A Comprehensive Guide to WordPress Plugin Development: From Basics to Advanced Features
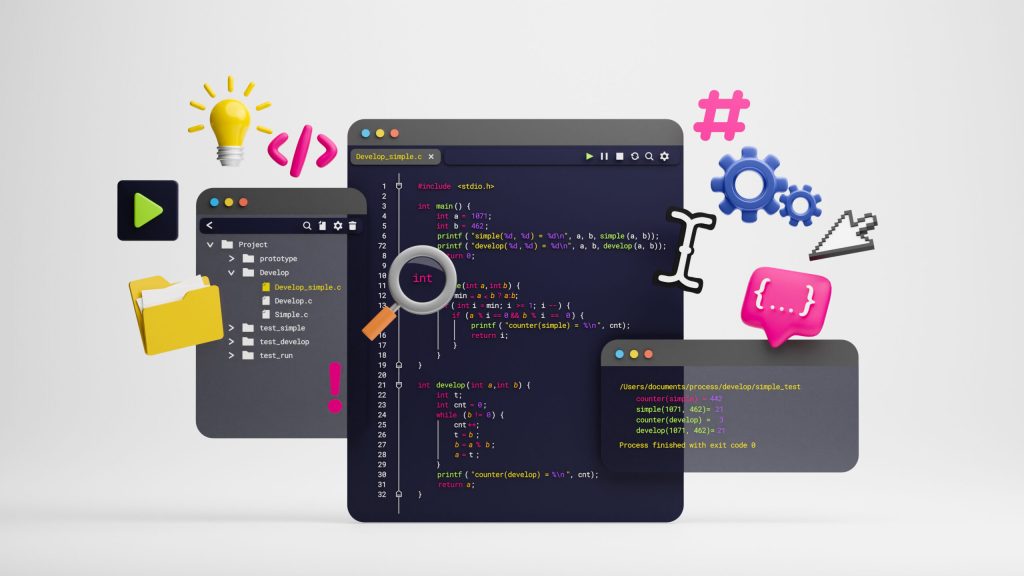
WordPress plugins are a powerful way to extend the functionality of your WordPress website. Plugins are the go-to solution if you want to add custom features, integrate third-party services, or simply optimize user experience. In this guide, we’ll take you through the essentials of developing a WordPress plugin—from understanding the basics to implementing more advanced features. This guide is designed to be beginner-friendly, with clear instructions and practical examples to help you get started.
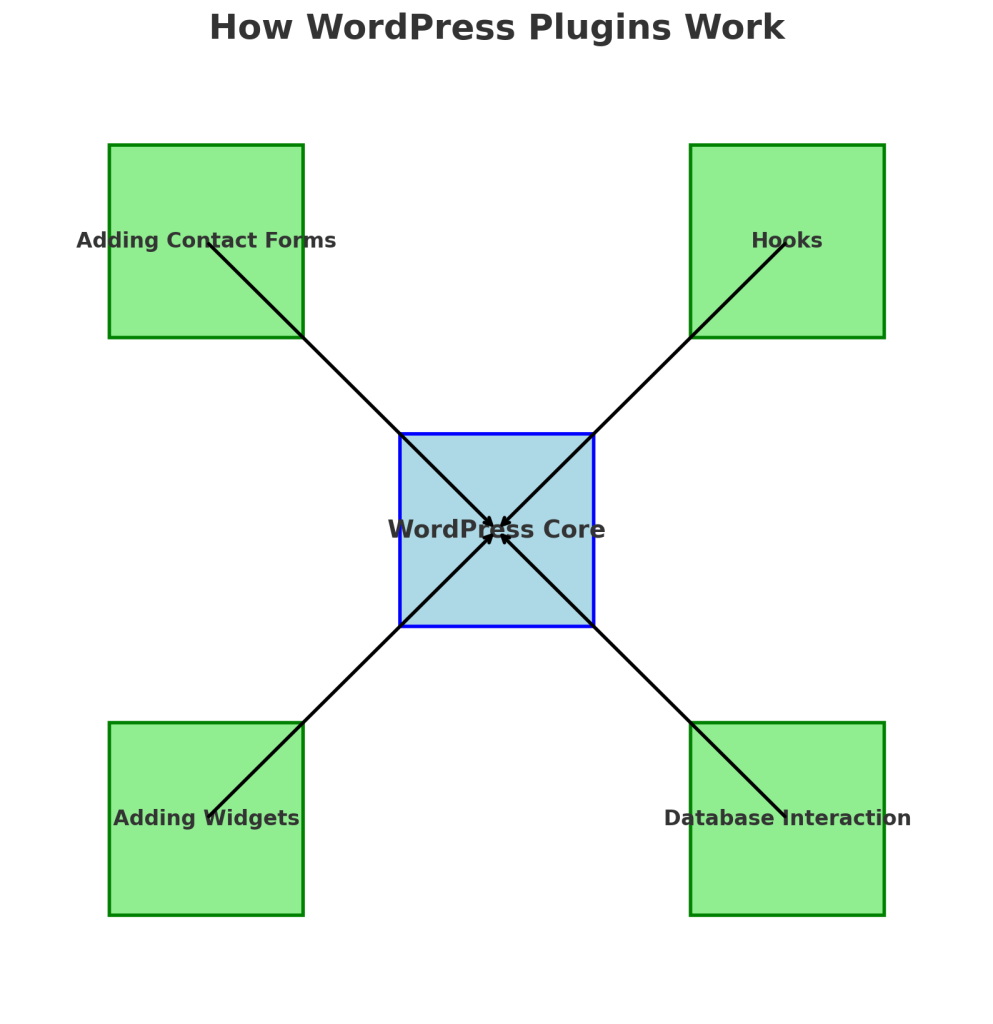
What is a WordPress Plugin?
At its core, a WordPress plugin is software that adds specific features or functionalities to a WordPress website. Plugins enable customization without altering the core code, making it possible to create unique websites while benefiting from the security and updates provided by WordPress. Plugins can range from something simple, like adding a contact form, to more complex systems, like e-commerce solutions.
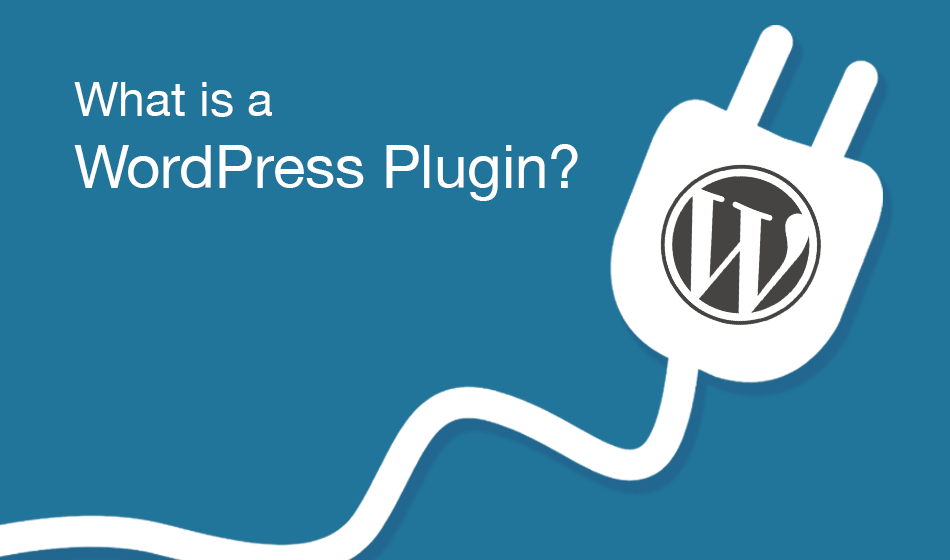
Example: Contact Form 7
To better understand how plugins work, consider a popular plugin: Contact Form 7. This plugin allows users to easily create and manage multiple contact forms, customize them with simple markup, and ensure that all submissions are emailed directly to the website owner. It allows users to add a contact form to their site without needing to write any code from scratch, making it easy for website owners to stay connected with their audience while ensuring a seamless experience.
Plugins like Contact Form 7 showcase how easy it is to extend WordPress functionality with minimal effort, providing essential features that enhance user interaction.
A WordPress plugin is software that adds specific features or functionalities to a WordPress website. Plugins enable customization without altering the core code, making it possible to create unique websites while benefiting from the security and updates provided by WordPress. Plugins can range from something simple, like adding a contact form, to more complex systems, like e-commerce solutions.
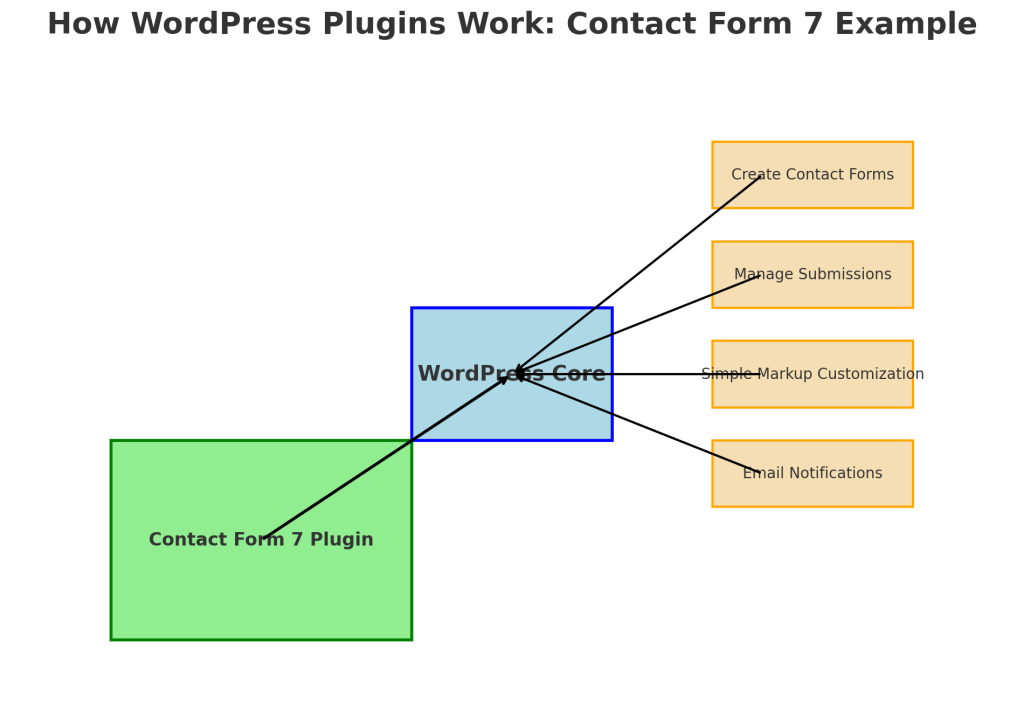
Getting Started: Basics of Plugin Development
Setting Up Your Plugin
Accessing the WordPress file system can be challenging for beginners unfamiliar with server directories. Here’s a step-by-step guide to help you get started:
- Accessing the File System: To access the WordPress file system, you need to have access to your website’s server. There are two common ways to do this:
- Using FTP (File Transfer Protocol): You can use an FTP client like FileZilla to connect to your server. You will need your FTP credentials, which are usually provided by your hosting provider. Once connected, navigate to the
/wp-content/plugins/
directory. - Using a Hosting File Manager: Many hosting providers offer a file manager in their control panel (such as cPanel or Plesk). You can use this file manager to navigate to the
/wp-content/plugins/
directory and create or edit files directly.
- Using FTP (File Transfer Protocol): You can use an FTP client like FileZilla to connect to your server. You will need your FTP credentials, which are usually provided by your hosting provider. Once connected, navigate to the
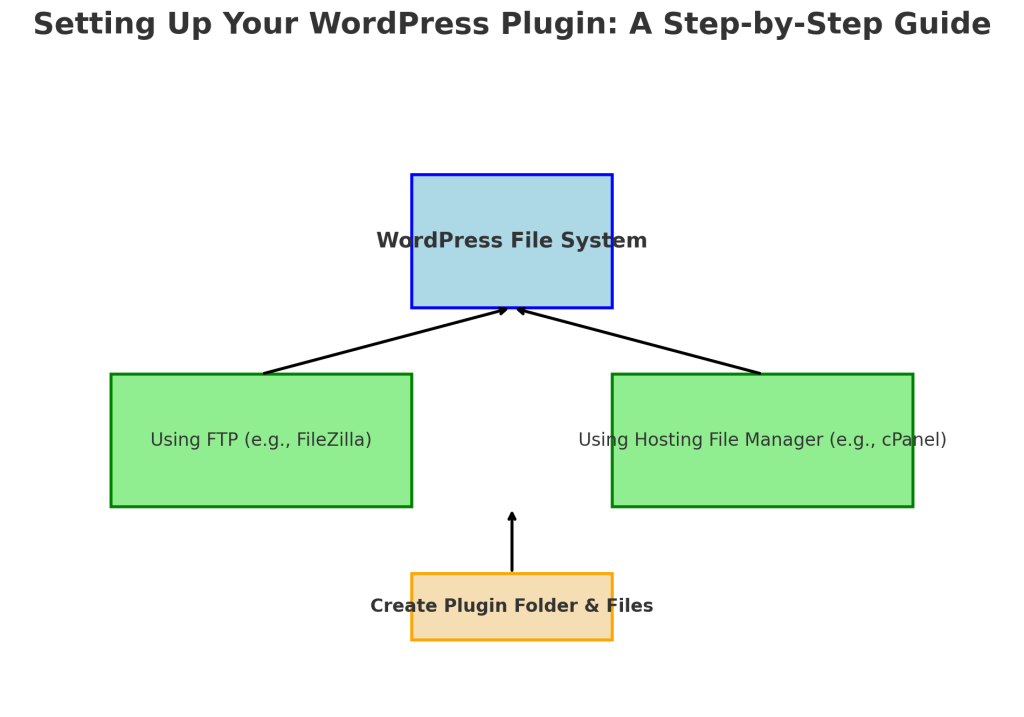
Once you have access to the file system, you can create your plugin folder and files as described below.
To start developing a WordPress plugin, follow these detailed steps:
- Create the Plugin Folder:
- Navigate to the
/wp-content/plugins/
directory on your WordPress installation. - Create a new folder named
my-first-plugin
. This folder will contain all the files related to your plugin.
- Navigate to the
- Create the Main PHP File:
- Inside the newly created folder, create a file named
my-first-plugin.php
. This will serve as the main entry point for your plugin.
- Inside the newly created folder, create a file named
- Add Plugin Header Information:
- Add a header comment at the top of the file to let WordPress recognize it as a plugin:
- This header information is crucial as it allows WordPress to display the plugin correctly in the admin dashboard.
- Activate the Plugin:
- Go to your WordPress dashboard, navigate to Plugins, and you should see your new plugin listed there. Click Activate to enable it.
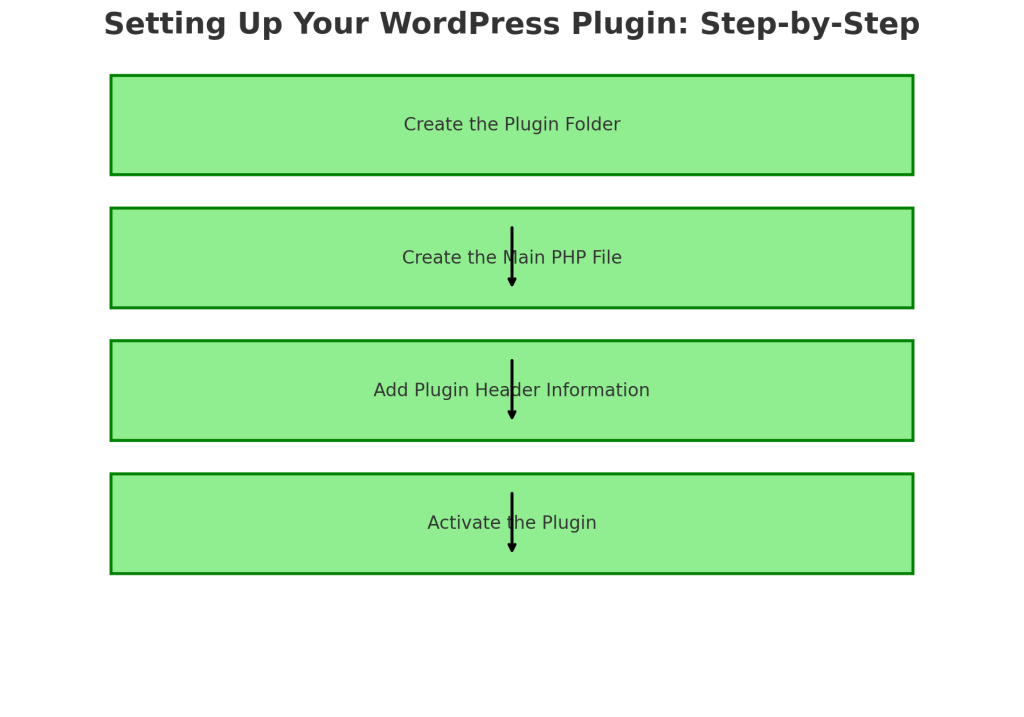
This simple setup forms the skeleton of a WordPress plugin. From here, you can add more complex features to enhance functionality.
Understanding Hooks: Actions and Filters
Hooks are the backbone of WordPress plugin development. They allow you to “hook into” WordPress, making it possible to modify the default behavior or add new functionalities without changing core files.
To help visualize how hooks work, imagine WordPress as a train moving along a track. Hooks are like train stations where you can stop and either add more passengers (functionality) or change the passengers (data) already on board. Action Hooks are like stops where you can add or remove passengers (run custom code), while Filter Hooks are like checkpoints where you can inspect and modify the passengers (data) before they continue their journey.
If you’re a visual learner, think of action hooks as opportunities to add new tasks at specific points in the WordPress process, while filter hooks let you adjust or transform data before it’s displayed. Visual diagrams, like flowcharts of how WordPress processes a request, can also be very helpful to understand where hooks are applied.
Hooks are the backbone of WordPress plugin development. They allow you to “hook into” WordPress, making it possible to modify the default behavior or add new functionalities without changing core files.
- Action Hooks are used to execute custom code at specific points during WordPress’s execution, such as when a post is published.
- Filter Hooks are used to modify existing data before it is displayed, like customizing the content of a post.
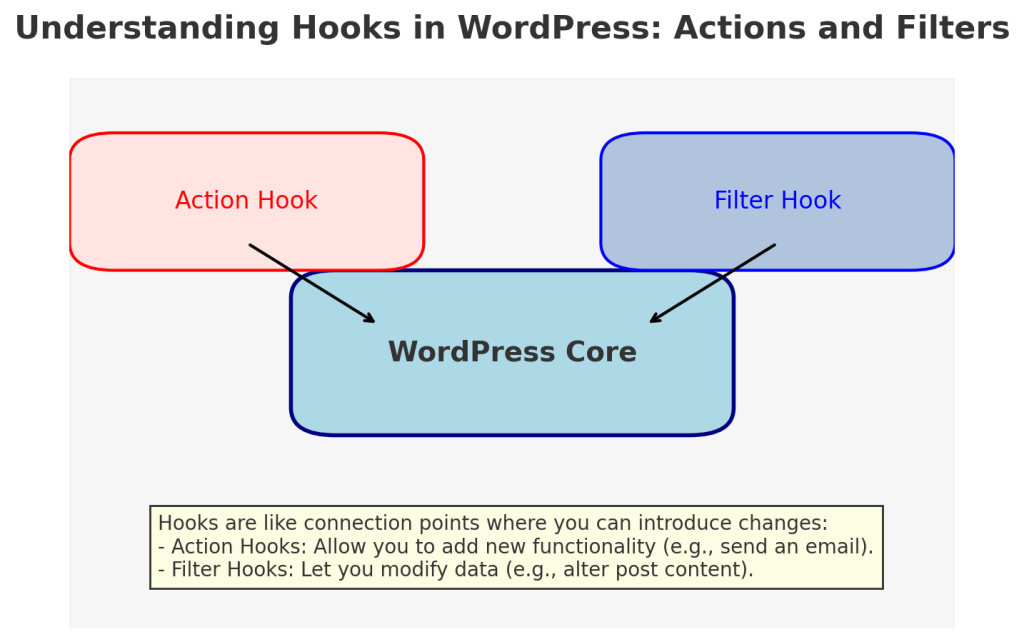
Example: Adding a Custom Message to Posts
Let’s say you want to add a message at the end of every post. You can use the the_content
filter like this:
Let’s break down what each part of this code does:
add_filter('the_content', 'add_custom_message');
- This line registers the custom function
add_custom_message
to thethe_content
filter hook. This means that whenever WordPress is preparing to display the content of a post, it will apply our function to modify it.
- This line registers the custom function
function add_custom_message($content)
- Here, we define the
add_custom_message
function. The function takes one parameter,$content
, which is the existing content of the post that WordPress has generated.
- Here, we define the
if (is_single()) { $content .= '<p>Thank you for reading! Follow us for more updates.</p>'; }
- The
is_single()
function checks if the current page is a single post page. If it is, we append (.=
) the custom message to the$content
variable. This ensures the message is only added to individual posts and not other types of content, like pages or archives.
- The
return $content;
- Finally, we return the modified
$content
so that WordPress can display it. Without this return statement, the content of the post would be empty.
- Finally, we return the modified
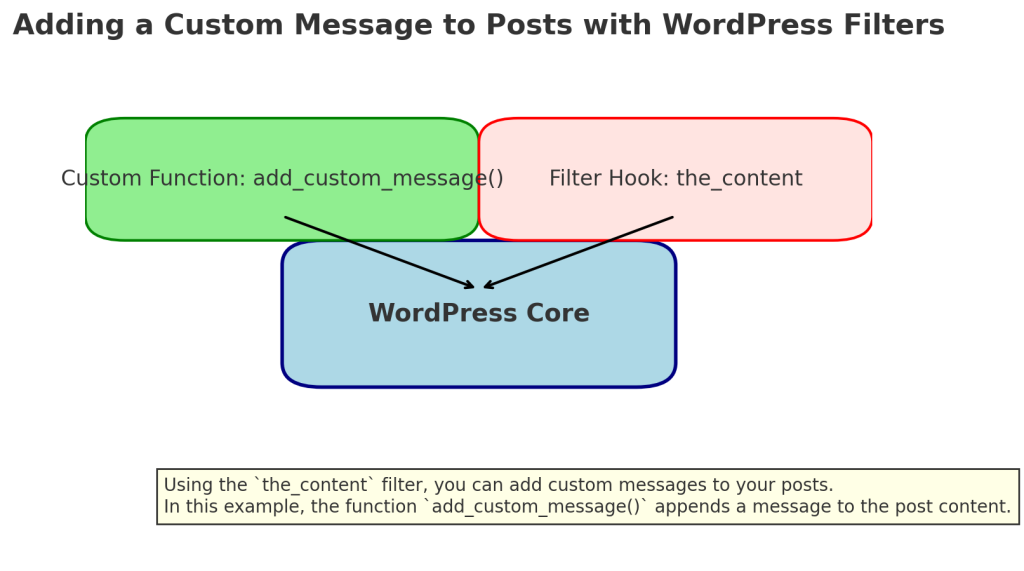
By using filters, you can easily manipulate the default output of WordPress to include custom messages, advertisements, or any other additional content.
Let’s say you want to add a message at the end of every post. You can use the the_content
filter like this:
This code appends a message to each single post’s content. By using filters, you can easily manipulate the default output of WordPress.
Adding Common Plugin Features
Shortcodes
Shortcodes are an easy way to add dynamic content into posts, pages, or widgets. They are particularly useful for embedding forms, media, and other reusable elements. For example, many contact form plugins use shortcodes to allow users to easily place a form anywhere on their site. Similarly, shortcodes can be used to embed videos, image galleries, or even product listings.
Let’s create a simple shortcode that outputs a greeting message:
Shortcodes are an easy way to add dynamic content into posts, pages, or widgets. Let’s create a simple shortcode that outputs a greeting message:
Now, you can add [greeting]
anywhere in your content, and it will display the greeting message.
Shortcodes are extremely useful for creating reusable elements that can be placed anywhere on your site.
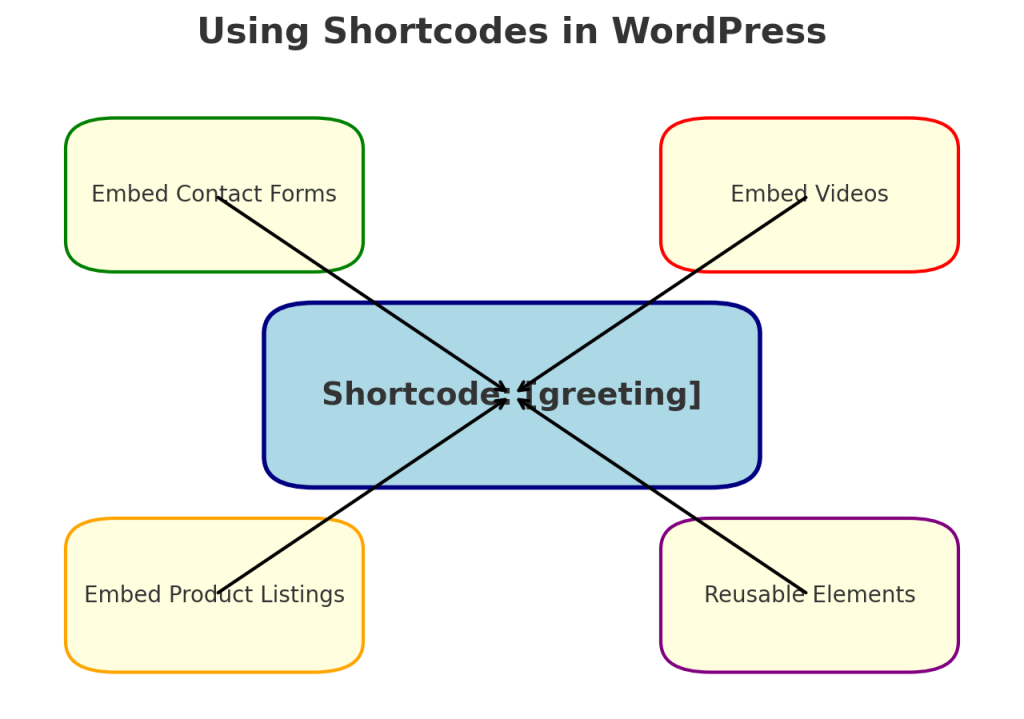
Widgets
Widgets are another powerful way to extend WordPress functionality. Widgets are small blocks that perform specific functions and can be added to widget areas in your theme, such as sidebars or footers. Many WordPress themes have predefined widget areas that you can customize by adding widgets, making it easy to enhance your site without modifying the theme code.
To use widgets effectively, you also need to understand how to register widget areas in your theme. For example, if you want to add a new area for widgets in your theme’s footer, you can do so by adding the following code to your theme’s functions.php
file:
This code registers a new widget area called “Footer Widget Area” that you can add widgets to from the WordPress admin dashboard.
To create a custom widget, extend the WP_Widget
class:
Widgets are another powerful way to extend WordPress functionality. To create a custom widget, extend the WP_Widget
class:
This widget will display a simple message and can be added to any widgetized area in your theme. Widgets are ideal for adding content to sidebars or footers.
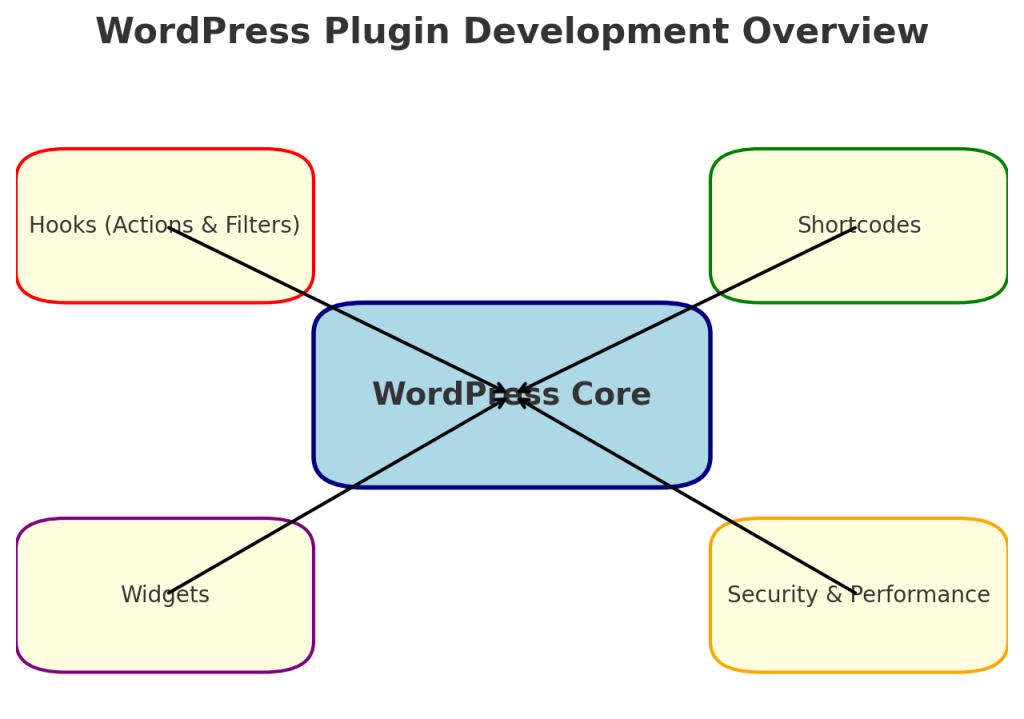
Ensuring Security and Performance
Security Best Practices
When developing a plugin, keeping your users’ data safe is crucial. Here are some key security practices:
- Sanitize User Input: Use functions like
sanitize_text_field()
,esc_html()
, oresc_url()
to sanitize data before saving or displaying it. This helps prevent malicious code from being executed on your site and mitigates common vulnerabilities like Cross-Site Scripting (XSS).- For example, if you are saving a user-provided URL, use
esc_url()
to ensure that only valid URLs are saved.
- For example, if you are saving a user-provided URL, use
- Escape Output: Always escape data before outputting it to the browser to prevent XSS attacks. Use functions like
esc_html()
,esc_attr()
, andesc_url()
depending on the context in which the data is being output. - Nonces: Nonces are used to verify that requests are coming from a legitimate source. Use
wp_create_nonce()
andcheck_admin_referer()
to secure forms and AJAX requests.- For instance, to ensure a form is secure, you can add a nonce field like this:And verify it upon form submission:
- This protects against Cross-Site Request Forgery (CSRF), ensuring that the form submission is coming from a valid source.
- SQL Injection Protection: When interacting with the database, avoid directly writing SQL queries with user input. Instead, use the
$wpdb
class to safely handle queries.- For example, to safely fetch data from a custom table:
- The
$wpdb->prepare()
function ensures that user input is properly escaped, preventing SQL injection attacks.
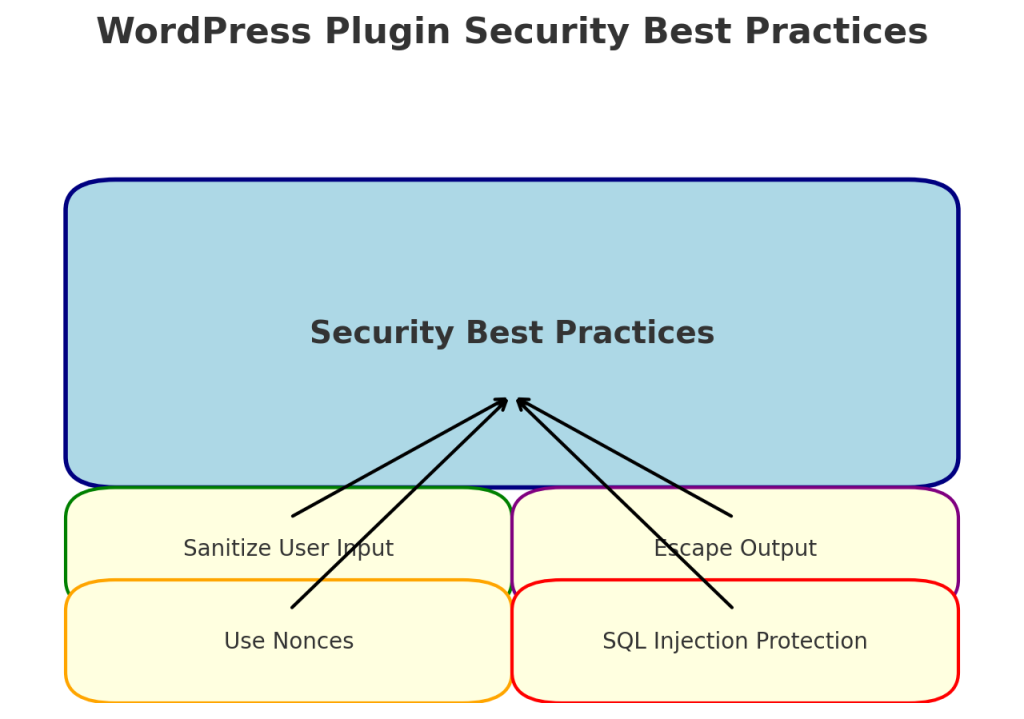
When developing a plugin, keeping your users’ data safe is crucial. Here are some key security practices:
- Sanitize User Input: Use functions like
sanitize_text_field()
,esc_html()
, oresc_url()
to sanitize data before saving or displaying it. This helps prevent malicious code from being executed on your site. - Nonces: Nonces are used to verify that requests are coming from a legitimate source. Use
wp_create_nonce()
andcheck_admin_referer()
to secure forms and AJAX requests.
For instance, to ensure a form is secure, you can add a nonce field like this:
And verify it upon form submission:
These measures help protect your plugin from common vulnerabilities like Cross-Site Request Forgery (CSRF).
Performance Optimization
- Load Scripts and Styles Properly: Use
wp_enqueue_script()
andwp_enqueue_style()
to load JavaScript and CSS files. This ensures that your scripts and styles are only loaded when needed, improving site performance.- Using Conditional Logic: To further optimize performance, you can use conditional logic to ensure that scripts and styles are only loaded on relevant pages. For example, if you only need a script on a specific admin page, you can use:This ensures that the script is only loaded on the relevant admin page, reducing the overall load on other pages.
- Database Optimization: If your plugin interacts with the database, make sure to use the
$wpdb
class to safely interact with the WordPress database and avoid direct SQL queries. - Load Scripts and Styles Properly: Use
wp_enqueue_script()
andwp_enqueue_style()
to load JavaScript and CSS files. This ensures that your scripts and styles are only loaded when needed, improving site performance. - Database Optimization: If your plugin interacts with the database, make sure to use the
$wpdb
class to safely interact with the WordPress database and avoid direct SQL queries.
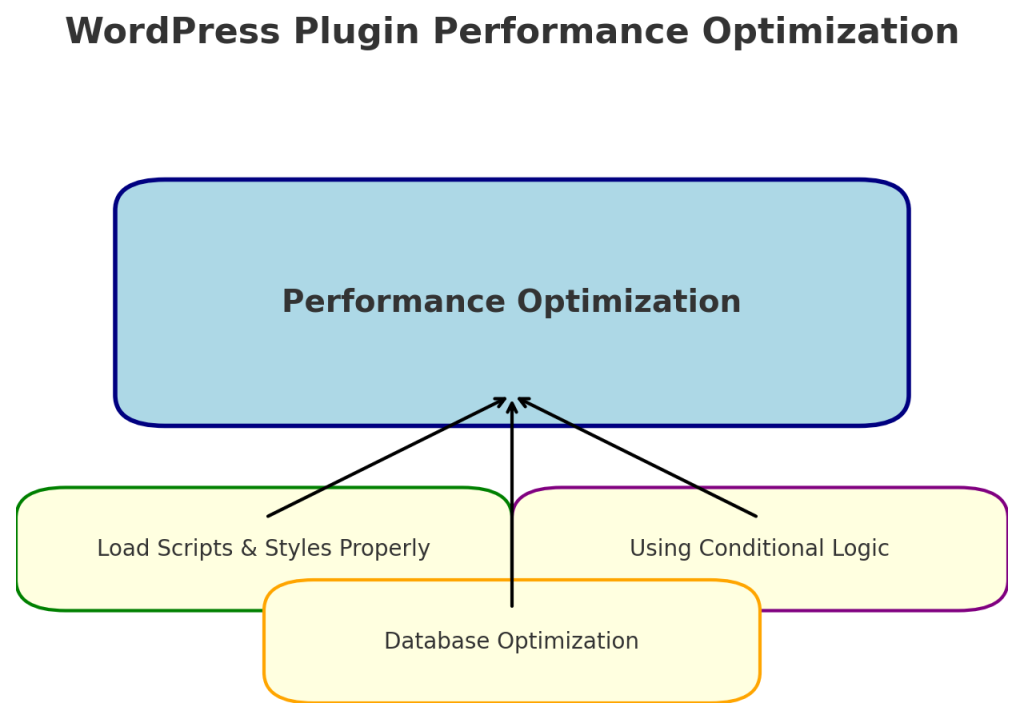
For example, to safely fetch data from a custom table:
Always validate and sanitize the data used in queries to prevent SQL injection.
Releasing and Maintaining Your Plugin
Once your plugin is functional, you might want to share it with the community. Here is a checklist to prepare your plugin for distribution:
- Prepare for Distribution:
- Version Control: Use a version control system like Git to keep track of changes to your plugin code. This helps manage updates and collaboration.
- Documentation: Write comprehensive documentation for your plugin. Include installation instructions, usage guides, and FAQs. Good documentation helps users understand how to use your plugin effectively.
- WordPress Coding Standards: Make sure your code adheres to the WordPress Coding Standards. This ensures your code is readable and maintainable.
- Test Thoroughly:
- Clean WordPress Installation: Test your plugin on a clean WordPress installation to ensure it works out of the box.
- Compatibility Testing: Check for compatibility with popular themes and other plugins to avoid conflicts.
- Debugging: Use the
WP_DEBUG
mode to catch any errors or warnings during testing.
- Submit to the WordPress Plugin Repository:
- You can submit your plugin via the WordPress Plugin Repository. The submission process includes a review by the WordPress team to ensure your plugin meets quality and security standards.
- Ongoing Maintenance:
- Keep Your Plugin Updated: Update your plugin regularly to fix bugs, patch security vulnerabilities, and ensure compatibility with the latest WordPress version.
- User Feedback: Pay attention to user feedback and support requests to improve the plugin and address any issues.
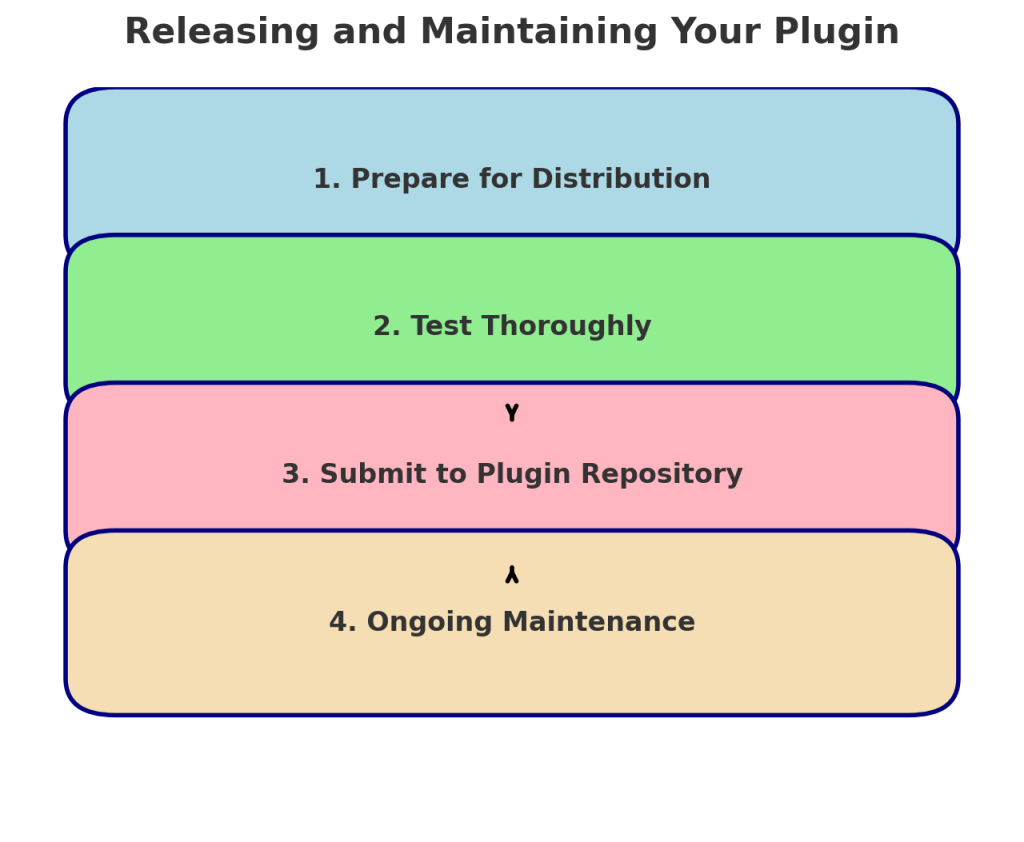
Maintaining your plugin is just as important as developing it. Keeping your plugin up to date ensures it remains useful, secure, and compatible with future versions of WordPress.
Once your plugin is functional, you might want to share it with the community. Here are the basic steps to release your plugin:
- Prepare for Distribution: Make sure your code is well-documented and your plugin adheres to the WordPress Coding Standards.
- Test Thoroughly: Test your plugin on a clean WordPress installation and ensure compatibility with different themes and other plugins. Testing helps ensure that your plugin doesn’t conflict with other popular plugins.
- Submit to the WordPress Plugin Repository: You can submit your plugin via the WordPress Plugin Repository. The submission process includes a review by the WordPress team to ensure your plugin meets quality and security standards.
Maintaining your plugin is just as important as developing it. Keep your plugin up to date, patch security vulnerabilities, and ensure compatibility with the latest WordPress version.
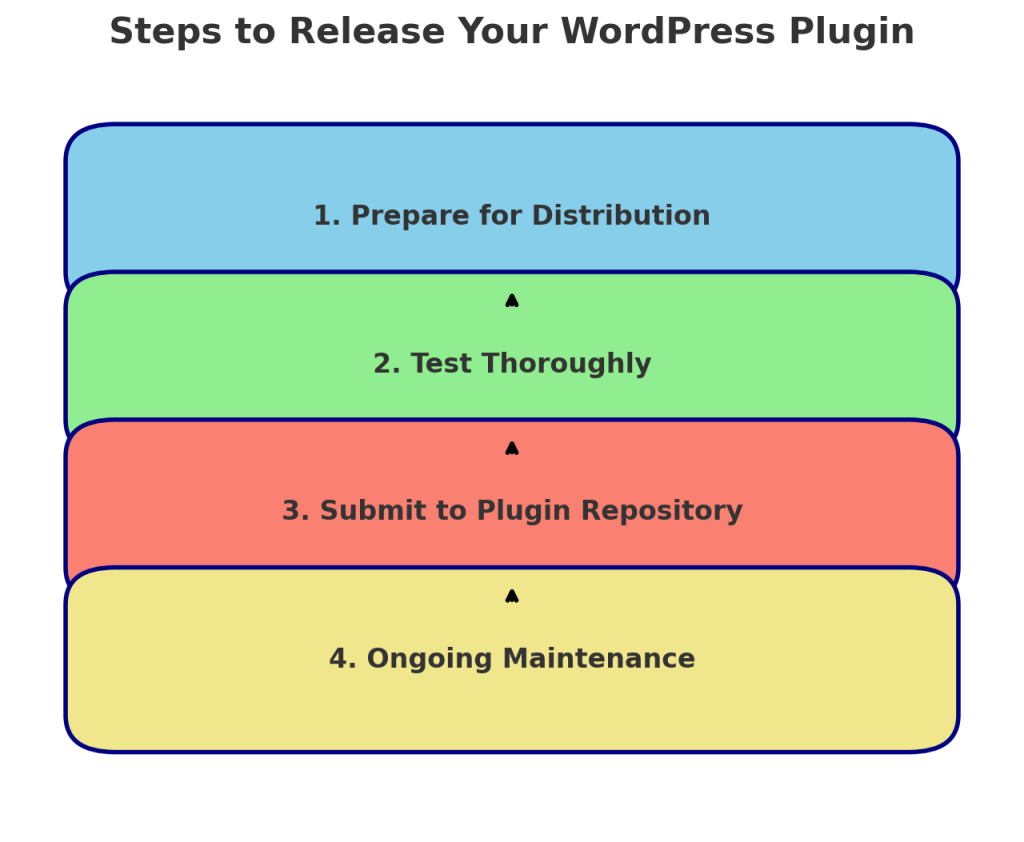
Conclusion
WordPress plugin development offers an incredible opportunity to customize and extend the functionality of WordPress websites. By understanding the basics, leveraging hooks, and following best practices, you can create powerful plugins that cater to specific needs. The possibilities are endless, whether you’re just starting or looking to add advanced features.
If you’re a beginner, start small—perhaps by adding a simple custom message or creating a basic shortcode. As you grow more comfortable, you can move on to more advanced features like widgets, custom admin settings, or integrating third-party APIs.
Responses