Why Is rest_api_init Not Working in My Custom WordPress Plugin?
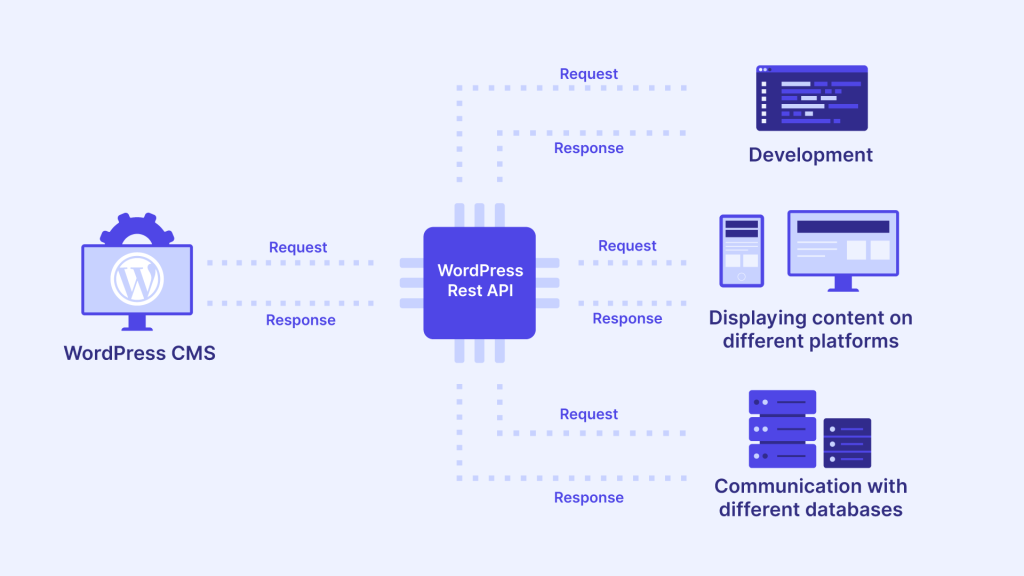
Encountering issues with rest_api_init
not working in your custom WordPress plugin can be quite frustrating, especially when it seems like everything should be configured correctly. In this article, we will explore the possible reasons why rest_api_init
might not be working, examine common pitfalls, and discuss best practices to ensure your custom REST API routes work seamlessly. This is a technical deep dive designed for developers looking to build robust custom APIs in WordPress.
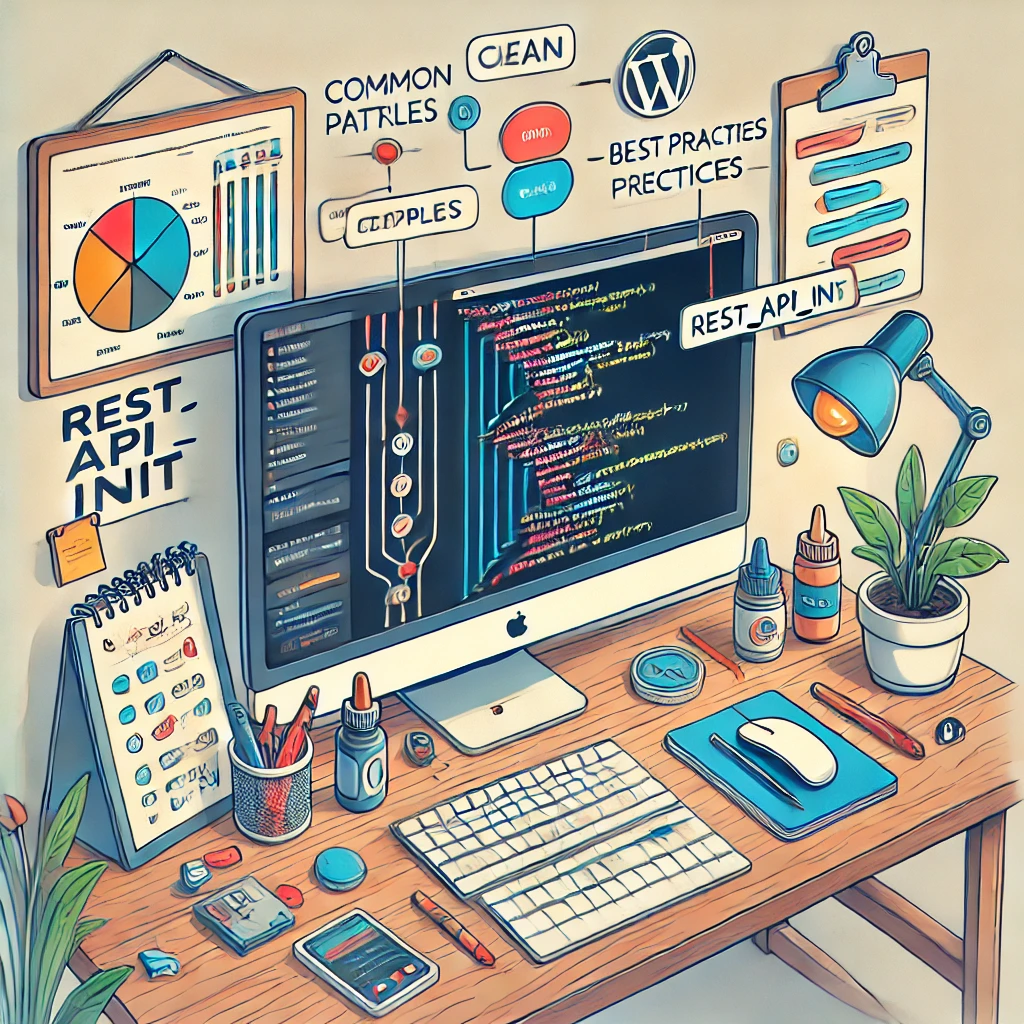
Understanding rest_api_init and Its Role in WordPress
The rest_api_init
action hook is used to add custom routes and endpoints to the WordPress REST API. It runs when the REST API server is initialized and is typically used to register new REST API routes. If your custom endpoint is not functioning as expected, it’s often due to a misconfiguration or improper use of rest_api_init
. Understanding the hook’s lifecycle and its role in WordPress is essential to successfully extending the API.
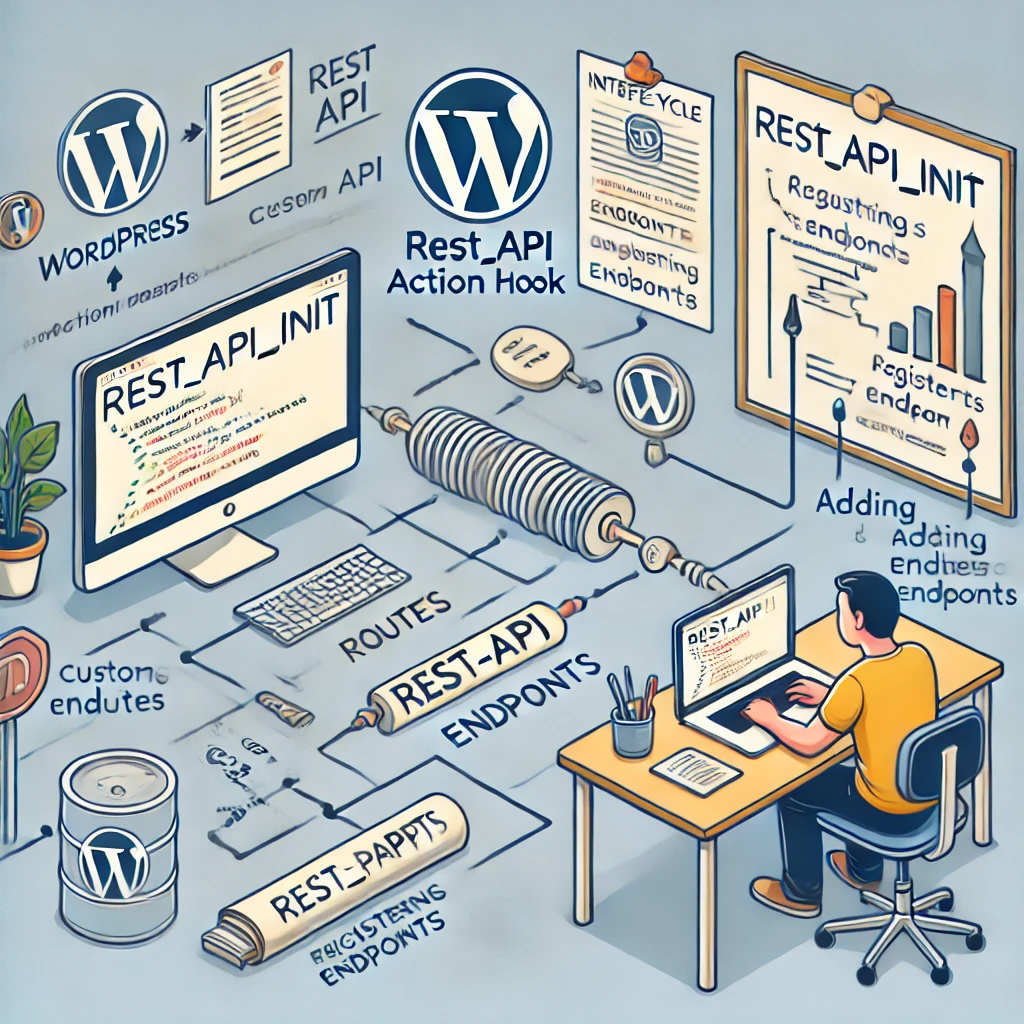
Common Reasons Why rest_api_init Is Not Working
Let’s break down the most common reasons why rest_api_init
might not be working in your custom WordPress plugin and provide practical solutions to each issue.
1. Hook Placement and Timing Issues
The most common reason for rest_api_init
not working is incorrect hook placement. The rest_api_init
action hook needs to be called after WordPress has initialized all of its components. Typically, you should add this action in the main plugin file or in a separate initialization function, ensuring it is called after WordPress core and any dependent plugins have fully loaded.
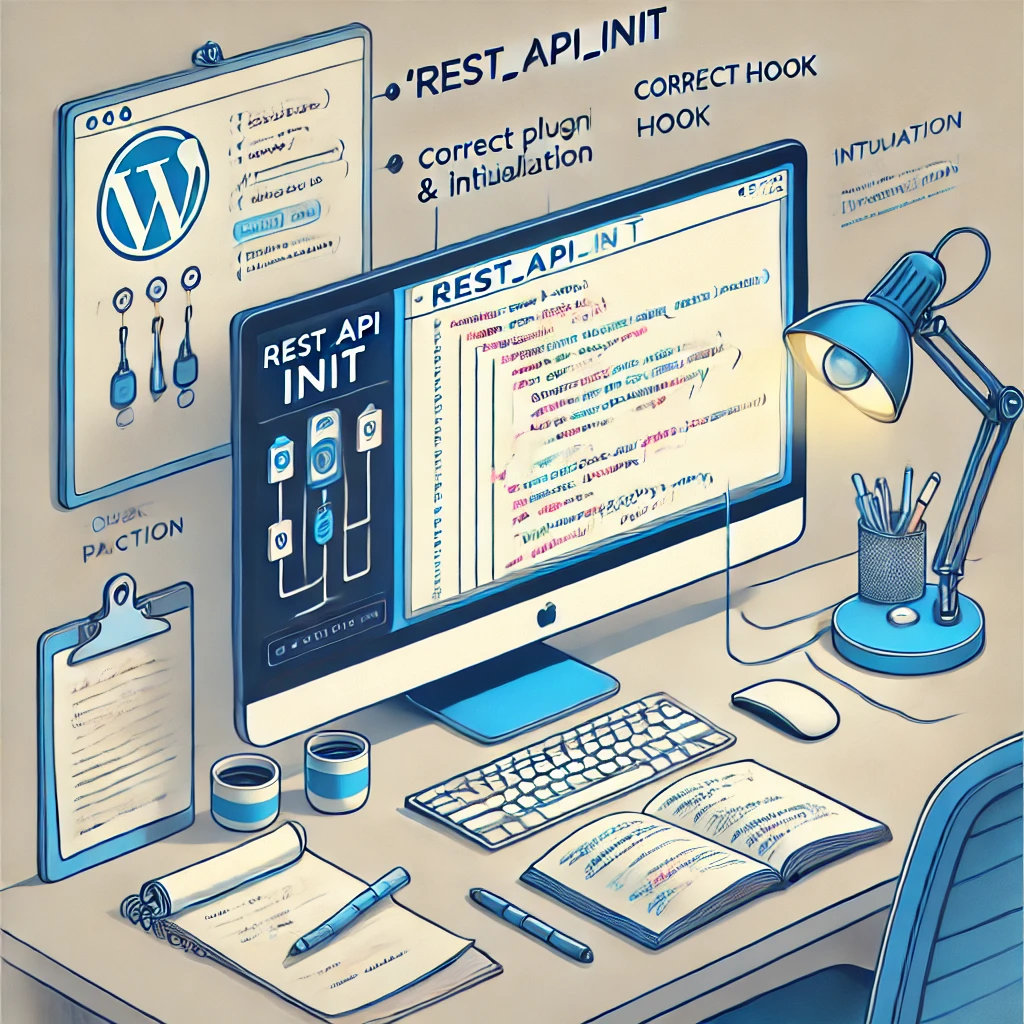
Solution: Make sure you’re registering your custom route using rest_api_init
in the correct context:
add_action('rest_api_init', 'register_custom_api_routes');
function register_custom_api_routes() {
register_rest_route('myplugin/v1', '/content/', array(
'methods' => 'GET',
'callback' => 'get_custom_content',
'permission_callback' => '__return_true', // Set permissions appropriately
));
}
Explanation:
add_action('rest_api_init', 'register_custom_api_routes');
– This line hooks intorest_api_init
to register our custom routes once the REST API is initialized.register_rest_route('myplugin/v1', '/content/', ...)
– This function registers a new REST API route. The namespace ('myplugin/v1'
) helps to uniquely identify the route to avoid conflicts with other plugins.'permission_callback' => '__return_true'
– This callback grants permission to anyone accessing the endpoint. For real applications, replace this with proper user capability checks to ensure security.
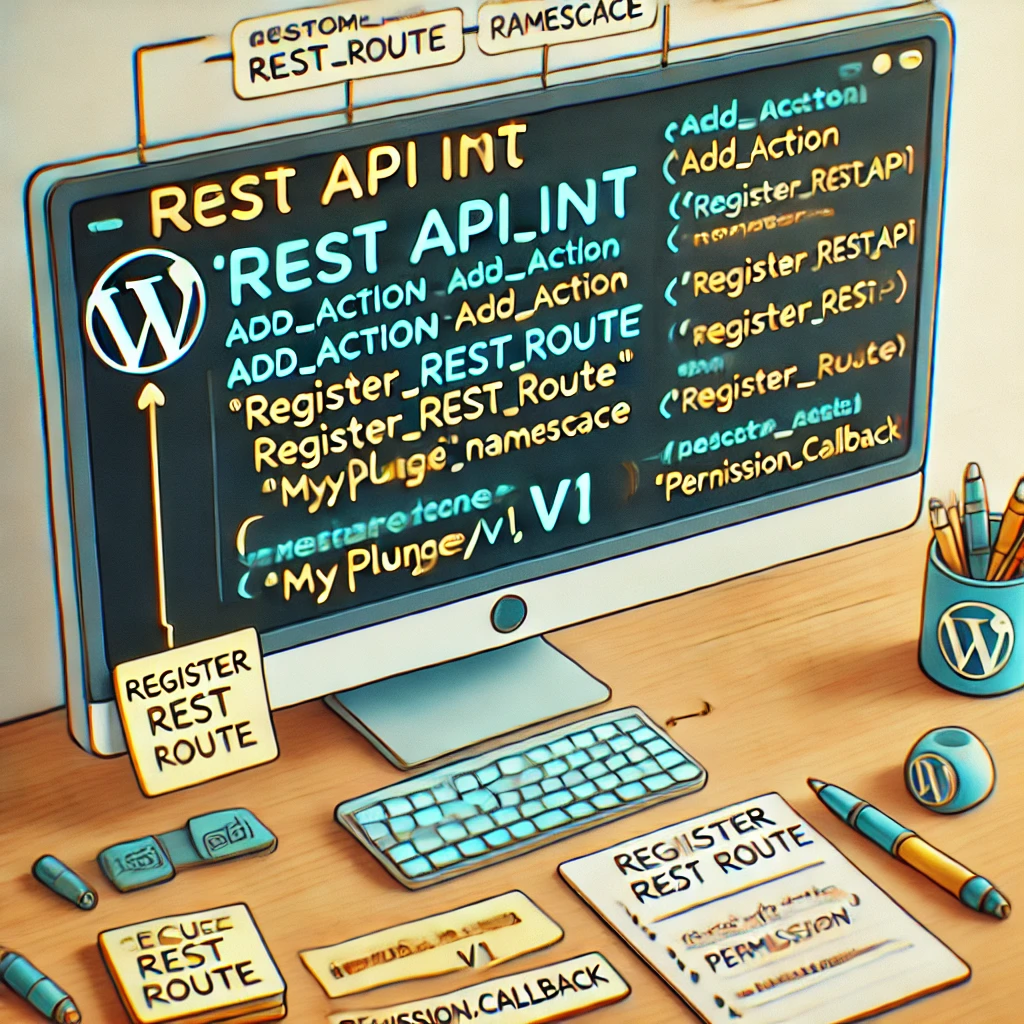
Place this code either in the main plugin file or within a function that runs after all plugins are loaded, such as plugins_loaded
. Incorrect hook placement may lead to the route not being registered correctly.
2. Conflicting Plugins or Themes
Another common reason for rest_api_init
not working could be conflicts with other plugins or themes that also register routes. If two plugins attempt to register the same endpoint or if a theme has a similar route, conflicts can arise, preventing your custom endpoint from functioning properly.
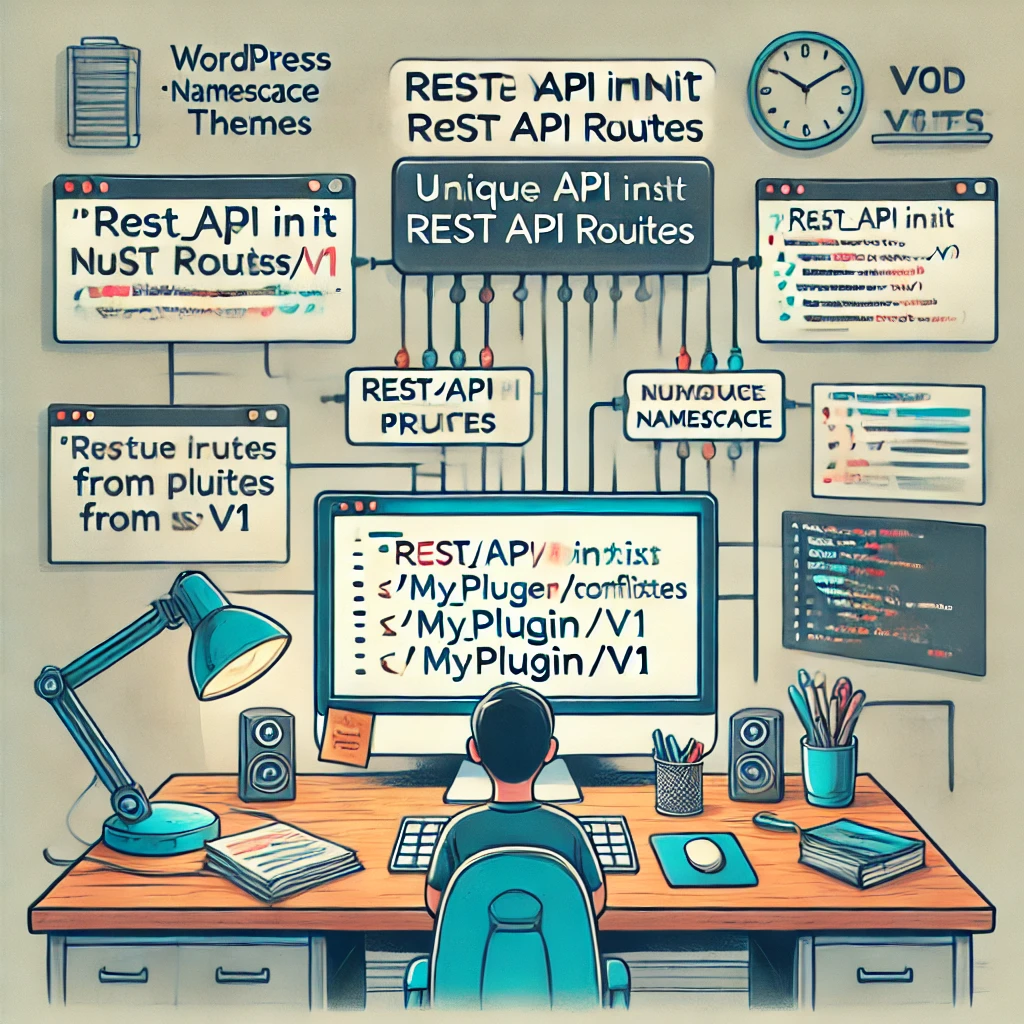
Solution: Use unique namespace prefixes to avoid conflicts. Namespaces in REST API routes are intended to prevent such collisions. For example:
register_rest_route('myplugin/v1', '/custom-content/', array(
'methods' => 'GET',
'callback' => 'get_custom_content',
'permission_callback' => '__return_true',
));
Explanation:
- The
'myplugin/v1'
namespace helps uniquely identify the route to prevent naming conflicts. - Testing with conflicting plugins disabled can help isolate whether a specific plugin is causing issues.
3. Permalink Structure Not Set Properly
The WordPress REST API relies on the permalink structure to function correctly. If your site is using plain permalinks, the REST API routes may not be available or may not work as expected.
Solution: Update your permalink structure by navigating to Settings > Permalinks in the WordPress admin panel. Choose any structure other than “Plain” and save the changes. This refreshes the rewrite rules and ensures that your REST API routes are accessible.
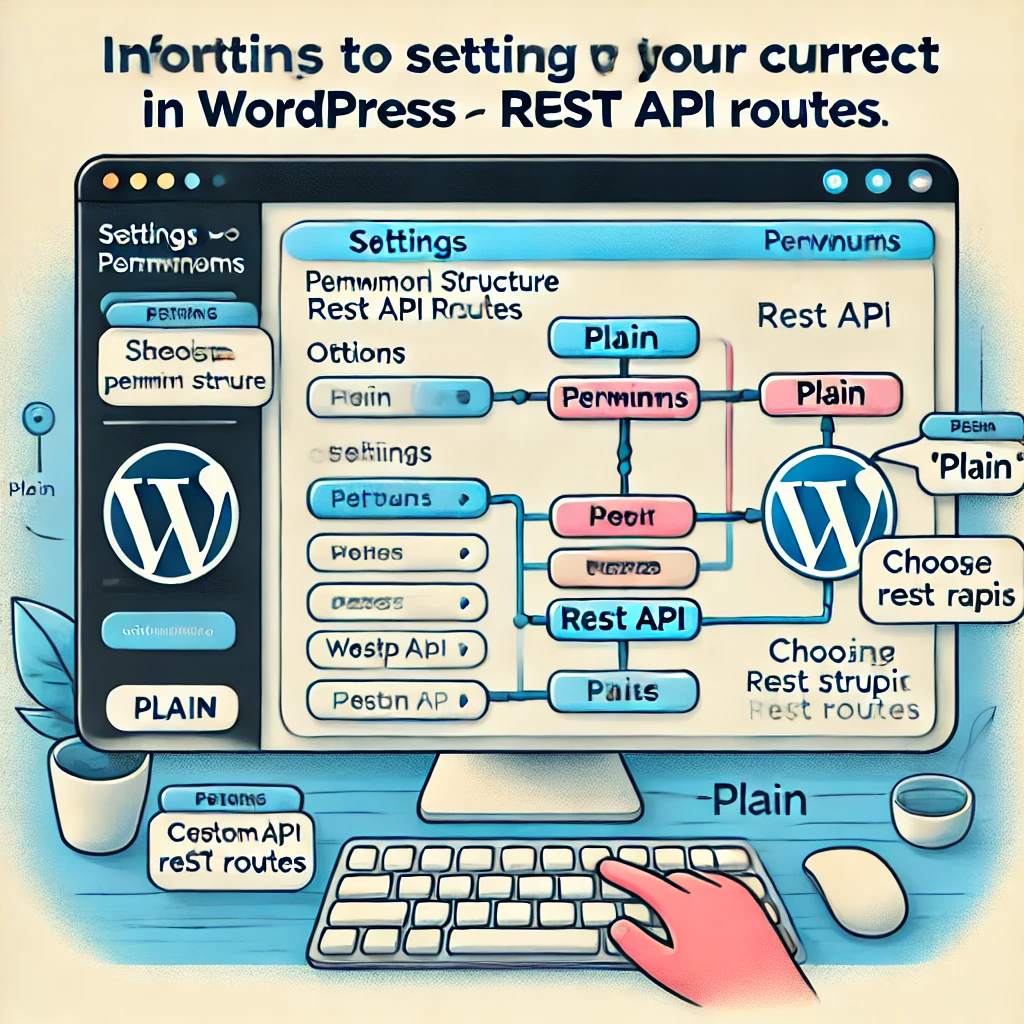
4. Caching Issues
Caching plugins or server-level caching can interfere with the proper functioning of REST API endpoints. When caching is enabled, changes to your routes might not be reflected immediately, leading to the perception that rest_api_init
is not working.
Solution: Clear any caching mechanisms, both server-side (such as Varnish or Nginx cache) and WordPress-level caching plugins. In development environments, consider disabling caching altogether to avoid issues during development and testing.
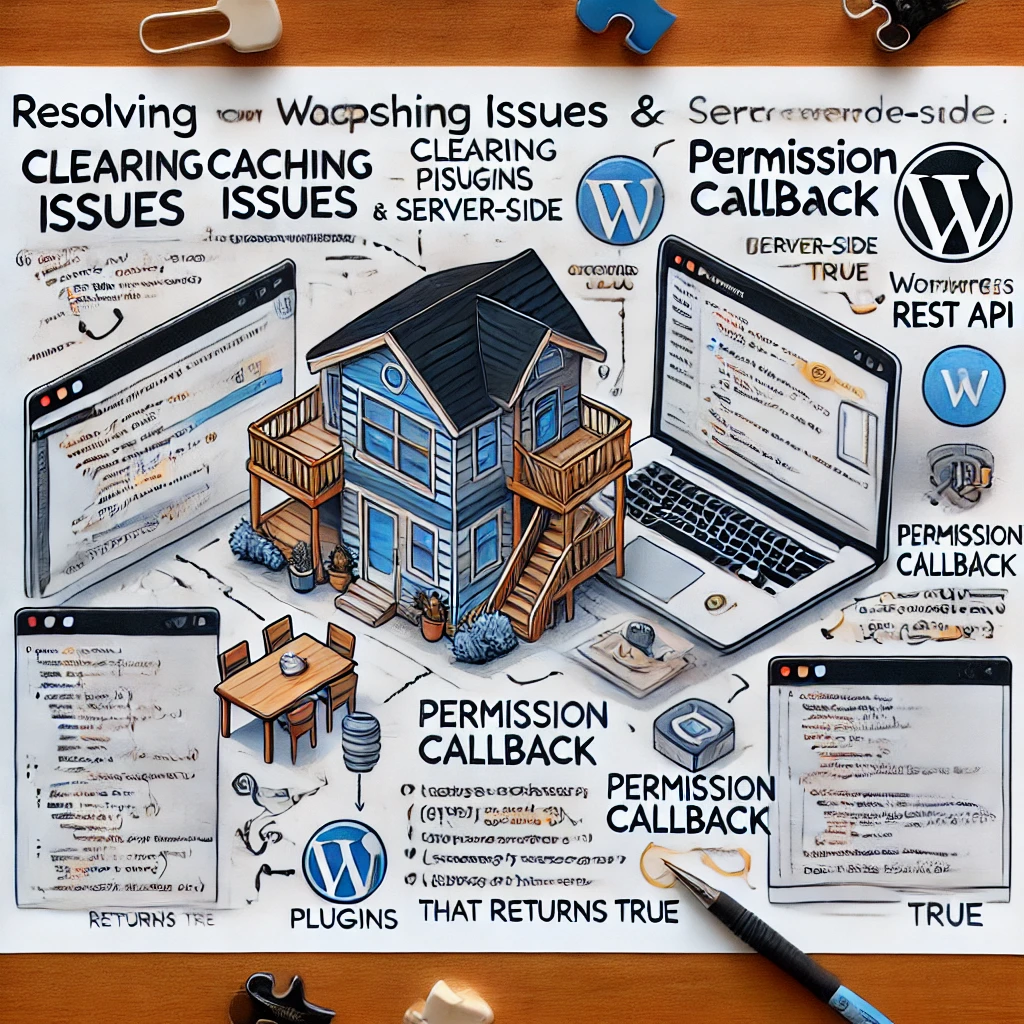
5. Incorrect Permission Callback
The permission_callback
parameter is used to determine whether a user can access the REST API endpoint. If this callback function returns false or encounters an error, the endpoint won’t be accessible, giving the impression that rest_api_init
isn’t working.
Solution: Verify that the permission_callback
function is correctly implemented and returns true
if access is allowed. For example:
function get_custom_content_permission() {
return current_user_can('read'); // Adjust the capability as needed
}
add_action('rest_api_init', 'register_custom_api_routes');
function register_custom_api_routes() {
register_rest_route('myplugin/v1', '/custom-content/', array(
'methods' => 'GET',
'callback' => 'get_custom_content',
'permission_callback' => 'get_custom_content_permission',
));
}
Explanation:
current_user_can('read')
checks whether the current user has the appropriate permissions. Adjust the capability as necessary for your endpoint.- If users without the required permissions try to access the endpoint, they will receive an error, which helps secure your API.
6. Debugging Tools and Techniques
Debugging REST API issues can be challenging without proper tools. Here are some ways to debug why rest_api_init
might not be working:
- WordPress Debug Mode: Enable WordPress debug mode by adding
define('WP_DEBUG', true);
in yourwp-config.php
file. This helps you identify errors in your plugin code that might prevent therest_api_init
from firing correctly. - Use Postman for Testing: Postman is an excellent tool for testing REST API endpoints. To test your custom route, send a GET request to your endpoint URL (e.g.,
http://yourdomain.com/wp-json/myplugin/v1/custom-content
). This helps you see if the endpoint is accessible and if it returns the expected data.- Step-by-Step Postman Testing:
- Open Postman and create a new GET request.
- Enter your API endpoint URL (e.g.,
http://yourdomain.com/wp-json/myplugin/v1/custom-content
). - Click “Send” to initiate the request.
- Observe the response status code and data. A 200 OK status indicates success, while a 404 or 403 indicates issues such as route not found or permissions error.
- If your endpoint requires authentication, include necessary headers such as an authorization token to access restricted routes.
- Step-by-Step Postman Testing:
- WordPress REST API Console Plugin: The REST API Console plugin can be used to interact with your WordPress REST API directly from the admin panel. This helps in real-time debugging and checking if your custom routes are registered correctly.
- cURL for Command Line Testing: You can also use cURL from the command line to test your endpoints:
curl -X GET "http://yourdomain.com/wp-json/myplugin/v1/custom-content"
Explanation: This command sends a GET request to your endpoint. It allows you to verify if the route is accessible and troubleshoot any network-level issues. Pay attention to response headers and status codes.
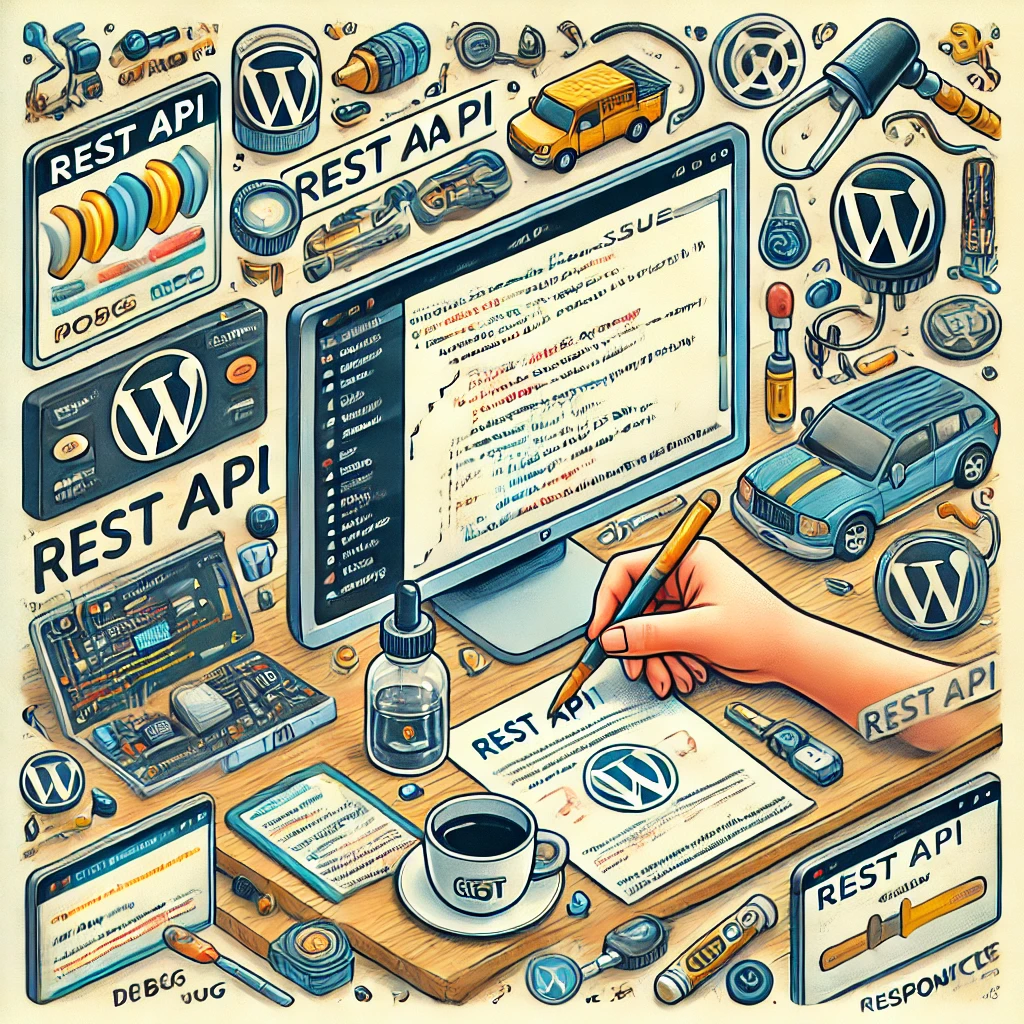
Detailed Plugin Integration Steps for WPML and Polylang
To make your custom REST API multilingual, you may be using plugins like WPML or Polylang. While these plugins offer comprehensive tools for translation, properly integrating them with custom REST API routes requires specific steps.
WPML Integration
- Install WPML Plugin: Install and activate the WPML plugin from the WordPress repository or your account on WPML’s website.
- Switch Language Context: Use
global $sitepress;
and$sitepress->switch_lang($language);
in your callback function to set the language context before querying content. - Register REST API Routes: Ensure that your route properly handles the
lang
parameter by dynamically switching languages before returning the content:function get_content_by_language($language) { global $sitepress; $sitepress->switch_lang($language); $args = array( 'post_type' => 'post', 'posts_per_page' => 5 ); $query = new WP_Query($args); return $query->posts; }
Explanation: This function switches the language context using WPML’s API before querying the posts. This ensures the content is fetched in the desired language.
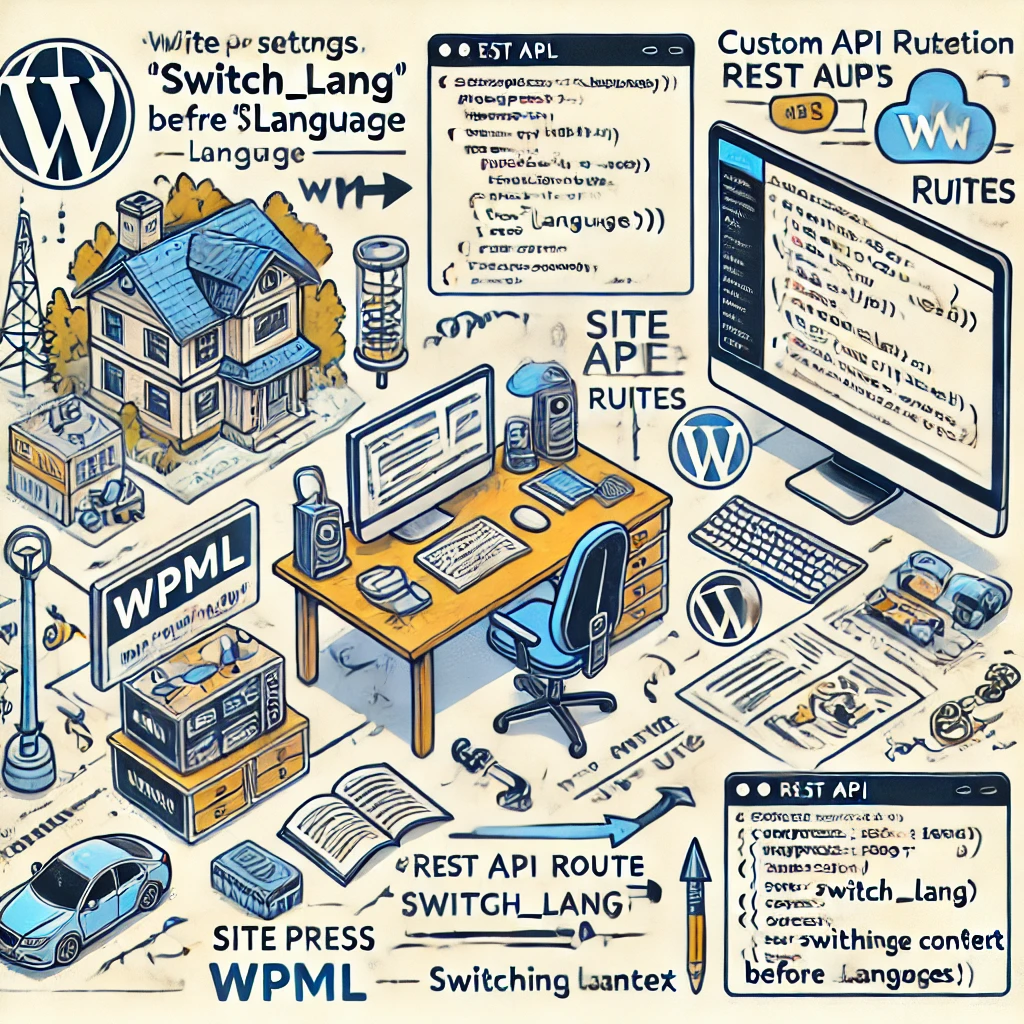
Polylang Integration
- Install Polylang: Install and activate the Polylang plugin.
- Set Language Using pll_set_language(): Use
pll_set_language($language);
to set the language context within your API callback function. - Modify Query to Reflect Language Context: Ensure that when querying content, the language is set to the desired value:
function get_content_by_language($language) { pll_set_language($language); $args = array( 'post_type' => 'post', 'posts_per_page' => 5 ); $query = new WP_Query($args); return $query->posts; }
Explanation: Thepll_set_language($language)
function sets the desired language for the content query, ensuring the correct version of the content is returned.
Real-World Example: Creating a Multilingual Blog API
Let’s create a simple multilingual blog API using rest_api_init
. This API will support querying posts in different languages using WPML.
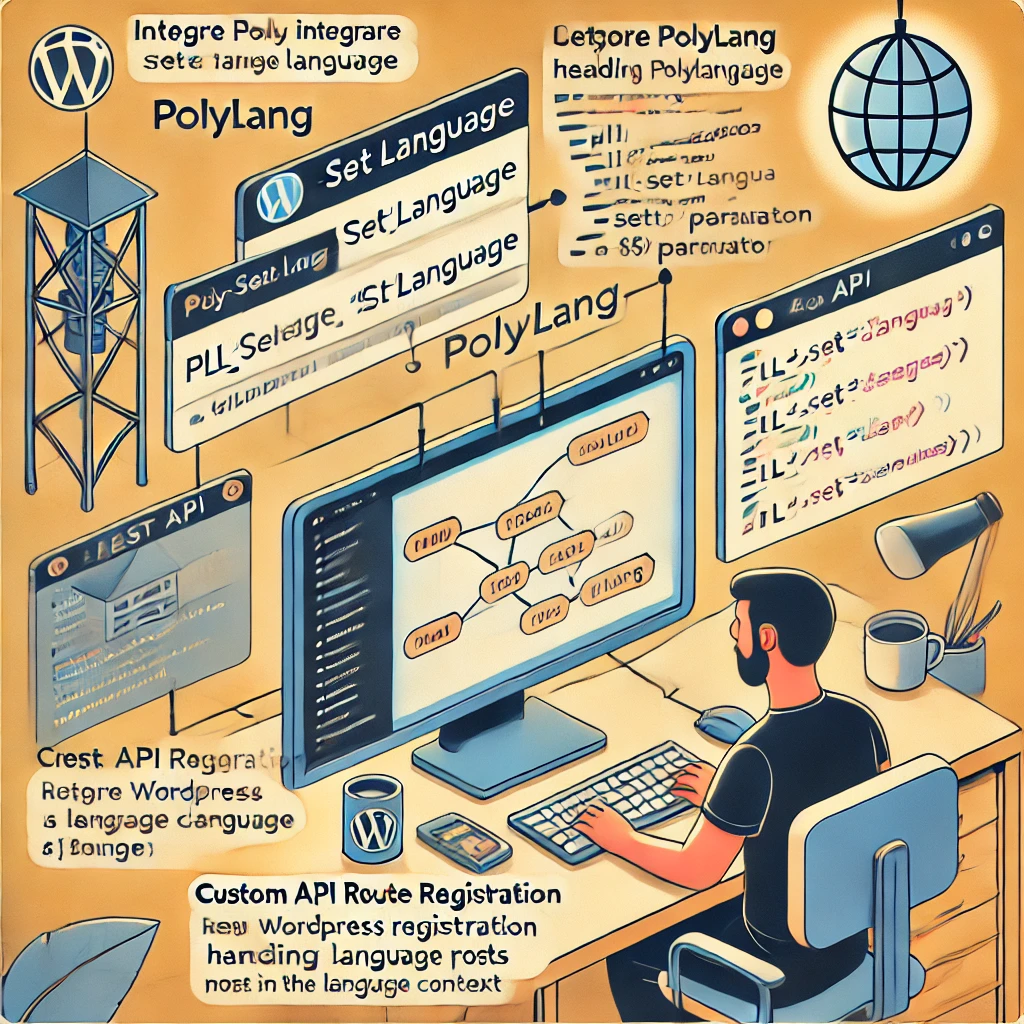
Step 1: Register the REST Route
add_action('rest_api_init', 'register_multilingual_blog_routes');
function register_multilingual_blog_routes() {
register_rest_route('myblog/v1', '/posts/', array(
'methods' => 'GET',
'callback' => 'get_blog_posts_by_language',
'permission_callback' => '__return_true',
));
}
Step 2: Define the Callback Function
function get_blog_posts_by_language($request) {
$language = $request->get_param('lang');
if (!$language) {
return new WP_Error('no_language', 'Language parameter is required', array('status' => 400));
}
global $sitepress;
$sitepress->switch_lang($language);
$args = array(
'post_type' => 'post',
'posts_per_page' => 5
);
$query = new WP_Query($args);
if (empty($query->posts)) {
return new WP_Error('no_posts', 'No posts found for the specified language', array('status' => 404));
}
return rest_ensure_response($query->posts);
}
Explanation:
- This example creates a REST API endpoint
/wp-json/myblog/v1/posts/
that accepts a language parameter (lang
). - The
get_blog_posts_by_language
function switches the language context using WPML and queries posts accordingly. - Proper error handling is included to ensure the endpoint returns meaningful responses if no language or posts are found.
Conclusion
The rest_api_init
hook is a powerful tool for extending the WordPress REST API, but its proper implementation requires careful consideration of timing, conflicts, permissions, and debugging techniques. By ensuring your hook is placed correctly, managing potential conflicts, understanding the role of permalinks, and utilizing robust debugging methods, you can ensure your custom REST API endpoints work effectively.
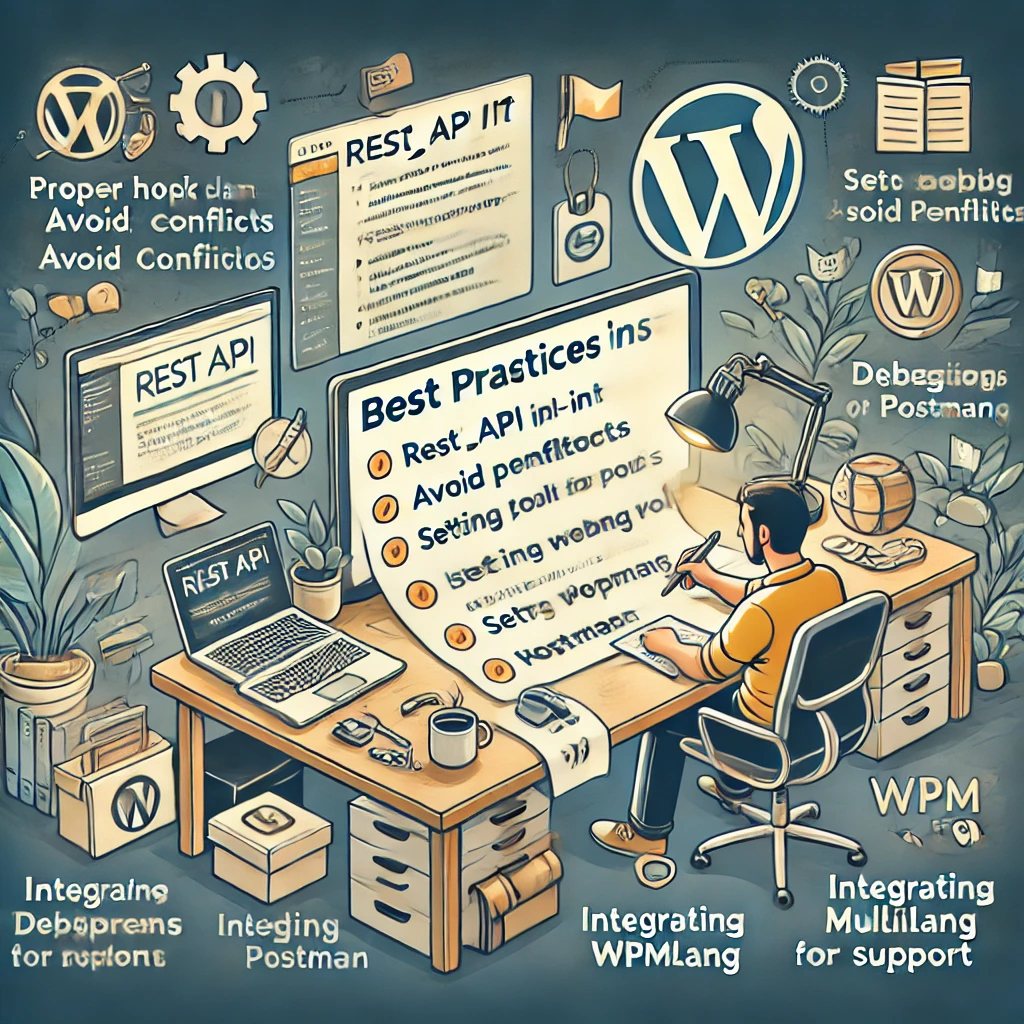
Additionally, integrating multilingual plugins like WPML and Polylang into your custom API requires specific attention to detail to ensure the correct language context is applied. Following these best practices and troubleshooting techniques will save you time and headaches when developing custom WordPress plugins that extend the REST API.
If you’re still struggling with rest_api_init
not working, consider systematically disabling plugins, using debugging tools like Postman or the REST API Console, and confirming that your environment meets all the necessary requirements for REST API development.
Feel free to leave a comment below if you have questions or need further assistance in getting your custom REST API routes working in WordPress.
Responses