- Efficient Solutions for PHP High Concurrency in WordPress
- Practical Caching and PHP-FPM Techniques for High Traffic
- Asynchronous PHP Handling to Optimize WordPress Performance
If you’re managing a WordPress site and experiencing performance issues during high traffic, you might be facing PHP concurrency problems. This can be especially challenging if you’re running promotions, have viral content, or simply a growing user base. In this guide, we’ll break down the common causes of high concurrency issues, explain how to solve them using practical tools and server optimizations, and give tips on how to prevent these problems in the future.
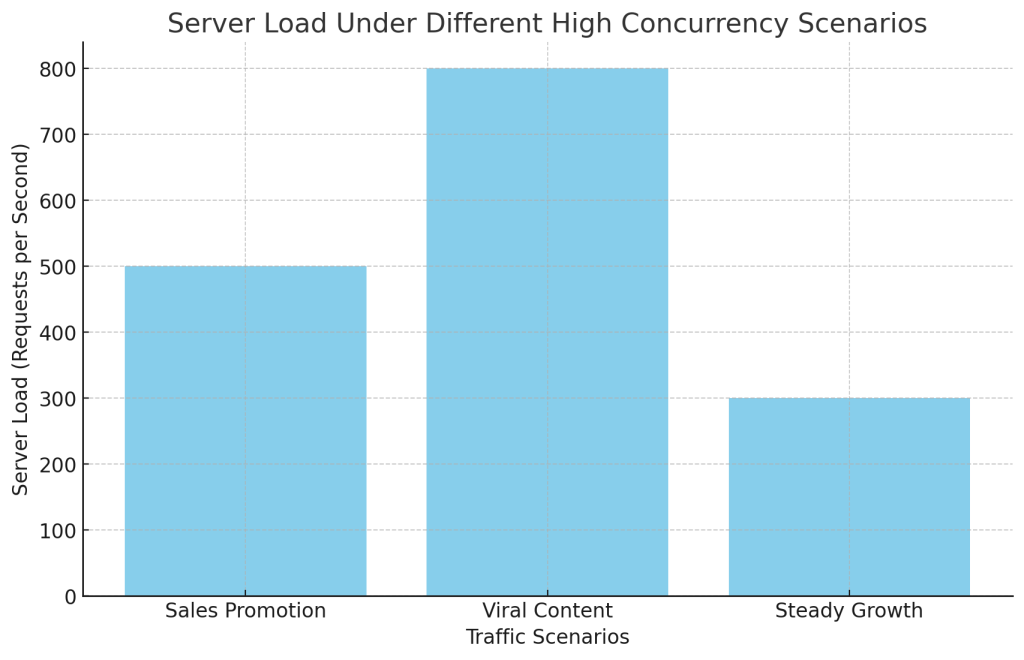
Understanding High Concurrency
High concurrency means multiple users are trying to access your website simultaneously, putting a lot of strain on your server. When this happens, the server may struggle to handle all the requests, leading to slow load times, server errors, or even downtime. This can occur due to:
- Sales and Promotions: Running a special event or discount can lead to a sudden surge in traffic.
- Viral Content: When a blog post or product goes viral, the number of visitors can increase dramatically.
- Steady Growth: Daily traffic naturally grows as your website becomes more popular, straining your server.
It would help if you optimized your server to manage multiple PHP scripts running concurrently and efficiently to handle these situations.
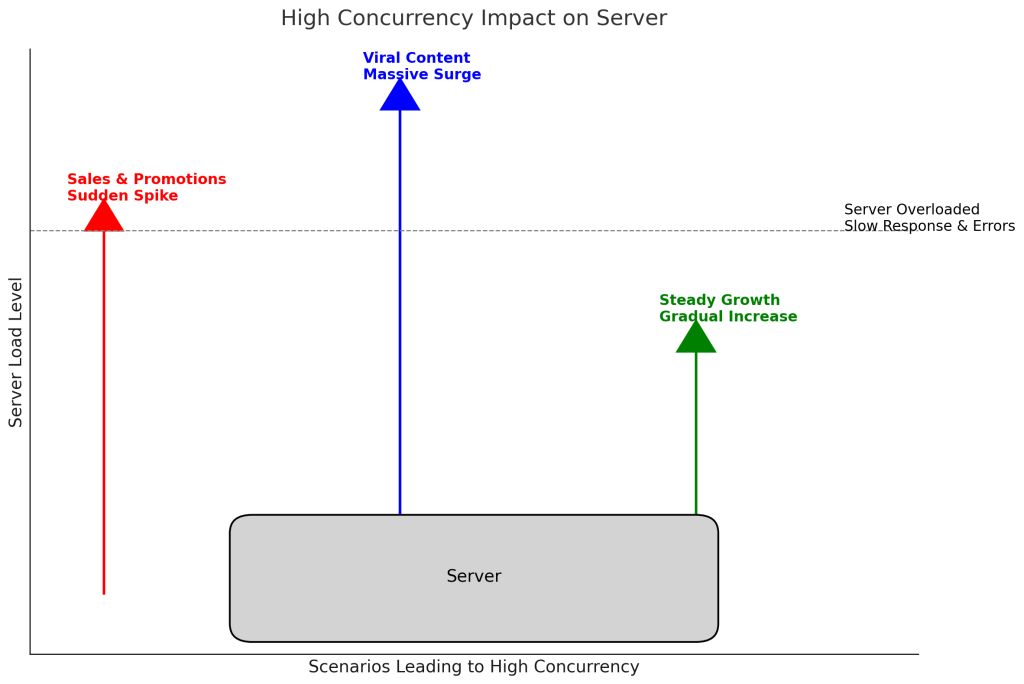
1. Leverage Caching for High Performance
Caching is a powerful way to handle high concurrency. It reduces server load, minimizes the need to process PHP scripts repeatedly, and delivers content faster.
- Data Caching with Redis or Memcached: By using caching systems like Redis oder Memcached, you can store frequently accessed data in memory, reducing the need to query the database constantly. This is particularly useful for WordPress because it reduces the load on MySQL, which is often a bottleneck.
- Setting Up Redis: Many hosting providers support Redis. You can enable it through your control panel (e.g., cPanel) or install it using command line commands if you have root access.
sudo apt-get install redis-server
sudo service redis-server start
- WordPress Integration: Use plugins like Redis Object Cache to connect your WordPress to Redis for object caching, which can help speed up your site during heavy traffic.
- Object Caching with WordPress Plugins: Object caching stores the results of database queries so that WordPress doesn’t need to fetch the same data repeatedly.
- Plugin Setup: Install and configure plugins like W3 Total Cache oder WP Rocket. Enable object caching in the plugin settings to reduce repeated queries and improve response times.
- Page Caching with WP Super Cache: Page caching is especially effective for reducing PHP processing. You serve a cached HTML copy instead of generating a page for every visitor.
- Step-by-Step: Install WP Super Cache, activate it, and configure page caching in its settings. You can also enable preloading to have pages cached in advance, reducing server load during high-traffic
Caching allows you to reduce server load and serve content faster, which is crucial during high concurrency scenarios.
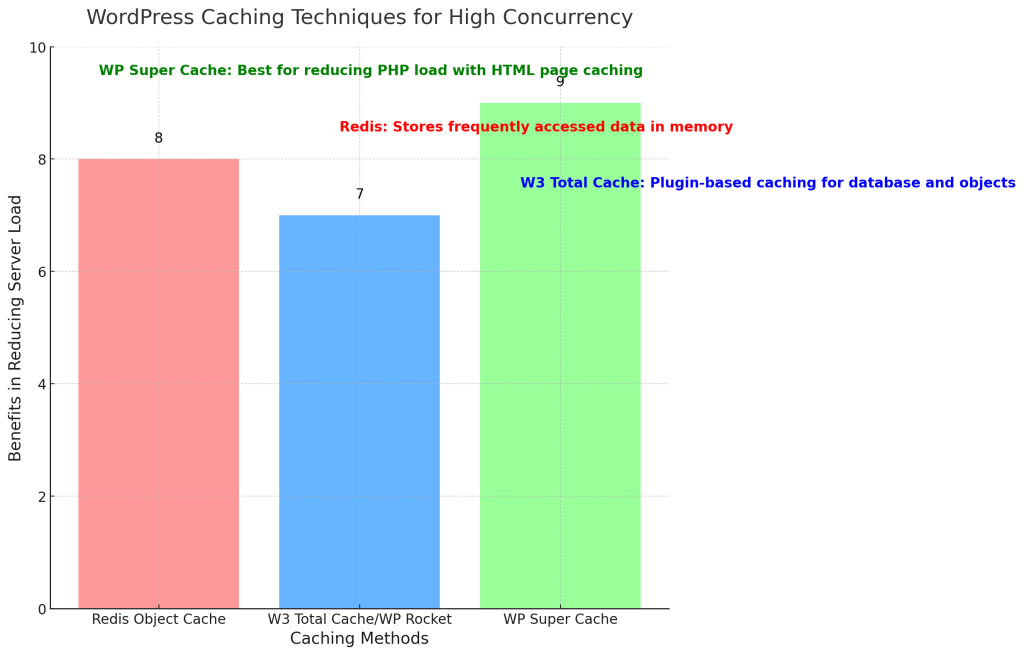
2. Optimize PHP Handling with PHP-FPM
PHP-FPM (FastCGI Process Manager) is an advanced method for managing PHP processes. It improves how your server handles multiple requests simultaneously.
uning pm.max_children: This setting in PHP-FPM defines how many child processes can handle PHP requests concurrently. The default might be too low for high traffic.
- Adjusting via cPanel: If you use cPanel, go to MultiPHP Manager > PHP-FPM Settings. Set the pm.max_children based on your server resources. Increasing this value allows more concurrent processes but requires more RAM, so adjust carefully.
Process Management Modes: PHP-FPM offers dynamic and on-demand process management modes.
- Dynamic: Keeps several processes ready to handle requests. Use this if you experience spikes in traffic.
- On-demand: Spawns new processes only when needed, saving memory during low-traffic periods. This can be useful if your site’s traffic fluctuates.
Increasing Memory Limits: To prevent processes from running out of memory, increase memory_limit
in your php.ini
file.
memory_limit = 512M
How to Increase via cPanel: Use the PHP INI Editor in cPanel to increase memory_limit
zu 512M
or higher, depending on your site’s requirements.
PHP-FPM allows your server to manage multiple requests efficiently, helping to avoid crashes during peak times.
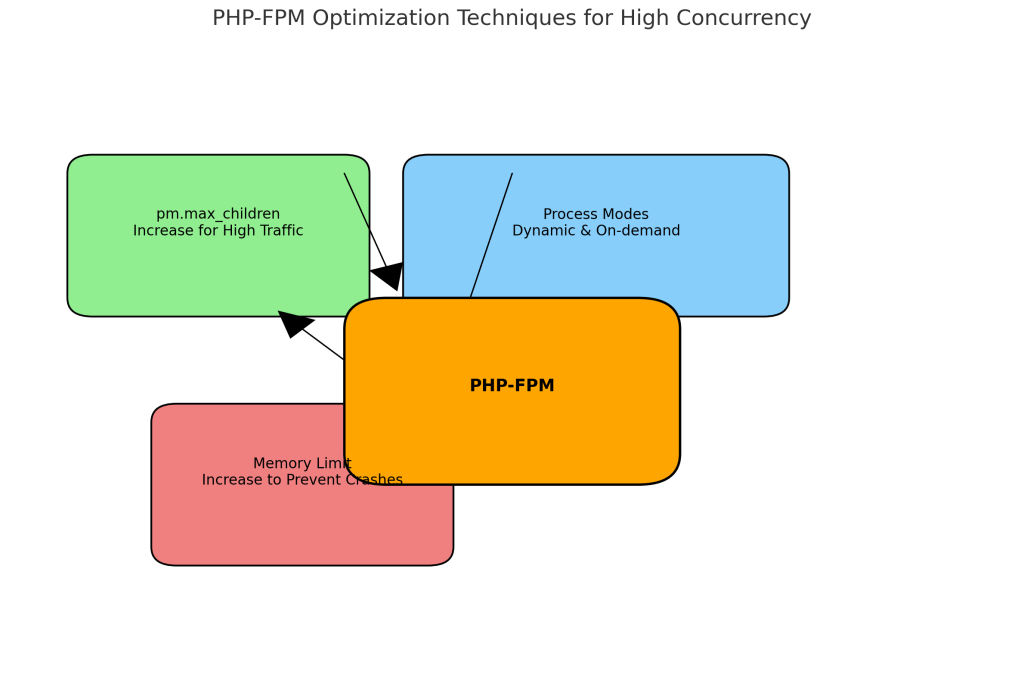
3. Asynchronous Processing for Heavy Tasks
Some operations, like sending emails or interacting with APIs, can take up a lot of server resources. If they’re processed synchronously, these tasks can slow down your site. Instead, asynchronous processing allows the server to handle these tasks in the background, improving overall responsiveness.
3.1 Using ReactPHP for Asynchronous Operations
ReactPHP is an open-source, event-driven, asynchronous programming framework for PHP. It enables you to build high-performance server applications and handle multiple tasks concurrently without blocking other operations.
How ReactPHP Works: ReactPHP uses an event loop that continuously listens for and processes incoming requests. This event-driven model allows PHP code to keep running while waiting to complete I/O tasks, such as database reads/writes or external API requests.
Practical Uses: ReactPHP is particularly useful for scenarios where non-blocking I/O is needed. For example, if your WordPress site fetches data from external sources (such as REST APIs), ReactPHP can handle these tasks in the background while continuing to serve other users.
Beispiel: You must pull data from an external API to update a product listing. Instead of blocking all other processes until the API call completes, ReactPHP allows you to continue handling other user requests, making your site faster and more responsive.
Implementation Example:
require 'vendor/autoload.php';
$loop = React\EventLoop\Factory::create();
$client = new React\Http\Client($loop);
$client->get('https://api.example.com/data')->then(function ($response) {
echo 'Data received: ' . $response->getBody();
});
$loop->run();
Explanation:
- Require ‘vendor/autoload.php’: This line loads all the necessary packages installed via Composer, including ReactPHP.
- $loop = React\EventLoop\Factory::create();: Creates the event loop that will keep the script running, waiting for I/O events.
- $client = new React\Http\Client($loop);: Creates an HTTP client using the event loop.
- $client->get(‘https://api.example.com/data’): Sends an HTTP GET request to the specified URL.
- ->then(function ($response) {…}): Handles the reaction when it arrives, allowing other operations to continue in the meantime.
- $loop->run();: Starts the event loop, processing all pending I/O operations.
ReactPHP is an excellent tool for building non-blocking PHP applications, enhancing your server’s ability to handle high concurrency.
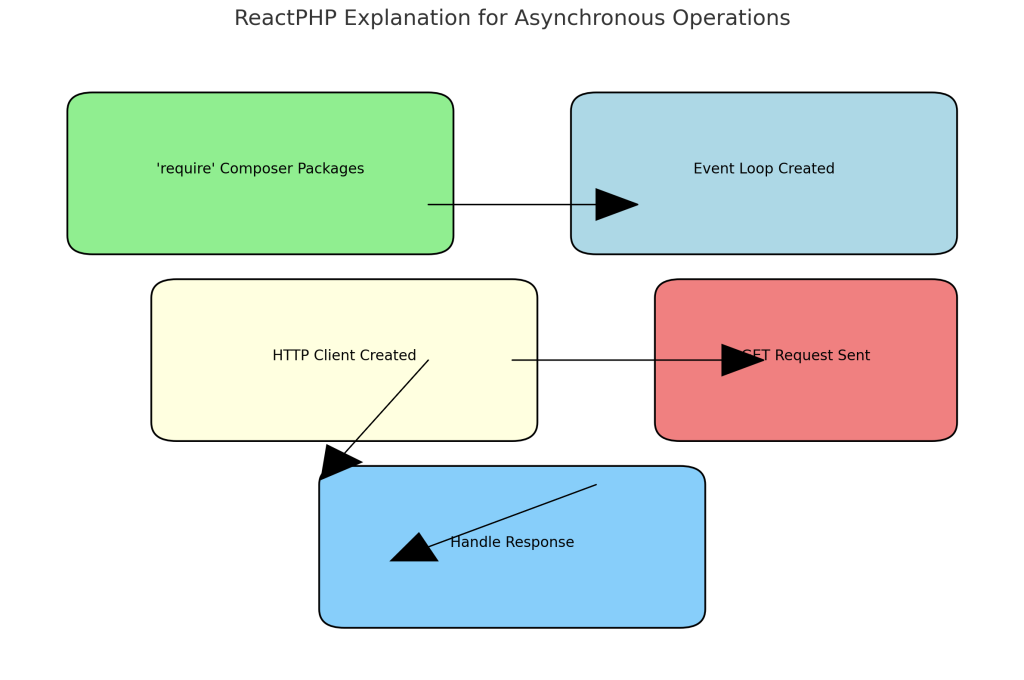
3.2 Using Swoole for High Performance
Swoole is a high-performance, coroutine-based PHP extension that brings asynchronous, parallel computing to PHP. It is especially well-suited for handling high concurrency, allowing PHP to act like an accurate asynchronous server.
Benefits of Coroutines: Swoole’s coroutines allow you to run multiple tasks simultaneously without blocking. For example, numerous database queries or network requests can be processed concurrently, reducing bottlenecks and improving performance.
Practical Uses of Swoole:
- WebSocket Server: Swoole makes it easy to build a WebSocket server, enabling real-time communication with users (e.g., for chat applications or live notifications).
- Task Scheduling: You can use Swoole to manage background tasks, such as sending bulk emails or processing image uploads, without impacting the responsiveness of your WordPress site.
- Beispiel: Imagine your WordPress site offers real-time chat support. Using Swoole, you can handle thousands of WebSocket connections concurrently, providing instant messaging without affecting the performance of the rest of your website.
Implementation Example:
<?php
$server = new Swoole\Http\Server("127.0.0.1", 9501);
$server->on("request", function ($request, $response) {
$response->header("Content-Type", "text/plain");
$response->end("Hello Swoole");
});
$server->start();
Explanation:
- $server = new Swoole\Http\Server(“127.0.0.1”, 9501);: Creates an HTTP server that listens on IP 127.0.0.1 and port 9501.
- $server->on(“request,” function ($request, $response) {…}): Defines the behavior when the server receives an HTTP request. The callback function processes the request and sends a response.
- $response->header(“Content-Type”, “text/plain”);: Sets the response header to indicate plain text content.
- $response->end(“Hello Swoole”);: Sends the response back to the client and ends the request.
- $server->start();: Starts the server, allowing it to accept incoming requests.
Swoole is powerful for building scalable applications and improving WordPress’s concurrency capabilities, especially in real-time and resource-intensive scenarios.
4. Server Optimization Tips for WordPress High Concurrency
- Upgrade Your Server Resources: If your site slows down during peak times, consider upgrading your server’s CPU and RAM. Moving from shared hosting to a VPS oder dedicated server can provide the resources you need to handle higher concurrency.
- When to Upgrade: If your website frequently runs into issues during high-traffic events, a VPS or dedicated server is an excellent investment to maintain speed and reliability.
- Use a Control Panel like cPanel: Tools like cPanel make server management easier for non-experts. You can tweak PHP settings, manage memory, and enable caching in one place.
- How to Use cPanel for Optimization: Go to MultiPHP Manager to adjust your PHP version and use the PHP INI Editor to modify memory limits or max execution times as required.
- MySQL Configuration: The database is a common bottleneck for high-concurrency websites. You can improve MySQL’s performance by adjusting settings like max_connections and query_cache_size.
- How to Configure via cPanel: Use phpMyAdmin in cPanel or access MySQL Configuration settings to increase max_connections and optimize your queries for better performance. Consider using MariaDB, which is often faster for WordPress.
- Enable OPcache: OPcache stores the compiled PHP scripts in memory, which means they don’t need to be compiled every time they are accessed. This can significantly reduce load times and server load.
- Enabling OPcache in cPanel: Navigate to PHP Extensions in cPanel and enable OPcache for improved PHP performance.
- Use a Content Delivery Network (CDN): Offload static content like images, JavaScript, and CSS using a CDN such as Cloudflare oder Amazon CloudFront. This reduces the load on your server and speeds up content delivery.
- How to Set Up Cloudflare: Register with Cloudflare, update your nameservers, and configure settings to cache and deliver static assets from their global network.
- Optimize Your .htaccess File: If you’re using Apache, optimizing your .htaccess file can help improve performance.
- Enable Gzip Compression and Browser Caching: Add directives to your .htaccess file to enable Gzip compression and leverage browser caching. This reduces the size of assets and ensures that returning visitors load the site faster.
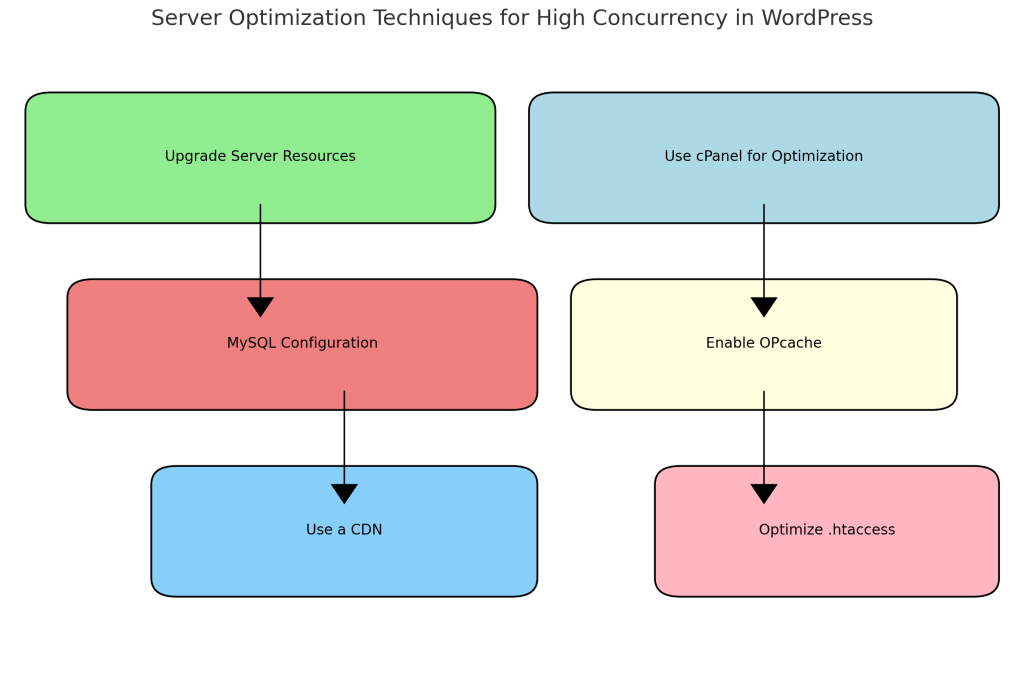
# Gzip Compression
<IfModule mod_deflate.c>
AddOutputFilterByType DEFLATE text/html text/plain text/xml text/css text/javascript application/javascript
</IfModule>
# Browser Caching
<IfModule mod_expires.c>
ExpiresActive On
ExpiresByType image/jpg "access plus 1 year"
ExpiresByType image/jpeg "access plus 1 year"
ExpiresByType image/gif "access plus 1 year"
ExpiresByType image/png "access plus 1 year"
ExpiresByType text/css "access plus 1 month"
ExpiresByType application/javascript "access plus 1 month"
</IfModule>
Preventing Future High Concurrency Issues
- Monitor Server Metrics: Use tools like New Relic oder UptimeRobot to monitor server performance. Monitor CPU, memory, and disk I/O usage and proactively address bottlenecks.
- Load Testing: Perform regular load tests using tools like Loader.io oder Apache JMeter to see how your site performs under heavy load. This helps you identify weak points before they become problematic during high-traffic events.
Fazit
Dealing with high concurrency issues in PHP is critical to ensuring your WordPress site runs smoothly during high-traffic times. By implementing caching, configuring PHP-FPM, using asynchronous processing tools like ReactPHP and Swoole, and optimizing your server settings, you can significantly improve your site’s ability to handle concurrent users.
Managing a high-traffic WordPress website can be challenging, but with the right tools and configurations, you can keep your site fast, reliable, and ready for growth. Take these steps to optimize your site now, and you’ll be prepared for whatever traffic comes your way.
Feel free to reach out if you have questions or need further assistance. With these proven methods, your WordPress site can thrive, even during peak traffic.
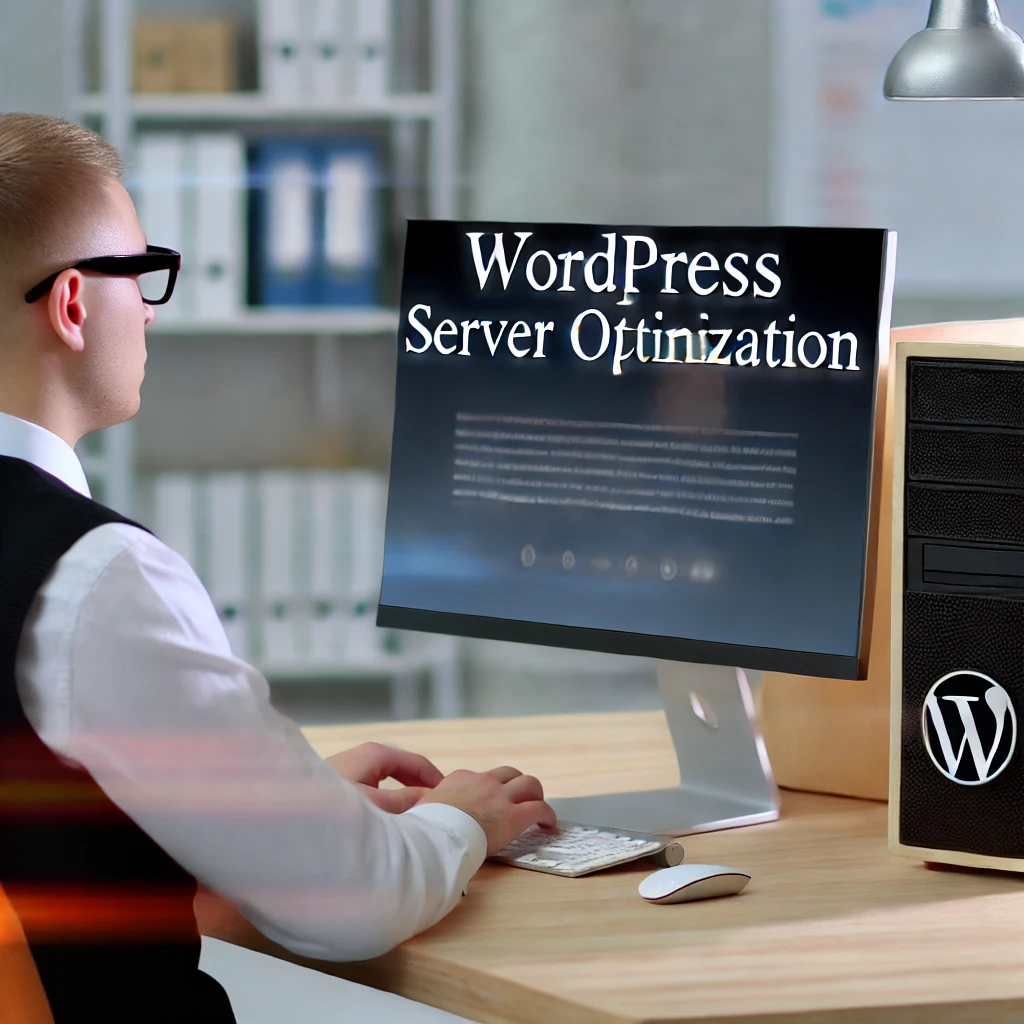