Die 5 wichtigsten Anwendungsfälle für REST API in der modernen Webentwicklung
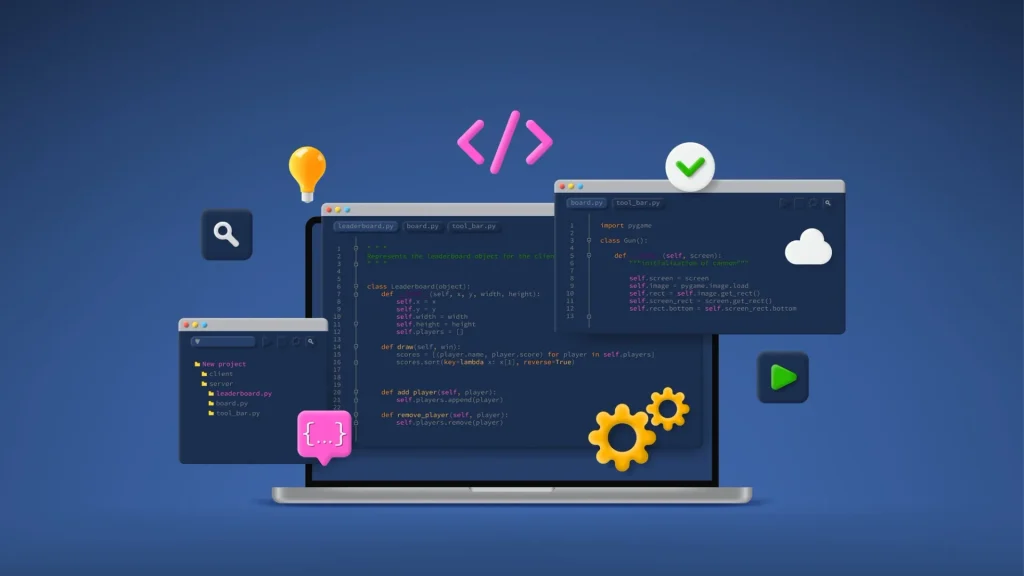
In der modernen Webentwicklung spielen REST-APIs (Representational State Transfer Application Programming Interfaces) eine entscheidende Rolle bei der Kommunikation und dem Datenaustausch von Anwendungen. REST-APIs ermöglichen die nahtlose Interaktion von Webdiensten und bieten die Flexibilität und Skalierbarkeit, die für die Entwicklung reichhaltiger, interaktiver Benutzererlebnisse erforderlich sind. Dieser Artikel richtet sich an Entwickler aller Qualifikationsstufen - egal, ob Sie gerade erst anfangen oder bereits Erfahrung mit REST-APIs haben. Wir gehen auf die fünf wichtigsten Anwendungsfälle für REST-APIs ein und liefern Beispiele, technische Erklärungen und praktische Szenarien, damit Sie deren Bedeutung für die Entwicklung robuster Anwendungen verstehen.
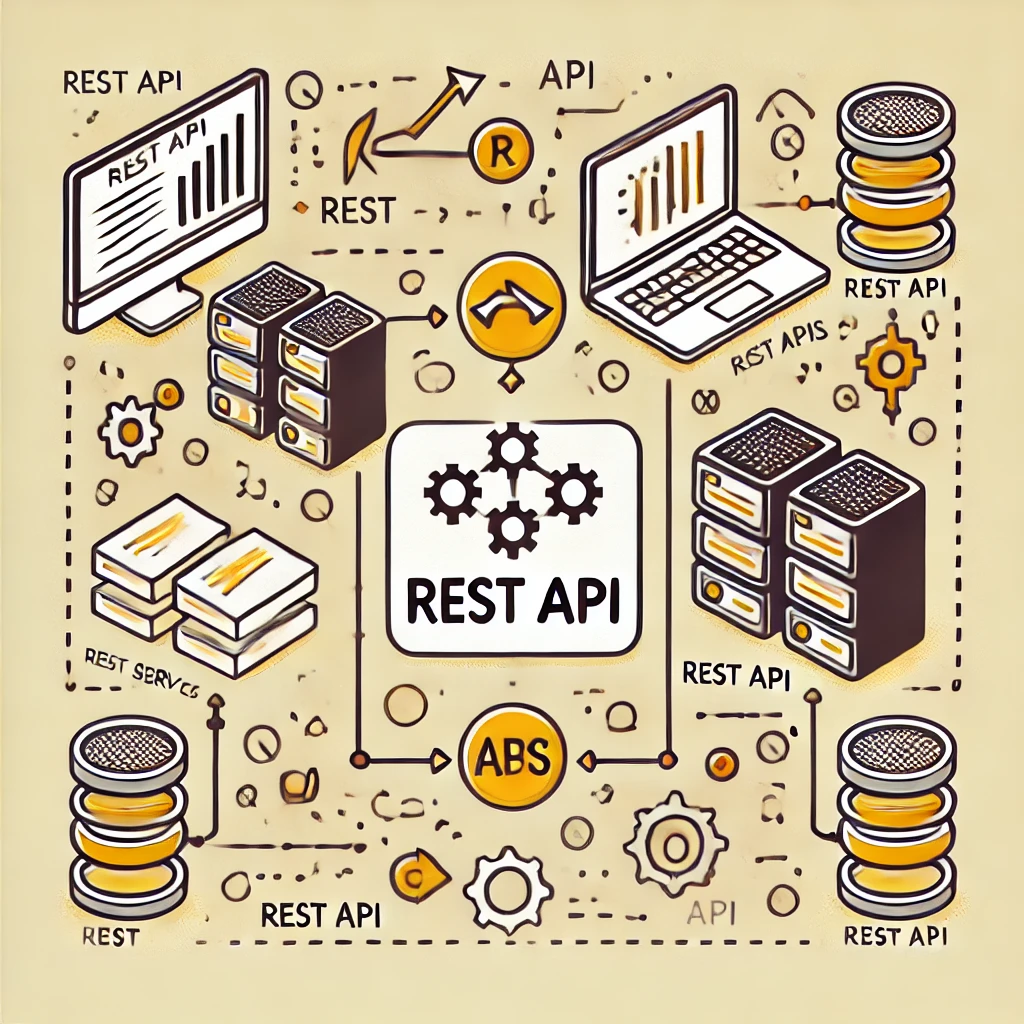
1. Datenabruf und Integration
Einer der häufigsten Anwendungsfälle für REST-APIs ist der Abruf und die Integration von Daten. REST-APIs bieten eine standardisierte Möglichkeit für Anwendungen, Daten von einem Server anzufordern und zu empfangen. Eine Webanwendung könnte beispielsweise eine REST-API verwenden, um Daten aus einer Datenbank abzurufen und sie den Benutzern in Echtzeit anzuzeigen. Dies ist besonders nützlich für Nachrichten-Websites, Social Media-Plattformen und Wetter-Apps.
Nehmen wir zur Veranschaulichung eine Wetter-App, die eine REST-API zum Abrufen von Wetterdaten von einem Server verwendet. Hier ist ein einfaches Beispiel dafür, wie eine GET-Anfrage aussehen könnte:
Importanträge
response = requests.get('https://api.weather.com/v3/weather/conditions?city=London')
if response.status_code == 200:
wetter_daten = response.json()
print(wetter_daten)
sonst:
print("Fehler beim Abrufen der Daten")
Erläuterung: In diesem Beispiel sendet die Anfragebibliothek eine HTTP GET-Anfrage an die Wetter-API. Die JSON-Daten werden geparst und gedruckt, wenn der Antwort-Statuscode 200 ist (was auf Erfolg hindeutet). Andernfalls wird eine Fehlermeldung angezeigt.
REST-APIs machen die Integration von Daten aus verschiedenen Quellen in eine einzige Anwendung einfach und ermöglichen es Entwicklern, ein einheitliches Benutzererlebnis zu schaffen. Eine Website für Reisebuchungen kann beispielsweise mehrere APIs verwenden, um Daten von Fluggesellschaften, Hotels und Autovermietungen abzurufen und den Benutzern alle verfügbaren Optionen an einem Ort zu präsentieren.
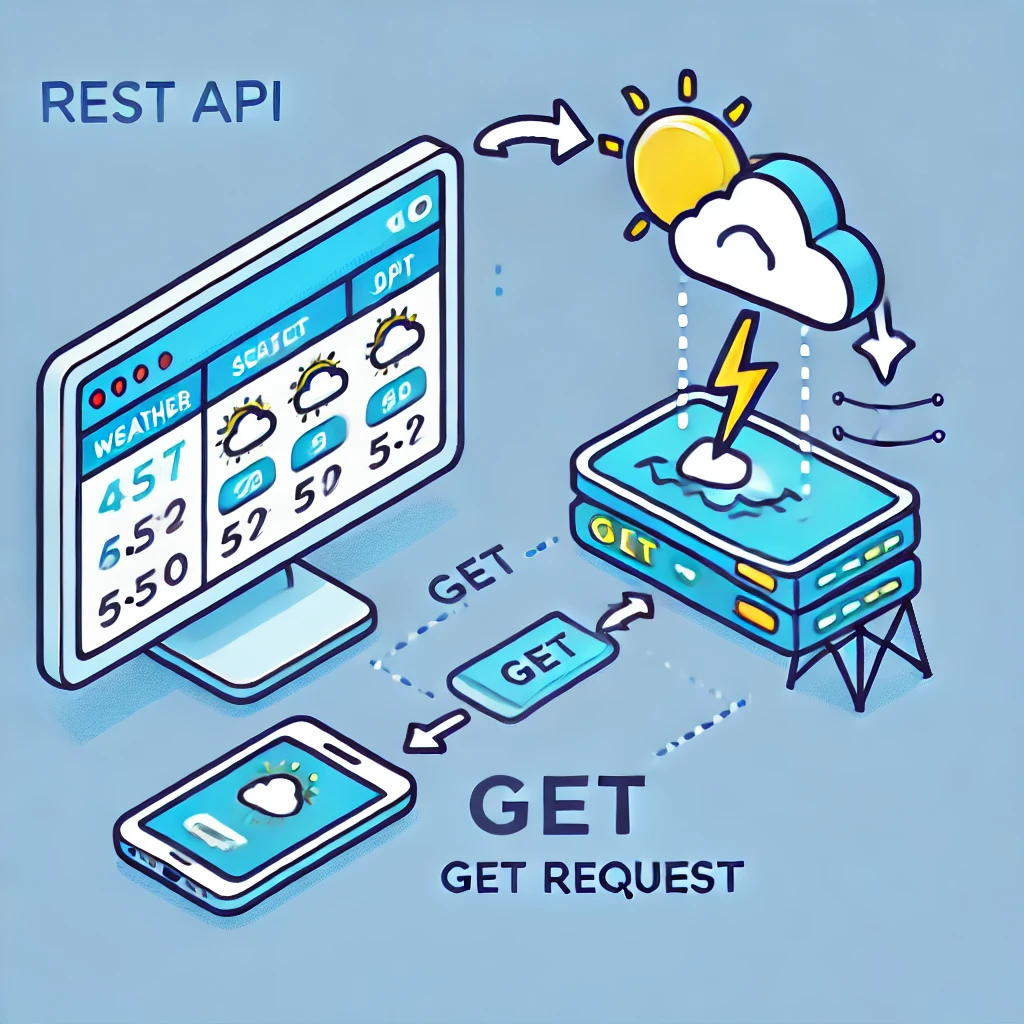
2. Benutzerauthentifizierung und Autorisierung
REST-APIs werden häufig für die Authentifizierung und Autorisierung von Benutzern verwendet. Bei vielen Webanwendungen müssen sich Benutzer mit herkömmlichen Anmeldeinformationen oder mit Diensten von Drittanbietern wie Google, Facebook oder GitHub anmelden. REST-APIs erleichtern die Implementierung von Authentifizierungssystemen wie OAuth 2.0 und ermöglichen eine sichere Benutzerauthentifizierung.
Mit OAuth 2.0 können sich Nutzer beispielsweise mit ihren Google-Anmeldedaten bei Ihrer Webanwendung anmelden, ohne ein neues Konto erstellen zu müssen. Hier finden Sie einen Überblick darüber, wie OAuth 2.0 funktioniert:
- Der Benutzer klickt auf die Schaltfläche "Mit Google anmelden".
- Die Anwendung sendet eine Anfrage an den Autorisierungsserver von Google.
- Wenn der Nutzer seine Zustimmung erteilt, sendet Google einen Autorisierungscode an die Anwendung.
- Die Anwendung tauscht den Code gegen ein Zugriffstoken aus, das dann für den Zugriff auf die Benutzerdaten verwendet werden kann.
Detailliertes Szenario: Angenommen, Sie möchten Nutzern die Möglichkeit geben, sich mit ihren Google-Konten anzumelden. Sie müssten Ihre Anwendung bei Google registrieren, die Anmeldedaten des Kunden einholen und den OAuth-Datenfluss verarbeiten, um ein sicheres Zugriffstoken zu erhalten. Dieses Zugriffs-Token wird dann zur Authentifizierung des Benutzers bei nachfolgenden Anfragen verwendet.
Autorisierung ist ein weiterer wichtiger Aspekt moderner Webanwendungen. REST-APIs verwalten Zugriffsberechtigungen und stellen sicher, dass nur autorisierte Benutzer auf bestimmte Teile einer Anwendung zugreifen können. Ein Online-Shop könnte beispielsweise eine REST-API verwenden, um zu überprüfen, ob ein Benutzer über administrative Rechte verfügt, bevor er Änderungen an den Produktlisten zulässt.
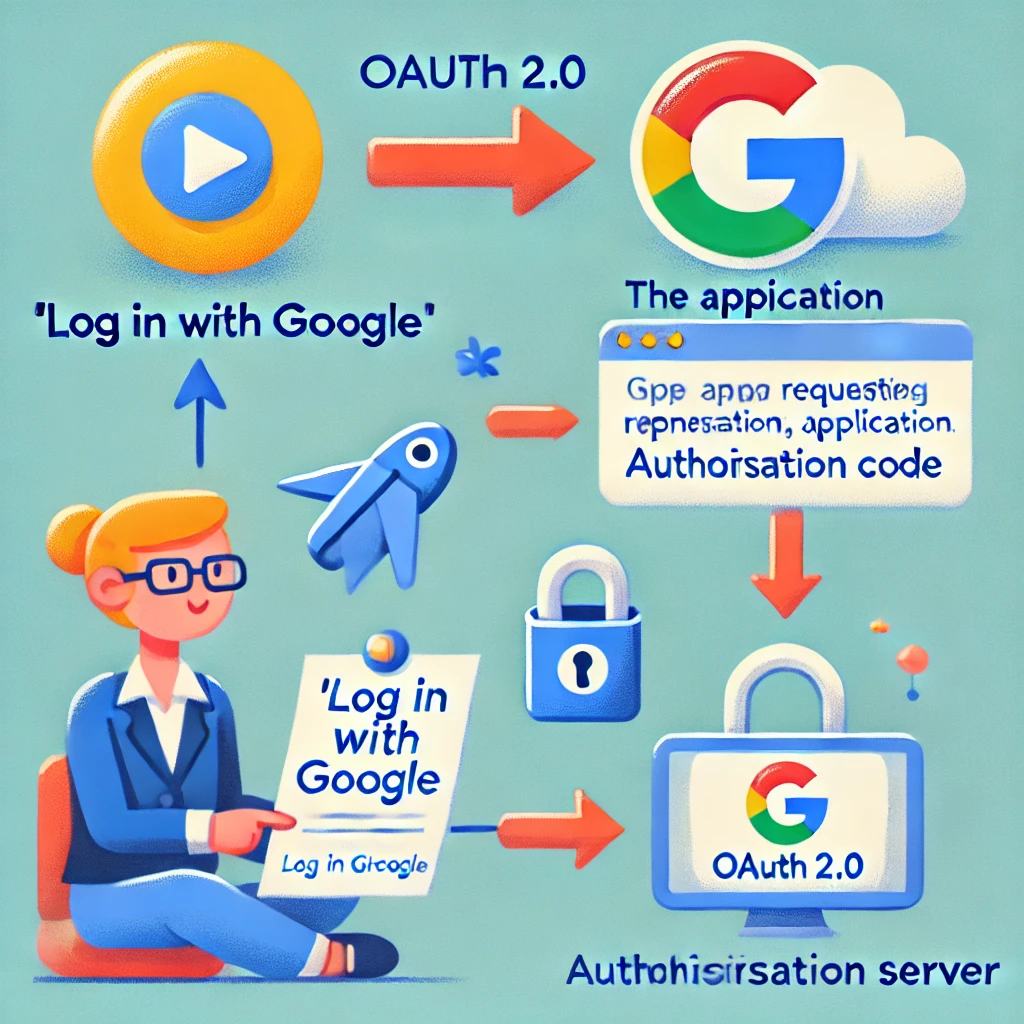
3. CRUD-Operationen
Operationen zum Erstellen, Lesen, Aktualisieren und Löschen (CRUD) sind für die meisten Webanwendungen von grundlegender Bedeutung, und REST-APIs bieten eine standardisierte Möglichkeit, diese Operationen auf einem Server durchzuführen. CRUD-Funktionen sind für Anwendungen mit nutzergenerierten Inhalten wie Blogs, Foren und E-Commerce-Seiten von entscheidender Bedeutung.
Eine Blog-Plattform könnte beispielsweise eine REST-API verwenden, damit Benutzer neue Beiträge erstellen, Inhalte aktualisieren, Beiträge löschen oder Artikel für die Homepage abrufen können. Hier ist ein Beispiel für eine CRUD-Operation mit REST:
Importanträge
# Einen neuen Blog-Eintrag erstellen
new_post = {
"title": "Mein erster Blogbeitrag",
"content": "Dies ist der Inhalt meines ersten Blogbeitrags."
}
response = requests.post('https://api.blogplatform.com/posts', json=new_post)
if response.status_code == 201:
print("Beitrag erfolgreich erstellt!")
sonst:
print("Fehler beim Erstellen des Beitrags")
Erläuterung: In diesem Beispiel wird durch eine HTTP POST-Anfrage ein neuer Blogbeitrag erstellt. Die Beitragsdaten werden im JSON-Format übergeben, und die API antwortet mit einem Statuscode, der angibt, ob der Vorgang erfolgreich war.
Indem Sie die RESTful-Prinzipien befolgen, können Entwickler CRUD-Operationen intuitiv und benutzerfreundlich gestalten, wobei jedes HTTP-Verb (POST, GET, PUT, DELETE) eine bestimmte Aktion darstellt.
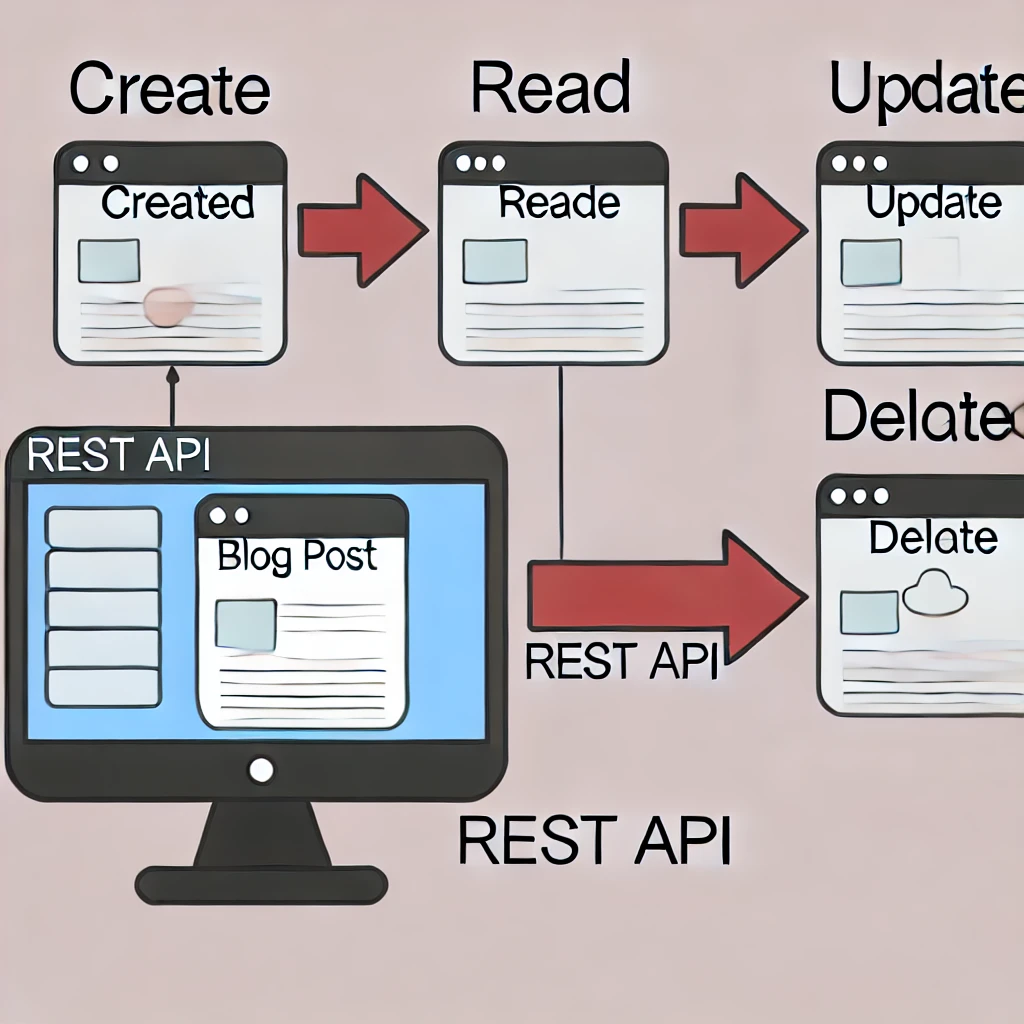
4. Integration von Drittanbieterdiensten
REST-APIs werden häufig verwendet, um Dienste von Drittanbietern in Webanwendungen zu integrieren. Die moderne Webentwicklung stützt sich häufig auf mehrere externe Dienste, um das Benutzererlebnis zu verbessern. Beispiele hierfür sind Zahlungsgateways (wie Stripe oder PayPal), die gemeinsame Nutzung sozialer Medien, Kartendienste (wie Google Maps) und Analyseplattformen.
Eine App für die Auslieferung von Lebensmitteln könnte beispielsweise eine REST-API verwenden, um Google Maps für die Standortverfolgung in Echtzeit einzubinden oder um Lieferrouten anzuzeigen und so die App benutzerfreundlicher zu gestalten. Hier ist ein vereinfachtes Beispiel für die Verwendung einer Drittanbieter-API zum Abrufen von Kartendaten:
Importanträge
response = requests.get('https://maps.googleapis.com/maps/api/directions/json?origin=NYC&destination=Boston&key=YOUR_API_KEY')
if response.status_code == 200:
map_data = response.json()
print(map_data)
sonst:
print("Fehler beim Abrufen von Kartendaten")
Erläuterung: Die Anfragen
Bibliothek sendet eine GET-Anfrage an die API von Google Maps und gibt den Start- und Zielort sowie den API-Schlüssel an. Die Antwort enthält die Routeninformationen, die zur Anzeige von Wegbeschreibungen oder zur Berechnung von Fahrzeiten verwendet werden können.
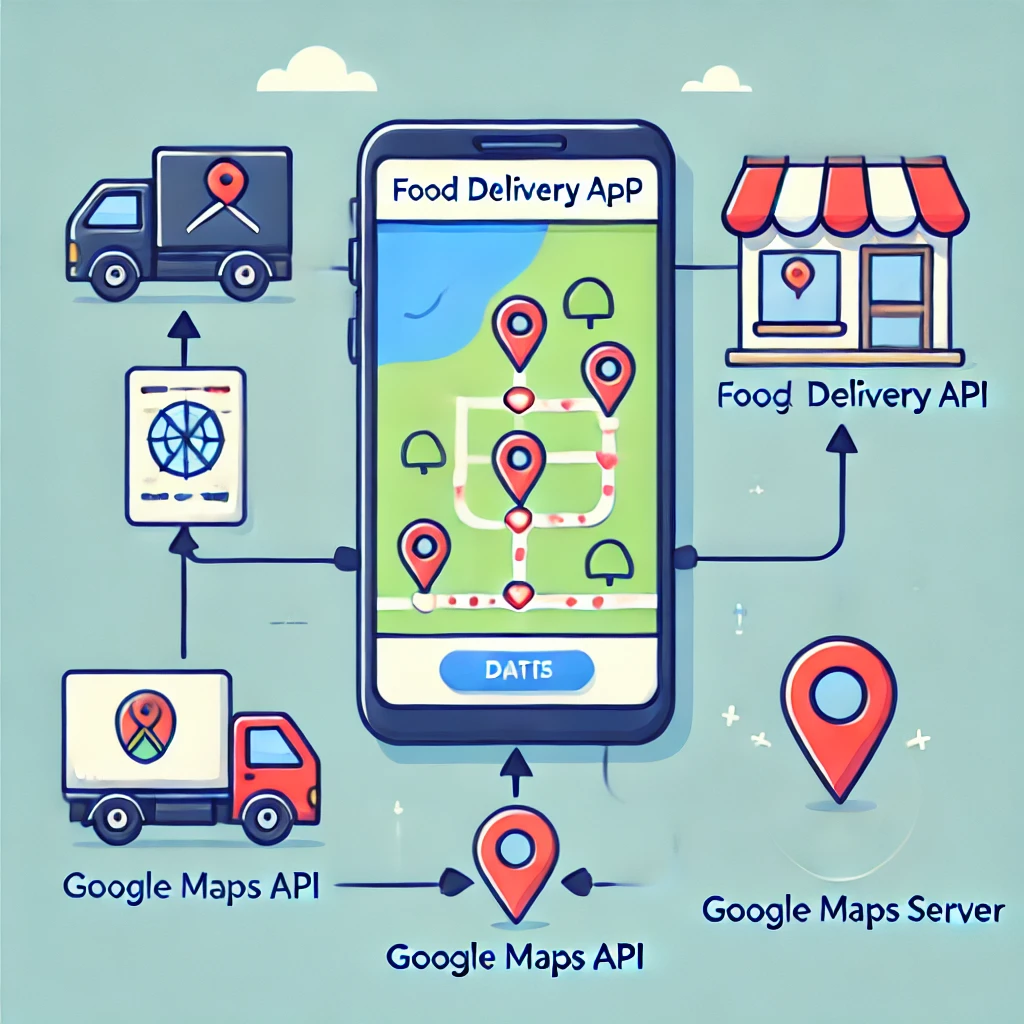
5. Microservices Architektur
Die Microservices-Architektur ist ein beliebter Ansatz zur Erstellung skalierbarer und wartbarer Webanwendungen, wobei REST-APIs eine Schlüsselkomponente dieser Architektur sind. In einem auf Microservices basierenden System wird eine Anwendung in kleinere, unabhängige Dienste unterteilt, die über REST-APIs miteinander kommunizieren.
Jeder Microservice ist für eine bestimmte Funktionalität zuständig, z. B. für die Benutzerverwaltung, Zahlungen oder Benachrichtigungen. REST-APIs ermöglichen eine effiziente Interaktion zwischen diesen Diensten und erleichtern so die unabhängige Entwicklung, Skalierung und Pflege verschiedener Anwendungsteile.
Eine E-Commerce-Plattform könnte zum Beispiel einen Microservice für Produktkataloge, einen anderen für die Bearbeitung von Bestellungen und einen weiteren für die Verwaltung von Kundenkonten verwenden, die alle über REST-APIs kommunizieren. Das folgende Diagramm veranschaulicht, wie Microservices über REST-APIs interagieren:
Ausführliche Erläuterung: Jeder Microservice ist isoliert, d. h. er kann unabhängig entwickelt, bereitgestellt und skaliert werden. REST-APIs stellen die Kommunikationsschicht bereit, die ein nahtloses Zusammenspiel dieser Dienste ermöglicht und sicherstellt, dass die gesamte Anwendung als eine zusammenhängende Einheit funktioniert.
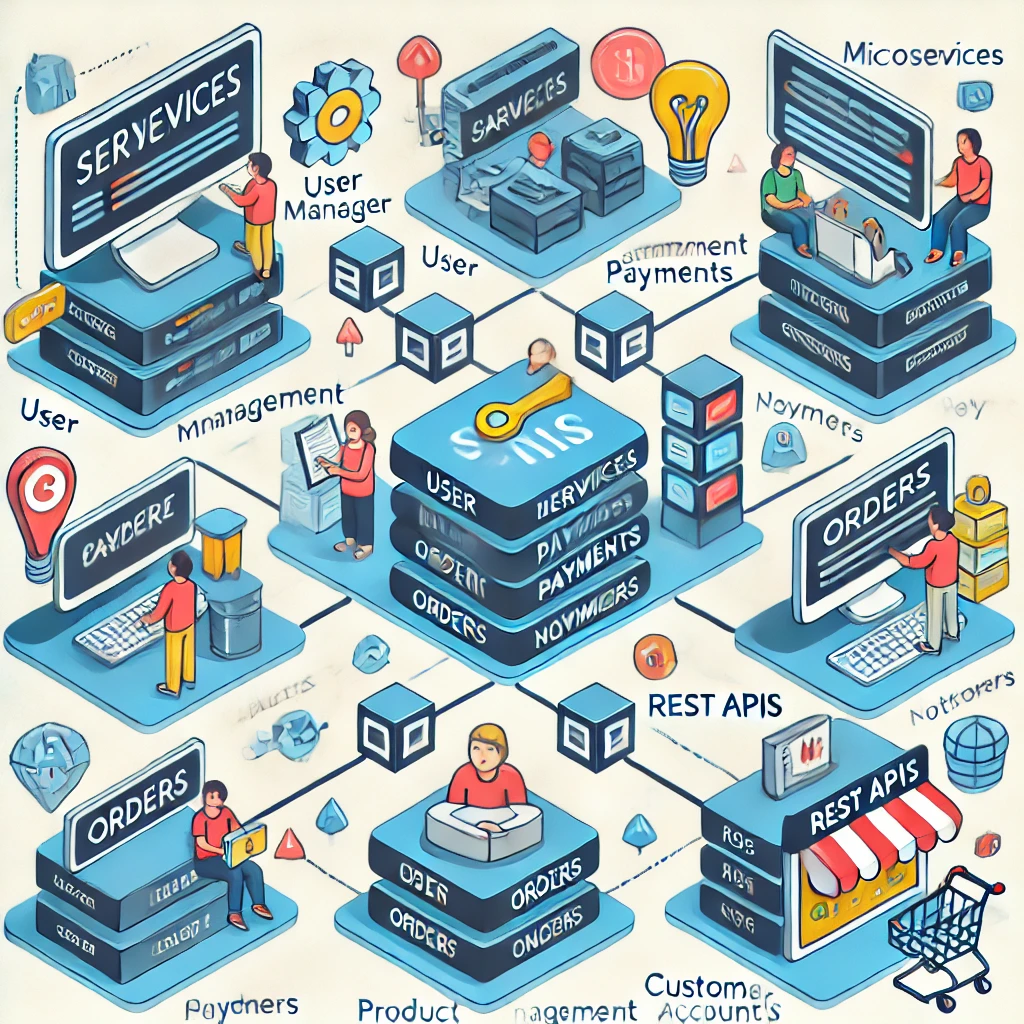
Vorteile und Herausforderungen
REST-APIs bieten zwar zahlreiche Vorteile, wie z.B. Skalierbarkeit, Flexibilität und einfache Integration, aber sie bringen auch Herausforderungen mit sich. Hier sind einige häufige Herausforderungen und Möglichkeiten, sie zu bewältigen:
- Sicherheitsbedenken: REST-APIs können anfällig für Angriffe sein, wie z.B. Man-in-the-Middle-Angriffe, wenn sie nicht richtig gesichert sind. Lösung: Verwenden Sie HTTPS, um die Datenübertragung zu verschlüsseln, eine angemessene Authentifizierung (z.B. OAuth 2.0) zu implementieren und Eingaben zu validieren, um Injektionsangriffe zu verhindern.
- Raten-Grenzwerte: Viele APIs von Drittanbietern erzwingen Ratenbeschränkungen, d. h. sie begrenzen die Anzahl der Anfragen, die ein Client innerhalb eines bestimmten Zeitrahmens stellen kann. Lösung: Implementieren Sie eine Zwischenspeicherung, um die Anzahl der API-Aufrufe zu reduzieren, und entwerfen Sie Ihre Anwendung so, dass sie Fehler bei der Ratenbegrenzung anständig behandelt, indem sie es nach einer Verzögerung erneut versucht.
- Datenkonsistenz: Die Datenkonsistenz kann schwierig sein, wenn mehrere Dienste über REST-APIs kommunizieren, insbesondere in verteilten Systemen. Lösung: Verwenden Sie eventuelle Konsistenzmodelle, implementieren Sie Wiederholungsversuche für fehlgeschlagene Anfragen und sorgen Sie für eine angemessene Protokollierung, um Probleme bei der Datensynchronisierung zu überwachen.
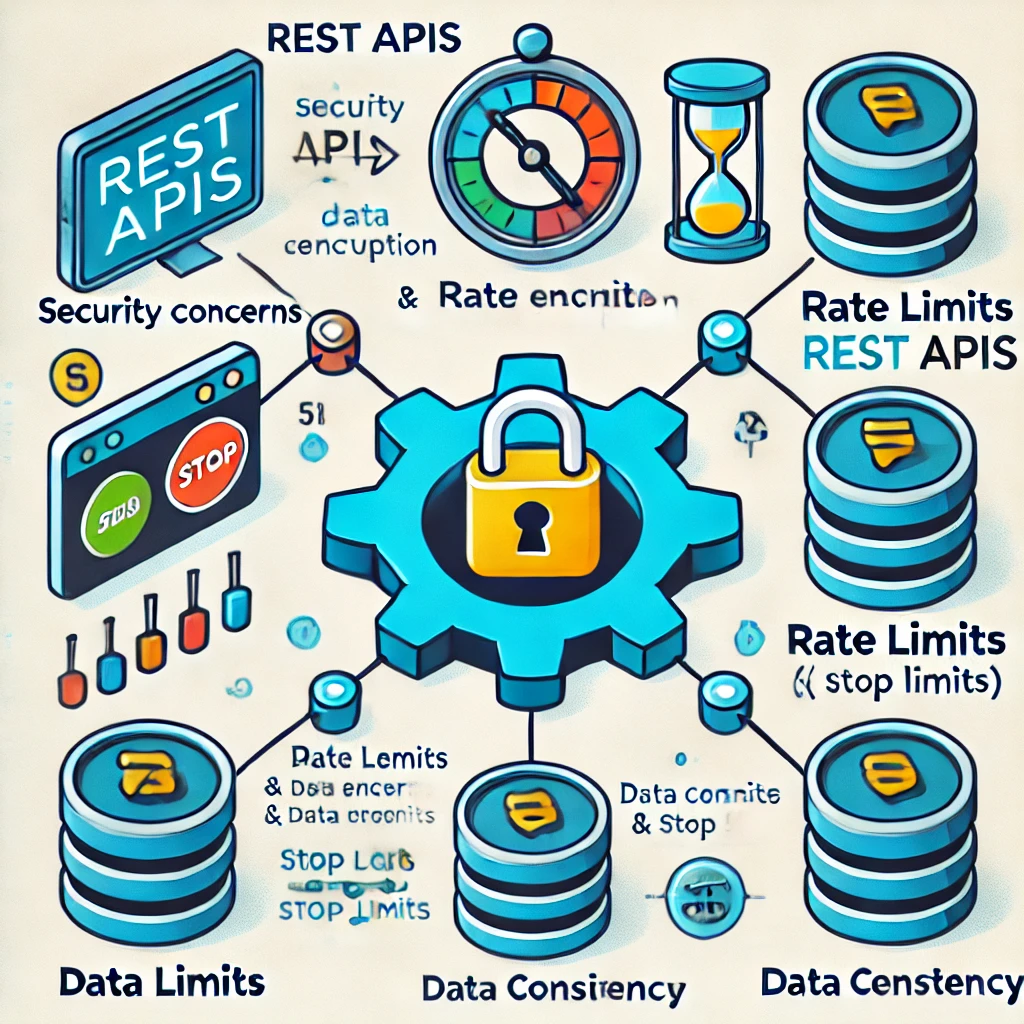
Fazit
REST-APIs sind zu einem unverzichtbaren Bestandteil der modernen Webentwicklung geworden. Sie ermöglichen die nahtlose Kommunikation zwischen Diensten und geben Entwicklern die Werkzeuge an die Hand, um skalierbare, sichere und funktionsreiche Anwendungen zu erstellen. Ob Sie nun Daten aus verschiedenen Quellen integrieren, die Benutzerauthentifizierung verwalten, CRUD-Operationen durchführen, eine Verbindung zu Diensten von Drittanbietern herstellen oder eine Microservices-Architektur implementieren - REST-APIs bieten die Flexibilität und Zuverlässigkeit, die Sie für die Entwicklung moderner Webanwendungen benötigen.
Um REST-APIs effektiv zu nutzen, versuchen Sie, eine einfache API eines Drittanbieters in Ihr nächstes Projekt zu integrieren oder eine auf Microservices basierende Anwendung zu entwickeln. Es gibt zahlreiche Ressourcen wie Online-Kurse, Dokumentationen und Community-Foren, die Ihnen helfen, Ihr Verständnis von REST-APIs zu vertiefen und Ihre Projekte auf die nächste Stufe zu heben.l.
Antworten