Automated testing can feel daunting for WordPress developers, mainly if you’ve never dealt with it. Think of it like having a safety net while performing on a tightrope—once in place, it ensures that even if something goes wrong, you’re protected. Just as a safety net catches mistakes, automated tests catch errors before they make it into production. But in a development world that values stability, efficiency, and the ability to scale, automated testing is one of the most effective tools you can adopt to streamline your workflow. This guide is written for WordPress developers looking to enhance their quality assurance process and grow as professionals. I’ll walk you through setting up automated testing, complete with code examples and practical explanations.
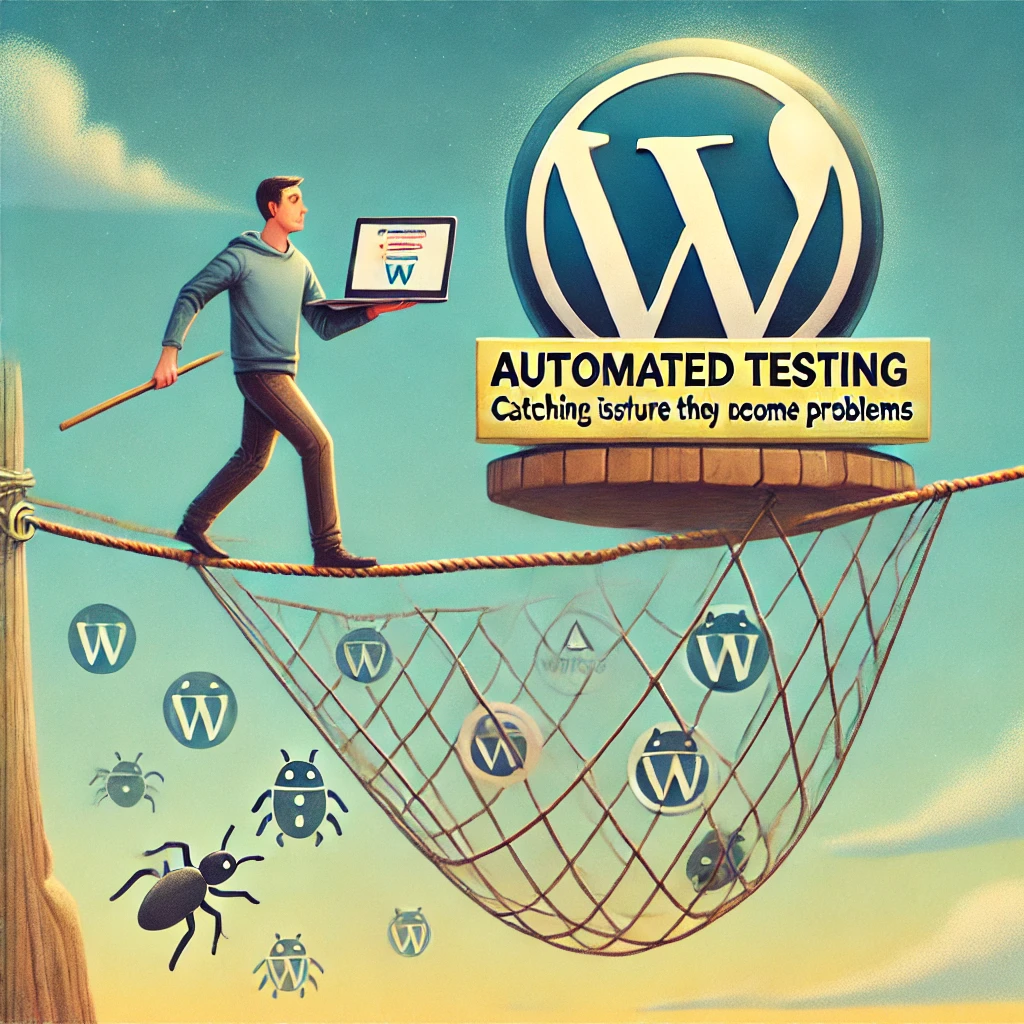
什麼是自動化測試?
簡單來說,自動化測試是一種方法,可確保您所寫的程式碼能如預期般運作,而無需手動檢查每個功能。它是關於執行腳本,以驗證您應用程式的不同方面。這個外掛程式在最新更新之後是否仍然運作?主題自訂是否仍能正常呈現?自動化測試有助於在開發生命週期中及早發現潛在問題,為您節省無數的錯誤修復與除錯時間。
WordPress 的自動化測試可以包含單元測試、整合測試,甚至是端對端測試,涵蓋小至邏輯片段,大至完整呈現的網頁。讓我們來看看如何將自動測試整合到 WordPress 開發工作流程中。
設定您的環境
First, you’ll need the right environment to get started. PHPUnit is an excellent choice for WordPress because it is specifically designed to test PHP code, which comprises the core of WordPress plugins and themes. It allows developers to isolate individual functions and verify their behavior in a controlled environment, making it especially useful for catching errors early in development. A popular tool for running automated tests in a WordPress setup is PHPUnit. PHPUnit is perfect for unit testing PHP code—a crucial WordPress plugins and themes component. Here’s what you need to set up:
- 安裝 PHPUnit:確保您的系統已安裝 Composer,因為 PHPUnit 是透過 Composer 安裝的。您可以使用以下指令來安裝:
composer require --dev phpunit/phpunit
- 設定 WordPress 測試套件: Download and configure the WordPress testing library. This is a simplified version, assuming you’re setting this up locally:
bash bin/install-wp-tests.sh wordpress_test_dbroot 'password' localhost latest
使用您的實際資料庫憑證取代占位符。為了加強安全性,請避免直接在腳本中硬體編碼敏感資訊。取而代之的是,考慮使用環境變數或不在版本控制中追蹤的安全組態檔案。
撰寫簡單的單元測試
讓我們為自訂函式建立一個簡單的單元測試。單元測試的設計是為了驗證單獨的程式碼,例如函式或方法,是否能獨立正確運作。它們是自動化測試的基礎,有別於整合測試 (確保多個元件一起運作) 和端對端測試 (從頭到尾測試應用程式的整個流程)。假設您的外掛程式中有一個函數 calculate_discount():
To write a unit test for this function, you’ll need to create a new test file under your tests folder. Here’s an example:
// 檔案: my-plugin/tests/test-functions.php
使用 PHPUnit\Framework\TestCase;
class FunctionsTest extends TestCase {
public function test_calculate_discount() {
require_once dirname(__FILE__) .'/../includes/functions.php';
$result = calculate_discount(100, 20);
$this->assertEquals(80, $result, '20% discount on $100 should return $80');
}
public function test_invalid_discount() {
$this->expectException(InvalidArgumentException::class);
calculate_discount(100, -10);
}
}
執行測試
寫完測試後,您可以使用下列指令執行:
供應商/bin/phpunit
使用 Cypress 進行端對端測試
Unit testing is a fantastic starting point, but for complex WordPress sites, you’ll also want to check the site’s full functionality. Unit tests focus on testing individual components in isolation, whereas integration tests ensure that different modules work together as expected. On the other hand, end-to-end tests simulate real user scenarios to verify that the entire system works as intended, from the backend to the user interface. That’s where 賽普拉斯 來的。Cypress 是一套以 JavaScript 為基礎的端對端測試工具,可與 WordPress 搭配使用,並允許您以程式化的方式與實際的網頁介面互動。
首先,將 Cypress 加入您的開發環境:
npm install cypress --save-dev
接下來,建立一個簡單的 Cypress 測試,檢查您的 WordPress 首頁是否能正確載入:
// 檔案:cypress/integration/homepage.spec.js
describe(「WordPress 首頁」, () => {
it('should load the homepage', () => {
cy.visit('http://localhost:8000');
cy.contains('Welcome to WordPress').should('be.visible');
});
});
運行 Cypress 與:
npx 檜木開口
將測試整合至 CI/CD 輸送管道
當自動測試與持續整合/持續部署 (CI/CD) 管道整合時,就能發揮效益。服務如 GitHub 動作 或 GitLab CI 允許您在每次推送程式碼變更時執行測試。以下是 PHPUnit 的 GitHub Actions YAML 配置範例:.
# File: .github/workflows/phpunit.yml
name: PHPUnit Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up PHP
uses: shivammathur/setup-php@v2
with:
php-version: '8.0'
- name: Install dependencies
run: composer install
- name: Run PHPUnit
run: vendor/bin/phpunit
每次推送新程式碼或提出拉取請求時,此設定都會執行您的單元測試。在這些階段執行測試可確保新變更不會引入回歸或破壞現有功能,有助於在整個開發過程中維持程式碼品質。透過在管道中加入 Cypress 測試,您可以自動驗證後端邏輯和前端互動。
總結
在 WordPress 工作流程中實作自動化測試是改善程式碼品質與可靠性的決定性步驟。然而,開發人員經常面臨一些挑戰,例如設定測試環境、管理相依性,以及撰寫令人信服的測試案例。克服這些挑戰包括
- 仔細規劃。
- 使用環境配置的最佳實務。
- 利用社區資源解決共同問題。
雖然入門可能看起來很辛苦,但長期的好處--更少的錯誤、更簡易的維護、更快的部署--讓它變得值得。使用 PHPUnit 和 Cypress 等工具,您可以涵蓋測試的不同層面,並確保開發人員和使用者都能享有順暢的體驗。其他工具也值得考慮,例如用於測試 JavaScript 元件的 Jest 或用於更全面的瀏覽器自動化測試的 Selenium。
透過建立穩固的測試套件並將其整合到 CI/CD 管道中,您就可以放心地發佈功能,因為您知道每一個程式碼都經過徹底的檢查。自動化測試不只是花俏的工具,而是任何現代開發工作流程的支柱。