Automated testing can feel daunting for WordPress developers, mainly if you’ve never dealt with it. Think of it like having a safety net while performing on a tightrope—once in place, it ensures that even if something goes wrong, you’re protected. Just as a safety net catches mistakes, automated tests catch errors before they make it into production. But in a development world that values stability, efficiency, and the ability to scale, automated testing is one of the most effective tools you can adopt to streamline your workflow. This guide is written for WordPress developers looking to enhance their quality assurance process and grow as professionals. I’ll walk you through setting up automated testing, complete with code examples and practical explanations.
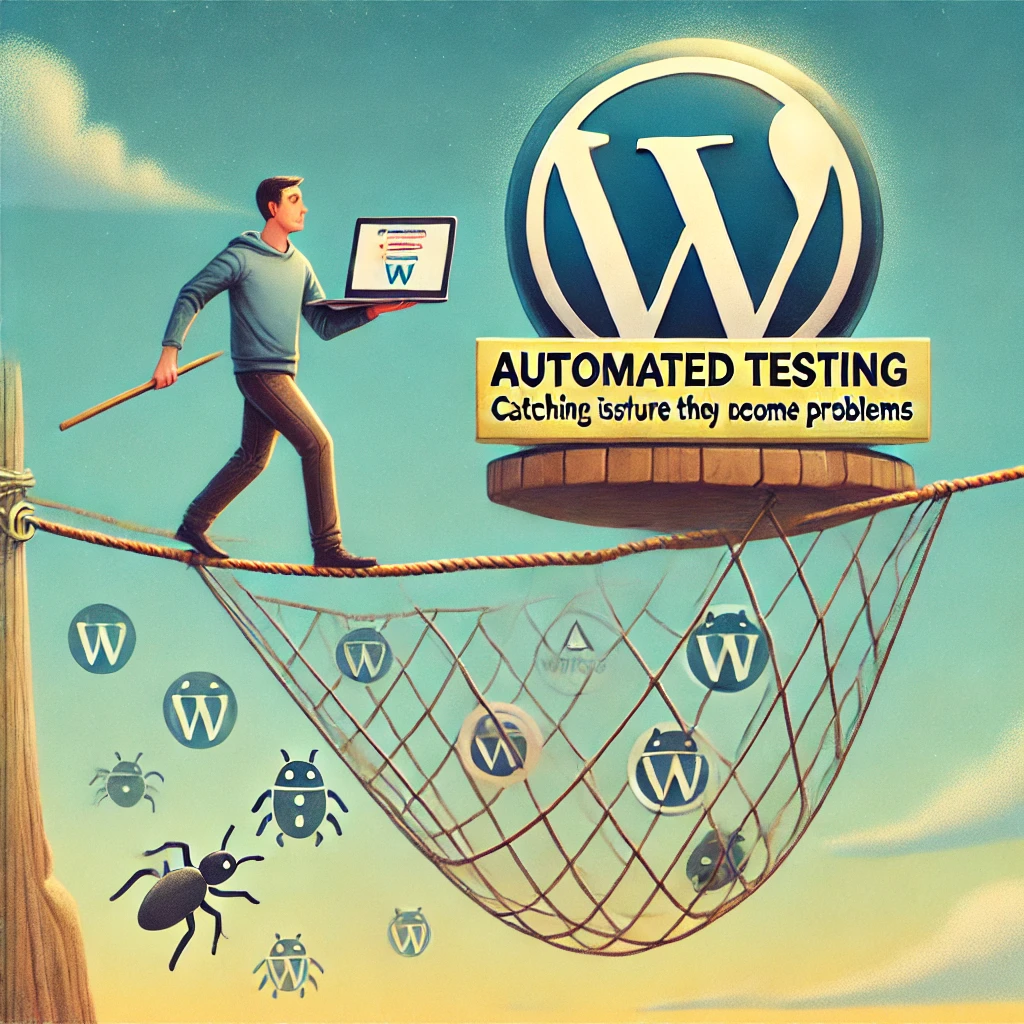
自動テストとは?
簡単に言えば、自動テストは、すべての機能を手動で検査することなく、あなたが書いたコードが意図したとおりに動作することを保証する方法です。アプリケーションのさまざまな側面を検証するスクリプトを実行することです。このプラグインは最新のアップデート後も動作しますか?テーマのカスタマイズは正しくレンダリングされていますか?自動テストは、開発ライフサイクルの早い段階で潜在的な問題を発見するのに役立ち、バグ修正やデバッグにかかる数え切れないほどの時間を節約します。
WordPress の自動テストには、単体テスト、統合テスト、さらには小さなロジックの断片から完全にレンダリングされたページまでをカバーするエンドツーエンドのテストまで含まれます。自動テストを WordPress の開発ワークフローに統合する方法を見てみましょう。
環境の設定
First, you’ll need the right environment to get started. PHPUnit is an excellent choice for WordPress because it is specifically designed to test PHP code, which comprises the core of WordPress plugins and themes. It allows developers to isolate individual functions and verify their behavior in a controlled environment, making it especially useful for catching errors early in development. A popular tool for running automated tests in a WordPress setup is PHPUnit. PHPUnit is perfect for unit testing PHP code—a crucial WordPress plugins and themes component. Here’s what you need to set up:
- PHPUnitのインストール:PHPUnit は Composer を使ってインストールします。以下のコマンドでインストールできます:
composer require --dev phpunit/phpunit
- WordPressテストスイートのセットアップ: Download and configure the WordPress testing library. This is a simplified version, assuming you’re setting this up locally:
bash bin/install-wp-tests.sh wordpress_test_dbroot 'password' localhost latest
プレースホルダを実際のデータベース認証情報に置き換えます。セキュリティを強化するために、スクリプトに直接機密情報をハードコーディングするのは避けましょう。代わりに、環境変数を使用するか、バージョン管理で追跡されない安全な設定ファイルを使用することを検討してください。
簡単なユニットテストの書き方
カスタム関数の簡単なユニットテストを作ってみましょう。ユニットテストは、関数やメソッドのような個々のコードの断片が、分離された状態で正しく動作することを検証するために設計されます。複数のコンポーネントが協調して動作することを保証する統合テストや、 アプリケーションの最初から最後までのフロー全体をテストするエンドツーエンドテストとは異なります。あなたのプラグインに calculate_discount() という関数があるとしましょう:
To write a unit test for this function, you’ll need to create a new test file under your tests folder. Here’s an example:
// ファイル: my-plugin/tests/test-functions.php
PHPUnitFramework を使用します;
クラス FunctionsTest extends TestCase {
public function test_calculate_discount() {
require_once dirname(__FILE__) .'/../includes/functions.php';
$result = calculate_discount(100, 20);
$this->assertEquals(80, $result, '20% discount on $100 should return $80');
}
public function test_invalid_discount() { $this->assert_invalid_discount(){。
$this->expectException(InvalidArgumentException::class);
calculate_discount(100, -10);
}
}
テストの実施
テストを書いたら、次のコマンドで実行できます:
ベンダ/bin/phpunit
サイプレスによるエンドツーエンド・テストの利用
Unit testing is a fantastic starting point, but for complex WordPress sites, you’ll also want to check the site’s full functionality. Unit tests focus on testing individual components in isolation, whereas integration tests ensure that different modules work together as expected. On the other hand, end-to-end tests simulate real user scenarios to verify that the entire system works as intended, from the backend to the user interface. That’s where サイプレス が入ってきます。CypressはJavaScriptベースのエンドツーエンドのテストツールで、WordPressとうまく連動し、実際のウェブインターフェイスとプログラムでやりとりすることができます。
まず、開発環境にCypressを追加します:
npm install cypress --save-dev
次に、WordPressのホームページが正しく読み込まれるかどうかをチェックする簡単なCypressテストを作成します:
// ファイル:cypress/integration/homepage.spec.js
describe('WordPressのホームページ', () => {
it('ホームページを読み込む', () => {
cy.visit('http://localhost:8000');
cy.contains('Welcome to WordPress').should('be.visible');
});
});
でサイプレスを走らせます:
NPXサイプレスオープン
CI/CDパイプラインへのテストの統合
自動テストは、継続的インテグレーション/継続的デプロイメント(CI/CD)パイプラインと統合すると有益です。以下のようなサービスがあります。 GitHub アクション または GitLab CI を使うと、コードの変更をプッシュするたびにテストを実行できるようになります。以下に、PHPUnit 用の GitHub Actions YAML 設定の例を示します。
# File: .github/workflows/phpunit.yml
name: PHPUnit Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up PHP
uses: shivammathur/setup-php@v2
with:
php-version: '8.0'
- name: Install dependencies
run: composer install
- name: Run PHPUnit
run: vendor/bin/phpunit
この設定は、新しいコードがプッシュされたりプルリクエストが行われたりするたびにユニットテストを実行します。これらの段階でテストを実行することで、新しい変更がリグレッションを引き起こしたり、既存の機能を壊したりしないことを保証し、開発全体を通してコードの品質を維持するのに役立ちます。パイプラインに Cypress テストを含めることで、バックエンドのロジックとフロントエンドのインタラクションを自動的に検証できます。
結論
WordPress のワークフローに自動テストを導入することは、コードの品質と信頼性を向上させるための決定的な一歩です。しかし、開発者はテスト環境の設定、依存関係の管理、説得力のあるテストケースの作成といった課題に直面することがよくあります。これらの課題を克服するには
- 入念な計画。
- 環境設定のベストプラクティスの使用
- 地域資源を活用して共通の問題を解決
始めるのは大変に思えるかもしれませんが、長期的なメリット - バグの減少、メンテナンスの容易さ、デプロイの迅速化 - を考えれば、それだけの価値はあります。PHPUnit や Cypress のようなツールを使えば、 さまざまな側面からテストを行うことができ、 開発者やユーザーがスムーズにテストを行えるようになります。JavaScript コンポーネントをテストする Jest や、 より包括的なブラウザ自動化テストを行う Selenium などのツールも検討する価値があります。
しっかりとしたテストスイートを作成し、CI/CDパイプラインに統合することで、すべてのコードが徹底的にチェックされていることを認識しながら、自信を持って機能をリリースすることができます。自動テストは単なる空想的なツールではなく、最新の開発ワークフローのバックボーンなのです。