Boost Performance: Best Practices for Optimizing PHP Scripts
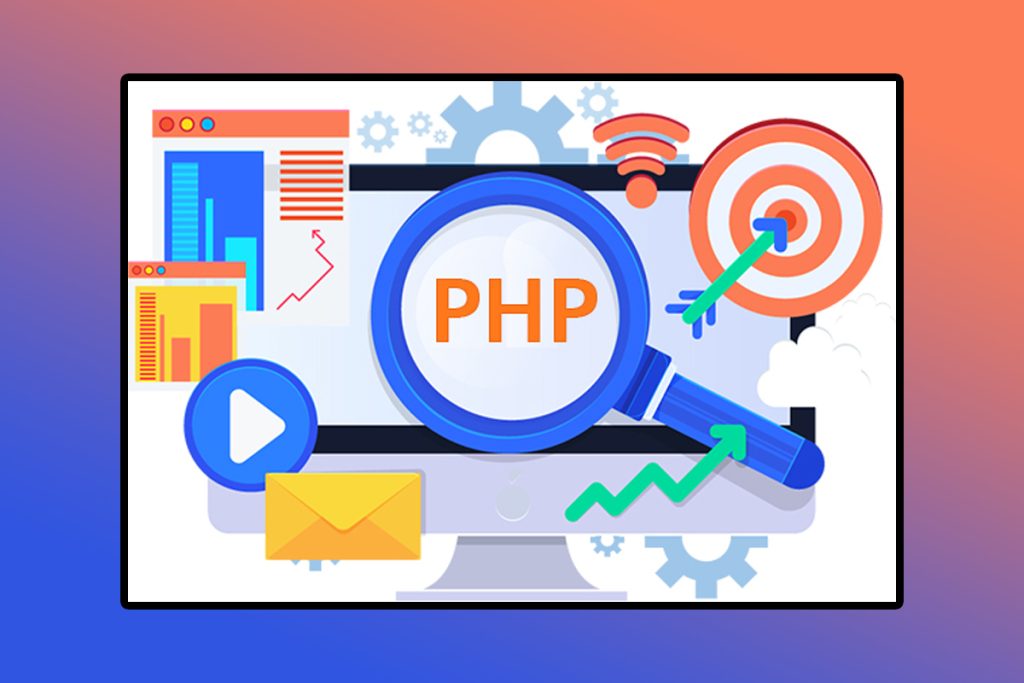
Optimizing your PHP scripts is crucial for building fast and efficient web applications. No one likes a slow website, and with user patience thinner than ever, boosting performance should be a top priority for any developer. Today, we’re exploring some tried-and-true methods to help you supercharge your PHP scripts and ensure your application runs smoothly and efficiently.
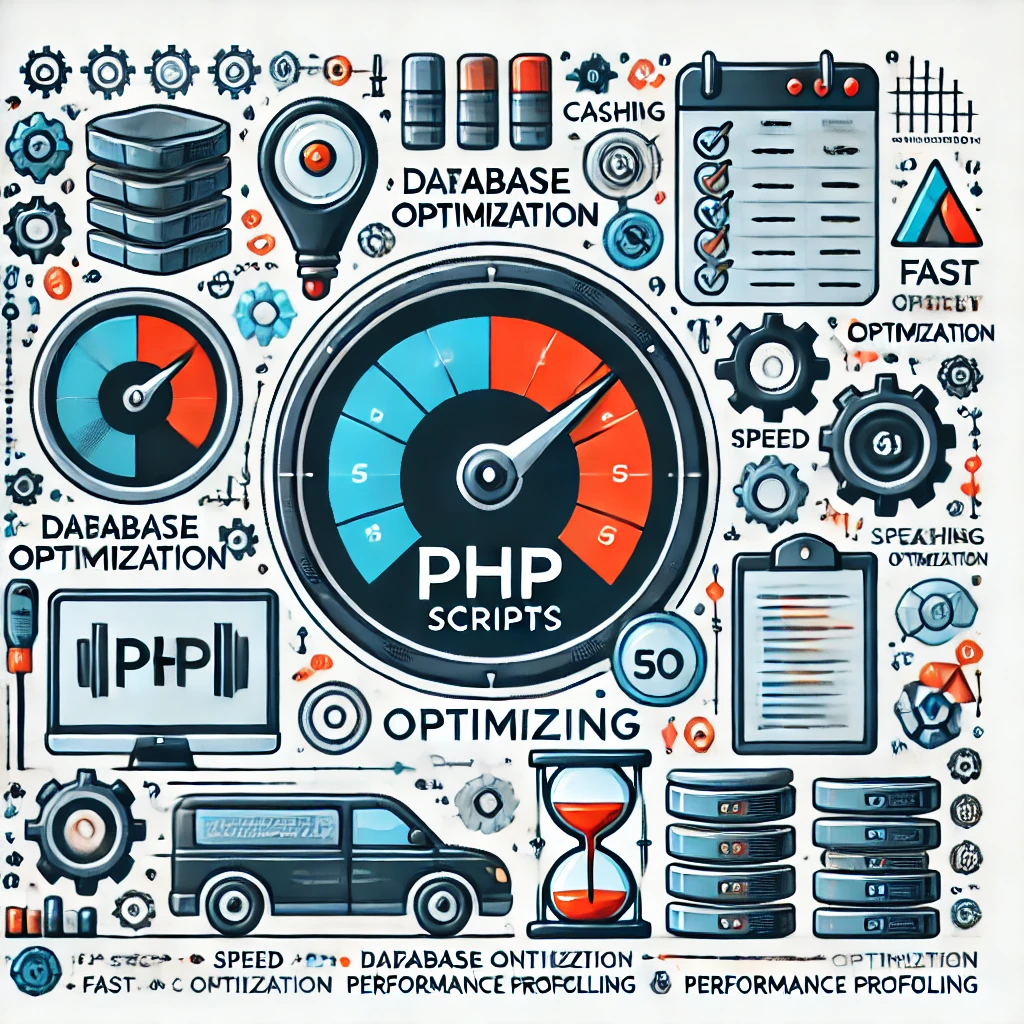
Target Audience: This article is aimed at beginner to intermediate PHP developers who are looking to improve the performance of their web applications by applying practical optimization techniques.
1.Cache It Like You Mean It
One of the most effective ways to improve PHP performance is through caching. The idea is simple: instead of executing the same script repeatedly, store the results and serve them up when needed. Tools like OPcache or even Memcached can drastically reduce execution time by saving compiled script bytecode, which means PHP doesn’t need to recompile your code every time it’s called. Implementing caching effectively can lead to significant performance gains, especially for content-heavy websites.
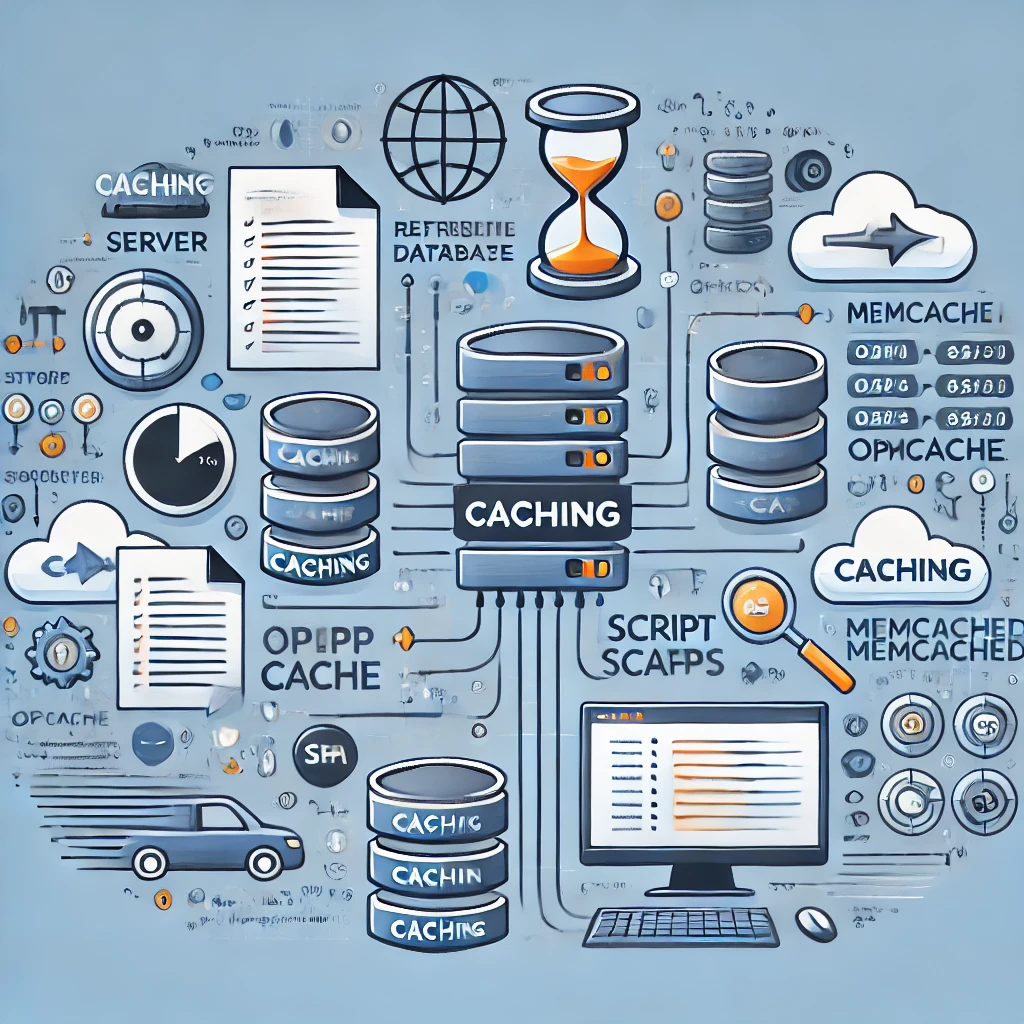
Real-World Scenario: Imagine running a high-traffic e-commerce site. Each user request might require multiple database calls to display product information, user details, and recommendations. Using OPcache to store compiled bytecode and caching database results with Memcached, you can drastically reduce response times, ensuring customers don’t abandon their carts due to slow page loads.
Beginner Example: Suppose you have a product listing page that fetches product details from the database each time a user visits. By caching these product details for a few minutes, you reduce the number of database hits, resulting in faster page load times and less server strain.
Step-by-Step Setup for OPcache:
- Ensure OPcache is installed:
sudo apt-get install php-opcache
- Enable OPcache in your
php.ini
file:
opcache.enable=1
opcache.memory_consumption=128
opcache.max_accelerated_files=10000
3.Restart your web server: sudo service apache2 restart
2.Optimize Database Queries
In most PHP applications, the database is the performance bottleneck. Ensure you’re not making unnecessary database calls—fetch only the data you need and leverage indexed fields to speed up search operations. Consider joining tables or using prepared statements instead of looping through multiple database calls. An optimized query is worth over a hundred PHP code lines.
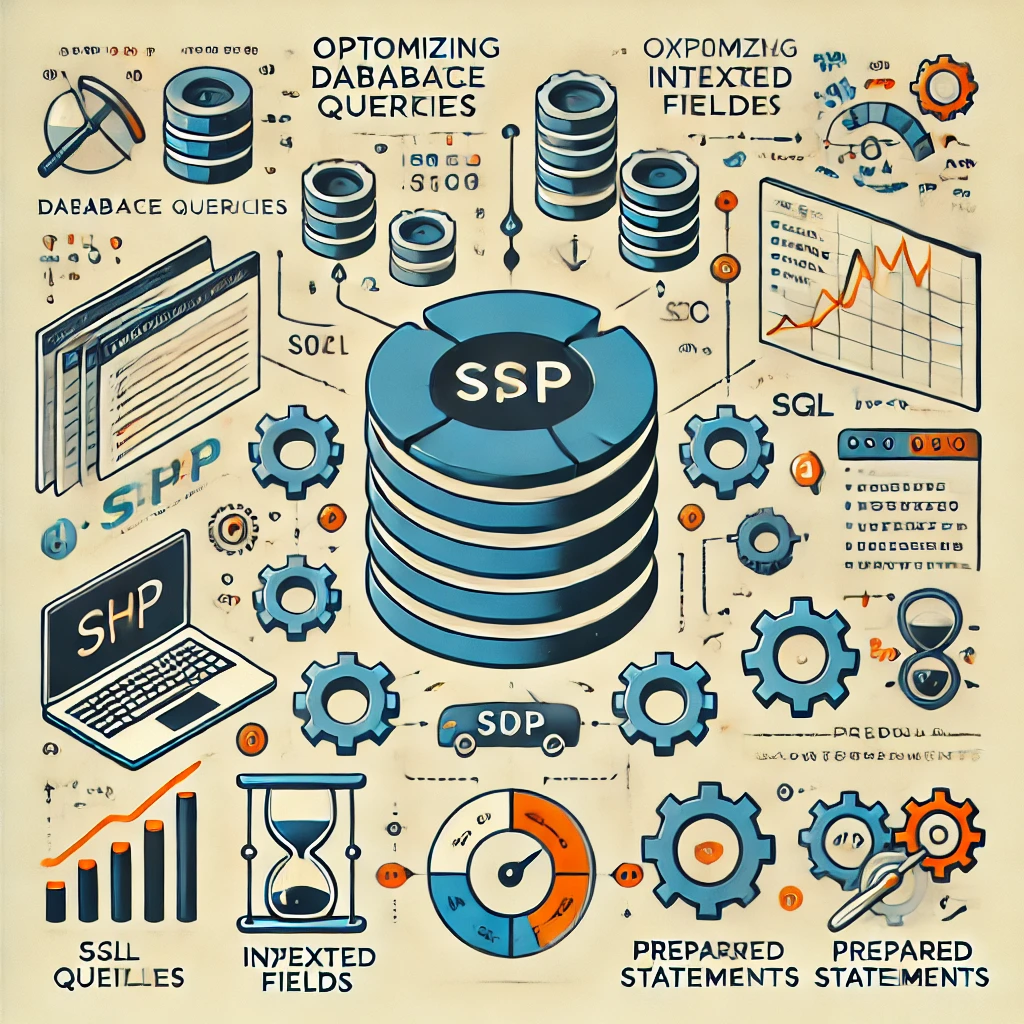
Code Example:
// Using a JOIN to optimize fetching blog post with comments
$query = "SELECT posts.*, comments.* FROM posts LEFT JOIN comments ON posts.id = comments.post_id WHERE posts.id = ?";
$stmt = $db->prepare($query);
$stmt->execute([$postId]);
$results = $stmt->fetchAll();
Real-World Scenario: Imagine running a high-traffic e-commerce site. Each user request might require multiple database calls to display product information, user details, and recommendations. Using OPcache to store compiled bytecode and caching database results with Memcached, you can drastically reduce response times, ensuring customers don’t abandon their carts due to slow page loads.
Beginner Example: Suppose you have a product listing page that fetches product details from the database each time a user visits. By caching these product details for a few minutes, you reduce the number of database hits, resulting in faster page load times and less server strain.
3.Use Built-In Functions Wisely
PHP comes packed with numerous built-in functions that are optimized for performance. When possible, use these functions rather than writing your own. For instance, functions like in_array()
or array_search()
are highly efficient and optimized for their purpose. If there’s a built-in function that suits your needs, always prefer it over a custom solution.
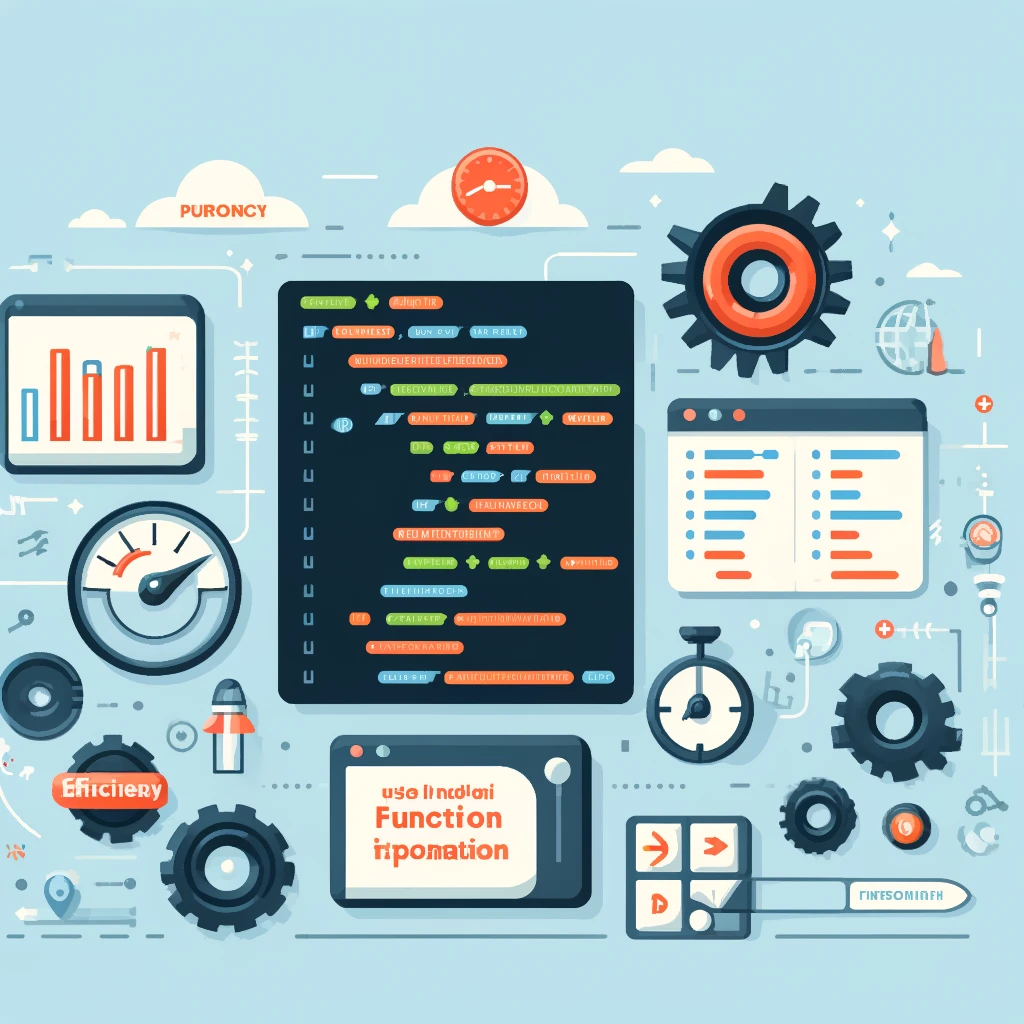
Code Example
// Instead of writing a custom function to search for a value in an array
function customSearch($needle, $haystack) {
foreach ($haystack as $value) {
if ($value === $needle) {
return true;
}
}
return false;
}
// Use the built-in function
$found = in_array($needle, $haystack); // This is faster and more efficient
4. Avoid Overloading with Loops
Nested loops are notorious for dragging down performance. Always try to minimize loop nesting or find ways to reduce iterations. Instead of using multiple for
loops, consider array functions such as array_map(), array_filter(), or foreach with logical breaks to keep operations efficient.
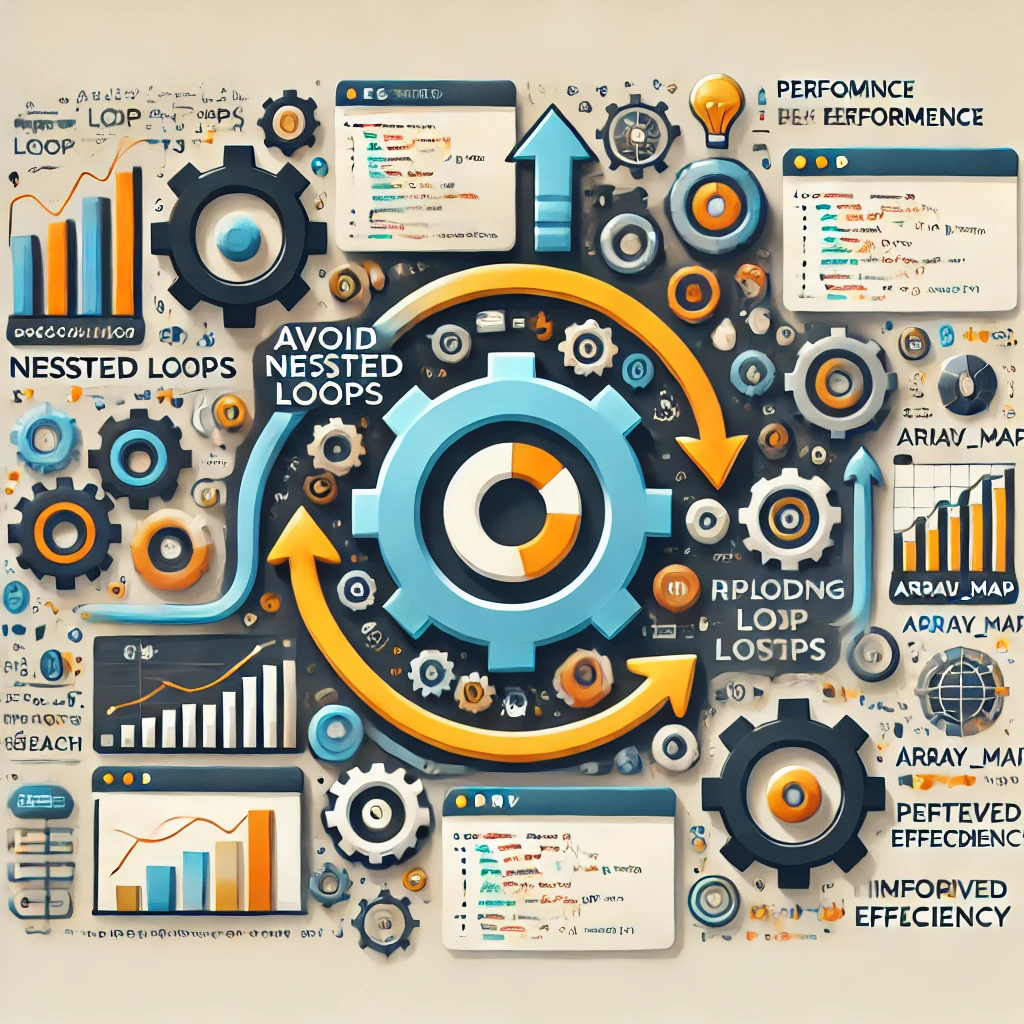
Code Example:
// Using array_map to apply a function to each element
$numbers = [1, 2, 3, 4, 5];
$squaredNumbers = array_map(function($number) {
return $number * $number;
}, $numbers);
5. Profile Your Code
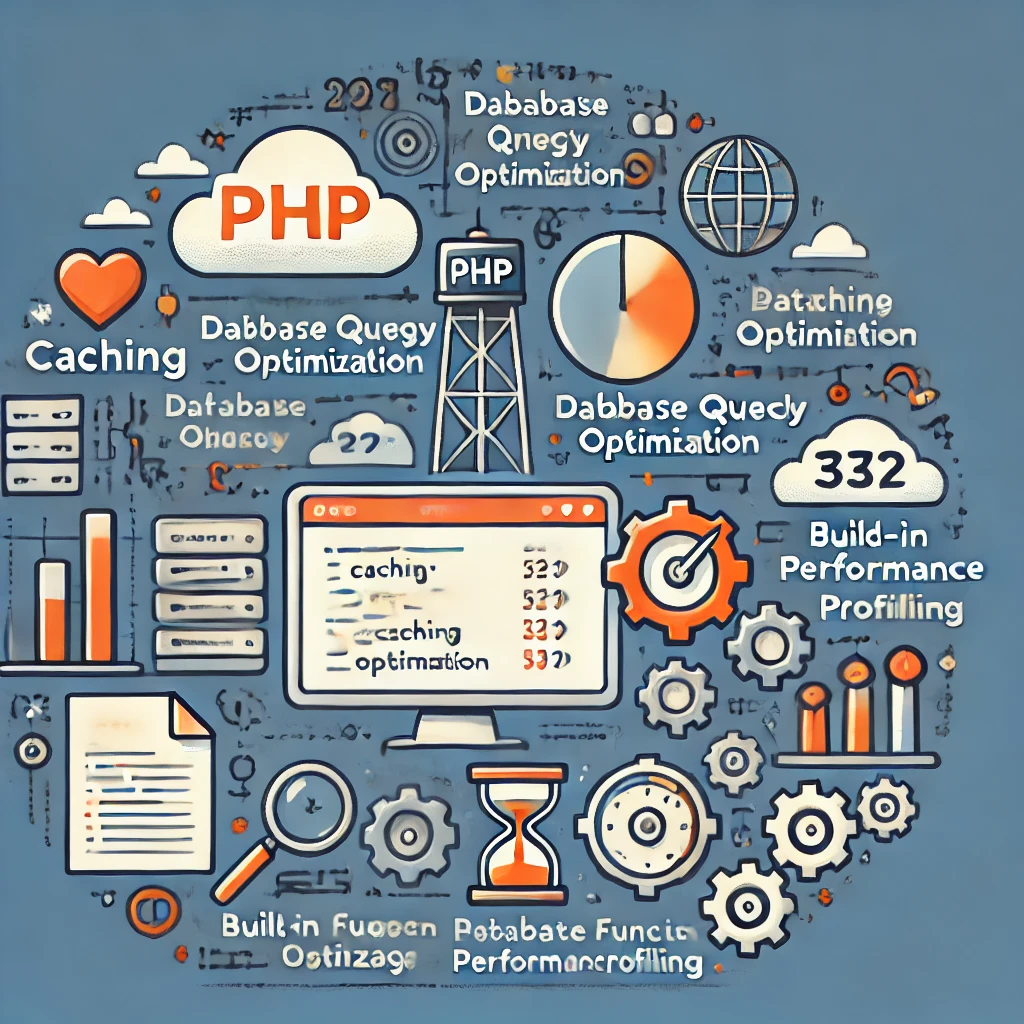
How can you optimize if you don’t know where the bottlenecks are? Tools like Xdebug and Blackfire help you understand which parts of your code are taking up the most time. Profiling tools give a clear picture of function execution times, memory usage, and other performance metrics. By knowing what’s slowing you down, you can target your optimization efforts more effectively.
Real-World Scenario: Suppose you have an API endpoint that’s taking too long to respond. By profiling the code, you find that a specific function involving multiple nested loops consumes most of the time. You can significantly reduce response times by optimizing this function or replacing it with a more efficient algorithm.
Beginner-Friendly Setup for Xdebug:
Install Xdebug: pecl install xdebug
Enable Xdebug
in your php.ini
file:
zend_extension="xdebug.so"
xdebug.profiler_enable = 1
Restart your server and analyze the generated cachegrind file using tools like KCacheGrind.
Code Example (Using Xdebug):
// To profile your script with Xdebug, enable Xdebug profiling in your php.ini
xdebug.profiler_enable = 1;
// Analyze the generated cachegrind file using tools like KCacheGrind.
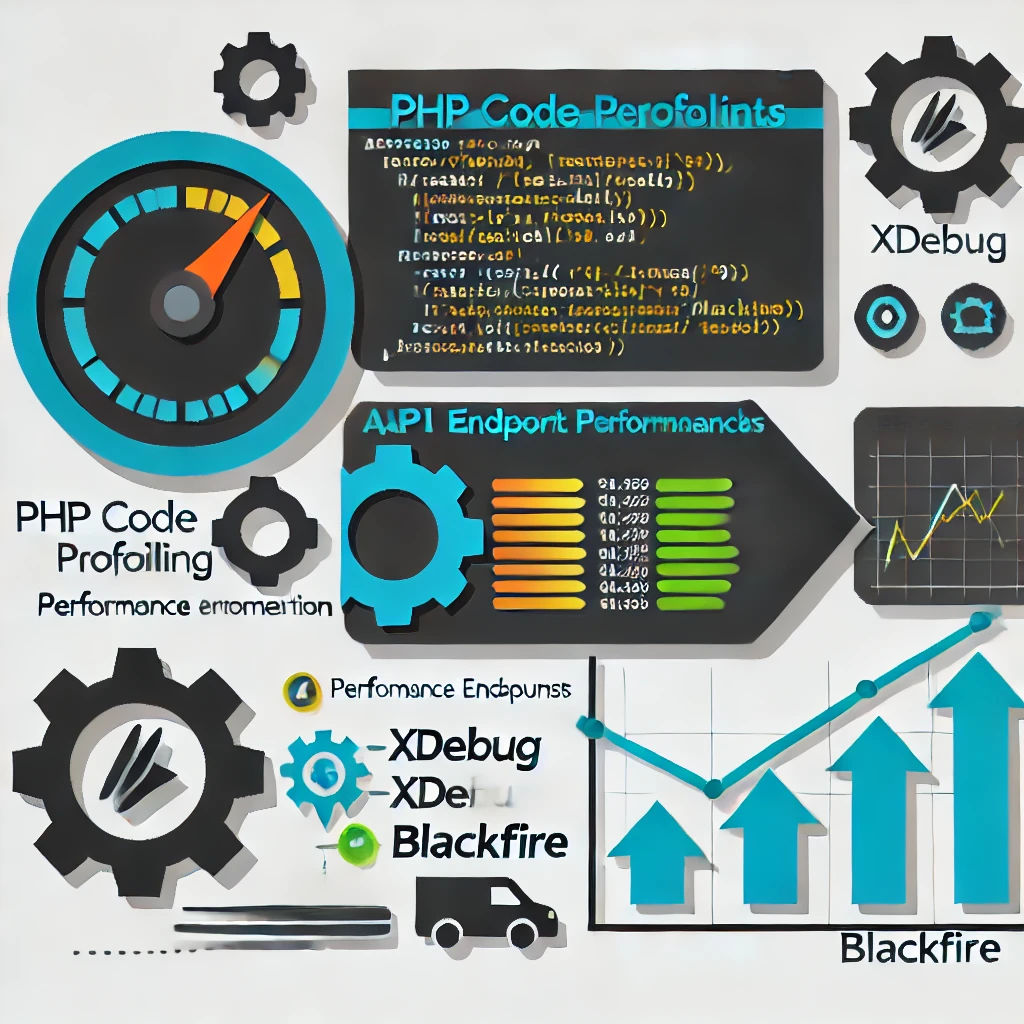
6.Use Autoloaders Wisely
Using autoloaders helps keep your code clean, but overuse can add significant overhead. Ensure you’re only loading what you need and keeping unnecessary classes out of memory. Consider using Composer’s built-in autoload optimization to load only the required courses.
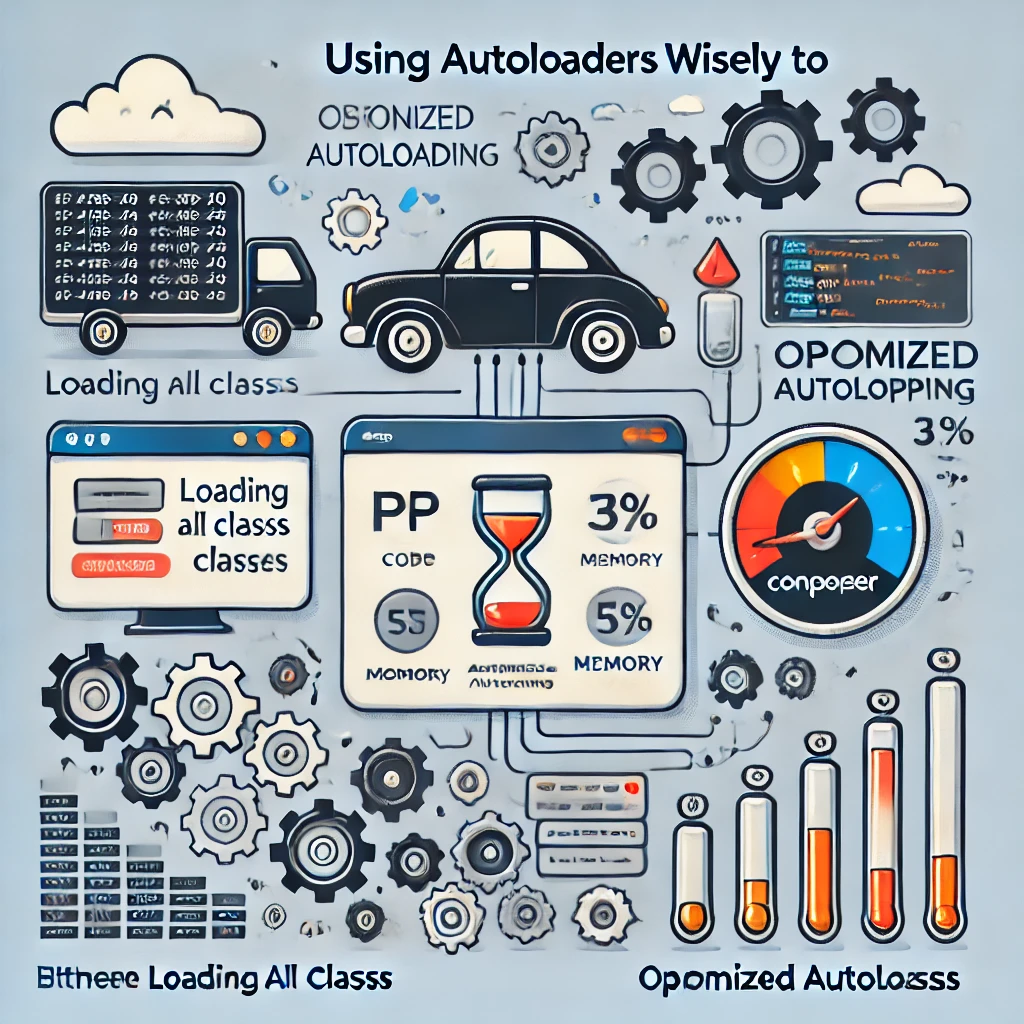
Real-World Scenario: In a large web application with many different modules, using a global autoloader can lead to significant overhead. For example, if every request loads all classes, it will increase memory usage unnecessarily. Instead, configure Composer’s autoloading to load only what is required, which can significantly reduce load times and memory consumption.
Beginner Example: When setting up autoloading with Composer, use:
{
"autoload": {
"psr-4": {
"App\\": "src/"
}
}
}
After editing composer.json
, run composer dump-autoload --optimize
to ensure only the necessary files are loaded.
7. Leverage Output Buffering
Output buffering is a lesser-known trick for PHP performance optimization. Instead of sending data directly to the client line-by-line, enable output buffering to gather all your output and send it in one go. This reduces the number of trips back and forth, thereby improving load times.
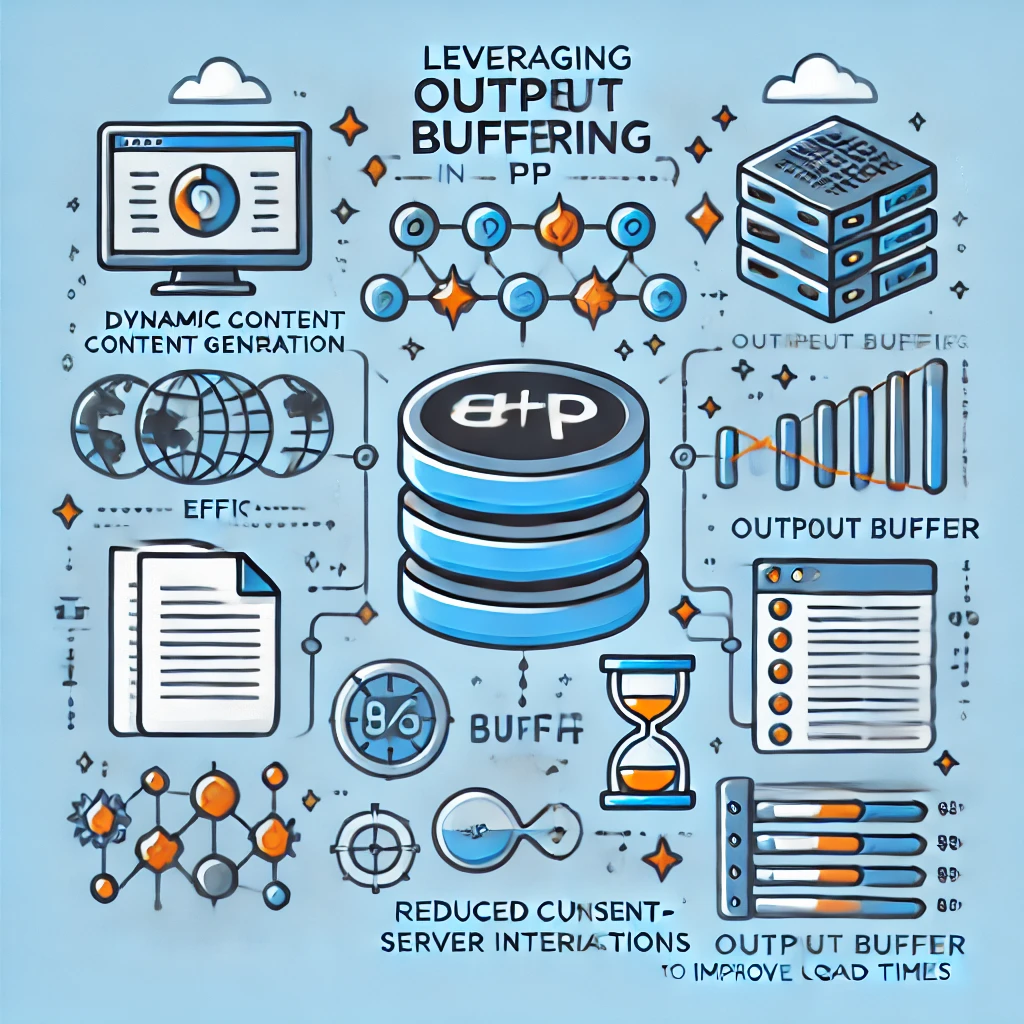
Real-World Scenario: For a high-traffic blog site that serves dynamic content, enabling output buffering can help reduce the load time significantly. By collecting all content and sending it at once, the server minimizes interactions with the client, especially when generating large HTML pages.
Code Example:
// Enable output buffering
ob_start();
echo "This is some content.";
echo " More content.";
// Send all output at once
echo ob_get_clean();
Beginner Example: For a simple form submission, instead of echoing each response line by line, use output buffering to collect the output and send it in one go. This reduces server-client interaction time.
8. Minimize File I/O
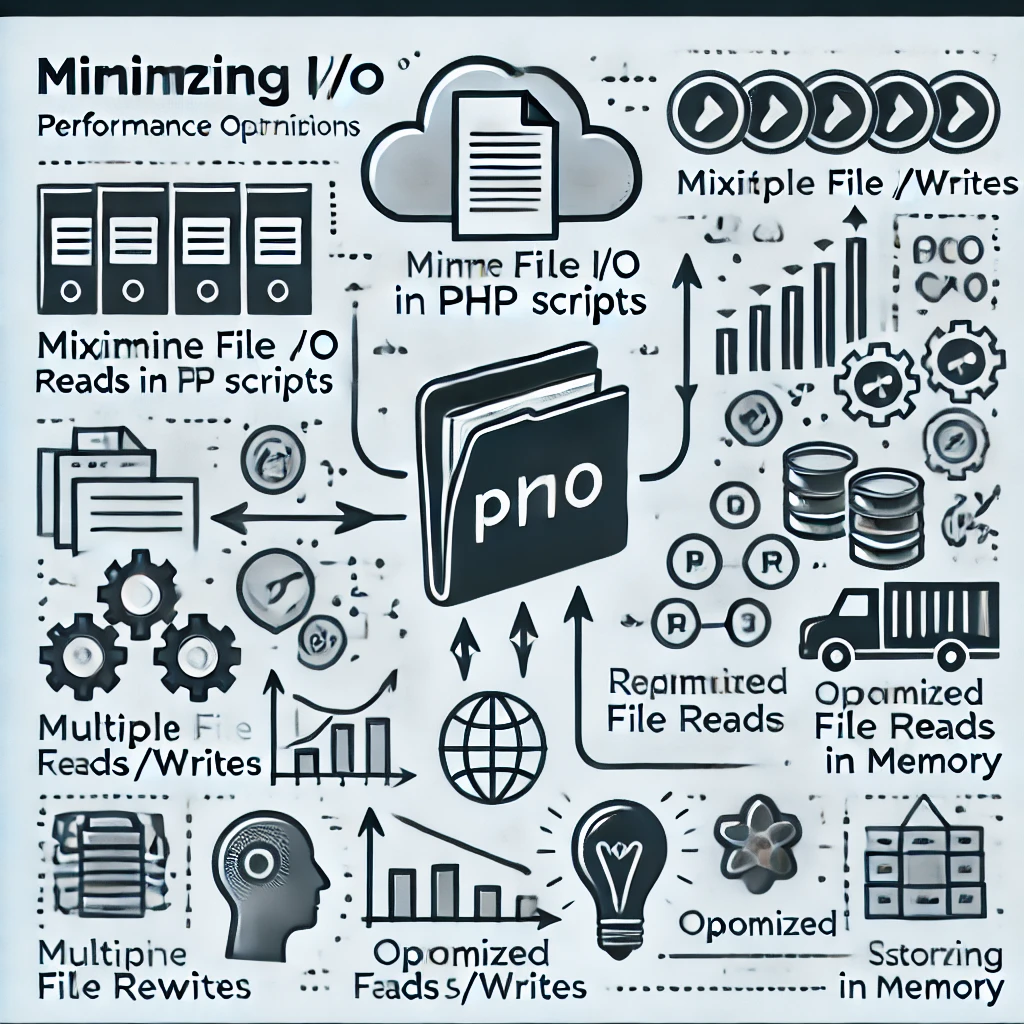
Accessing files can be costly in terms of performance, especially when dealing with large datasets. Where possible, try to minimize file reads and writes. Keep commonly accessed files in memory or use caching mechanisms to avoid redundant I/O operations. Additionally, if you’re including multiple PHP files, combine them where possible to reduce the number of include
or require
statements.
Real-World Scenario: In a reporting system where you need to generate large CSV files, minimize the file writes by collecting all the data in memory first, then writing it all at once. This can save considerable I/O time and improve script performance.
Beginner Example: If your script reads configuration settings from a file repeatedly, consider reading the file once and storing the data in an array. This way, you avoid the overhead of opening and reading the file multiple times.
9. Turn Off Debugging in Production
Make sure to turn off error reporting and debugging tools in a production environment. Not only do they pose a security risk, but they also slow down your application by generating unnecessary logs and outputs.
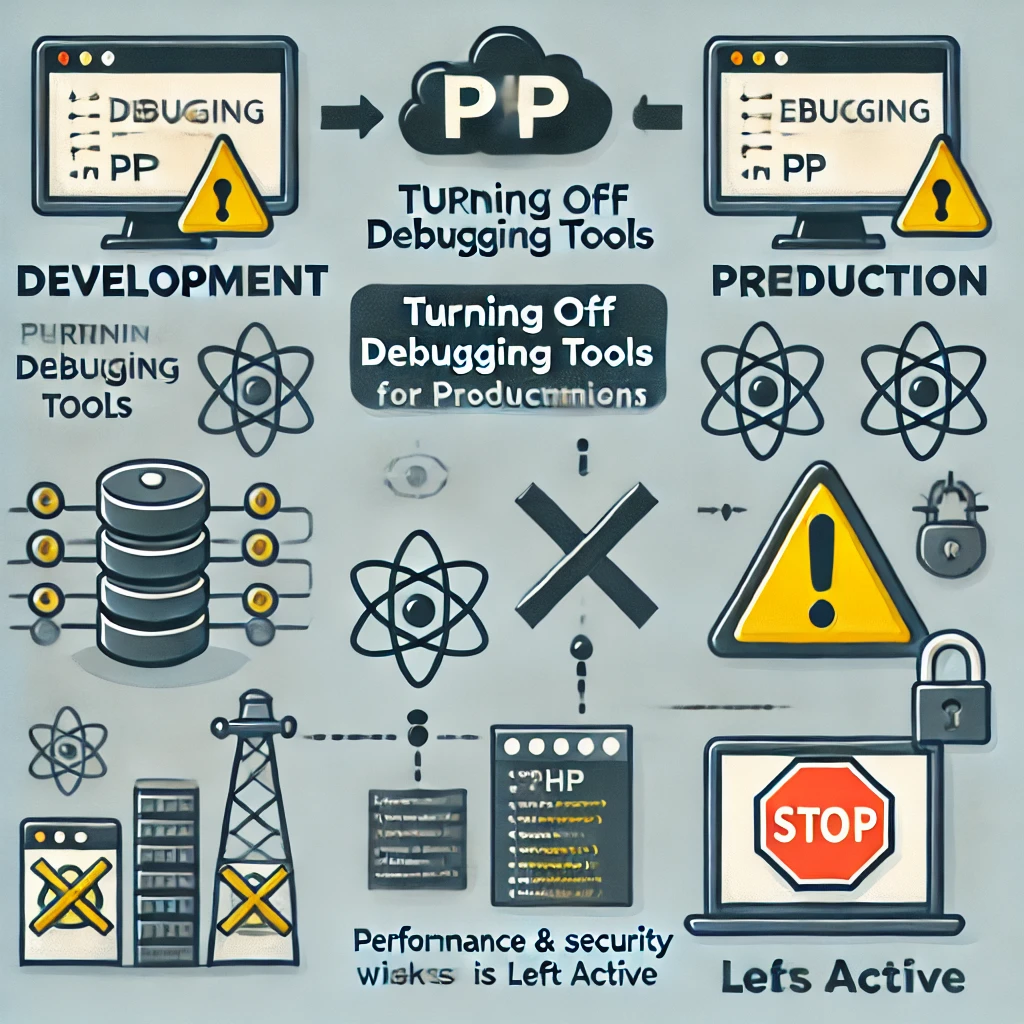
10. Use Content Delivery Networks (CDNs)
While this isn’t directly related to your PHP code, using a CDN can greatly improve the performance of your web applications by offloading static assets and reducing server load. This allows your server to focus on executing PHP scripts, resulting in a much faster and more responsive experience.
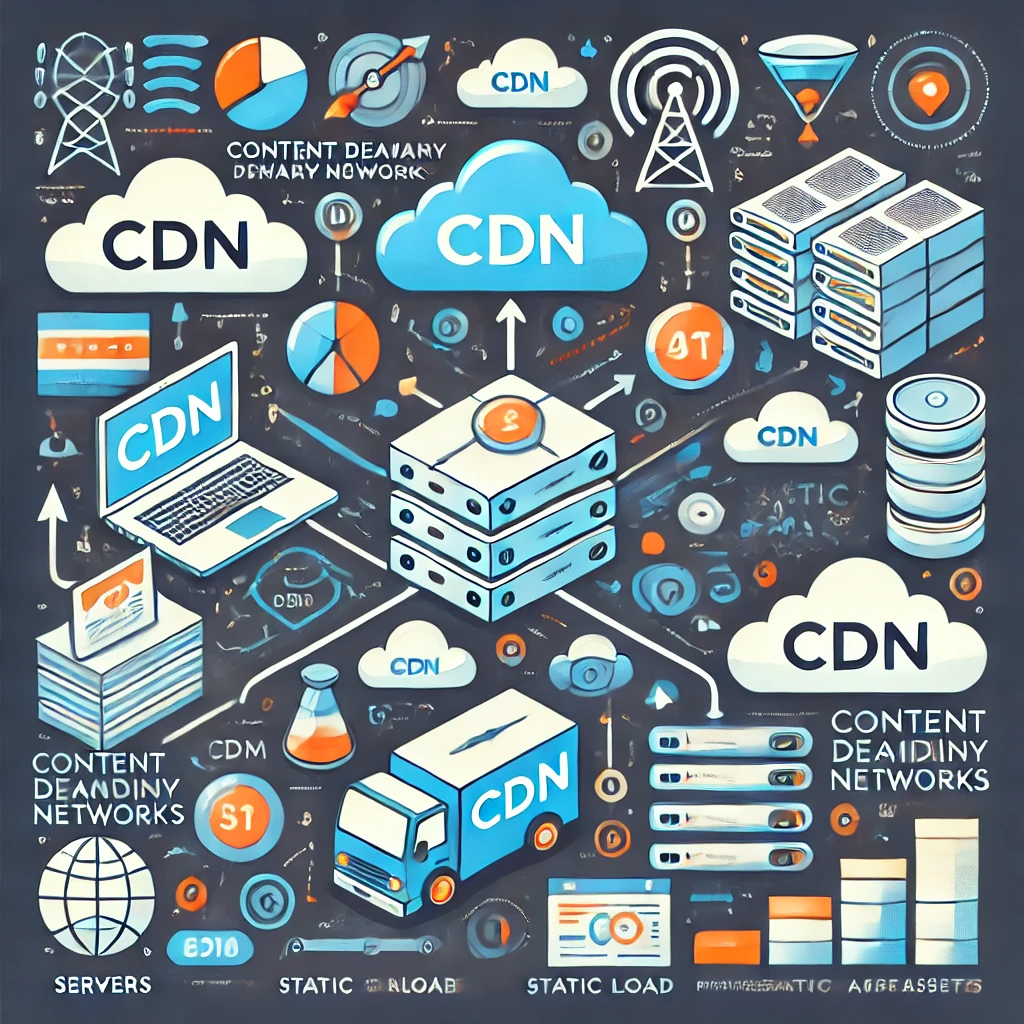
Conclusion
Performance optimization isn’t just about writing code that works; it’s about writing code that works efficiently. By following these best practices—caching results, optimizing database interactions, leveraging built-in functions, and profiling your scripts—you can ensure that your PHP application remains snappy and delivers a top-notch user experience. Remember, a faster website is a happier website—both for you and your users.
Have you tried any of these techniques, or do you have a favorite method for optimizing your PHP scripts? Drop a comment below and share your insights!
Responses