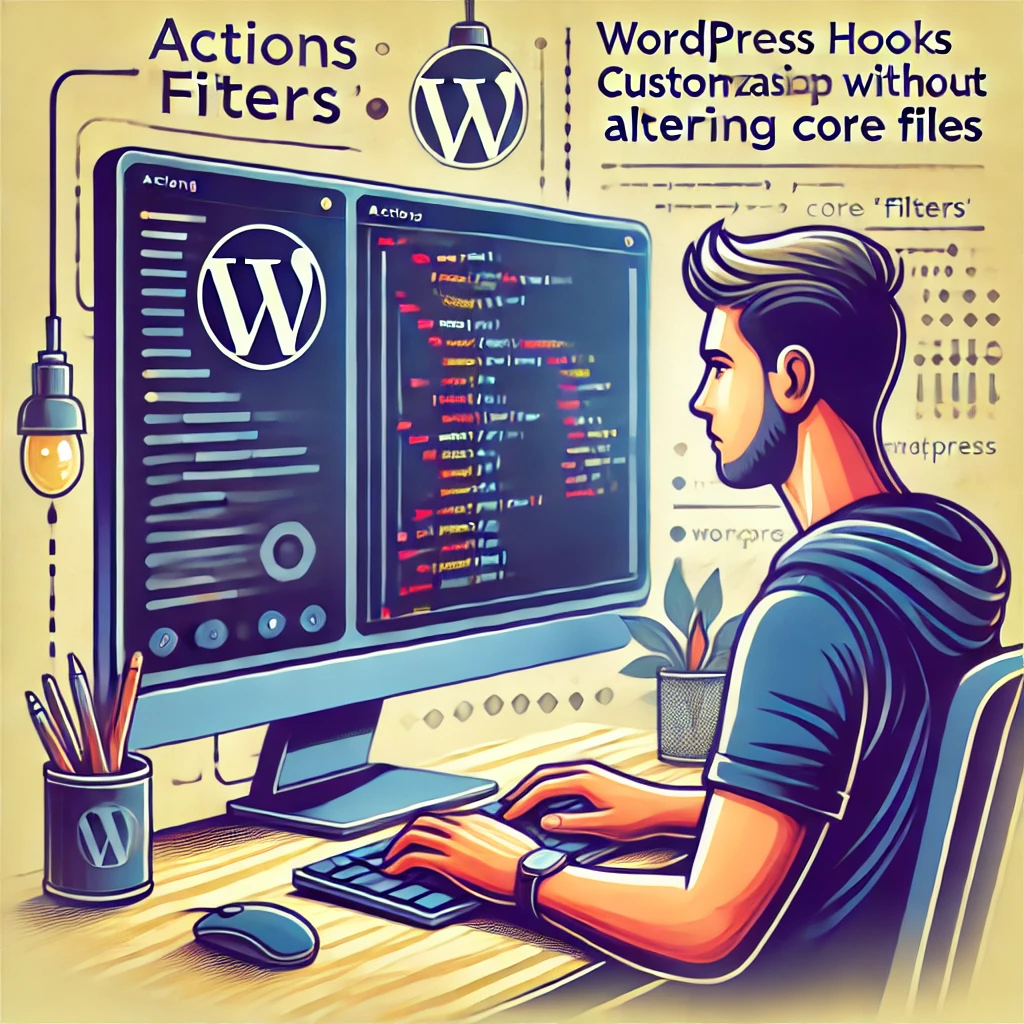
WordPress, renowned for its flexibility and robust ecosystem of themes and plugins, offers endless possibilities for building websites. However, one of the most remarkable yet often underutilised features is its hooks system, particularly Actions and Filters. These provide developers with a non-intrusive method to extend or modify functionality without touching the core WordPress files. By leveraging these hooks, you can make your WordPress site more robust, versatile, and tailored to your needs—all while maintaining best practices in web development.
The Power of Hooks in WordPress
In WordPress, hooks are predefined points within the codebase where you can insert your custom functions. WordPress will execute these functions at specific moments during its workflow. These hooks come in two main varieties:
- Actions: Allow you to run custom code at particular points in WordPress’s execution. For instance, you can send an email after a post is published or inject custom content before or after a post is displayed.
- Filters: Enable you to modify existing data before it is saved to the database or outputted to the front end. You can use filters to alter post content, titles, metadata, or anything else that passes through WordPress’s pipeline.

What Are Actions and How Do They Work?
Actions are used to perform tasks or trigger events at specific points in WordPress’s lifecycle. During operation, WordPress fires numerous actions, from initialising the site to rendering the content and managing user logins. By hooking into these points, you can add custom functionality without disrupting the normal flow of the core code.
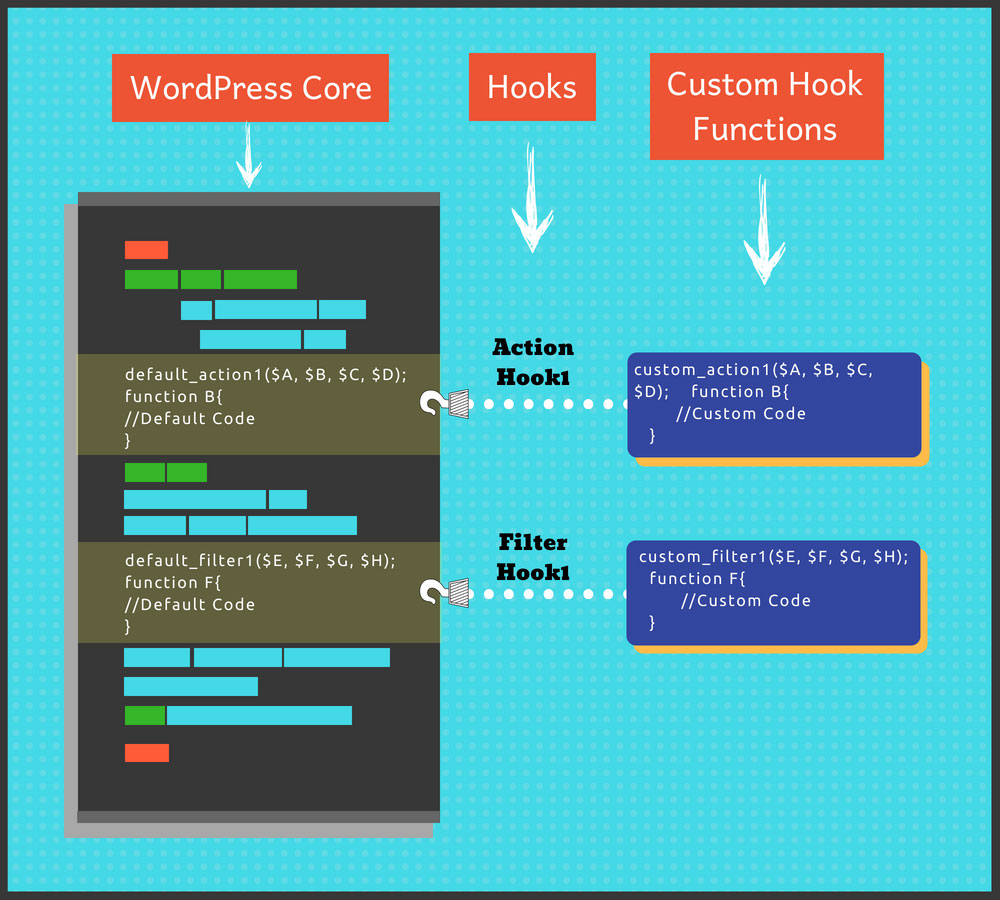
Example: Adding Custom Messages to Posts
Let us say you want to add a custom message at the end of every blog post to encourage readers to subscribe to your newsletter. With an Action hook, this can be done quickly without editing your theme’s template files.
function add_custom_message_to_post($content) {
if (is_single()) {
$content .= '<p>Thanks for reading! Don’t forget to subscribe to our newsletter for the latest updates.</p>';
}
return $content;
}
add_action('the_content', 'add_custom_message_to_post');
In this example:
- the_content is the hook that WordPress fires when rendering post content.
- add_custom_message_to_post is the function that appends the message to the post content.
This approach allows you to enhance your site’s functionality without needing to alter your theme’s core files, making your changes future-proof and easier to maintain.
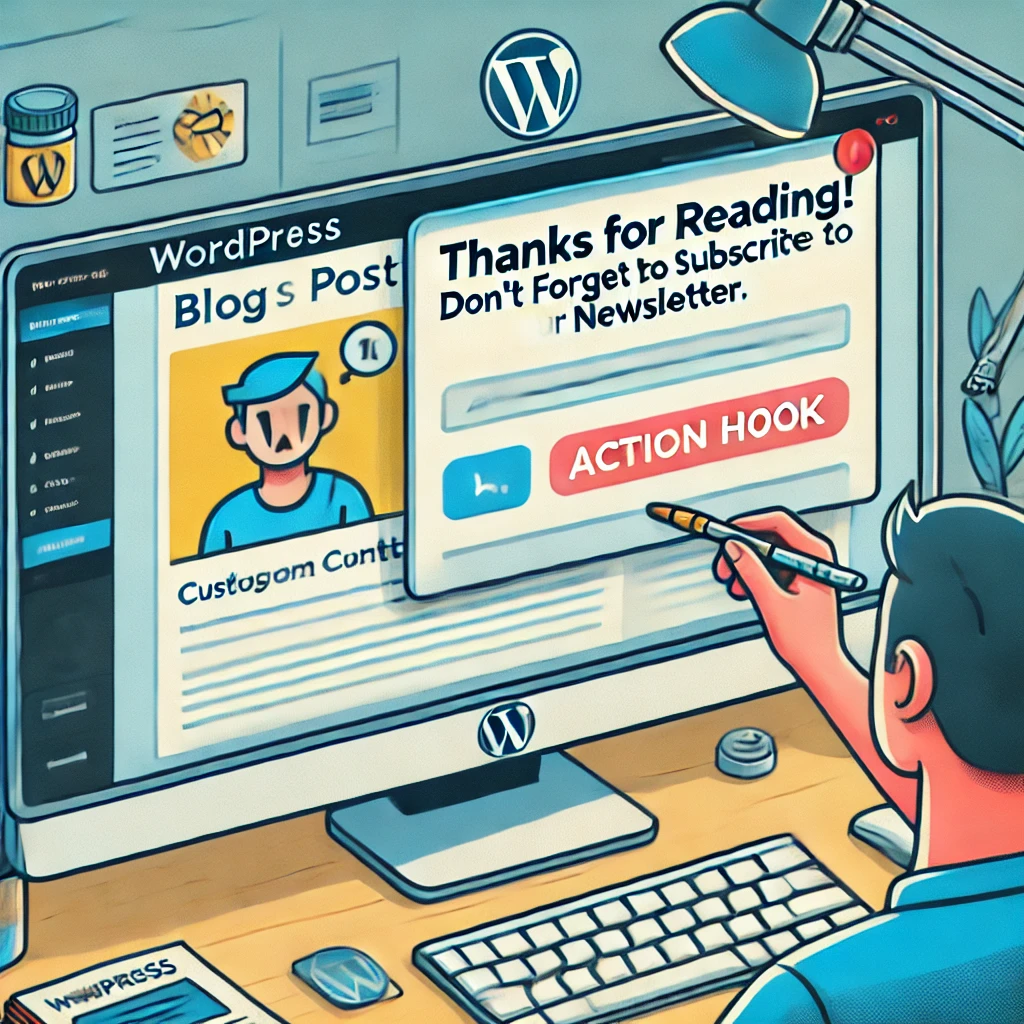
Example: Triggering an Action After a Post Is Published
Another everyday use of Actions is to trigger a custom function when a new post is published. For example, you might want to send a tweet or email notification whenever a new post goes live.
function notify_on_publish($ID, $post) {
$message = 'A new post titled "' . $post->post_title . '" has just been published. Check it out: ' . $post->guid;
// You can send this message via email or use a social media API to tweet it
}
add_action('publish_post', 'notify_on_publish', 10, 2);
In this example:
publish_post is the Action that is triggered whenever a post is published.
notify_on_publish is the function that handles the notification logic.
Using actions in this way, you can automate various tasks behind the scenes, improving your site’s functionality and user engagement.
Filters: Modifying Data Before It’s Output
While Actions allow you to add functionality, Filters allow you to modify data as it flows through WordPress. Filters are perfect for tweaking content, adjusting post titles, or altering data before it’s saved to the database or displayed on the front end.

Example: Adding a Prefix to Post Titles
Imagine you want every post title on your site to be prefixed with “Breaking News: “. Instead of manually editing each post title, you can use a filter to prepend this text automatically.
function prefix_post_title($title) {
if (is_single()) {
$title = 'Breaking News: ' . $title;
}
return $title;
}
add_filter('the_title', 'prefix_post_title');
Here, the the_title filter allows you to modify post titles before they are displayed. This ensures the prefix will appear consistently across your site without modifying each post individually.
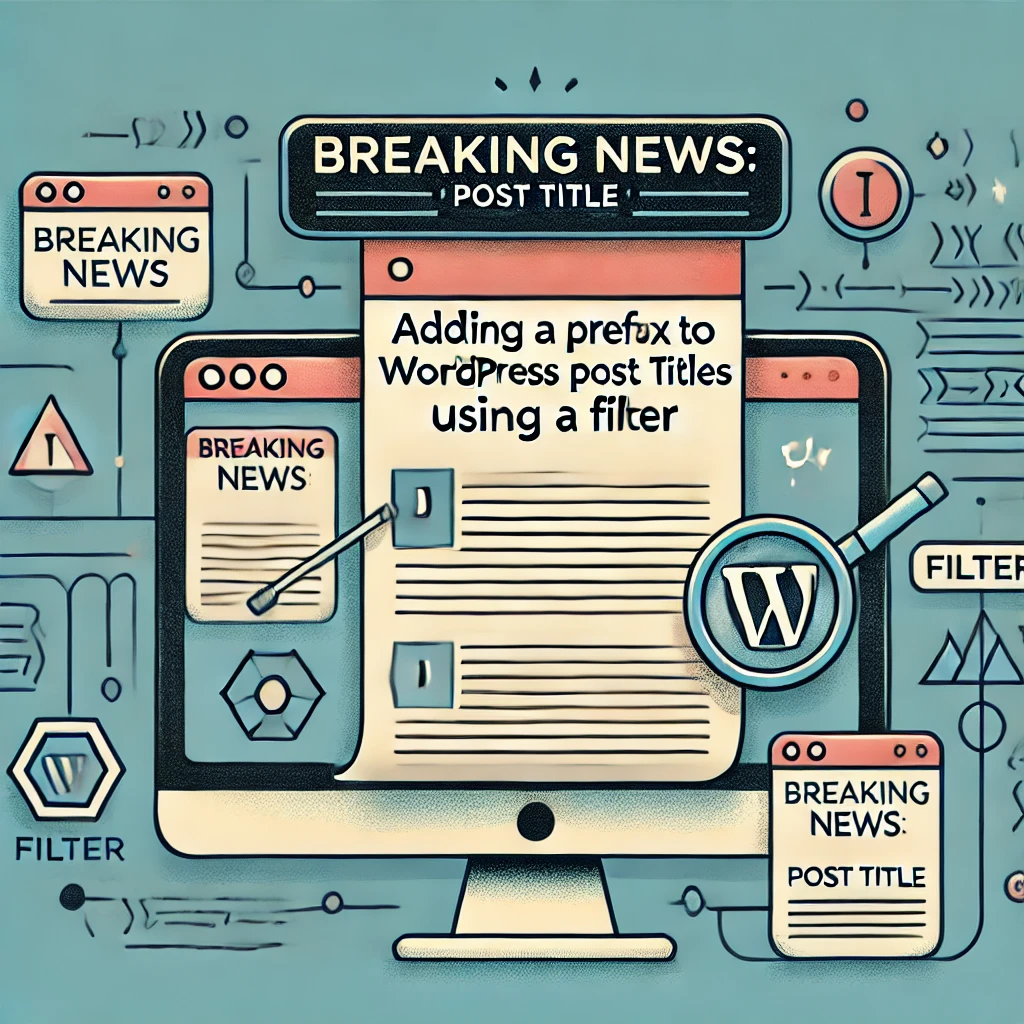
Example: Customising the Excerpt Length
WordPress allows you to display a post excerpt as a summary, but the default length might not suit your needs. Using the excerpt_length filter, you can easily adjust this to your preferred length.
function custom_excerpt_length($length) {
return 20; // Set the excerpt length to 20 words
}
add_filter('excerpt_length', 'custom_excerpt_length');
This filter ensures that your post excerpts are concise and consistent throughout the site.
Why Hooks Are Better Than Direct File Modifications
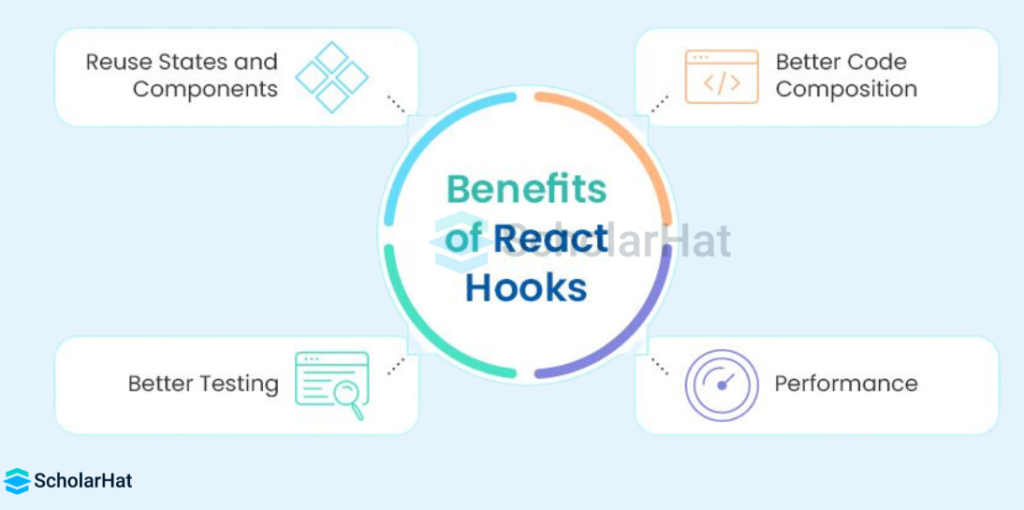
While it might be tempting to modify your theme’s core files to achieve custom functionality, this practice is highly discouraged. Directly altering core files can lead to a host of issues, including:
- Loss of customisations during updates: When you update WordPress, themes, or plugins, any changes made directly to core files will be overwritten.
- Compatibility problems: Modifying core files can introduce conflicts with plugins, themes, or future updates, leading to bugs or functionality breakage.
- Maintenance challenges: Custom code added to core files becomes difficult to manage and maintain over time.
By using hooks (both Actions and Filters), you can cleanly insert custom code into WordPress in a way that is sustainable and easy to manage. Hooks allow you to keep your customisations separate from the core code, ensuring your website remains flexible and future-proof.
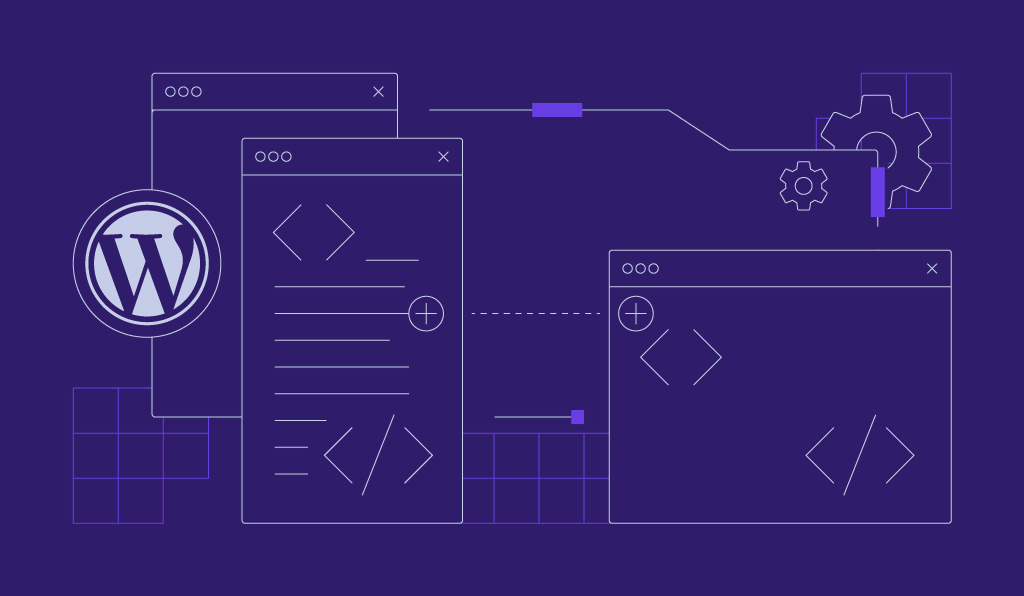
Best Practices for Using Hooks
- Use Unique Function Names
To avoid conflicts with other themes or plugins, always use a unique prefix for your function names. For instance, instead of naming a functionmodify_content
, use something like
function mytheme_modify_content($content) {
// Custom functionality goes here
}
2. Understand Hook Priorities
WordPress hooks allow you to specify the priority of when your function should be executed. The default priority is 10, but you can change this to ensure your function runs at the appropriate time.
add_action('wp_head', 'my_custom_function', 15);
A higher number means the function will run later, whereas a lower number will run earlier.
Choose the Right Hook
WordPress provides a wide range of hooks tied to specific life points. Choosing the right hook for your customizations is crucial. For instance, use the wp_enqueue_scripts action to load stylesheets and JavaScript, rather than init, to ensure that your assets are loaded at the correct stage of the page loading process.
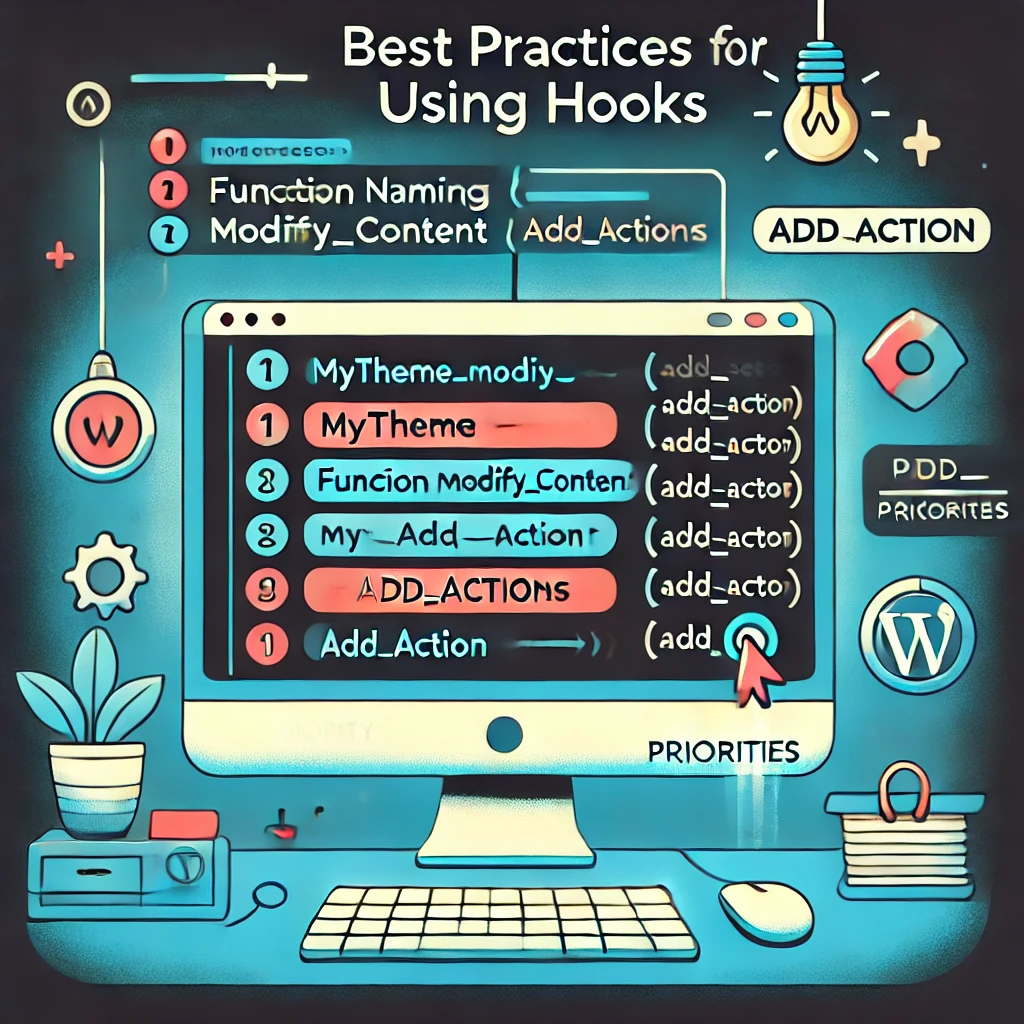
Wrapping Up
The WordPress hook system—comprising Actions and Filters—provides a robust and flexible way to extend your site’s functionality without touching the core files. Whether you’re adding a custom feature, modifying output, or altering content, hooks make it possible to maintain a clean, organised, and future-proof website.
By using hooks, you ensure that your site is more adaptable and that your customisations are less prone to issues during updates. When it comes to enhancing WordPress, Actions and Filters are your best friends. They provide the ultimate level of control over your site while keeping your code maintainable, secure, and upgrade-proof.
Responses