How to Enable Multilingual Support for REST API Endpoints with WordPress rest_api_init
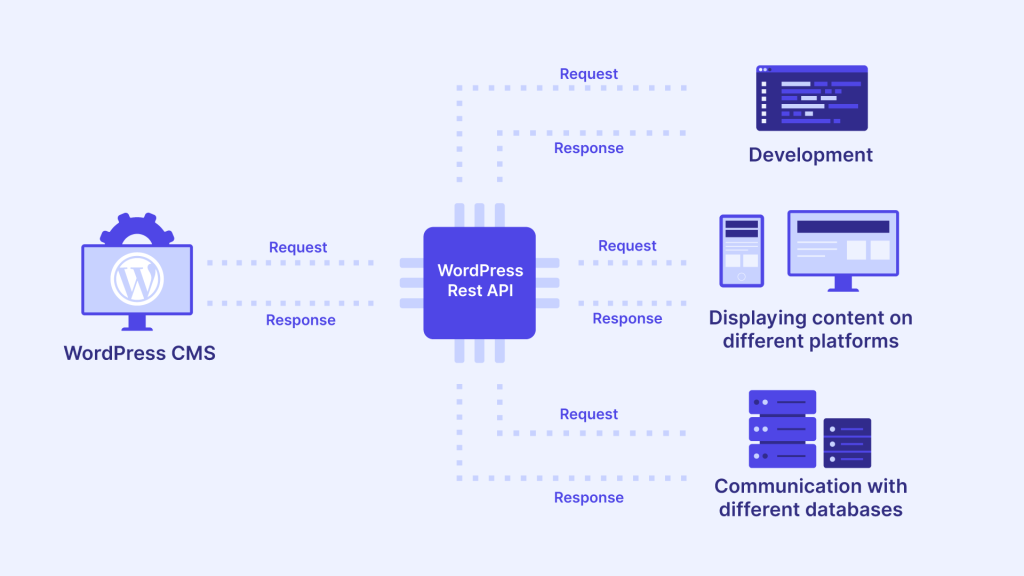
If you’ve ever tried to make your WordPress REST API endpoints multilingual and found yourself stuck with “rest_api_init not working” issues, you’re not alone. Implementing multilingual support can be challenging, especially when it involves customizing core functionalities like the REST API. In this article, we’ll explore in-depth how to use the rest_api_init
hook effectively to create REST API endpoints that support multiple languages.
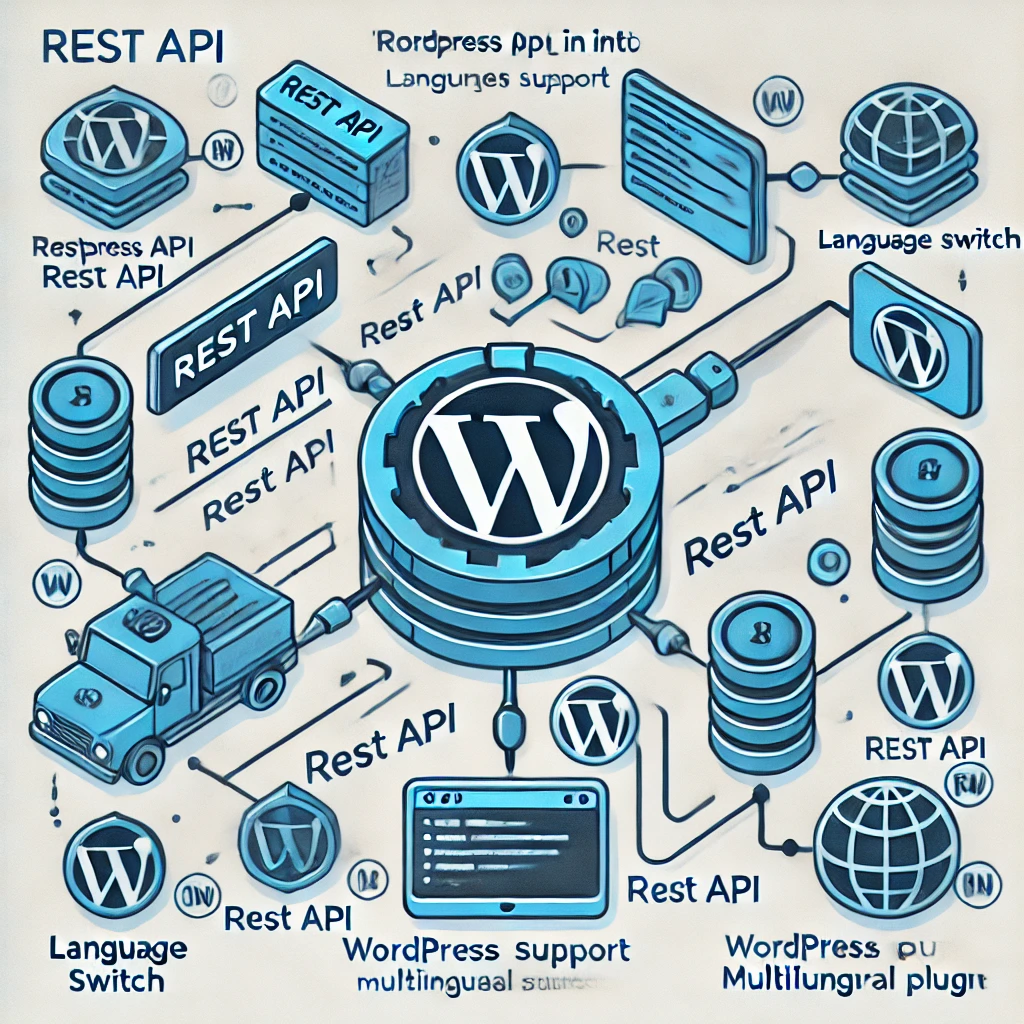
Understanding rest_api_init and Common Pitfalls
The rest_api_init
hook is an essential part of extending WordPress REST APIs. It allows developers to register custom routes and endpoints to suit the needs of their projects. However, many developers encounter the dreaded “rest_api_init not working” scenario, which usually happens due to misconfigurations, plugin conflicts, or incorrect hook placement.
To successfully use rest_api_init
for multilingual support, it is crucial to understand how to properly register routes, handle translation, and debug common issues. Before diving into the implementation, ensure your environment is set up correctly: confirm all plugins are up-to-date, and there are no other hooks overriding your endpoints.
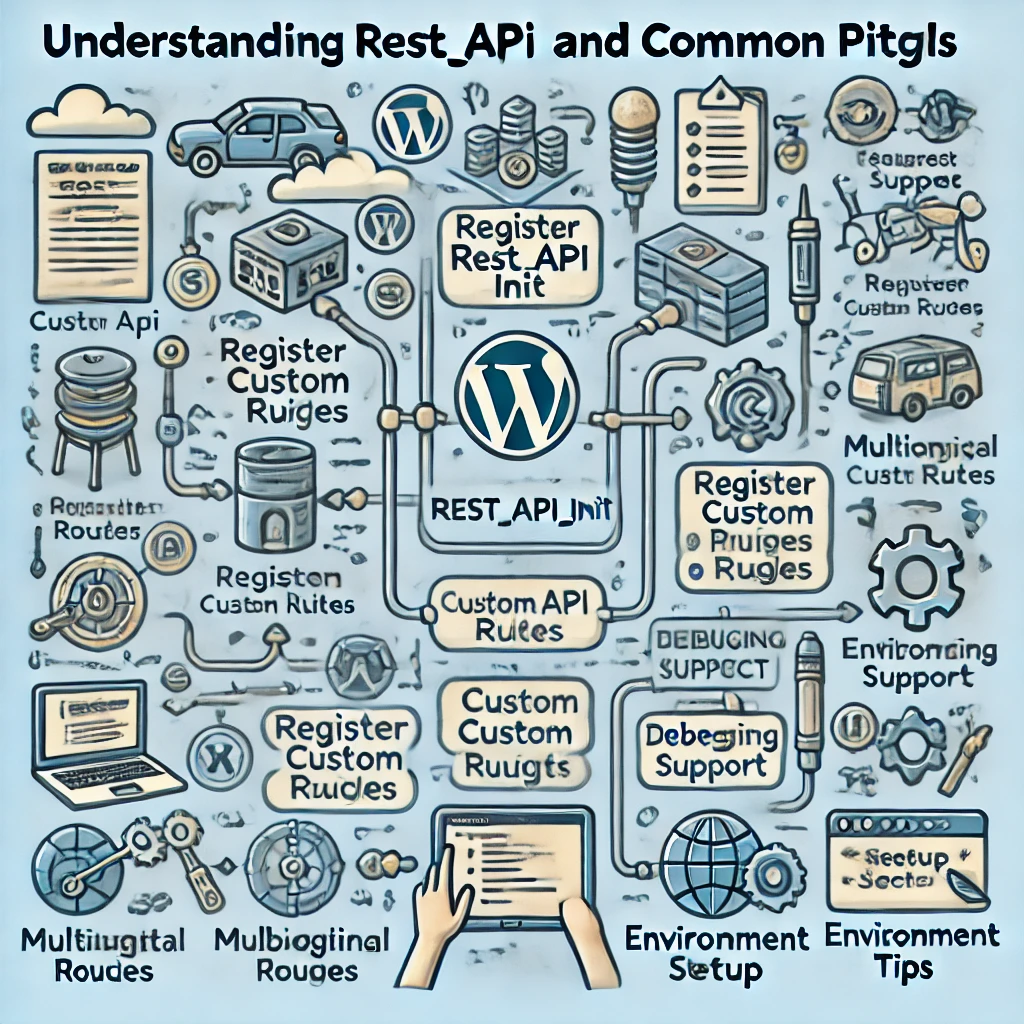
Step-by-Step Guide to Implementing Multilingual Endpoints
1. Registering Custom Endpoints with rest_api_init
To start, use the rest_api_init
action to register custom routes for your API. Place this code in your theme’s functions.php
file or in a custom plugin:
add_action('rest_api_init', 'register_multilingual_api_routes');
function register_multilingual_api_routes() {
register_rest_route('myplugin/v1', '/content/', array(
'methods' => 'GET',
'callback' => 'get_multilingual_content',
'permission_callback' => '__return_true', // Add permission callback to ensure security
));
}
This code creates a custom REST API endpoint at /wp-json/myplugin/v1/content/
. The permission_callback
parameter is important to ensure that unauthorized users do not access sensitive data. Now, we need to make it multilingual.
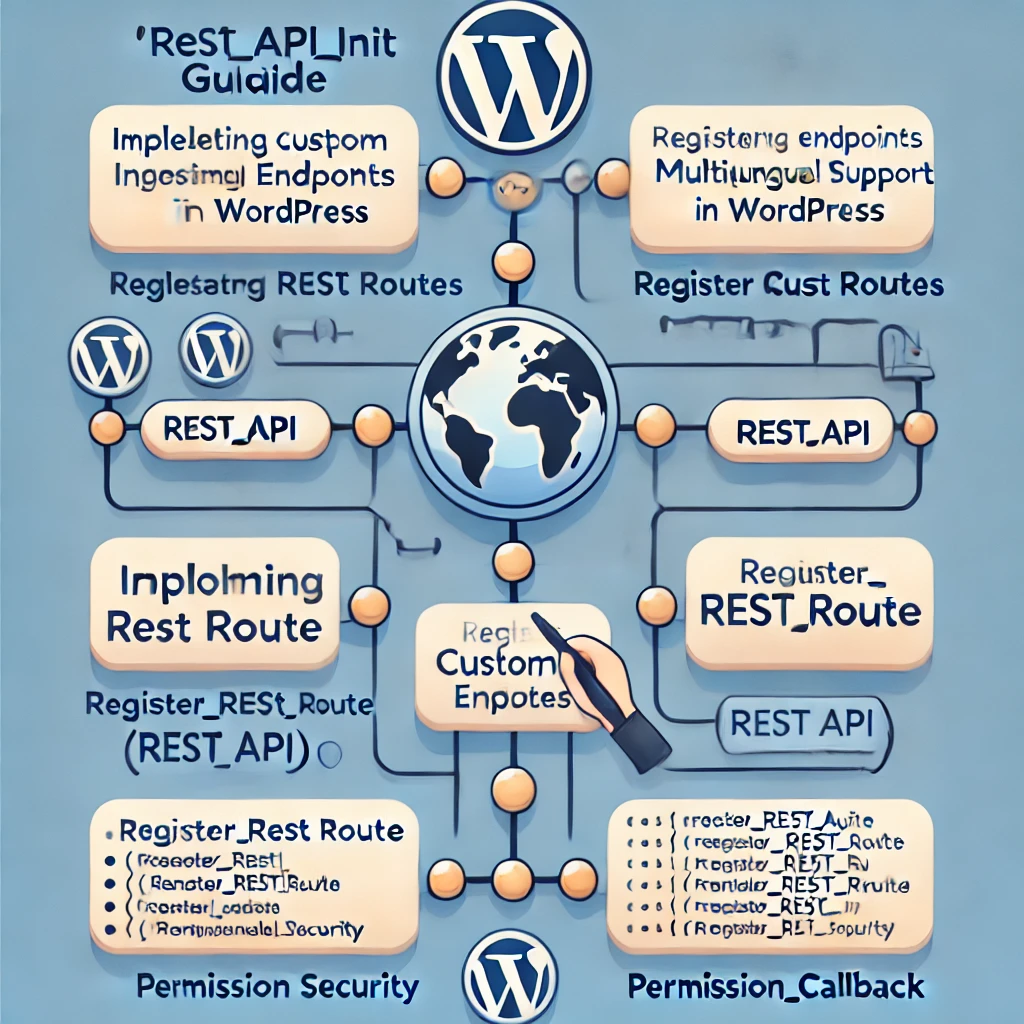
2. Adding Language Parameters
To make the API multilingual, we need to add a language parameter to the route. You can modify the callback to accept language arguments like this:
function get_multilingual_content($request) {
$language = $request->get_param('lang');
if (!$language) {
return new WP_Error('no_language', 'Language parameter is required', array('status' => 400));
}
// Fetch content based on the language parameter
$content = get_content_by_language($language);
if (empty($content)) {
return new WP_Error('no_content', 'No content found for the specified language', array('status' => 404));
}
return rest_ensure_response($content);
}
This implementation ensures that a language parameter (lang
) is required, and it returns language-specific content accordingly. This method enhances the flexibility of your API by allowing users to specify the language directly.
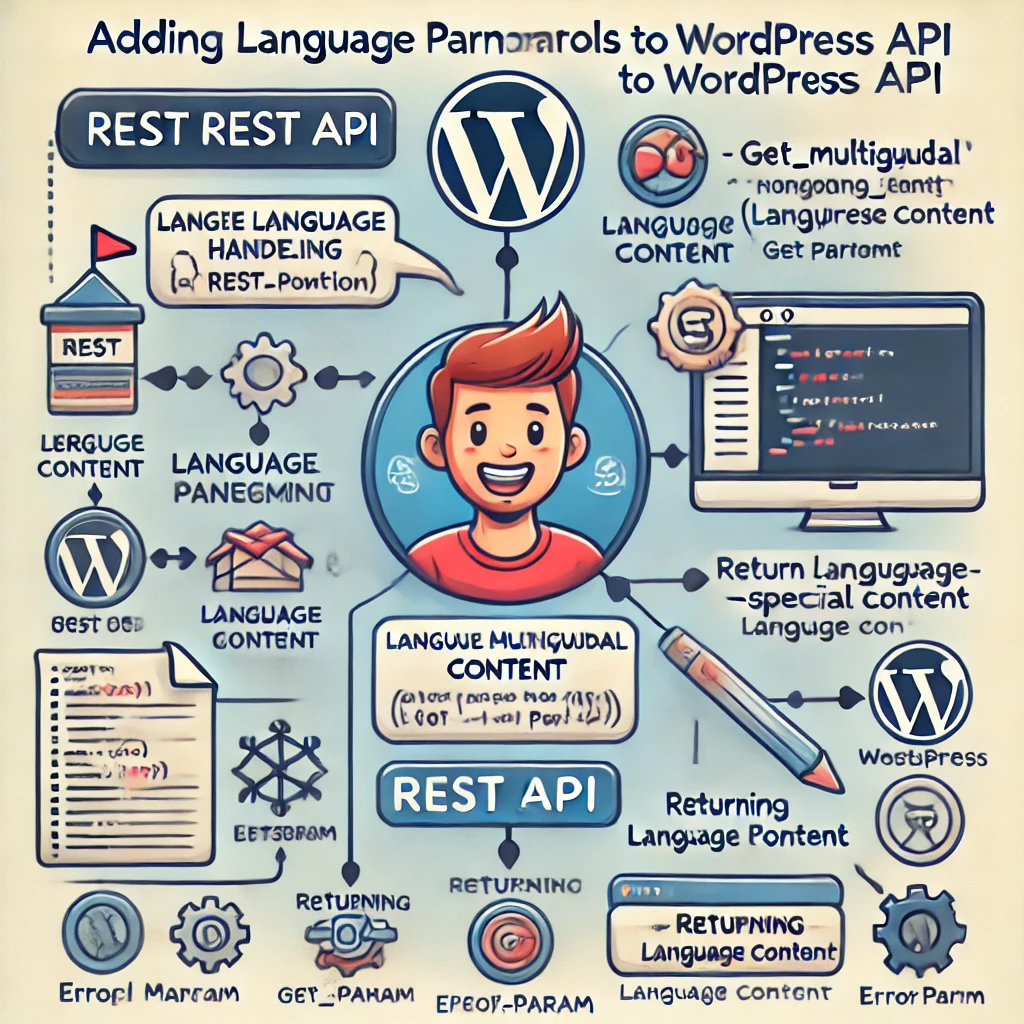
3. Handling Multilingual Content in WordPress
To actually serve multilingual content, you need to store and retrieve the data properly. You might use plugins like WPML or Polylang to manage translations. In the get_content_by_language
function, leverage these plugins to fetch the correct translation based on the lang
parameter.
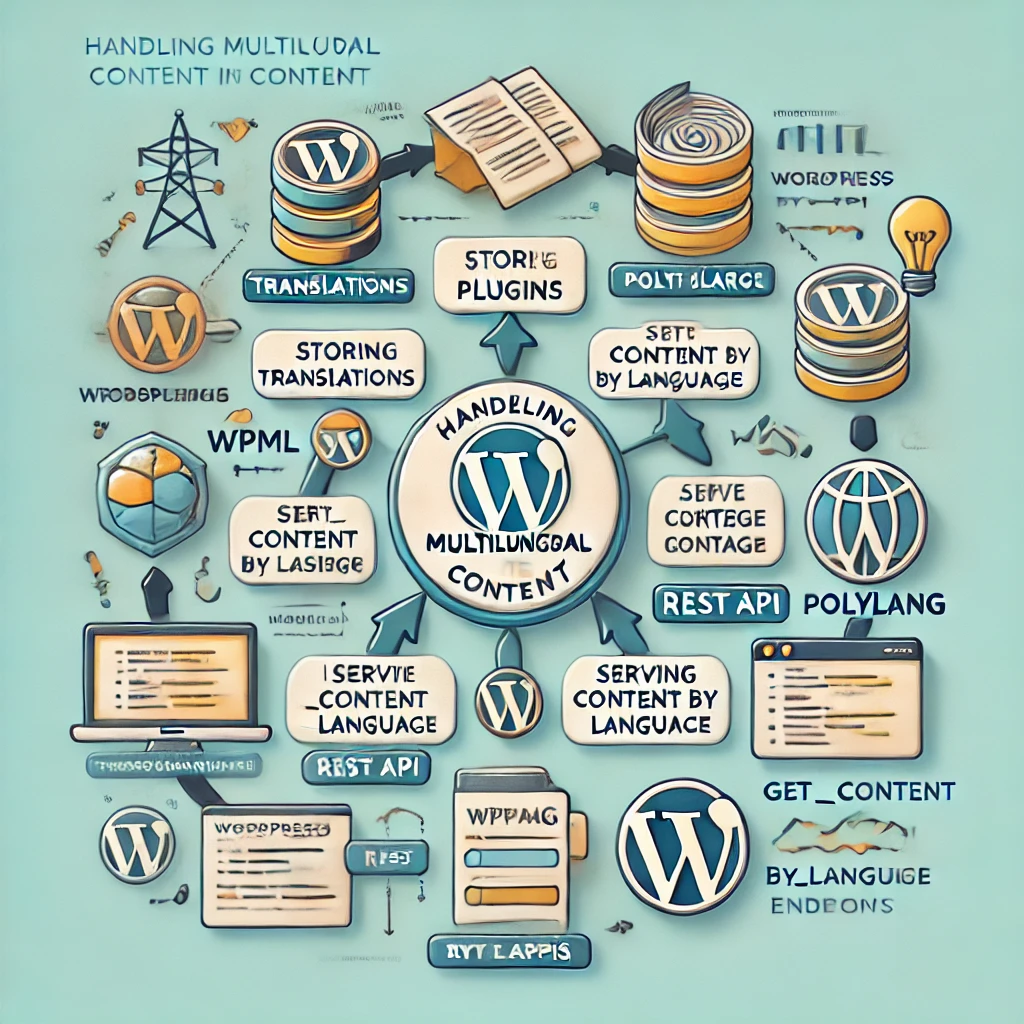
For example:
function get_content_by_language($language) {
// Assume WPML is being used
global $sitepress;
$sitepress->switch_lang($language);
// Query translated content
$args = array(
'post_type' => 'post',
'posts_per_page' => 5
);
$query = new WP_Query($args);
return $query->posts;
}
This example uses WPML’s switch_lang
method to set the language context before running the query, ensuring the returned content matches the specified language. You can adapt this method based on the plugin or translation approach you are using.
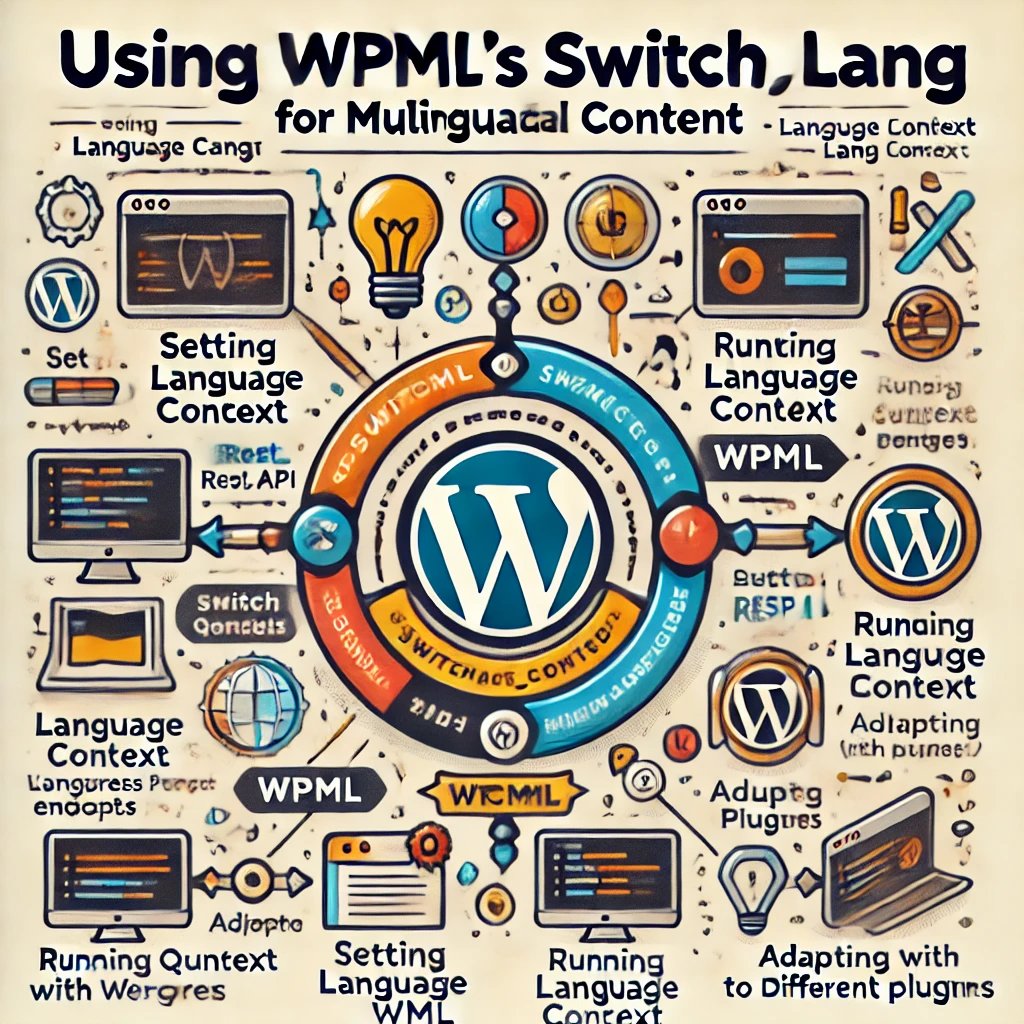
Advanced Techniques for Multilingual REST APIs
1. Caching for Performance
Handling multilingual content dynamically can lead to performance issues, especially if your site has a lot of traffic. Implementing caching for your REST API responses is highly recommended. You can use plugins like WP REST Cache to store API responses or utilize a custom caching mechanism to avoid repeatedly querying translated content.
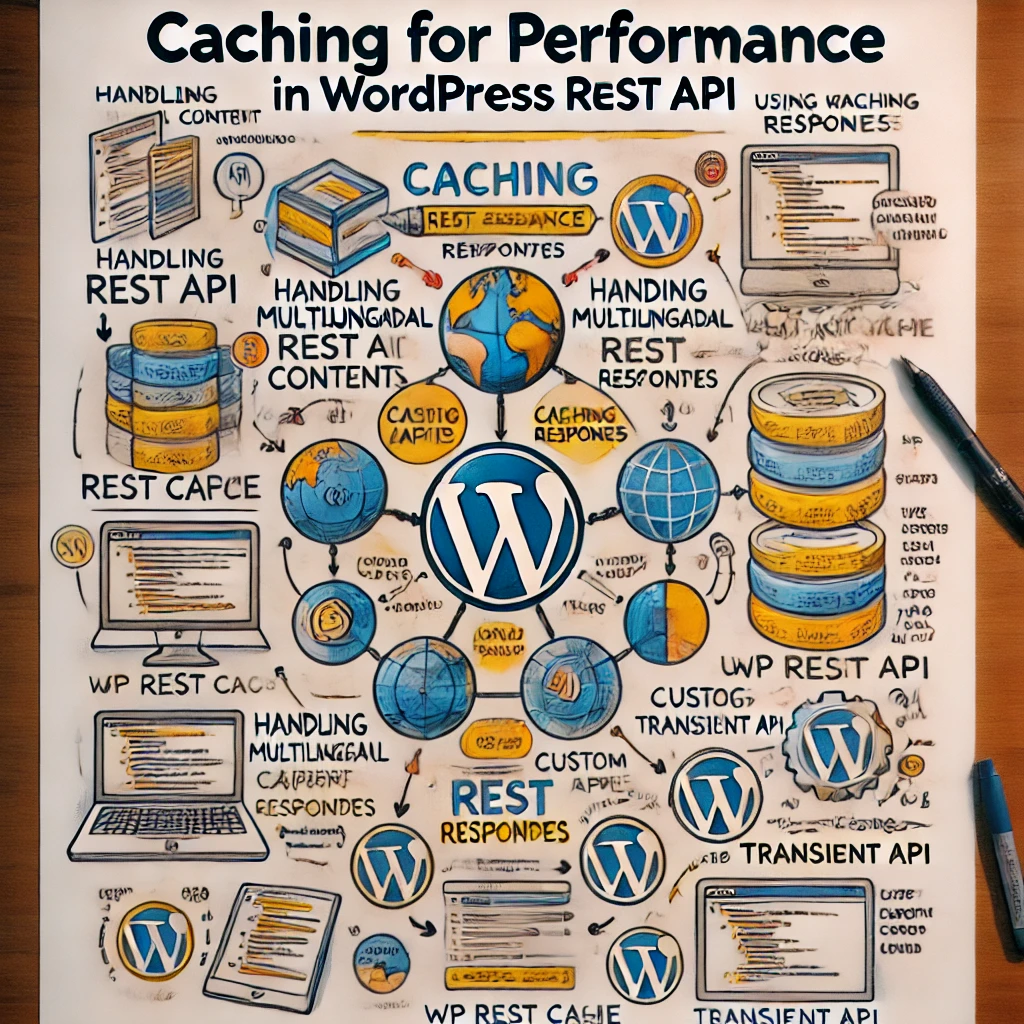
For instance, you can leverage transient API in WordPress to cache the response:
function get_multilingual_content($request) {
$language = $request->get_param('lang');
$cache_key = 'multilingual_content_' . $language;
$cached_content = get_transient($cache_key);
if ($cached_content) {
return rest_ensure_response($cached_content);
}
// Fetch content based on the language parameter
$content = get_content_by_language($language);
if (empty($content)) {
return new WP_Error('no_content', 'No content found for the specified language', array('status' => 404));
}
// Store the content in transient cache for 12 hours
set_transient($cache_key, $content, 12 * HOUR_IN_SECONDS);
return rest_ensure_response($content);
}
This ensures that only valid languages are processed, improving both security and usability.
3. Structuring the Response for Localization
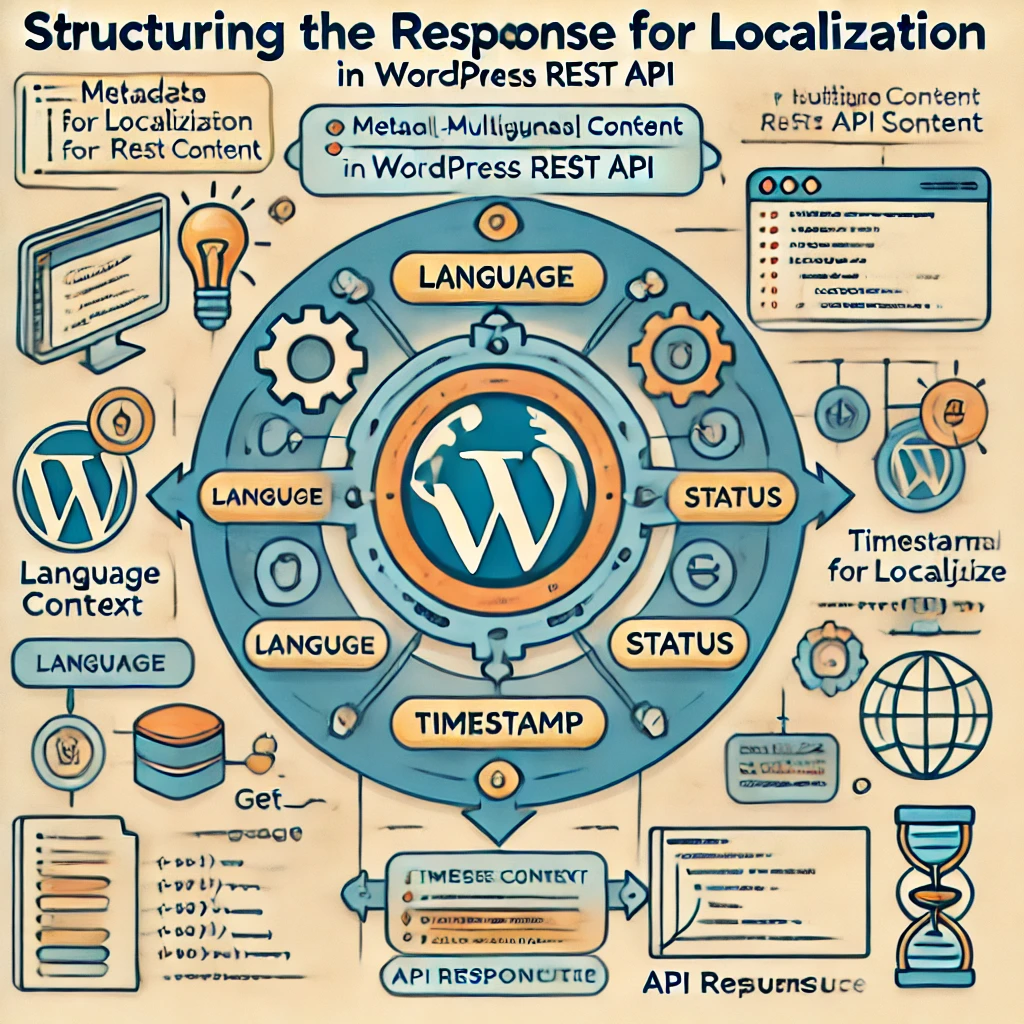
Consider structuring your API response to include metadata about the language and any other relevant information, which can be particularly useful for frontend applications consuming your API.
function get_multilingual_content($request) {
$language = $request->get_param('lang');
$content = get_content_by_language($language);
if (empty($content)) {
return new WP_Error('no_content', 'No content found for the specified language', array('status' => 404));
}
$response = array(
'language' => $language,
'content' => $content,
'timestamp' => current_time('mysql'),
'status' => 'success'
);
return rest_ensure_response($response);
}
Adding metadata like the language, timestamp, and status helps consumers of the API (such as JavaScript frontend frameworks) to understand the context of the data.
Plugin Integration for Multilingual Support
WPML and Polylang Integration
If you’re using WPML or Polylang, integrating them with your REST API can simplify handling multilingual content. Here’s how you can effectively use WPML with your custom API:
- WPML Integration: Use the
wpml_object_id_filter
to get translated versions of custom posts. For example:
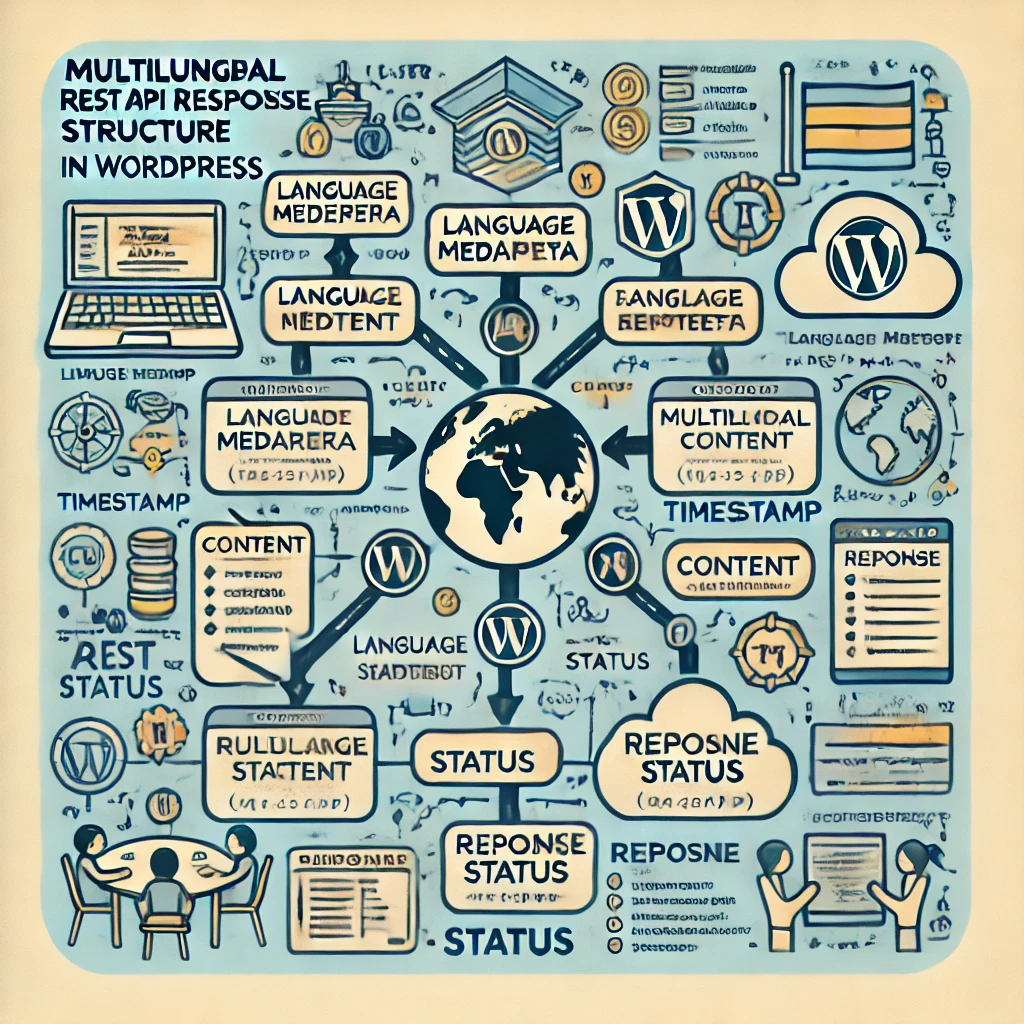
function get_content_by_language($language) {
global $sitepress;
$sitepress->switch_lang($language);
$args = array(
'post_type' => 'post',
'posts_per_page' => 5
);
$query = new WP_Query($args);
$posts = array();
foreach ($query->posts as $post) {
$translated_id = apply_filters('wpml_object_id', $post->ID, 'post', true, $language);
if ($translated_id) {
$posts[] = get_post($translated_id);
}
}
return $posts;
}
Polylang Integration: Use Polylang’s API to get content in the desired language:
function get_content_by_language($language) {
pll_set_language($language);
$args = array(
'post_type' => 'post',
'posts_per_page' => 5
);
$query = new WP_Query($args);
return $query->posts;
}
These integrations ensure that your content is properly translated and served based on the requested language.
Troubleshooting rest_api_init Not Working
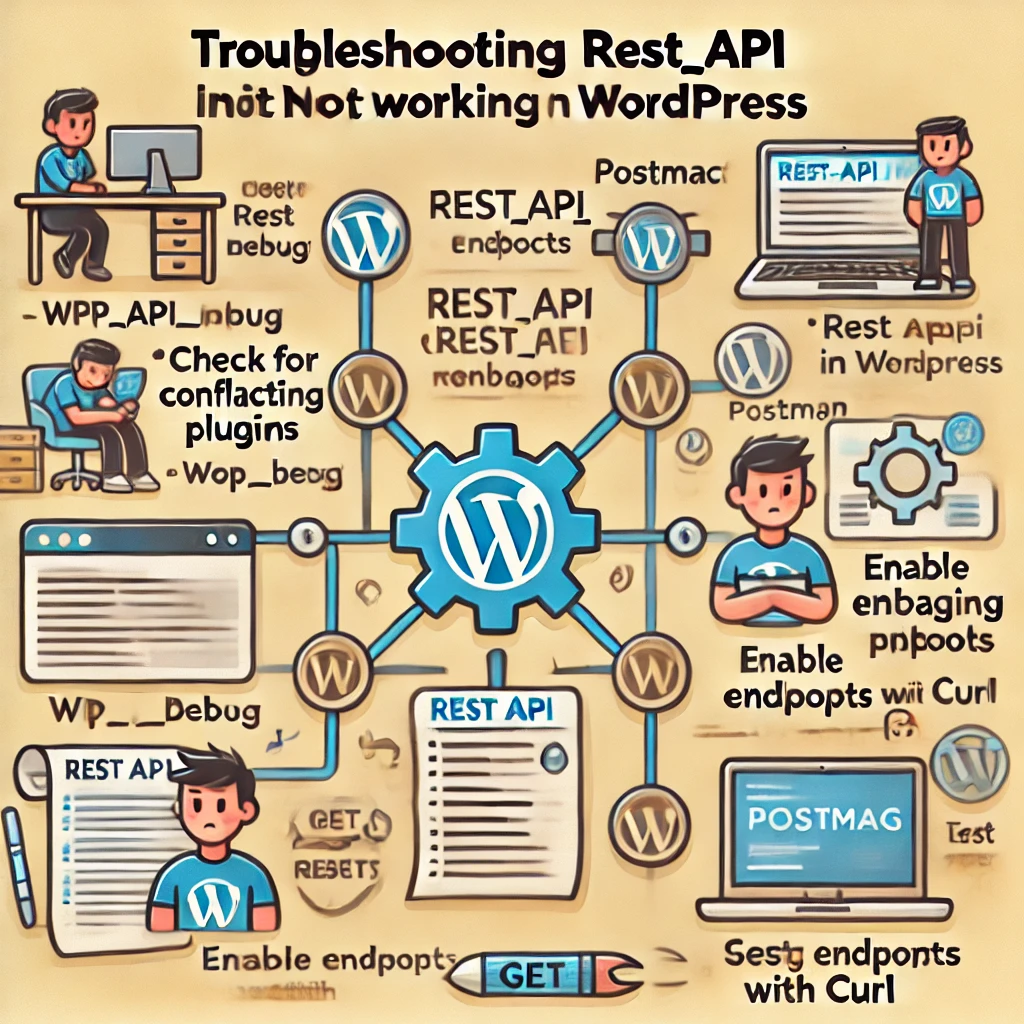
If you find that rest_api_init
isn’t working as expected, consider the following detailed solutions:
- Check for Conflicting Plugins: Plugins that also interact with the REST API can create conflicts. Deactivate plugins one by one to identify the culprit. It’s also helpful to review each plugin’s documentation to see if they modify or restrict the REST API.
- Enable Debugging Tools: Enable debugging in WordPress (
define('WP_DEBUG', true)
) and use tools like Postman or cURL to test your endpoints and validate responses. For example, use cURL to send a GET request:
curl -X GET "http://yourdomain.com/wp-json/myplugin/v1/content?lang=en"
curl -X GET "http://yourdomain.com/wp-json/myplugin/v1/content?lang=en"
These tools help ensure that your endpoints are functioning as expected and provide detailed error responses.- Correct Hook Placement: Ensure that
rest_api_init
is being called at the right time, i.e., when all other dependencies are loaded. Incorrect placement can prevent the routes from being registered properly. It’s advisable to use this hook in a plugin orfunctions.php
of your theme but not before all plugins have initialized. - Permalinks Settings: Sometimes, permalink structures are the root cause of failing routes. Go to Settings > Permalinks and re-save the settings to refresh the permalink configuration.
Security Considerations
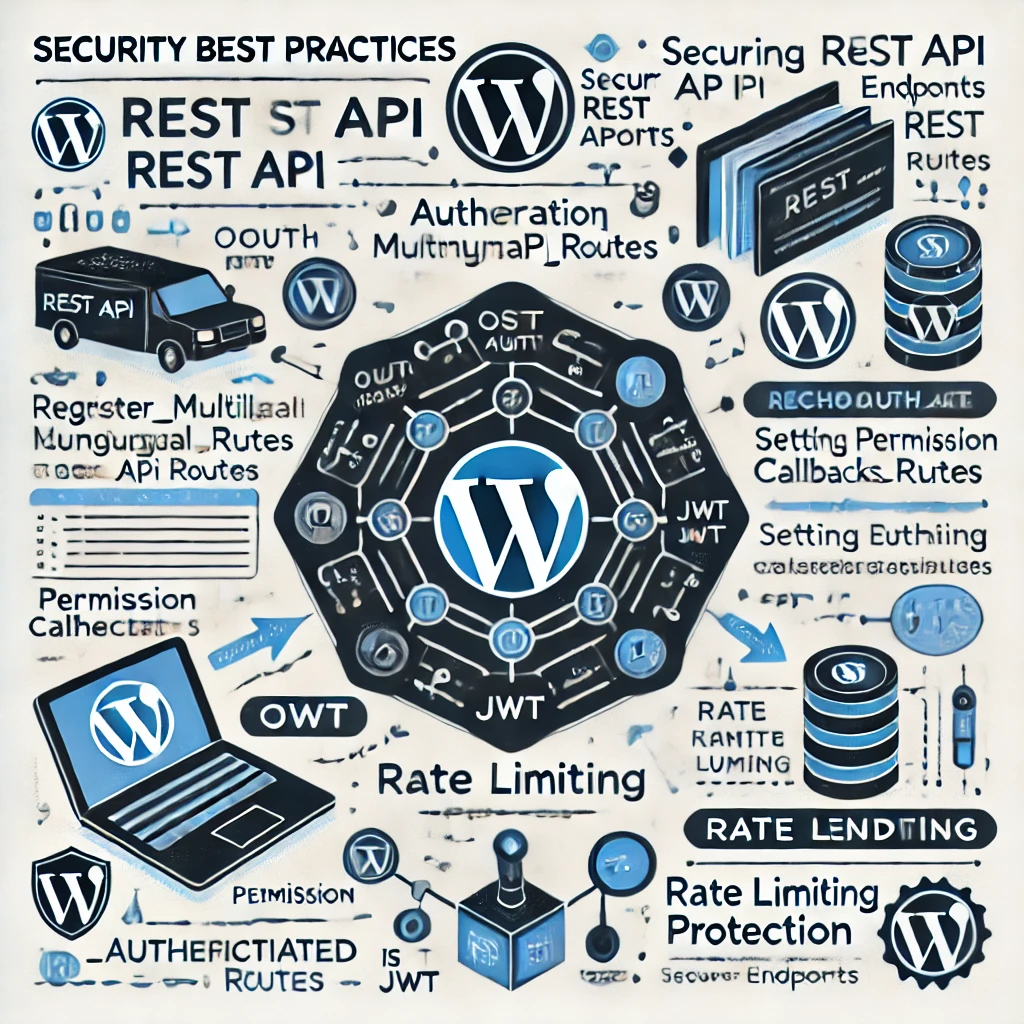
To ensure the security of your REST API endpoints, you should implement proper authentication and authorization:
- Authentication with OAuth or JWT: Consider using OAuth or JSON Web Tokens (JWT) for secure access. These methods provide a more robust way to authenticate requests compared to relying solely on the
permission_callback
. For example, using the JWT Authentication for WP REST API plugin allows you to validate requests with a token.
function register_multilingual_api_routes() {
register_rest_route('myplugin/v1', '/content/', array(
'methods' => 'GET',
'callback' => 'get_multilingual_content',
'permission_callback' => 'is_authenticated_user'
));
}
function is_authenticated_user() {
return is_user_logged_in(); // Example check, replace with JWT validation if needed
}
- Rate Limiting: To protect your API from abuse, consider implementing rate limiting. You could use plugins like Limit Login Attempts Reloaded to prevent brute force attacks or create a custom rate limiter for API requests.
Conclusion
Creating multilingual REST API endpoints with rest_api_init
in WordPress requires a deep understanding of route registration, parameter handling, translation plugins, caching, and security measures. By following best practices—like properly adding language parameters, handling errors robustly, caching for performance, and securing endpoints—you can build powerful multilingual REST APIs that meet the needs of your project.
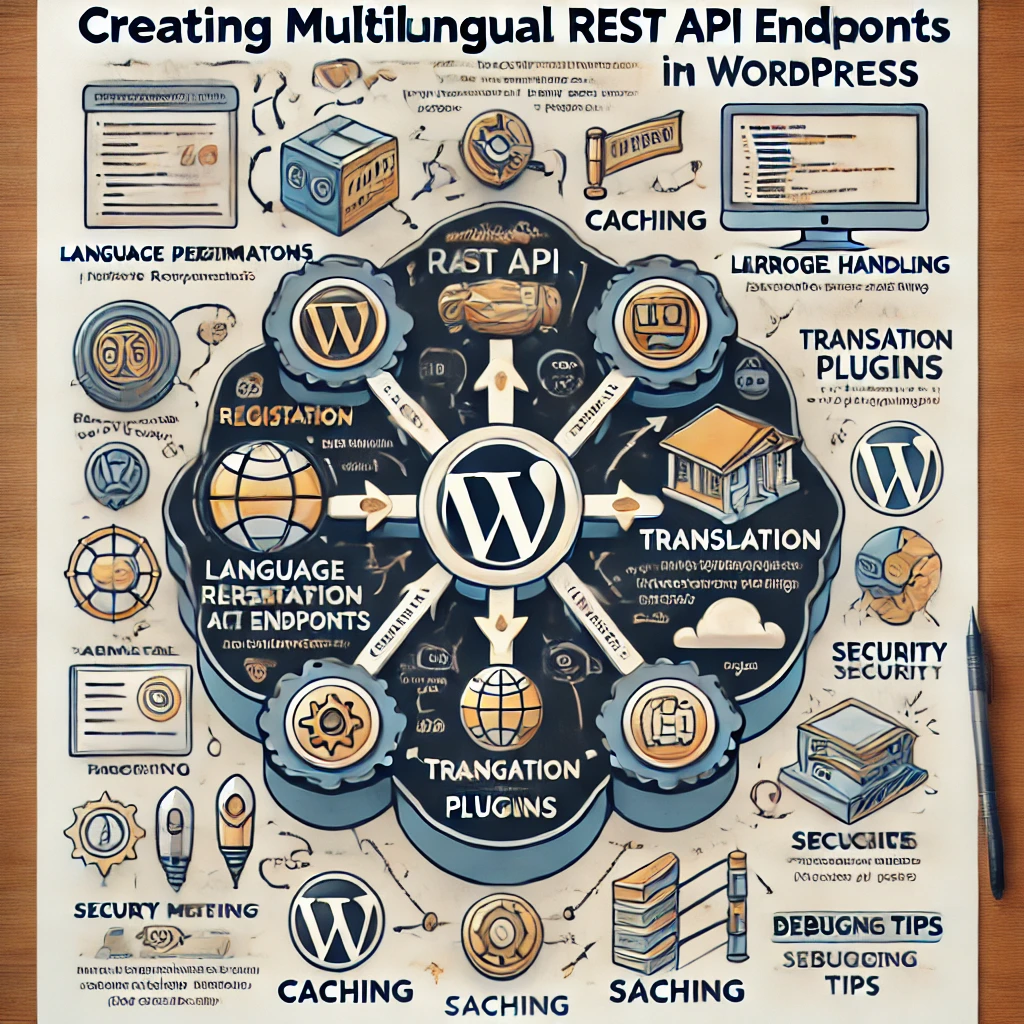
If “rest_api_init not working” has been your frustration, this guide aims to provide the depth needed to address common issues and ensure smooth operation. Remember, API development is as much about understanding the infrastructure as it is about writing code, and taking the time to debug effectively will pay off in building resilient and feature-rich solutions.
Do you have any questions or need further guidance on implementing multilingual support for your WordPress REST API? Feel free to leave a comment below or reach out for more detailed troubleshooting.
Responses